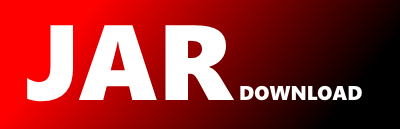
com.pulumi.aws.neptune.kotlin.ClusterInstance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.neptune.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [ClusterInstance].
*/
@PulumiTagMarker
public class ClusterInstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterInstanceArgs = ClusterInstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterInstanceArgsBuilder.() -> Unit) {
val builder = ClusterInstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ClusterInstance {
val builtJavaResource = com.pulumi.aws.neptune.ClusterInstance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ClusterInstance(builtJavaResource)
}
}
/**
* A Cluster Instance Resource defines attributes that are specific to a single instance in a Neptune Cluster.
* You can simply add neptune instances and Neptune manages the replication. You can use the count
* meta-parameter to make multiple instances and join them all to the same Neptune Cluster, or you may specify different Cluster Instance resources with various `instance_class` sizes.
* ## Example Usage
* The following example will create a neptune cluster with two neptune instances(one writer and one reader).
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _default = new aws.neptune.Cluster("default", {
* clusterIdentifier: "neptune-cluster-demo",
* engine: "neptune",
* backupRetentionPeriod: 5,
* preferredBackupWindow: "07:00-09:00",
* skipFinalSnapshot: true,
* iamDatabaseAuthenticationEnabled: true,
* applyImmediately: true,
* });
* const example: aws.neptune.ClusterInstance[] = [];
* for (const range = {value: 0}; range.value < 2; range.value++) {
* example.push(new aws.neptune.ClusterInstance(`example-${range.value}`, {
* clusterIdentifier: _default.id,
* engine: "neptune",
* instanceClass: "db.r4.large",
* applyImmediately: true,
* }));
* }
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default = aws.neptune.Cluster("default",
* cluster_identifier="neptune-cluster-demo",
* engine="neptune",
* backup_retention_period=5,
* preferred_backup_window="07:00-09:00",
* skip_final_snapshot=True,
* iam_database_authentication_enabled=True,
* apply_immediately=True)
* example = []
* for range in [{"value": i} for i in range(0, 2)]:
* example.append(aws.neptune.ClusterInstance(f"example-{range['value']}",
* cluster_identifier=default.id,
* engine="neptune",
* instance_class="db.r4.large",
* apply_immediately=True))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Aws.Neptune.Cluster("default", new()
* {
* ClusterIdentifier = "neptune-cluster-demo",
* Engine = "neptune",
* BackupRetentionPeriod = 5,
* PreferredBackupWindow = "07:00-09:00",
* SkipFinalSnapshot = true,
* IamDatabaseAuthenticationEnabled = true,
* ApplyImmediately = true,
* });
* var example = new List();
* for (var rangeIndex = 0; rangeIndex < 2; rangeIndex++)
* {
* var range = new { Value = rangeIndex };
* example.Add(new Aws.Neptune.ClusterInstance($"example-{range.Value}", new()
* {
* ClusterIdentifier = @default.Id,
* Engine = "neptune",
* InstanceClass = "db.r4.large",
* ApplyImmediately = true,
* }));
* }
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/neptune"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := neptune.NewCluster(ctx, "default", &neptune.ClusterArgs{
* ClusterIdentifier: pulumi.String("neptune-cluster-demo"),
* Engine: pulumi.String("neptune"),
* BackupRetentionPeriod: pulumi.Int(5),
* PreferredBackupWindow: pulumi.String("07:00-09:00"),
* SkipFinalSnapshot: pulumi.Bool(true),
* IamDatabaseAuthenticationEnabled: pulumi.Bool(true),
* ApplyImmediately: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* var example []*neptune.ClusterInstance
* for index := 0; index < 2; index++ {
* key0 := index
* _ := index
* __res, err := neptune.NewClusterInstance(ctx, fmt.Sprintf("example-%v", key0), &neptune.ClusterInstanceArgs{
* ClusterIdentifier: _default.ID(),
* Engine: pulumi.String("neptune"),
* InstanceClass: pulumi.String("db.r4.large"),
* ApplyImmediately: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* example = append(example, __res)
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.neptune.Cluster;
* import com.pulumi.aws.neptune.ClusterArgs;
* import com.pulumi.aws.neptune.ClusterInstance;
* import com.pulumi.aws.neptune.ClusterInstanceArgs;
* import com.pulumi.codegen.internal.KeyedValue;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("neptune-cluster-demo")
* .engine("neptune")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .skipFinalSnapshot(true)
* .iamDatabaseAuthenticationEnabled(true)
* .applyImmediately(true)
* .build());
* for (var i = 0; i < 2; i++) {
* new ClusterInstance("example-" + i, ClusterInstanceArgs.builder()
* .clusterIdentifier(default_.id())
* .engine("neptune")
* .instanceClass("db.r4.large")
* .applyImmediately(true)
* .build());
* }
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: aws:neptune:Cluster
* properties:
* clusterIdentifier: neptune-cluster-demo
* engine: neptune
* backupRetentionPeriod: 5
* preferredBackupWindow: 07:00-09:00
* skipFinalSnapshot: true
* iamDatabaseAuthenticationEnabled: true
* applyImmediately: true
* example:
* type: aws:neptune:ClusterInstance
* properties:
* clusterIdentifier: ${default.id}
* engine: neptune
* instanceClass: db.r4.large
* applyImmediately: true
* options: {}
* ```
*
* ## Import
* Using `pulumi import`, import `aws_neptune_cluster_instance` using the instance identifier. For example:
* ```sh
* $ pulumi import aws:neptune/clusterInstance:ClusterInstance example my-instance
* ```
*/
public class ClusterInstance internal constructor(
override val javaResource: com.pulumi.aws.neptune.ClusterInstance,
) : KotlinCustomResource(javaResource, ClusterInstanceMapper) {
/**
* The hostname of the instance. See also `endpoint` and `port`.
*/
public val address: Output
get() = javaResource.address().applyValue({ args0 -> args0 })
/**
* Specifies whether any instance modifications
* are applied immediately, or during the next maintenance window. Default is`false`.
*/
public val applyImmediately: Output
get() = javaResource.applyImmediately().applyValue({ args0 -> args0 })
/**
* Amazon Resource Name (ARN) of neptune instance
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Indicates that minor engine upgrades will be applied automatically to the instance during the maintenance window. Default is `true`.
*/
public val autoMinorVersionUpgrade: Output?
get() = javaResource.autoMinorVersionUpgrade().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The EC2 Availability Zone that the neptune instance is created in.
*/
public val availabilityZone: Output
get() = javaResource.availabilityZone().applyValue({ args0 -> args0 })
/**
* The identifier of the `aws.neptune.Cluster` in which to launch this instance.
*/
public val clusterIdentifier: Output
get() = javaResource.clusterIdentifier().applyValue({ args0 -> args0 })
/**
* The region-unique, immutable identifier for the neptune instance.
*/
public val dbiResourceId: Output
get() = javaResource.dbiResourceId().applyValue({ args0 -> args0 })
/**
* The connection endpoint in `address:port` format.
*/
public val endpoint: Output
get() = javaResource.endpoint().applyValue({ args0 -> args0 })
/**
* The name of the database engine to be used for the neptune instance. Defaults to `neptune`. Valid Values: `neptune`.
*/
public val engine: Output?
get() = javaResource.engine().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The neptune engine version. Currently configuring this argumnet has no effect.
*/
public val engineVersion: Output
get() = javaResource.engineVersion().applyValue({ args0 -> args0 })
/**
* The identifier for the neptune instance, if omitted, this provider will assign a random, unique identifier.
*/
public val identifier: Output
get() = javaResource.identifier().applyValue({ args0 -> args0 })
/**
* Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*/
public val identifierPrefix: Output
get() = javaResource.identifierPrefix().applyValue({ args0 -> args0 })
/**
* The instance class to use.
*/
public val instanceClass: Output
get() = javaResource.instanceClass().applyValue({ args0 -> args0 })
/**
* The ARN for the KMS encryption key if one is set to the neptune cluster.
*/
public val kmsKeyArn: Output
get() = javaResource.kmsKeyArn().applyValue({ args0 -> args0 })
/**
* The name of the neptune parameter group to associate with this instance.
*/
public val neptuneParameterGroupName: Output
get() = javaResource.neptuneParameterGroupName().applyValue({ args0 -> args0 })
/**
* A subnet group to associate with this neptune instance. **NOTE:** This must match the `neptune_subnet_group_name` of the attached `aws.neptune.Cluster`.
*/
public val neptuneSubnetGroupName: Output
get() = javaResource.neptuneSubnetGroupName().applyValue({ args0 -> args0 })
/**
* The port on which the DB accepts connections. Defaults to `8182`.
*/
public val port: Output?
get() = javaResource.port().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The daily time range during which automated backups are created if automated backups are enabled. Eg: "04:00-09:00"
*/
public val preferredBackupWindow: Output
get() = javaResource.preferredBackupWindow().applyValue({ args0 -> args0 })
/**
* The window to perform maintenance in.
* Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00".
*/
public val preferredMaintenanceWindow: Output
get() = javaResource.preferredMaintenanceWindow().applyValue({ args0 -> args0 })
/**
* Default 0. Failover Priority setting on instance level. The reader who has lower tier has higher priority to get promoter to writer.
*/
public val promotionTier: Output?
get() = javaResource.promotionTier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Bool to control if instance is publicly accessible. Default is `false`.
*/
public val publiclyAccessible: Output?
get() = javaResource.publiclyAccessible().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Determines whether a final DB snapshot is created before the DB instance is deleted.
*/
public val skipFinalSnapshot: Output?
get() = javaResource.skipFinalSnapshot().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether the neptune cluster is encrypted.
*/
public val storageEncrypted: Output
get() = javaResource.storageEncrypted().applyValue({ args0 -> args0 })
/**
* Storage type associated with the cluster `standard/iopt1`.
*/
public val storageType: Output
get() = javaResource.storageType().applyValue({ args0 -> args0 })
/**
* A map of tags to assign to the instance. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy