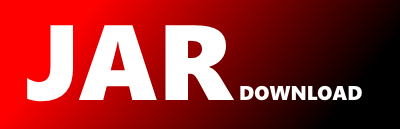
com.pulumi.aws.paymentcryptography.kotlin.inputs.KeyKeyAttributesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.paymentcryptography.kotlin.inputs
import com.pulumi.aws.paymentcryptography.inputs.KeyKeyAttributesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property keyAlgorithm Key algorithm to be use during creation of an AWS Payment Cryptography key.
* @property keyClass Type of AWS Payment Cryptography key to create.
* @property keyModesOfUse List of cryptographic operations that you can perform using the key.
* @property keyUsage Cryptographic usage of an AWS Payment Cryptography key as defined in section A.5.2 of the TR-31 spec.
*/
public data class KeyKeyAttributesArgs(
public val keyAlgorithm: Output,
public val keyClass: Output,
public val keyModesOfUse: Output? = null,
public val keyUsage: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.paymentcryptography.inputs.KeyKeyAttributesArgs =
com.pulumi.aws.paymentcryptography.inputs.KeyKeyAttributesArgs.builder()
.keyAlgorithm(keyAlgorithm.applyValue({ args0 -> args0 }))
.keyClass(keyClass.applyValue({ args0 -> args0 }))
.keyModesOfUse(keyModesOfUse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.keyUsage(keyUsage.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KeyKeyAttributesArgs].
*/
@PulumiTagMarker
public class KeyKeyAttributesArgsBuilder internal constructor() {
private var keyAlgorithm: Output? = null
private var keyClass: Output? = null
private var keyModesOfUse: Output? = null
private var keyUsage: Output? = null
/**
* @param value Key algorithm to be use during creation of an AWS Payment Cryptography key.
*/
@JvmName("qwhlbtfjupppljph")
public suspend fun keyAlgorithm(`value`: Output) {
this.keyAlgorithm = value
}
/**
* @param value Type of AWS Payment Cryptography key to create.
*/
@JvmName("vlfykbglfvqgqekw")
public suspend fun keyClass(`value`: Output) {
this.keyClass = value
}
/**
* @param value List of cryptographic operations that you can perform using the key.
*/
@JvmName("mfqetlkcrnqxserm")
public suspend fun keyModesOfUse(`value`: Output) {
this.keyModesOfUse = value
}
/**
* @param value Cryptographic usage of an AWS Payment Cryptography key as defined in section A.5.2 of the TR-31 spec.
*/
@JvmName("rjnkueuyxjmjgetr")
public suspend fun keyUsage(`value`: Output) {
this.keyUsage = value
}
/**
* @param value Key algorithm to be use during creation of an AWS Payment Cryptography key.
*/
@JvmName("buimxqgrmqdttesa")
public suspend fun keyAlgorithm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyAlgorithm = mapped
}
/**
* @param value Type of AWS Payment Cryptography key to create.
*/
@JvmName("rabxgarosoyggswb")
public suspend fun keyClass(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyClass = mapped
}
/**
* @param value List of cryptographic operations that you can perform using the key.
*/
@JvmName("bmdbbcfkfmnglunb")
public suspend fun keyModesOfUse(`value`: KeyKeyAttributesKeyModesOfUseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyModesOfUse = mapped
}
/**
* @param argument List of cryptographic operations that you can perform using the key.
*/
@JvmName("sjrqmjhykmcmuxcj")
public suspend fun keyModesOfUse(argument: suspend KeyKeyAttributesKeyModesOfUseArgsBuilder.() -> Unit) {
val toBeMapped = KeyKeyAttributesKeyModesOfUseArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.keyModesOfUse = mapped
}
/**
* @param value Cryptographic usage of an AWS Payment Cryptography key as defined in section A.5.2 of the TR-31 spec.
*/
@JvmName("iirwqblxkxwpcpxq")
public suspend fun keyUsage(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyUsage = mapped
}
internal fun build(): KeyKeyAttributesArgs = KeyKeyAttributesArgs(
keyAlgorithm = keyAlgorithm ?: throw PulumiNullFieldException("keyAlgorithm"),
keyClass = keyClass ?: throw PulumiNullFieldException("keyClass"),
keyModesOfUse = keyModesOfUse,
keyUsage = keyUsage ?: throw PulumiNullFieldException("keyUsage"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy