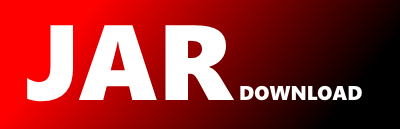
com.pulumi.aws.pinpoint.kotlin.EventStream.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.pinpoint.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [EventStream].
*/
@PulumiTagMarker
public class EventStreamResourceBuilder internal constructor() {
public var name: String? = null
public var args: EventStreamArgs = EventStreamArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EventStreamArgsBuilder.() -> Unit) {
val builder = EventStreamArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EventStream {
val builtJavaResource = com.pulumi.aws.pinpoint.EventStream(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EventStream(builtJavaResource)
}
}
/**
* /* /* /* /* /* /*
* Provides a Pinpoint Event Stream resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const app = new aws.pinpoint.App("app", {});
* const testStream = new aws.kinesis.Stream("test_stream", {
* name: "pinpoint-kinesis-test",
* shardCount: 1,
* });
* const assumeRole = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["pinpoint.us-east-1.amazonaws.com"],
* }],
* actions: ["sts:AssumeRole"],
* }],
* });
* const testRole = new aws.iam.Role("test_role", {assumeRolePolicy: assumeRole.then(assumeRole => assumeRole.json)});
* const stream = new aws.pinpoint.EventStream("stream", {
* applicationId: app.applicationId,
* destinationStreamArn: testStream.arn,
* roleArn: testRole.arn,
* });
* const testRolePolicy = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* actions: [
* "kinesis:PutRecords",
* "kinesis:DescribeStream",
* ],
* resources: ["arn:aws:kinesis:us-east-1:*:*/*"],
* }],
* });
* const testRolePolicyRolePolicy = new aws.iam.RolePolicy("test_role_policy", {
* name: "test_policy",
* role: testRole.id,
* policy: testRolePolicy.then(testRolePolicy => testRolePolicy.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* app = aws.pinpoint.App("app")
* test_stream = aws.kinesis.Stream("test_stream",
* name="pinpoint-kinesis-test",
* shard_count=1)
* assume_role = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["pinpoint.us-east-1.amazonaws.com"],
* }],
* "actions": ["sts:AssumeRole"],
* }])
* test_role = aws.iam.Role("test_role", assume_role_policy=assume_role.json)
* stream = aws.pinpoint.EventStream("stream",
* application_id=app.application_id,
* destination_stream_arn=test_stream.arn,
* role_arn=test_role.arn)
* test_role_policy = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "actions": [
* "kinesis:PutRecords",
* "kinesis:DescribeStream",
* ],
* "resources": ["arn:aws:kinesis:us-east-1:*:*/*"],
* }])
* test_role_policy_role_policy = aws.iam.RolePolicy("test_role_policy",
* name="test_policy",
* role=test_role.id,
* policy=test_role_policy.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var app = new Aws.Pinpoint.App("app");
* var testStream = new Aws.Kinesis.Stream("test_stream", new()
* {
* Name = "pinpoint-kinesis-test",
* ShardCount = 1,
* });
* var assumeRole = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "pinpoint.us-east-1.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* },
* },
* });
* var testRole = new Aws.Iam.Role("test_role", new()
* {
* AssumeRolePolicy = assumeRole.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var stream = new Aws.Pinpoint.EventStream("stream", new()
* {
* ApplicationId = app.ApplicationId,
* DestinationStreamArn = testStream.Arn,
* RoleArn = testRole.Arn,
* });
* var testRolePolicy = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Actions = new[]
* {
* "kinesis:PutRecords",
* "kinesis:DescribeStream",
* },
* Resources = new[]
* {
* "arn:aws:kinesis:us-east-1:*:*/*",
* },
* },
* },
* });
* var testRolePolicyRolePolicy = new Aws.Iam.RolePolicy("test_role_policy", new()
* {
* Name = "test_policy",
* Role = testRole.Id,
* Policy = testRolePolicy.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kinesis"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/pinpoint"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* app, err := pinpoint.NewApp(ctx, "app", nil)
* if err != nil {
* return err
* }
* testStream, err := kinesis.NewStream(ctx, "test_stream", &kinesis.StreamArgs{
* Name: pulumi.String("pinpoint-kinesis-test"),
* ShardCount: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* assumeRole, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "pinpoint.us-east-1.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "sts:AssumeRole",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* testRole, err := iam.NewRole(ctx, "test_role", &iam.RoleArgs{
* AssumeRolePolicy: pulumi.String(assumeRole.Json),
* })
* if err != nil {
* return err
* }
* _, err = pinpoint.NewEventStream(ctx, "stream", &pinpoint.EventStreamArgs{
* ApplicationId: app.ApplicationId,
* DestinationStreamArn: testStream.Arn,
* RoleArn: testRole.Arn,
* })
* if err != nil {
* return err
* }
* testRolePolicy, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Actions: []string{
* "kinesis:PutRecords",
* "kinesis:DescribeStream",
* },
* Resources: []string{
* "arn:aws:kinesis:us-east-1:*:*/*",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* _, err = iam.NewRolePolicy(ctx, "test_role_policy", &iam.RolePolicyArgs{
* Name: pulumi.String("test_policy"),
* Role: testRole.ID(),
* Policy: pulumi.String(testRolePolicy.Json),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.pinpoint.App;
* import com.pulumi.aws.kinesis.Stream;
* import com.pulumi.aws.kinesis.StreamArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.pinpoint.EventStream;
* import com.pulumi.aws.pinpoint.EventStreamArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var app = new App("app");
* var testStream = new Stream("testStream", StreamArgs.builder()
* .name("pinpoint-kinesis-test")
* .shardCount(1)
* .build());
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("pinpoint.us-east-1.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
* var testRole = new Role("testRole", RoleArgs.builder()
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var stream = new EventStream("stream", EventStreamArgs.builder()
* .applicationId(app.applicationId())
* .destinationStreamArn(testStream.arn())
* .roleArn(testRole.arn())
* .build());
* final var testRolePolicy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "kinesis:PutRecords",
* "kinesis:DescribeStream")
* .resources("arn:aws:kinesis:us-east-1:*:*/*")
* .build())
* .build());
* var testRolePolicyRolePolicy = new RolePolicy("testRolePolicyRolePolicy", RolePolicyArgs.builder()
* .name("test_policy")
* .role(testRole.id())
* .policy(testRolePolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* stream:
* type: aws:pinpoint:EventStream
* properties:
* applicationId: ${app.applicationId}
* destinationStreamArn: ${testStream.arn}
* roleArn: ${testRole.arn}
* app:
* type: aws:pinpoint:App
* testStream:
* type: aws:kinesis:Stream
* name: test_stream
* properties:
* name: pinpoint-kinesis-test
* shardCount: 1
* testRole:
* type: aws:iam:Role
* name: test_role
* properties:
* assumeRolePolicy: ${assumeRole.json}
* testRolePolicyRolePolicy:
* type: aws:iam:RolePolicy
* name: test_role_policy
* properties:
* name: test_policy
* role: ${testRole.id}
* policy: ${testRolePolicy.json}
* variables:
* assumeRole:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: Service
* identifiers:
* - pinpoint.us-east-1.amazonaws.com
* actions:
* - sts:AssumeRole
* testRolePolicy:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* actions:
* - kinesis:PutRecords
* - kinesis:DescribeStream
* resources:
* - arn:aws:kinesis:us-east-1:*:*/*
* ```
*
* ## Import
* Using `pulumi import`, import Pinpoint Event Stream using the `application-id`. For example:
* ```sh
* $ pulumi import aws:pinpoint/eventStream:EventStream stream application-id
* ```
*/
public class EventStream internal constructor(
override val javaResource: com.pulumi.aws.pinpoint.EventStream,
) : KotlinCustomResource(javaResource, EventStreamMapper) {
/**
* The application ID.
*/
public val applicationId: Output
get() = javaResource.applicationId().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of the Amazon Kinesis stream or Firehose delivery stream to which you want to publish events.
*/
public val destinationStreamArn: Output
get() = javaResource.destinationStreamArn().applyValue({ args0 -> args0 })
/**
* The IAM role that authorizes Amazon Pinpoint to publish events to the stream in your account.
*/
public val roleArn: Output
get() = javaResource.roleArn().applyValue({ args0 -> args0 })
}
public object EventStreamMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.pinpoint.EventStream::class == javaResource::class
override fun map(javaResource: Resource): EventStream = EventStream(
javaResource as
com.pulumi.aws.pinpoint.EventStream,
)
}
/**
* @see [EventStream].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [EventStream].
*/
public suspend fun eventStream(name: String, block: suspend EventStreamResourceBuilder.() -> Unit): EventStream {
val builder = EventStreamResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [EventStream].
* @param name The _unique_ name of the resulting resource.
*/
public fun eventStream(name: String): EventStream {
val builder = EventStreamResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy