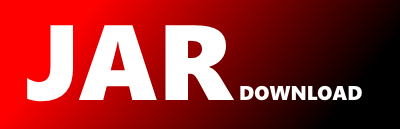
com.pulumi.aws.quicksight.kotlin.inputs.DataSetLogicalTableMapSourceJoinInstructionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.quicksight.kotlin.inputs
import com.pulumi.aws.quicksight.inputs.DataSetLogicalTableMapSourceJoinInstructionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property leftJoinKeyProperties Join key properties of the left operand. See left_join_key_properties.
* @property leftOperand Operand on the left side of a join.
* @property onClause Join instructions provided in the ON clause of a join.
* @property rightJoinKeyProperties Join key properties of the right operand. See right_join_key_properties.
* @property rightOperand Operand on the right side of a join.
* @property type Type of join. Valid values are `INNER`, `OUTER`, `LEFT`, and `RIGHT`.
*/
public data class DataSetLogicalTableMapSourceJoinInstructionArgs(
public val leftJoinKeyProperties: Output? = null,
public val leftOperand: Output,
public val onClause: Output,
public val rightJoinKeyProperties: Output? = null,
public val rightOperand: Output,
public val type: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.quicksight.inputs.DataSetLogicalTableMapSourceJoinInstructionArgs =
com.pulumi.aws.quicksight.inputs.DataSetLogicalTableMapSourceJoinInstructionArgs.builder()
.leftJoinKeyProperties(
leftJoinKeyProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.leftOperand(leftOperand.applyValue({ args0 -> args0 }))
.onClause(onClause.applyValue({ args0 -> args0 }))
.rightJoinKeyProperties(
rightJoinKeyProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rightOperand(rightOperand.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DataSetLogicalTableMapSourceJoinInstructionArgs].
*/
@PulumiTagMarker
public class DataSetLogicalTableMapSourceJoinInstructionArgsBuilder internal constructor() {
private var leftJoinKeyProperties:
Output? = null
private var leftOperand: Output? = null
private var onClause: Output? = null
private var rightJoinKeyProperties:
Output? = null
private var rightOperand: Output? = null
private var type: Output? = null
/**
* @param value Join key properties of the left operand. See left_join_key_properties.
*/
@JvmName("pvlpvntpgxvjbamn")
public suspend fun leftJoinKeyProperties(`value`: Output) {
this.leftJoinKeyProperties = value
}
/**
* @param value Operand on the left side of a join.
*/
@JvmName("rwygvohkpvchklst")
public suspend fun leftOperand(`value`: Output) {
this.leftOperand = value
}
/**
* @param value Join instructions provided in the ON clause of a join.
*/
@JvmName("ckmglhwxqnwbguqh")
public suspend fun onClause(`value`: Output) {
this.onClause = value
}
/**
* @param value Join key properties of the right operand. See right_join_key_properties.
*/
@JvmName("igmntypodqwipafx")
public suspend fun rightJoinKeyProperties(`value`: Output) {
this.rightJoinKeyProperties = value
}
/**
* @param value Operand on the right side of a join.
*/
@JvmName("ljtcthuuvahvshjl")
public suspend fun rightOperand(`value`: Output) {
this.rightOperand = value
}
/**
* @param value Type of join. Valid values are `INNER`, `OUTER`, `LEFT`, and `RIGHT`.
*/
@JvmName("fcbvcsqqnswvqlpm")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Join key properties of the left operand. See left_join_key_properties.
*/
@JvmName("dyoychaukidfoeer")
public suspend fun leftJoinKeyProperties(`value`: DataSetLogicalTableMapSourceJoinInstructionLeftJoinKeyPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.leftJoinKeyProperties = mapped
}
/**
* @param argument Join key properties of the left operand. See left_join_key_properties.
*/
@JvmName("qurrhhxqpctakwic")
public suspend fun leftJoinKeyProperties(argument: suspend DataSetLogicalTableMapSourceJoinInstructionLeftJoinKeyPropertiesArgsBuilder.() -> Unit) {
val toBeMapped =
DataSetLogicalTableMapSourceJoinInstructionLeftJoinKeyPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.leftJoinKeyProperties = mapped
}
/**
* @param value Operand on the left side of a join.
*/
@JvmName("ubpdhqbdlklighwb")
public suspend fun leftOperand(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.leftOperand = mapped
}
/**
* @param value Join instructions provided in the ON clause of a join.
*/
@JvmName("yvtyhnmekrqjsydx")
public suspend fun onClause(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.onClause = mapped
}
/**
* @param value Join key properties of the right operand. See right_join_key_properties.
*/
@JvmName("fekpolwrnjtqkvgj")
public suspend fun rightJoinKeyProperties(`value`: DataSetLogicalTableMapSourceJoinInstructionRightJoinKeyPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rightJoinKeyProperties = mapped
}
/**
* @param argument Join key properties of the right operand. See right_join_key_properties.
*/
@JvmName("oivwcddoxyaklolj")
public suspend fun rightJoinKeyProperties(argument: suspend DataSetLogicalTableMapSourceJoinInstructionRightJoinKeyPropertiesArgsBuilder.() -> Unit) {
val toBeMapped =
DataSetLogicalTableMapSourceJoinInstructionRightJoinKeyPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rightJoinKeyProperties = mapped
}
/**
* @param value Operand on the right side of a join.
*/
@JvmName("avrxowffifyvdwfb")
public suspend fun rightOperand(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.rightOperand = mapped
}
/**
* @param value Type of join. Valid values are `INNER`, `OUTER`, `LEFT`, and `RIGHT`.
*/
@JvmName("evlolhdswdeahpsd")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): DataSetLogicalTableMapSourceJoinInstructionArgs =
DataSetLogicalTableMapSourceJoinInstructionArgs(
leftJoinKeyProperties = leftJoinKeyProperties,
leftOperand = leftOperand ?: throw PulumiNullFieldException("leftOperand"),
onClause = onClause ?: throw PulumiNullFieldException("onClause"),
rightJoinKeyProperties = rightJoinKeyProperties,
rightOperand = rightOperand ?: throw PulumiNullFieldException("rightOperand"),
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy