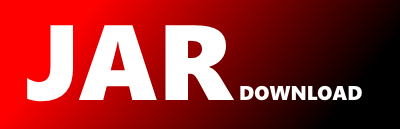
com.pulumi.aws.networkmanager.kotlin.CustomerGatewayAssociationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.networkmanager.kotlin
import com.pulumi.aws.networkmanager.CustomerGatewayAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Associates a customer gateway with a device and optionally, with a link.
* If you specify a link, it must be associated with the specified device.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.networkmanager.GlobalNetwork("example", {description: "example"});
* const exampleSite = new aws.networkmanager.Site("example", {globalNetworkId: example.id});
* const exampleDevice = new aws.networkmanager.Device("example", {
* globalNetworkId: example.id,
* siteId: exampleSite.id,
* });
* const exampleCustomerGateway = new aws.ec2.CustomerGateway("example", {
* bgpAsn: "65000",
* ipAddress: "172.83.124.10",
* type: "ipsec.1",
* });
* const exampleTransitGateway = new aws.ec2transitgateway.TransitGateway("example", {});
* const exampleVpnConnection = new aws.ec2.VpnConnection("example", {
* customerGatewayId: exampleCustomerGateway.id,
* transitGatewayId: exampleTransitGateway.id,
* type: exampleCustomerGateway.type,
* staticRoutesOnly: true,
* });
* const exampleTransitGatewayRegistration = new aws.networkmanager.TransitGatewayRegistration("example", {
* globalNetworkId: example.id,
* transitGatewayArn: exampleTransitGateway.arn,
* }, {
* dependsOn: [exampleVpnConnection],
* });
* const exampleCustomerGatewayAssociation = new aws.networkmanager.CustomerGatewayAssociation("example", {
* globalNetworkId: example.id,
* customerGatewayArn: exampleCustomerGateway.arn,
* deviceId: exampleDevice.id,
* }, {
* dependsOn: [exampleTransitGatewayRegistration],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.networkmanager.GlobalNetwork("example", description="example")
* example_site = aws.networkmanager.Site("example", global_network_id=example.id)
* example_device = aws.networkmanager.Device("example",
* global_network_id=example.id,
* site_id=example_site.id)
* example_customer_gateway = aws.ec2.CustomerGateway("example",
* bgp_asn="65000",
* ip_address="172.83.124.10",
* type="ipsec.1")
* example_transit_gateway = aws.ec2transitgateway.TransitGateway("example")
* example_vpn_connection = aws.ec2.VpnConnection("example",
* customer_gateway_id=example_customer_gateway.id,
* transit_gateway_id=example_transit_gateway.id,
* type=example_customer_gateway.type,
* static_routes_only=True)
* example_transit_gateway_registration = aws.networkmanager.TransitGatewayRegistration("example",
* global_network_id=example.id,
* transit_gateway_arn=example_transit_gateway.arn,
* opts = pulumi.ResourceOptions(depends_on=[example_vpn_connection]))
* example_customer_gateway_association = aws.networkmanager.CustomerGatewayAssociation("example",
* global_network_id=example.id,
* customer_gateway_arn=example_customer_gateway.arn,
* device_id=example_device.id,
* opts = pulumi.ResourceOptions(depends_on=[example_transit_gateway_registration]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.NetworkManager.GlobalNetwork("example", new()
* {
* Description = "example",
* });
* var exampleSite = new Aws.NetworkManager.Site("example", new()
* {
* GlobalNetworkId = example.Id,
* });
* var exampleDevice = new Aws.NetworkManager.Device("example", new()
* {
* GlobalNetworkId = example.Id,
* SiteId = exampleSite.Id,
* });
* var exampleCustomerGateway = new Aws.Ec2.CustomerGateway("example", new()
* {
* BgpAsn = "65000",
* IpAddress = "172.83.124.10",
* Type = "ipsec.1",
* });
* var exampleTransitGateway = new Aws.Ec2TransitGateway.TransitGateway("example");
* var exampleVpnConnection = new Aws.Ec2.VpnConnection("example", new()
* {
* CustomerGatewayId = exampleCustomerGateway.Id,
* TransitGatewayId = exampleTransitGateway.Id,
* Type = exampleCustomerGateway.Type,
* StaticRoutesOnly = true,
* });
* var exampleTransitGatewayRegistration = new Aws.NetworkManager.TransitGatewayRegistration("example", new()
* {
* GlobalNetworkId = example.Id,
* TransitGatewayArn = exampleTransitGateway.Arn,
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleVpnConnection,
* },
* });
* var exampleCustomerGatewayAssociation = new Aws.NetworkManager.CustomerGatewayAssociation("example", new()
* {
* GlobalNetworkId = example.Id,
* CustomerGatewayArn = exampleCustomerGateway.Arn,
* DeviceId = exampleDevice.Id,
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleTransitGatewayRegistration,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2transitgateway"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/networkmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := networkmanager.NewGlobalNetwork(ctx, "example", &networkmanager.GlobalNetworkArgs{
* Description: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* exampleSite, err := networkmanager.NewSite(ctx, "example", &networkmanager.SiteArgs{
* GlobalNetworkId: example.ID(),
* })
* if err != nil {
* return err
* }
* exampleDevice, err := networkmanager.NewDevice(ctx, "example", &networkmanager.DeviceArgs{
* GlobalNetworkId: example.ID(),
* SiteId: exampleSite.ID(),
* })
* if err != nil {
* return err
* }
* exampleCustomerGateway, err := ec2.NewCustomerGateway(ctx, "example", &ec2.CustomerGatewayArgs{
* BgpAsn: pulumi.String("65000"),
* IpAddress: pulumi.String("172.83.124.10"),
* Type: pulumi.String("ipsec.1"),
* })
* if err != nil {
* return err
* }
* exampleTransitGateway, err := ec2transitgateway.NewTransitGateway(ctx, "example", nil)
* if err != nil {
* return err
* }
* exampleVpnConnection, err := ec2.NewVpnConnection(ctx, "example", &ec2.VpnConnectionArgs{
* CustomerGatewayId: exampleCustomerGateway.ID(),
* TransitGatewayId: exampleTransitGateway.ID(),
* Type: exampleCustomerGateway.Type,
* StaticRoutesOnly: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* exampleTransitGatewayRegistration, err := networkmanager.NewTransitGatewayRegistration(ctx, "example", &networkmanager.TransitGatewayRegistrationArgs{
* GlobalNetworkId: example.ID(),
* TransitGatewayArn: exampleTransitGateway.Arn,
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleVpnConnection,
* }))
* if err != nil {
* return err
* }
* _, err = networkmanager.NewCustomerGatewayAssociation(ctx, "example", &networkmanager.CustomerGatewayAssociationArgs{
* GlobalNetworkId: example.ID(),
* CustomerGatewayArn: exampleCustomerGateway.Arn,
* DeviceId: exampleDevice.ID(),
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleTransitGatewayRegistration,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.networkmanager.GlobalNetwork;
* import com.pulumi.aws.networkmanager.GlobalNetworkArgs;
* import com.pulumi.aws.networkmanager.Site;
* import com.pulumi.aws.networkmanager.SiteArgs;
* import com.pulumi.aws.networkmanager.Device;
* import com.pulumi.aws.networkmanager.DeviceArgs;
* import com.pulumi.aws.ec2.CustomerGateway;
* import com.pulumi.aws.ec2.CustomerGatewayArgs;
* import com.pulumi.aws.ec2transitgateway.TransitGateway;
* import com.pulumi.aws.ec2.VpnConnection;
* import com.pulumi.aws.ec2.VpnConnectionArgs;
* import com.pulumi.aws.networkmanager.TransitGatewayRegistration;
* import com.pulumi.aws.networkmanager.TransitGatewayRegistrationArgs;
* import com.pulumi.aws.networkmanager.CustomerGatewayAssociation;
* import com.pulumi.aws.networkmanager.CustomerGatewayAssociationArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new GlobalNetwork("example", GlobalNetworkArgs.builder()
* .description("example")
* .build());
* var exampleSite = new Site("exampleSite", SiteArgs.builder()
* .globalNetworkId(example.id())
* .build());
* var exampleDevice = new Device("exampleDevice", DeviceArgs.builder()
* .globalNetworkId(example.id())
* .siteId(exampleSite.id())
* .build());
* var exampleCustomerGateway = new CustomerGateway("exampleCustomerGateway", CustomerGatewayArgs.builder()
* .bgpAsn(65000)
* .ipAddress("172.83.124.10")
* .type("ipsec.1")
* .build());
* var exampleTransitGateway = new TransitGateway("exampleTransitGateway");
* var exampleVpnConnection = new VpnConnection("exampleVpnConnection", VpnConnectionArgs.builder()
* .customerGatewayId(exampleCustomerGateway.id())
* .transitGatewayId(exampleTransitGateway.id())
* .type(exampleCustomerGateway.type())
* .staticRoutesOnly(true)
* .build());
* var exampleTransitGatewayRegistration = new TransitGatewayRegistration("exampleTransitGatewayRegistration", TransitGatewayRegistrationArgs.builder()
* .globalNetworkId(example.id())
* .transitGatewayArn(exampleTransitGateway.arn())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleVpnConnection)
* .build());
* var exampleCustomerGatewayAssociation = new CustomerGatewayAssociation("exampleCustomerGatewayAssociation", CustomerGatewayAssociationArgs.builder()
* .globalNetworkId(example.id())
* .customerGatewayArn(exampleCustomerGateway.arn())
* .deviceId(exampleDevice.id())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleTransitGatewayRegistration)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:networkmanager:GlobalNetwork
* properties:
* description: example
* exampleSite:
* type: aws:networkmanager:Site
* name: example
* properties:
* globalNetworkId: ${example.id}
* exampleDevice:
* type: aws:networkmanager:Device
* name: example
* properties:
* globalNetworkId: ${example.id}
* siteId: ${exampleSite.id}
* exampleCustomerGateway:
* type: aws:ec2:CustomerGateway
* name: example
* properties:
* bgpAsn: 65000
* ipAddress: 172.83.124.10
* type: ipsec.1
* exampleTransitGateway:
* type: aws:ec2transitgateway:TransitGateway
* name: example
* exampleVpnConnection:
* type: aws:ec2:VpnConnection
* name: example
* properties:
* customerGatewayId: ${exampleCustomerGateway.id}
* transitGatewayId: ${exampleTransitGateway.id}
* type: ${exampleCustomerGateway.type}
* staticRoutesOnly: true
* exampleTransitGatewayRegistration:
* type: aws:networkmanager:TransitGatewayRegistration
* name: example
* properties:
* globalNetworkId: ${example.id}
* transitGatewayArn: ${exampleTransitGateway.arn}
* options:
* dependson:
* - ${exampleVpnConnection}
* exampleCustomerGatewayAssociation:
* type: aws:networkmanager:CustomerGatewayAssociation
* name: example
* properties:
* globalNetworkId: ${example.id}
* customerGatewayArn: ${exampleCustomerGateway.arn}
* deviceId: ${exampleDevice.id}
* options:
* dependson:
* - ${exampleTransitGatewayRegistration}
* ```
*
* ## Import
* Using `pulumi import`, import `aws_networkmanager_customer_gateway_association` using the global network ID and customer gateway ARN. For example:
* ```sh
* $ pulumi import aws:networkmanager/customerGatewayAssociation:CustomerGatewayAssociation example global-network-0d47f6t230mz46dy4,arn:aws:ec2:us-west-2:123456789012:customer-gateway/cgw-123abc05e04123abc
* ```
* @property customerGatewayArn The Amazon Resource Name (ARN) of the customer gateway.
* @property deviceId The ID of the device.
* @property globalNetworkId The ID of the global network.
* @property linkId The ID of the link.
*/
public data class CustomerGatewayAssociationArgs(
public val customerGatewayArn: Output? = null,
public val deviceId: Output? = null,
public val globalNetworkId: Output? = null,
public val linkId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.networkmanager.CustomerGatewayAssociationArgs =
com.pulumi.aws.networkmanager.CustomerGatewayAssociationArgs.builder()
.customerGatewayArn(customerGatewayArn?.applyValue({ args0 -> args0 }))
.deviceId(deviceId?.applyValue({ args0 -> args0 }))
.globalNetworkId(globalNetworkId?.applyValue({ args0 -> args0 }))
.linkId(linkId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CustomerGatewayAssociationArgs].
*/
@PulumiTagMarker
public class CustomerGatewayAssociationArgsBuilder internal constructor() {
private var customerGatewayArn: Output? = null
private var deviceId: Output? = null
private var globalNetworkId: Output? = null
private var linkId: Output? = null
/**
* @param value The Amazon Resource Name (ARN) of the customer gateway.
*/
@JvmName("daecybhukndslrbr")
public suspend fun customerGatewayArn(`value`: Output) {
this.customerGatewayArn = value
}
/**
* @param value The ID of the device.
*/
@JvmName("qbujyctqilyfhvsu")
public suspend fun deviceId(`value`: Output) {
this.deviceId = value
}
/**
* @param value The ID of the global network.
*/
@JvmName("lpwoctpxvrsekste")
public suspend fun globalNetworkId(`value`: Output) {
this.globalNetworkId = value
}
/**
* @param value The ID of the link.
*/
@JvmName("vufbufvcoqdpsqvg")
public suspend fun linkId(`value`: Output) {
this.linkId = value
}
/**
* @param value The Amazon Resource Name (ARN) of the customer gateway.
*/
@JvmName("yqaodteoqgufclbf")
public suspend fun customerGatewayArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customerGatewayArn = mapped
}
/**
* @param value The ID of the device.
*/
@JvmName("iqldluonhbbosxyn")
public suspend fun deviceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deviceId = mapped
}
/**
* @param value The ID of the global network.
*/
@JvmName("arahjnpcfphclurd")
public suspend fun globalNetworkId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.globalNetworkId = mapped
}
/**
* @param value The ID of the link.
*/
@JvmName("aeyesloscvvkrjdy")
public suspend fun linkId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkId = mapped
}
internal fun build(): CustomerGatewayAssociationArgs = CustomerGatewayAssociationArgs(
customerGatewayArn = customerGatewayArn,
deviceId = deviceId,
globalNetworkId = globalNetworkId,
linkId = linkId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy