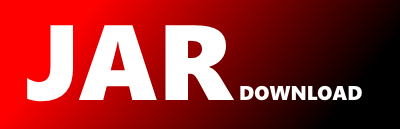
com.pulumi.aws.memorydb.kotlin.User.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.memorydb.kotlin
import com.pulumi.aws.memorydb.kotlin.outputs.UserAuthenticationMode
import com.pulumi.aws.memorydb.kotlin.outputs.UserAuthenticationMode.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [User].
*/
@PulumiTagMarker
public class UserResourceBuilder internal constructor() {
public var name: String? = null
public var args: UserArgs = UserArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend UserArgsBuilder.() -> Unit) {
val builder = UserArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): User {
val builtJavaResource = com.pulumi.aws.memorydb.User(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return User(builtJavaResource)
}
}
/**
* Provides a MemoryDB User.
* More information about users and ACL-s can be found in the [MemoryDB User Guide](https://docs.aws.amazon.com/memorydb/latest/devguide/clusters.acls.html).
* > **Note:** All arguments including the username and passwords will be stored in the raw state as plain-text.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* import * as random from "@pulumi/random";
* const example = new random.index.Password("example", {length: 16});
* const exampleUser = new aws.memorydb.User("example", {
* userName: "my-user",
* accessString: "on ~* &* +@all",
* authenticationMode: {
* type: "password",
* passwords: [example.result],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* import pulumi_random as random
* example = random.index.Password("example", length=16)
* example_user = aws.memorydb.User("example",
* user_name="my-user",
* access_string="on ~* &* +@all",
* authentication_mode={
* "type": "password",
* "passwords": [example["result"]],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* using Random = Pulumi.Random;
* return await Deployment.RunAsync(() =>
* {
* var example = new Random.Index.Password("example", new()
* {
* Length = 16,
* });
* var exampleUser = new Aws.MemoryDb.User("example", new()
* {
* UserName = "my-user",
* AccessString = "on ~* &* +@all",
* AuthenticationMode = new Aws.MemoryDb.Inputs.UserAuthenticationModeArgs
* {
* Type = "password",
* Passwords = new[]
* {
* example.Result,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/memorydb"
* "github.com/pulumi/pulumi-random/sdk/v4/go/random"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := random.NewPassword(ctx, "example", &random.PasswordArgs{
* Length: 16,
* })
* if err != nil {
* return err
* }
* _, err = memorydb.NewUser(ctx, "example", &memorydb.UserArgs{
* UserName: pulumi.String("my-user"),
* AccessString: pulumi.String("on ~* &* +@all"),
* AuthenticationMode: &memorydb.UserAuthenticationModeArgs{
* Type: pulumi.String("password"),
* Passwords: pulumi.StringArray{
* example.Result,
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.random.password;
* import com.pulumi.random.PasswordArgs;
* import com.pulumi.aws.memorydb.User;
* import com.pulumi.aws.memorydb.UserArgs;
* import com.pulumi.aws.memorydb.inputs.UserAuthenticationModeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Password("example", PasswordArgs.builder()
* .length(16)
* .build());
* var exampleUser = new User("exampleUser", UserArgs.builder()
* .userName("my-user")
* .accessString("on ~* &* +@all")
* .authenticationMode(UserAuthenticationModeArgs.builder()
* .type("password")
* .passwords(example.result())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: random:password
* properties:
* length: 16
* exampleUser:
* type: aws:memorydb:User
* name: example
* properties:
* userName: my-user
* accessString: on ~* &* +@all
* authenticationMode:
* type: password
* passwords:
* - ${example.result}
* ```
*
* ## Import
* Using `pulumi import`, import a user using the `user_name`. For example:
* ```sh
* $ pulumi import aws:memorydb/user:User example my-user
* ```
* The `passwords` are not available for imported resources, as this information cannot be read back from the MemoryDB API.
*/
public class User internal constructor(
override val javaResource: com.pulumi.aws.memorydb.User,
) : KotlinCustomResource(javaResource, UserMapper) {
/**
* Access permissions string used for this user.
*/
public val accessString: Output
get() = javaResource.accessString().applyValue({ args0 -> args0 })
/**
* ARN of the user.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Denotes the user's authentication properties. Detailed below.
*/
public val authenticationMode: Output
get() = javaResource.authenticationMode().applyValue({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
/**
* Minimum engine version supported for the user.
*/
public val minimumEngineVersion: Output
get() = javaResource.minimumEngineVersion().applyValue({ args0 -> args0 })
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy