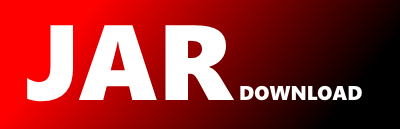
com.pulumi.aws.quicksight.kotlin.AnalysisArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.quicksight.kotlin
import com.pulumi.aws.quicksight.AnalysisArgs.builder
import com.pulumi.aws.quicksight.kotlin.inputs.AnalysisParametersArgs
import com.pulumi.aws.quicksight.kotlin.inputs.AnalysisParametersArgsBuilder
import com.pulumi.aws.quicksight.kotlin.inputs.AnalysisPermissionArgs
import com.pulumi.aws.quicksight.kotlin.inputs.AnalysisPermissionArgsBuilder
import com.pulumi.aws.quicksight.kotlin.inputs.AnalysisSourceEntityArgs
import com.pulumi.aws.quicksight.kotlin.inputs.AnalysisSourceEntityArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource for managing a QuickSight Analysis.
* ## Example Usage
* ### From Source Template
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.quicksight.Analysis("example", {
* analysisId: "example-id",
* name: "example-name",
* sourceEntity: {
* sourceTemplate: {
* arn: source.arn,
* dataSetReferences: [{
* dataSetArn: dataset.arn,
* dataSetPlaceholder: "1",
* }],
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.quicksight.Analysis("example",
* analysis_id="example-id",
* name="example-name",
* source_entity={
* "source_template": {
* "arn": source["arn"],
* "data_set_references": [{
* "data_set_arn": dataset["arn"],
* "data_set_placeholder": "1",
* }],
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Quicksight.Analysis("example", new()
* {
* AnalysisId = "example-id",
* Name = "example-name",
* SourceEntity = new Aws.Quicksight.Inputs.AnalysisSourceEntityArgs
* {
* SourceTemplate = new Aws.Quicksight.Inputs.AnalysisSourceEntitySourceTemplateArgs
* {
* Arn = source.Arn,
* DataSetReferences = new[]
* {
* new Aws.Quicksight.Inputs.AnalysisSourceEntitySourceTemplateDataSetReferenceArgs
* {
* DataSetArn = dataset.Arn,
* DataSetPlaceholder = "1",
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/quicksight"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := quicksight.NewAnalysis(ctx, "example", &quicksight.AnalysisArgs{
* AnalysisId: pulumi.String("example-id"),
* Name: pulumi.String("example-name"),
* SourceEntity: &quicksight.AnalysisSourceEntityArgs{
* SourceTemplate: &quicksight.AnalysisSourceEntitySourceTemplateArgs{
* Arn: pulumi.Any(source.Arn),
* DataSetReferences: quicksight.AnalysisSourceEntitySourceTemplateDataSetReferenceArray{
* &quicksight.AnalysisSourceEntitySourceTemplateDataSetReferenceArgs{
* DataSetArn: pulumi.Any(dataset.Arn),
* DataSetPlaceholder: pulumi.String("1"),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.quicksight.Analysis;
* import com.pulumi.aws.quicksight.AnalysisArgs;
* import com.pulumi.aws.quicksight.inputs.AnalysisSourceEntityArgs;
* import com.pulumi.aws.quicksight.inputs.AnalysisSourceEntitySourceTemplateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Analysis("example", AnalysisArgs.builder()
* .analysisId("example-id")
* .name("example-name")
* .sourceEntity(AnalysisSourceEntityArgs.builder()
* .sourceTemplate(AnalysisSourceEntitySourceTemplateArgs.builder()
* .arn(source.arn())
* .dataSetReferences(AnalysisSourceEntitySourceTemplateDataSetReferenceArgs.builder()
* .dataSetArn(dataset.arn())
* .dataSetPlaceholder("1")
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:quicksight:Analysis
* properties:
* analysisId: example-id
* name: example-name
* sourceEntity:
* sourceTemplate:
* arn: ${source.arn}
* dataSetReferences:
* - dataSetArn: ${dataset.arn}
* dataSetPlaceholder: '1'
* ```
*
* ### With Definition
*
* ```yaml
* resources:
* example:
* type: aws:quicksight:Analysis
* properties:
* analysisId: example-id
* name: example-name
* definition:
* dataSetIdentifiersDeclarations:
* - dataSetArn: ${dataset.arn}
* identifier: '1'
* sheets:
* - title: Example
* sheetId: Example1
* visuals:
* - lineChartVisual:
* visualId: LineChart
* title:
* formatText:
* plainText: Line Chart Example
* chartConfiguration:
* fieldWells:
* lineChartAggregatedFieldWells:
* categories:
* - categoricalDimensionField:
* fieldId: '1'
* column:
* dataSetIdentifier: '1'
* columnName: Column1
* values:
* - categoricalMeasureField:
* fieldId: '2'
* column:
* dataSetIdentifier: '1'
* columnName: Column1
* aggregationFunction: COUNT
* ```
*
* ## Import
* Using `pulumi import`, import a QuickSight Analysis using the AWS account ID and analysis ID separated by a comma (`,`). For example:
* ```sh
* $ pulumi import aws:quicksight/analysis:Analysis example 123456789012,example-id
* ```
* @property analysisId Identifier for the analysis.
* @property awsAccountId AWS account ID.
* @property name Display name for the analysis.
* The following arguments are optional:
* @property parameters The parameters for the creation of the analysis, which you want to use to override the default settings. An analysis can have any type of parameters, and some parameters might accept multiple values. See parameters.
* @property permissions A set of resource permissions on the analysis. Maximum of 64 items. See permissions.
* @property recoveryWindowInDays A value that specifies the number of days that Amazon QuickSight waits before it deletes the analysis. Use `0` to force deletion without recovery. Minimum value of `7`. Maximum value of `30`. Default to `30`.
* @property sourceEntity The entity that you are using as a source when you create the analysis (template). Only one of `definition` or `source_entity` should be configured. See source_entity.
* @property tags Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property themeArn The Amazon Resource Name (ARN) of the theme that is being used for this analysis. The theme ARN must exist in the same AWS account where you create the analysis.
*/
public data class AnalysisArgs(
public val analysisId: Output? = null,
public val awsAccountId: Output? = null,
public val name: Output? = null,
public val parameters: Output? = null,
public val permissions: Output>? = null,
public val recoveryWindowInDays: Output? = null,
public val sourceEntity: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy