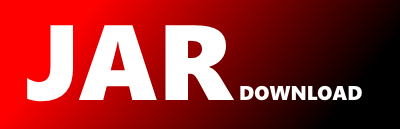
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265Settings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.outputs
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property adaptiveQuantization Enables or disables adaptive quantization.
* @property afdSignaling Indicates that AFD values will be written into the output stream.
* @property alternativeTransferFunction Whether or not EML should insert an Alternative Transfer Function SEI message.
* @property bitrate Average bitrate in bits/second.
* @property bufSize Size of buffer in bits.
* @property colorMetadata Includes color space metadata in the output.
* @property colorSpaceSettings Define the color metadata for the output. H265 Color Space Settings for more details.
* @property filterSettings Filters to apply to an encode. See H265 Filter Settings for more details.
* @property fixedAfd Four bit AFD value to write on all frames of video in the output stream.
* @property flickerAq
* @property framerateDenominator Framerate denominator.
* @property framerateNumerator Framerate numerator.
* @property gopClosedCadence Frequency of closed GOPs.
* @property gopSize GOP size in units of either frames of seconds per `gop_size_units`.
* @property gopSizeUnits Indicates if the `gop_size` is specified in frames or seconds.
* @property level H265 level.
* @property lookAheadRateControl Amount of lookahead.
* @property maxBitrate Set the maximum bitrate in order to accommodate expected spikes in the complexity of the video.
* @property minIInterval Min interval.
* @property minQp Set the minimum QP.
* @property mvOverPictureBoundaries Enables or disables motion vector over picture boundaries.
* @property mvTemporalPredictor Enables or disables the motion vector temporal predictor.
* @property parDenominator Pixel Aspect Ratio denominator.
* @property parNumerator Pixel Aspect Ratio numerator.
* @property profile H265 profile.
* @property qvbrQualityLevel Controls the target quality for the video encode.
* @property rateControlMode Rate control mode.
* @property scanType Sets the scan type of the output.
* @property sceneChangeDetect Scene change detection.
* @property slices Number of slices per picture.
* @property tier Set the H265 tier in the output.
* @property tileHeight Sets the height of tiles.
* @property tilePadding Enables or disables padding of tiles.
* @property tileWidth Sets the width of tiles.
* @property timecodeBurninSettings Apply a burned in timecode. See H265 Timecode Burnin Settings for more details.
* @property timecodeInsertion Determines how timecodes should be inserted into the video elementary stream.
* @property treeblockSize Sets the size of the treeblock.
*/
public data class ChannelEncoderSettingsVideoDescriptionCodecSettingsH265Settings(
public val adaptiveQuantization: String? = null,
public val afdSignaling: String? = null,
public val alternativeTransferFunction: String? = null,
public val bitrate: Int,
public val bufSize: Int? = null,
public val colorMetadata: String? = null,
public val colorSpaceSettings: ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsColorSpaceSettings? = null,
public val filterSettings: ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsFilterSettings? = null,
public val fixedAfd: String? = null,
public val flickerAq: String? = null,
public val framerateDenominator: Int,
public val framerateNumerator: Int,
public val gopClosedCadence: Int? = null,
public val gopSize: Double? = null,
public val gopSizeUnits: String? = null,
public val level: String? = null,
public val lookAheadRateControl: String? = null,
public val maxBitrate: Int? = null,
public val minIInterval: Int? = null,
public val minQp: Int? = null,
public val mvOverPictureBoundaries: String? = null,
public val mvTemporalPredictor: String? = null,
public val parDenominator: Int? = null,
public val parNumerator: Int? = null,
public val profile: String? = null,
public val qvbrQualityLevel: Int? = null,
public val rateControlMode: String? = null,
public val scanType: String? = null,
public val sceneChangeDetect: String? = null,
public val slices: Int? = null,
public val tier: String? = null,
public val tileHeight: Int? = null,
public val tilePadding: String? = null,
public val tileWidth: Int? = null,
public val timecodeBurninSettings: ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsTimecodeBurninSettings? = null,
public val timecodeInsertion: String? = null,
public val treeblockSize: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265Settings): ChannelEncoderSettingsVideoDescriptionCodecSettingsH265Settings =
ChannelEncoderSettingsVideoDescriptionCodecSettingsH265Settings(
adaptiveQuantization = javaType.adaptiveQuantization().map({ args0 -> args0 }).orElse(null),
afdSignaling = javaType.afdSignaling().map({ args0 -> args0 }).orElse(null),
alternativeTransferFunction = javaType.alternativeTransferFunction().map({ args0 ->
args0
}).orElse(null),
bitrate = javaType.bitrate(),
bufSize = javaType.bufSize().map({ args0 -> args0 }).orElse(null),
colorMetadata = javaType.colorMetadata().map({ args0 -> args0 }).orElse(null),
colorSpaceSettings = javaType.colorSpaceSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsColorSpaceSettings.Companion.toKotlin(args0)
})
}).orElse(null),
filterSettings = javaType.filterSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsFilterSettings.Companion.toKotlin(args0)
})
}).orElse(null),
fixedAfd = javaType.fixedAfd().map({ args0 -> args0 }).orElse(null),
flickerAq = javaType.flickerAq().map({ args0 -> args0 }).orElse(null),
framerateDenominator = javaType.framerateDenominator(),
framerateNumerator = javaType.framerateNumerator(),
gopClosedCadence = javaType.gopClosedCadence().map({ args0 -> args0 }).orElse(null),
gopSize = javaType.gopSize().map({ args0 -> args0 }).orElse(null),
gopSizeUnits = javaType.gopSizeUnits().map({ args0 -> args0 }).orElse(null),
level = javaType.level().map({ args0 -> args0 }).orElse(null),
lookAheadRateControl = javaType.lookAheadRateControl().map({ args0 -> args0 }).orElse(null),
maxBitrate = javaType.maxBitrate().map({ args0 -> args0 }).orElse(null),
minIInterval = javaType.minIInterval().map({ args0 -> args0 }).orElse(null),
minQp = javaType.minQp().map({ args0 -> args0 }).orElse(null),
mvOverPictureBoundaries = javaType.mvOverPictureBoundaries().map({ args0 -> args0 }).orElse(null),
mvTemporalPredictor = javaType.mvTemporalPredictor().map({ args0 -> args0 }).orElse(null),
parDenominator = javaType.parDenominator().map({ args0 -> args0 }).orElse(null),
parNumerator = javaType.parNumerator().map({ args0 -> args0 }).orElse(null),
profile = javaType.profile().map({ args0 -> args0 }).orElse(null),
qvbrQualityLevel = javaType.qvbrQualityLevel().map({ args0 -> args0 }).orElse(null),
rateControlMode = javaType.rateControlMode().map({ args0 -> args0 }).orElse(null),
scanType = javaType.scanType().map({ args0 -> args0 }).orElse(null),
sceneChangeDetect = javaType.sceneChangeDetect().map({ args0 -> args0 }).orElse(null),
slices = javaType.slices().map({ args0 -> args0 }).orElse(null),
tier = javaType.tier().map({ args0 -> args0 }).orElse(null),
tileHeight = javaType.tileHeight().map({ args0 -> args0 }).orElse(null),
tilePadding = javaType.tilePadding().map({ args0 -> args0 }).orElse(null),
tileWidth = javaType.tileWidth().map({ args0 -> args0 }).orElse(null),
timecodeBurninSettings = javaType.timecodeBurninSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsTimecodeBurninSettings.Companion.toKotlin(args0)
})
}).orElse(null),
timecodeInsertion = javaType.timecodeInsertion().map({ args0 -> args0 }).orElse(null),
treeblockSize = javaType.treeblockSize().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy