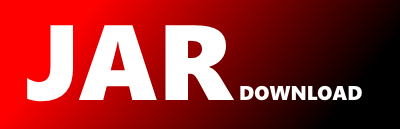
com.pulumi.aws.pinpoint.kotlin.ApnsVoipSandboxChannelArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.pinpoint.kotlin
import com.pulumi.aws.pinpoint.ApnsVoipSandboxChannelArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a Pinpoint APNs VoIP Sandbox Channel resource.
* > **Note:** All arguments, including certificates and tokens, will be stored in the raw state as plain-text.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* import * as std from "@pulumi/std";
* const app = new aws.pinpoint.App("app", {});
* const apnsVoipSandbox = new aws.pinpoint.ApnsVoipSandboxChannel("apns_voip_sandbox", {
* applicationId: app.applicationId,
* certificate: std.file({
* input: "./certificate.pem",
* }).then(invoke => invoke.result),
* privateKey: std.file({
* input: "./private_key.key",
* }).then(invoke => invoke.result),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* import pulumi_std as std
* app = aws.pinpoint.App("app")
* apns_voip_sandbox = aws.pinpoint.ApnsVoipSandboxChannel("apns_voip_sandbox",
* application_id=app.application_id,
* certificate=std.file(input="./certificate.pem").result,
* private_key=std.file(input="./private_key.key").result)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var app = new Aws.Pinpoint.App("app");
* var apnsVoipSandbox = new Aws.Pinpoint.ApnsVoipSandboxChannel("apns_voip_sandbox", new()
* {
* ApplicationId = app.ApplicationId,
* Certificate = Std.File.Invoke(new()
* {
* Input = "./certificate.pem",
* }).Apply(invoke => invoke.Result),
* PrivateKey = Std.File.Invoke(new()
* {
* Input = "./private_key.key",
* }).Apply(invoke => invoke.Result),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/pinpoint"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* app, err := pinpoint.NewApp(ctx, "app", nil)
* if err != nil {
* return err
* }
* invokeFile, err := std.File(ctx, &std.FileArgs{
* Input: "./certificate.pem",
* }, nil)
* if err != nil {
* return err
* }
* invokeFile1, err := std.File(ctx, &std.FileArgs{
* Input: "./private_key.key",
* }, nil)
* if err != nil {
* return err
* }
* _, err = pinpoint.NewApnsVoipSandboxChannel(ctx, "apns_voip_sandbox", &pinpoint.ApnsVoipSandboxChannelArgs{
* ApplicationId: app.ApplicationId,
* Certificate: pulumi.String(invokeFile.Result),
* PrivateKey: pulumi.String(invokeFile1.Result),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.pinpoint.App;
* import com.pulumi.aws.pinpoint.ApnsVoipSandboxChannel;
* import com.pulumi.aws.pinpoint.ApnsVoipSandboxChannelArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var app = new App("app");
* var apnsVoipSandbox = new ApnsVoipSandboxChannel("apnsVoipSandbox", ApnsVoipSandboxChannelArgs.builder()
* .applicationId(app.applicationId())
* .certificate(StdFunctions.file(FileArgs.builder()
* .input("./certificate.pem")
* .build()).result())
* .privateKey(StdFunctions.file(FileArgs.builder()
* .input("./private_key.key")
* .build()).result())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* apnsVoipSandbox:
* type: aws:pinpoint:ApnsVoipSandboxChannel
* name: apns_voip_sandbox
* properties:
* applicationId: ${app.applicationId}
* certificate:
* fn::invoke:
* Function: std:file
* Arguments:
* input: ./certificate.pem
* Return: result
* privateKey:
* fn::invoke:
* Function: std:file
* Arguments:
* input: ./private_key.key
* Return: result
* app:
* type: aws:pinpoint:App
* ```
*
* ## Import
* Using `pulumi import`, import Pinpoint APNs VoIP Sandbox Channel using the `application-id`. For example:
* ```sh
* $ pulumi import aws:pinpoint/apnsVoipSandboxChannel:ApnsVoipSandboxChannel apns_voip_sandbox application-id
* ```
* @property applicationId The application ID.
* @property bundleId The ID assigned to your iOS app. To find this value, choose Certificates, IDs & Profiles, choose App IDs in the Identifiers section, and choose your app.
* @property certificate The pem encoded TLS Certificate from Apple.
* @property defaultAuthenticationMethod The default authentication method used for APNs.
* __NOTE__: Amazon Pinpoint uses this default for every APNs push notification that you send using the console.
* You can override the default when you send a message programmatically using the Amazon Pinpoint API, the AWS CLI, or an AWS SDK.
* If your default authentication type fails, Amazon Pinpoint doesn't attempt to use the other authentication type.
* One of the following sets of credentials is also required.
* If you choose to use __Certificate credentials__ you will have to provide:
* @property enabled Whether the channel is enabled or disabled. Defaults to `true`.
* @property privateKey The Certificate Private Key file (ie. `.key` file).
* If you choose to use __Key credentials__ you will have to provide:
* @property teamId The ID assigned to your Apple developer account team. This value is provided on the Membership page.
* @property tokenKey The `.p8` file that you download from your Apple developer account when you create an authentication key.
* @property tokenKeyId The ID assigned to your signing key. To find this value, choose Certificates, IDs & Profiles, and choose your key in the Keys section.
*/
public data class ApnsVoipSandboxChannelArgs(
public val applicationId: Output? = null,
public val bundleId: Output? = null,
public val certificate: Output? = null,
public val defaultAuthenticationMethod: Output? = null,
public val enabled: Output? = null,
public val privateKey: Output? = null,
public val teamId: Output? = null,
public val tokenKey: Output? = null,
public val tokenKeyId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.pinpoint.ApnsVoipSandboxChannelArgs =
com.pulumi.aws.pinpoint.ApnsVoipSandboxChannelArgs.builder()
.applicationId(applicationId?.applyValue({ args0 -> args0 }))
.bundleId(bundleId?.applyValue({ args0 -> args0 }))
.certificate(certificate?.applyValue({ args0 -> args0 }))
.defaultAuthenticationMethod(defaultAuthenticationMethod?.applyValue({ args0 -> args0 }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.privateKey(privateKey?.applyValue({ args0 -> args0 }))
.teamId(teamId?.applyValue({ args0 -> args0 }))
.tokenKey(tokenKey?.applyValue({ args0 -> args0 }))
.tokenKeyId(tokenKeyId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApnsVoipSandboxChannelArgs].
*/
@PulumiTagMarker
public class ApnsVoipSandboxChannelArgsBuilder internal constructor() {
private var applicationId: Output? = null
private var bundleId: Output? = null
private var certificate: Output? = null
private var defaultAuthenticationMethod: Output? = null
private var enabled: Output? = null
private var privateKey: Output? = null
private var teamId: Output? = null
private var tokenKey: Output? = null
private var tokenKeyId: Output? = null
/**
* @param value The application ID.
*/
@JvmName("pcnfrrysxfbxyvml")
public suspend fun applicationId(`value`: Output) {
this.applicationId = value
}
/**
* @param value The ID assigned to your iOS app. To find this value, choose Certificates, IDs & Profiles, choose App IDs in the Identifiers section, and choose your app.
*/
@JvmName("sskyijvmalwequxn")
public suspend fun bundleId(`value`: Output) {
this.bundleId = value
}
/**
* @param value The pem encoded TLS Certificate from Apple.
*/
@JvmName("rigvtvtkymbcersw")
public suspend fun certificate(`value`: Output) {
this.certificate = value
}
/**
* @param value The default authentication method used for APNs.
* __NOTE__: Amazon Pinpoint uses this default for every APNs push notification that you send using the console.
* You can override the default when you send a message programmatically using the Amazon Pinpoint API, the AWS CLI, or an AWS SDK.
* If your default authentication type fails, Amazon Pinpoint doesn't attempt to use the other authentication type.
* One of the following sets of credentials is also required.
* If you choose to use __Certificate credentials__ you will have to provide:
*/
@JvmName("rjskkvfcodyacvvb")
public suspend fun defaultAuthenticationMethod(`value`: Output) {
this.defaultAuthenticationMethod = value
}
/**
* @param value Whether the channel is enabled or disabled. Defaults to `true`.
*/
@JvmName("wkfgfhvehwnkcgrk")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value The Certificate Private Key file (ie. `.key` file).
* If you choose to use __Key credentials__ you will have to provide:
*/
@JvmName("eydlrbdgrvrltyul")
public suspend fun privateKey(`value`: Output) {
this.privateKey = value
}
/**
* @param value The ID assigned to your Apple developer account team. This value is provided on the Membership page.
*/
@JvmName("opxuwirinrdbopmw")
public suspend fun teamId(`value`: Output) {
this.teamId = value
}
/**
* @param value The `.p8` file that you download from your Apple developer account when you create an authentication key.
*/
@JvmName("qyhwajhlsavmkpdf")
public suspend fun tokenKey(`value`: Output) {
this.tokenKey = value
}
/**
* @param value The ID assigned to your signing key. To find this value, choose Certificates, IDs & Profiles, and choose your key in the Keys section.
*/
@JvmName("qyijxndfprrbbuwi")
public suspend fun tokenKeyId(`value`: Output) {
this.tokenKeyId = value
}
/**
* @param value The application ID.
*/
@JvmName("ojxbvgkvioqsrseq")
public suspend fun applicationId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applicationId = mapped
}
/**
* @param value The ID assigned to your iOS app. To find this value, choose Certificates, IDs & Profiles, choose App IDs in the Identifiers section, and choose your app.
*/
@JvmName("oetgxkjvjdbxjkdb")
public suspend fun bundleId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bundleId = mapped
}
/**
* @param value The pem encoded TLS Certificate from Apple.
*/
@JvmName("oriimuosbdwhwwat")
public suspend fun certificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificate = mapped
}
/**
* @param value The default authentication method used for APNs.
* __NOTE__: Amazon Pinpoint uses this default for every APNs push notification that you send using the console.
* You can override the default when you send a message programmatically using the Amazon Pinpoint API, the AWS CLI, or an AWS SDK.
* If your default authentication type fails, Amazon Pinpoint doesn't attempt to use the other authentication type.
* One of the following sets of credentials is also required.
* If you choose to use __Certificate credentials__ you will have to provide:
*/
@JvmName("ivdfuxhoryalvsww")
public suspend fun defaultAuthenticationMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultAuthenticationMethod = mapped
}
/**
* @param value Whether the channel is enabled or disabled. Defaults to `true`.
*/
@JvmName("viwfyfosmysatcaf")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value The Certificate Private Key file (ie. `.key` file).
* If you choose to use __Key credentials__ you will have to provide:
*/
@JvmName("nujbgqstpgjvajio")
public suspend fun privateKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateKey = mapped
}
/**
* @param value The ID assigned to your Apple developer account team. This value is provided on the Membership page.
*/
@JvmName("xdguxcoohraqivae")
public suspend fun teamId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.teamId = mapped
}
/**
* @param value The `.p8` file that you download from your Apple developer account when you create an authentication key.
*/
@JvmName("prtgjqfxmjqwpwvc")
public suspend fun tokenKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenKey = mapped
}
/**
* @param value The ID assigned to your signing key. To find this value, choose Certificates, IDs & Profiles, and choose your key in the Keys section.
*/
@JvmName("pwoergolceuvsess")
public suspend fun tokenKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenKeyId = mapped
}
internal fun build(): ApnsVoipSandboxChannelArgs = ApnsVoipSandboxChannelArgs(
applicationId = applicationId,
bundleId = bundleId,
certificate = certificate,
defaultAuthenticationMethod = defaultAuthenticationMethod,
enabled = enabled,
privateKey = privateKey,
teamId = teamId,
tokenKey = tokenKey,
tokenKeyId = tokenKeyId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy