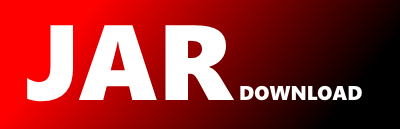
com.pulumi.aws.mq.kotlin.inputs.BrokerLdapServerMetadataArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.mq.kotlin.inputs
import com.pulumi.aws.mq.inputs.BrokerLdapServerMetadataArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property hosts List of a fully qualified domain name of the LDAP server and an optional failover server.
* @property roleBase Fully qualified name of the directory to search for a user’s groups.
* @property roleName Specifies the LDAP attribute that identifies the group name attribute in the object returned from the group membership query.
* @property roleSearchMatching Search criteria for groups.
* @property roleSearchSubtree Whether the directory search scope is the entire sub-tree.
* @property serviceAccountPassword Service account password.
* @property serviceAccountUsername Service account username.
* @property userBase Fully qualified name of the directory where you want to search for users.
* @property userRoleName Specifies the name of the LDAP attribute for the user group membership.
* @property userSearchMatching Search criteria for users.
* @property userSearchSubtree Whether the directory search scope is the entire sub-tree.
*/
public data class BrokerLdapServerMetadataArgs(
public val hosts: Output>? = null,
public val roleBase: Output? = null,
public val roleName: Output? = null,
public val roleSearchMatching: Output? = null,
public val roleSearchSubtree: Output? = null,
public val serviceAccountPassword: Output? = null,
public val serviceAccountUsername: Output? = null,
public val userBase: Output? = null,
public val userRoleName: Output? = null,
public val userSearchMatching: Output? = null,
public val userSearchSubtree: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.mq.inputs.BrokerLdapServerMetadataArgs =
com.pulumi.aws.mq.inputs.BrokerLdapServerMetadataArgs.builder()
.hosts(hosts?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.roleBase(roleBase?.applyValue({ args0 -> args0 }))
.roleName(roleName?.applyValue({ args0 -> args0 }))
.roleSearchMatching(roleSearchMatching?.applyValue({ args0 -> args0 }))
.roleSearchSubtree(roleSearchSubtree?.applyValue({ args0 -> args0 }))
.serviceAccountPassword(serviceAccountPassword?.applyValue({ args0 -> args0 }))
.serviceAccountUsername(serviceAccountUsername?.applyValue({ args0 -> args0 }))
.userBase(userBase?.applyValue({ args0 -> args0 }))
.userRoleName(userRoleName?.applyValue({ args0 -> args0 }))
.userSearchMatching(userSearchMatching?.applyValue({ args0 -> args0 }))
.userSearchSubtree(userSearchSubtree?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BrokerLdapServerMetadataArgs].
*/
@PulumiTagMarker
public class BrokerLdapServerMetadataArgsBuilder internal constructor() {
private var hosts: Output>? = null
private var roleBase: Output? = null
private var roleName: Output? = null
private var roleSearchMatching: Output? = null
private var roleSearchSubtree: Output? = null
private var serviceAccountPassword: Output? = null
private var serviceAccountUsername: Output? = null
private var userBase: Output? = null
private var userRoleName: Output? = null
private var userSearchMatching: Output? = null
private var userSearchSubtree: Output? = null
/**
* @param value List of a fully qualified domain name of the LDAP server and an optional failover server.
*/
@JvmName("dhpvfrxbtrfbfctn")
public suspend fun hosts(`value`: Output>) {
this.hosts = value
}
@JvmName("xijgqiqbuxtvufeh")
public suspend fun hosts(vararg values: Output) {
this.hosts = Output.all(values.asList())
}
/**
* @param values List of a fully qualified domain name of the LDAP server and an optional failover server.
*/
@JvmName("dfwqifiuxvsujtiy")
public suspend fun hosts(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy