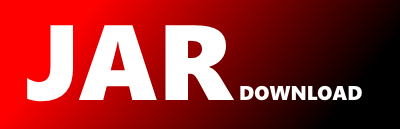
com.pulumi.aws.opensearch.kotlin.OutboundConnectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.opensearch.kotlin
import com.pulumi.aws.opensearch.OutboundConnectionArgs.builder
import com.pulumi.aws.opensearch.kotlin.inputs.OutboundConnectionConnectionPropertiesArgs
import com.pulumi.aws.opensearch.kotlin.inputs.OutboundConnectionConnectionPropertiesArgsBuilder
import com.pulumi.aws.opensearch.kotlin.inputs.OutboundConnectionLocalDomainInfoArgs
import com.pulumi.aws.opensearch.kotlin.inputs.OutboundConnectionLocalDomainInfoArgsBuilder
import com.pulumi.aws.opensearch.kotlin.inputs.OutboundConnectionRemoteDomainInfoArgs
import com.pulumi.aws.opensearch.kotlin.inputs.OutboundConnectionRemoteDomainInfoArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages an AWS Opensearch Outbound Connection.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getCallerIdentity({});
* const currentGetRegion = aws.getRegion({});
* const foo = new aws.opensearch.OutboundConnection("foo", {
* connectionAlias: "outbound_connection",
* connectionMode: "DIRECT",
* localDomainInfo: {
* ownerId: current.then(current => current.accountId),
* region: currentGetRegion.then(currentGetRegion => currentGetRegion.name),
* domainName: localDomain.domainName,
* },
* remoteDomainInfo: {
* ownerId: current.then(current => current.accountId),
* region: currentGetRegion.then(currentGetRegion => currentGetRegion.name),
* domainName: remoteDomain.domainName,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.get_caller_identity()
* current_get_region = aws.get_region()
* foo = aws.opensearch.OutboundConnection("foo",
* connection_alias="outbound_connection",
* connection_mode="DIRECT",
* local_domain_info={
* "owner_id": current.account_id,
* "region": current_get_region.name,
* "domain_name": local_domain["domainName"],
* },
* remote_domain_info={
* "owner_id": current.account_id,
* "region": current_get_region.name,
* "domain_name": remote_domain["domainName"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetCallerIdentity.Invoke();
* var currentGetRegion = Aws.GetRegion.Invoke();
* var foo = new Aws.OpenSearch.OutboundConnection("foo", new()
* {
* ConnectionAlias = "outbound_connection",
* ConnectionMode = "DIRECT",
* LocalDomainInfo = new Aws.OpenSearch.Inputs.OutboundConnectionLocalDomainInfoArgs
* {
* OwnerId = current.Apply(getCallerIdentityResult => getCallerIdentityResult.AccountId),
* Region = currentGetRegion.Apply(getRegionResult => getRegionResult.Name),
* DomainName = localDomain.DomainName,
* },
* RemoteDomainInfo = new Aws.OpenSearch.Inputs.OutboundConnectionRemoteDomainInfoArgs
* {
* OwnerId = current.Apply(getCallerIdentityResult => getCallerIdentityResult.AccountId),
* Region = currentGetRegion.Apply(getRegionResult => getRegionResult.Name),
* DomainName = remoteDomain.DomainName,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/opensearch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := aws.GetCallerIdentity(ctx, &aws.GetCallerIdentityArgs{}, nil)
* if err != nil {
* return err
* }
* currentGetRegion, err := aws.GetRegion(ctx, &aws.GetRegionArgs{}, nil)
* if err != nil {
* return err
* }
* _, err = opensearch.NewOutboundConnection(ctx, "foo", &opensearch.OutboundConnectionArgs{
* ConnectionAlias: pulumi.String("outbound_connection"),
* ConnectionMode: pulumi.String("DIRECT"),
* LocalDomainInfo: &opensearch.OutboundConnectionLocalDomainInfoArgs{
* OwnerId: pulumi.String(current.AccountId),
* Region: pulumi.String(currentGetRegion.Name),
* DomainName: pulumi.Any(localDomain.DomainName),
* },
* RemoteDomainInfo: &opensearch.OutboundConnectionRemoteDomainInfoArgs{
* OwnerId: pulumi.String(current.AccountId),
* Region: pulumi.String(currentGetRegion.Name),
* DomainName: pulumi.Any(remoteDomain.DomainName),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.opensearch.OutboundConnection;
* import com.pulumi.aws.opensearch.OutboundConnectionArgs;
* import com.pulumi.aws.opensearch.inputs.OutboundConnectionLocalDomainInfoArgs;
* import com.pulumi.aws.opensearch.inputs.OutboundConnectionRemoteDomainInfoArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getCallerIdentity();
* final var currentGetRegion = AwsFunctions.getRegion();
* var foo = new OutboundConnection("foo", OutboundConnectionArgs.builder()
* .connectionAlias("outbound_connection")
* .connectionMode("DIRECT")
* .localDomainInfo(OutboundConnectionLocalDomainInfoArgs.builder()
* .ownerId(current.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId()))
* .region(currentGetRegion.applyValue(getRegionResult -> getRegionResult.name()))
* .domainName(localDomain.domainName())
* .build())
* .remoteDomainInfo(OutboundConnectionRemoteDomainInfoArgs.builder()
* .ownerId(current.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId()))
* .region(currentGetRegion.applyValue(getRegionResult -> getRegionResult.name()))
* .domainName(remoteDomain.domainName())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: aws:opensearch:OutboundConnection
* properties:
* connectionAlias: outbound_connection
* connectionMode: DIRECT
* localDomainInfo:
* ownerId: ${current.accountId}
* region: ${currentGetRegion.name}
* domainName: ${localDomain.domainName}
* remoteDomainInfo:
* ownerId: ${current.accountId}
* region: ${currentGetRegion.name}
* domainName: ${remoteDomain.domainName}
* variables:
* current:
* fn::invoke:
* Function: aws:getCallerIdentity
* Arguments: {}
* currentGetRegion:
* fn::invoke:
* Function: aws:getRegion
* Arguments: {}
* ```
*
* ## Import
* Using `pulumi import`, import AWS Opensearch Outbound Connections using the Outbound Connection ID. For example:
* ```sh
* $ pulumi import aws:opensearch/outboundConnection:OutboundConnection foo connection-id
* ```
* @property acceptConnection Accepts the connection.
* @property connectionAlias Specifies the connection alias that will be used by the customer for this connection.
* @property connectionMode Specifies the connection mode. Accepted values are `DIRECT` or `VPC_ENDPOINT`.
* @property connectionProperties Configuration block for the outbound connection.
* @property localDomainInfo Configuration block for the local Opensearch domain.
* @property remoteDomainInfo Configuration block for the remote Opensearch domain.
*/
public data class OutboundConnectionArgs(
public val acceptConnection: Output? = null,
public val connectionAlias: Output? = null,
public val connectionMode: Output? = null,
public val connectionProperties: Output? = null,
public val localDomainInfo: Output? = null,
public val remoteDomainInfo: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.opensearch.OutboundConnectionArgs =
com.pulumi.aws.opensearch.OutboundConnectionArgs.builder()
.acceptConnection(acceptConnection?.applyValue({ args0 -> args0 }))
.connectionAlias(connectionAlias?.applyValue({ args0 -> args0 }))
.connectionMode(connectionMode?.applyValue({ args0 -> args0 }))
.connectionProperties(
connectionProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.localDomainInfo(localDomainInfo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.remoteDomainInfo(
remoteDomainInfo?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [OutboundConnectionArgs].
*/
@PulumiTagMarker
public class OutboundConnectionArgsBuilder internal constructor() {
private var acceptConnection: Output? = null
private var connectionAlias: Output? = null
private var connectionMode: Output? = null
private var connectionProperties: Output? = null
private var localDomainInfo: Output? = null
private var remoteDomainInfo: Output? = null
/**
* @param value Accepts the connection.
*/
@JvmName("pvbarqniqlbahtym")
public suspend fun acceptConnection(`value`: Output) {
this.acceptConnection = value
}
/**
* @param value Specifies the connection alias that will be used by the customer for this connection.
*/
@JvmName("bcgjmtcihueoaljr")
public suspend fun connectionAlias(`value`: Output) {
this.connectionAlias = value
}
/**
* @param value Specifies the connection mode. Accepted values are `DIRECT` or `VPC_ENDPOINT`.
*/
@JvmName("oqisquhuqniynlav")
public suspend fun connectionMode(`value`: Output) {
this.connectionMode = value
}
/**
* @param value Configuration block for the outbound connection.
*/
@JvmName("rwlfpfhtxgqhpqeo")
public suspend fun connectionProperties(`value`: Output) {
this.connectionProperties = value
}
/**
* @param value Configuration block for the local Opensearch domain.
*/
@JvmName("pglcfuyocbdjoied")
public suspend fun localDomainInfo(`value`: Output) {
this.localDomainInfo = value
}
/**
* @param value Configuration block for the remote Opensearch domain.
*/
@JvmName("sejuhcoqcqriodhu")
public suspend fun remoteDomainInfo(`value`: Output) {
this.remoteDomainInfo = value
}
/**
* @param value Accepts the connection.
*/
@JvmName("tfsmwgqrjvwckqef")
public suspend fun acceptConnection(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acceptConnection = mapped
}
/**
* @param value Specifies the connection alias that will be used by the customer for this connection.
*/
@JvmName("aqvarxbyoomgfkmw")
public suspend fun connectionAlias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionAlias = mapped
}
/**
* @param value Specifies the connection mode. Accepted values are `DIRECT` or `VPC_ENDPOINT`.
*/
@JvmName("sgchusbctfqafjmh")
public suspend fun connectionMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionMode = mapped
}
/**
* @param value Configuration block for the outbound connection.
*/
@JvmName("dnirwhaawqmlykgw")
public suspend fun connectionProperties(`value`: OutboundConnectionConnectionPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionProperties = mapped
}
/**
* @param argument Configuration block for the outbound connection.
*/
@JvmName("kaxiinbdltmeuweq")
public suspend fun connectionProperties(argument: suspend OutboundConnectionConnectionPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = OutboundConnectionConnectionPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.connectionProperties = mapped
}
/**
* @param value Configuration block for the local Opensearch domain.
*/
@JvmName("rqpslfkxdvtqpyar")
public suspend fun localDomainInfo(`value`: OutboundConnectionLocalDomainInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.localDomainInfo = mapped
}
/**
* @param argument Configuration block for the local Opensearch domain.
*/
@JvmName("qdirelnectokybqu")
public suspend fun localDomainInfo(argument: suspend OutboundConnectionLocalDomainInfoArgsBuilder.() -> Unit) {
val toBeMapped = OutboundConnectionLocalDomainInfoArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.localDomainInfo = mapped
}
/**
* @param value Configuration block for the remote Opensearch domain.
*/
@JvmName("houcyskvujibmqqu")
public suspend fun remoteDomainInfo(`value`: OutboundConnectionRemoteDomainInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.remoteDomainInfo = mapped
}
/**
* @param argument Configuration block for the remote Opensearch domain.
*/
@JvmName("yswggrqijxrbrbpw")
public suspend fun remoteDomainInfo(argument: suspend OutboundConnectionRemoteDomainInfoArgsBuilder.() -> Unit) {
val toBeMapped = OutboundConnectionRemoteDomainInfoArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.remoteDomainInfo = mapped
}
internal fun build(): OutboundConnectionArgs = OutboundConnectionArgs(
acceptConnection = acceptConnection,
connectionAlias = connectionAlias,
connectionMode = connectionMode,
connectionProperties = connectionProperties,
localDomainInfo = localDomainInfo,
remoteDomainInfo = remoteDomainInfo,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy