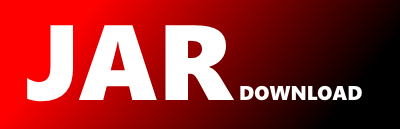
com.pulumi.aws.opensearch.kotlin.inputs.DomainSamlOptionsSamlOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.opensearch.kotlin.inputs
import com.pulumi.aws.opensearch.inputs.DomainSamlOptionsSamlOptionsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enabled Whether SAML authentication is enabled.
* @property idp Information from your identity provider.
* @property masterBackendRole This backend role from the SAML IdP receives full permissions to the cluster, equivalent to a new master user.
* @property masterUserName This username from the SAML IdP receives full permissions to the cluster, equivalent to a new master user.
* @property rolesKey Element of the SAML assertion to use for backend roles. Default is roles.
* @property sessionTimeoutMinutes Duration of a session in minutes after a user logs in. Default is 60. Maximum value is 1,440.
* @property subjectKey Element of the SAML assertion to use for username. Default is NameID.
*/
public data class DomainSamlOptionsSamlOptionsArgs(
public val enabled: Output? = null,
public val idp: Output? = null,
public val masterBackendRole: Output? = null,
public val masterUserName: Output? = null,
public val rolesKey: Output? = null,
public val sessionTimeoutMinutes: Output? = null,
public val subjectKey: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.opensearch.inputs.DomainSamlOptionsSamlOptionsArgs =
com.pulumi.aws.opensearch.inputs.DomainSamlOptionsSamlOptionsArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.idp(idp?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.masterBackendRole(masterBackendRole?.applyValue({ args0 -> args0 }))
.masterUserName(masterUserName?.applyValue({ args0 -> args0 }))
.rolesKey(rolesKey?.applyValue({ args0 -> args0 }))
.sessionTimeoutMinutes(sessionTimeoutMinutes?.applyValue({ args0 -> args0 }))
.subjectKey(subjectKey?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DomainSamlOptionsSamlOptionsArgs].
*/
@PulumiTagMarker
public class DomainSamlOptionsSamlOptionsArgsBuilder internal constructor() {
private var enabled: Output? = null
private var idp: Output? = null
private var masterBackendRole: Output? = null
private var masterUserName: Output? = null
private var rolesKey: Output? = null
private var sessionTimeoutMinutes: Output? = null
private var subjectKey: Output? = null
/**
* @param value Whether SAML authentication is enabled.
*/
@JvmName("jnijalxowqffrjwi")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Information from your identity provider.
*/
@JvmName("pummejpqwbuevssg")
public suspend fun idp(`value`: Output) {
this.idp = value
}
/**
* @param value This backend role from the SAML IdP receives full permissions to the cluster, equivalent to a new master user.
*/
@JvmName("wnjomfeugshqspnx")
public suspend fun masterBackendRole(`value`: Output) {
this.masterBackendRole = value
}
/**
* @param value This username from the SAML IdP receives full permissions to the cluster, equivalent to a new master user.
*/
@JvmName("dhrpajwibvwoyuks")
public suspend fun masterUserName(`value`: Output) {
this.masterUserName = value
}
/**
* @param value Element of the SAML assertion to use for backend roles. Default is roles.
*/
@JvmName("tbgkcodloavxtyeh")
public suspend fun rolesKey(`value`: Output) {
this.rolesKey = value
}
/**
* @param value Duration of a session in minutes after a user logs in. Default is 60. Maximum value is 1,440.
*/
@JvmName("ghloigcwbhrqjfau")
public suspend fun sessionTimeoutMinutes(`value`: Output) {
this.sessionTimeoutMinutes = value
}
/**
* @param value Element of the SAML assertion to use for username. Default is NameID.
*/
@JvmName("tnbrvwevnlngsqca")
public suspend fun subjectKey(`value`: Output) {
this.subjectKey = value
}
/**
* @param value Whether SAML authentication is enabled.
*/
@JvmName("qeeqwbsqtmsycrqf")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value Information from your identity provider.
*/
@JvmName("wdelkgorptmifkgx")
public suspend fun idp(`value`: DomainSamlOptionsSamlOptionsIdpArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.idp = mapped
}
/**
* @param argument Information from your identity provider.
*/
@JvmName("kbdcalpyfylcwovq")
public suspend fun idp(argument: suspend DomainSamlOptionsSamlOptionsIdpArgsBuilder.() -> Unit) {
val toBeMapped = DomainSamlOptionsSamlOptionsIdpArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.idp = mapped
}
/**
* @param value This backend role from the SAML IdP receives full permissions to the cluster, equivalent to a new master user.
*/
@JvmName("egutedceufouxojf")
public suspend fun masterBackendRole(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterBackendRole = mapped
}
/**
* @param value This username from the SAML IdP receives full permissions to the cluster, equivalent to a new master user.
*/
@JvmName("gvrejawwmxvgvorr")
public suspend fun masterUserName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterUserName = mapped
}
/**
* @param value Element of the SAML assertion to use for backend roles. Default is roles.
*/
@JvmName("sselnfscjwsbqneo")
public suspend fun rolesKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rolesKey = mapped
}
/**
* @param value Duration of a session in minutes after a user logs in. Default is 60. Maximum value is 1,440.
*/
@JvmName("ejbioqvnrudiiakc")
public suspend fun sessionTimeoutMinutes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sessionTimeoutMinutes = mapped
}
/**
* @param value Element of the SAML assertion to use for username. Default is NameID.
*/
@JvmName("wundlwamtgpfmvvp")
public suspend fun subjectKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subjectKey = mapped
}
internal fun build(): DomainSamlOptionsSamlOptionsArgs = DomainSamlOptionsSamlOptionsArgs(
enabled = enabled,
idp = idp,
masterBackendRole = masterBackendRole,
masterUserName = masterUserName,
rolesKey = rolesKey,
sessionTimeoutMinutes = sessionTimeoutMinutes,
subjectKey = subjectKey,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy