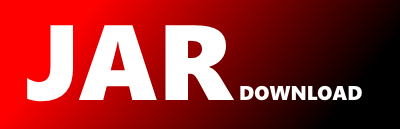
com.pulumi.aws.msk.kotlin.outputs.GetClusterResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.msk.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getCluster.
* @property arn ARN of the MSK cluster.
* @property bootstrapBrokers Comma separated list of one or more hostname:port pairs of kafka brokers suitable to bootstrap connectivity to the kafka cluster. Contains a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `PLAINTEXT` or `TLS_PLAINTEXT`. The resource sorts values alphabetically. AWS may not always return all endpoints so this value is not guaranteed to be stable across applies.
* @property bootstrapBrokersPublicSaslIam One or more DNS names (or IP addresses) and SASL IAM port pairs. For example, `b-1-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9198,b-2-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9198,b-3-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9198`. This attribute will have a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `TLS_PLAINTEXT` or `TLS` and `client_authentication.0.sasl.0.iam` is set to `true` and `broker_node_group_info.0.connectivity_info.0.public_access.0.type` is set to `SERVICE_PROVIDED_EIPS` and the cluster fulfill all other requirements for public access. The resource sorts the list alphabetically. AWS may not always return all endpoints so the values may not be stable across applies.
* @property bootstrapBrokersPublicSaslScram One or more DNS names (or IP addresses) and SASL SCRAM port pairs. For example, `b-1-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9196,b-2-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9196,b-3-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9196`. This attribute will have a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `TLS_PLAINTEXT` or `TLS` and `client_authentication.0.sasl.0.scram` is set to `true` and `broker_node_group_info.0.connectivity_info.0.public_access.0.type` is set to `SERVICE_PROVIDED_EIPS` and the cluster fulfill all other requirements for public access. The resource sorts the list alphabetically. AWS may not always return all endpoints so the values may not be stable across applies.
* @property bootstrapBrokersPublicTls One or more DNS names (or IP addresses) and TLS port pairs. For example, `b-1-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9194,b-2-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9194,b-3-public.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9194`. This attribute will have a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `TLS_PLAINTEXT` or `TLS` and `broker_node_group_info.0.connectivity_info.0.public_access.0.type` is set to `SERVICE_PROVIDED_EIPS` and the cluster fulfill all other requirements for public access. The resource sorts the list alphabetically. AWS may not always return all endpoints so the values may not be stable across applies.
* @property bootstrapBrokersSaslIam One or more DNS names (or IP addresses) and SASL IAM port pairs. For example, `b-1.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9098,b-2.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9098,b-3.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9098`. This attribute will have a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `TLS_PLAINTEXT` or `TLS` and `client_authentication.0.sasl.0.iam` is set to `true`. The resource sorts the list alphabetically. AWS may not always return all endpoints so the values may not be stable across applies.
* @property bootstrapBrokersSaslScram One or more DNS names (or IP addresses) and SASL SCRAM port pairs. For example, `b-1.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9096,b-2.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9096,b-3.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9096`. This attribute will have a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `TLS_PLAINTEXT` or `TLS` and `client_authentication.0.sasl.0.scram` is set to `true`. The resource sorts the list alphabetically. AWS may not always return all endpoints so the values may not be stable across applies.
* @property bootstrapBrokersTls One or more DNS names (or IP addresses) and TLS port pairs. For example, `b-1.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9094,b-2.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9094,b-3.exampleClusterName.abcde.c2.kafka.us-east-1.amazonaws.com:9094`. This attribute will have a value if `encryption_info.0.encryption_in_transit.0.client_broker` is set to `TLS_PLAINTEXT` or `TLS`. The resource sorts the list alphabetically. AWS may not always return all endpoints so the values may not be stable across applies.
* @property brokerNodeGroupInfos Configuration block for the broker nodes of the Kafka cluster.
* @property clusterName
* @property clusterUuid UUID of the MSK cluster, for use in IAM policies.
* @property id The provider-assigned unique ID for this managed resource.
* @property kafkaVersion Apache Kafka version.
* @property numberOfBrokerNodes Number of broker nodes in the cluster.
* @property tags Map of key-value pairs assigned to the cluster.
* @property zookeeperConnectString A comma separated list of one or more hostname:port pairs to use to connect to the Apache Zookeeper cluster. The returned values are sorted alphbetically. The AWS API may not return all endpoints, so this value is not guaranteed to be stable across applies.
* @property zookeeperConnectStringTls A comma separated list of one or more hostname:port pairs to use to connect to the Apache Zookeeper cluster via TLS. The returned values are sorted alphabetically. The AWS API may not return all endpoints, so this value is not guaranteed to be stable across applies.
*/
public data class GetClusterResult(
public val arn: String,
public val bootstrapBrokers: String,
public val bootstrapBrokersPublicSaslIam: String,
public val bootstrapBrokersPublicSaslScram: String,
public val bootstrapBrokersPublicTls: String,
public val bootstrapBrokersSaslIam: String,
public val bootstrapBrokersSaslScram: String,
public val bootstrapBrokersTls: String,
public val brokerNodeGroupInfos: List,
public val clusterName: String,
public val clusterUuid: String,
public val id: String,
public val kafkaVersion: String,
public val numberOfBrokerNodes: Int,
public val tags: Map,
public val zookeeperConnectString: String,
public val zookeeperConnectStringTls: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.msk.outputs.GetClusterResult): GetClusterResult =
GetClusterResult(
arn = javaType.arn(),
bootstrapBrokers = javaType.bootstrapBrokers(),
bootstrapBrokersPublicSaslIam = javaType.bootstrapBrokersPublicSaslIam(),
bootstrapBrokersPublicSaslScram = javaType.bootstrapBrokersPublicSaslScram(),
bootstrapBrokersPublicTls = javaType.bootstrapBrokersPublicTls(),
bootstrapBrokersSaslIam = javaType.bootstrapBrokersSaslIam(),
bootstrapBrokersSaslScram = javaType.bootstrapBrokersSaslScram(),
bootstrapBrokersTls = javaType.bootstrapBrokersTls(),
brokerNodeGroupInfos = javaType.brokerNodeGroupInfos().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.msk.kotlin.outputs.GetClusterBrokerNodeGroupInfo.Companion.toKotlin(args0)
})
}),
clusterName = javaType.clusterName(),
clusterUuid = javaType.clusterUuid(),
id = javaType.id(),
kafkaVersion = javaType.kafkaVersion(),
numberOfBrokerNodes = javaType.numberOfBrokerNodes(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
zookeeperConnectString = javaType.zookeeperConnectString(),
zookeeperConnectStringTls = javaType.zookeeperConnectStringTls(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy