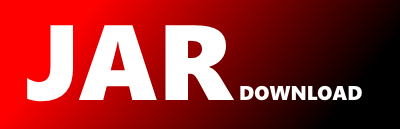
com.pulumi.aws.rds.kotlin.ClusterArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.rds.kotlin
import com.pulumi.aws.rds.ClusterArgs.builder
import com.pulumi.aws.rds.kotlin.enums.EngineMode
import com.pulumi.aws.rds.kotlin.enums.EngineType
import com.pulumi.aws.rds.kotlin.inputs.ClusterRestoreToPointInTimeArgs
import com.pulumi.aws.rds.kotlin.inputs.ClusterRestoreToPointInTimeArgsBuilder
import com.pulumi.aws.rds.kotlin.inputs.ClusterS3ImportArgs
import com.pulumi.aws.rds.kotlin.inputs.ClusterS3ImportArgsBuilder
import com.pulumi.aws.rds.kotlin.inputs.ClusterScalingConfigurationArgs
import com.pulumi.aws.rds.kotlin.inputs.ClusterScalingConfigurationArgsBuilder
import com.pulumi.aws.rds.kotlin.inputs.ClusterServerlessv2ScalingConfigurationArgs
import com.pulumi.aws.rds.kotlin.inputs.ClusterServerlessv2ScalingConfigurationArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a [RDS Aurora Cluster](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/CHAP_Aurora.html) or a [RDS Multi-AZ DB Cluster](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/multi-az-db-clusters-concepts.html). To manage cluster instances that inherit configuration from the cluster (when not running the cluster in `serverless` engine mode), see the `aws.rds.ClusterInstance` resource. To manage non-Aurora DB instances (e.g., MySQL, PostgreSQL, SQL Server, etc.), see the `aws.rds.Instance` resource.
* For information on the difference between the available Aurora MySQL engines see [Comparison between Aurora MySQL 1 and Aurora MySQL 2](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/AuroraMySQL.Updates.20180206.html) in the Amazon RDS User Guide.
* Changes to an RDS Cluster can occur when you manually change a parameter, such as `port`, and are reflected in the next maintenance window. Because of this, this provider may report a difference in its planning phase because a modification has not yet taken place. You can use the `apply_immediately` flag to instruct the service to apply the change immediately (see documentation below).
* > **Note:** Multi-AZ DB clusters are supported only for the MySQL and PostgreSQL DB engines.
* > **Note:** `ca_certificate_identifier` is only supported for Multi-AZ DB clusters.
* > **Note:** using `apply_immediately` can result in a brief downtime as the server reboots. See the AWS Docs on [RDS Maintenance](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_UpgradeDBInstance.Maintenance.html) for more information.
* > **Note:** All arguments including the username and password will be stored in the raw state as plain-text.
* > **NOTE on RDS Clusters and RDS Cluster Role Associations:** Pulumi provides both a standalone RDS Cluster Role Association - (an association between an RDS Cluster and a single IAM Role) and an RDS Cluster resource with `iam_roles` attributes. Use one resource or the other to associate IAM Roles and RDS Clusters. Not doing so will cause a conflict of associations and will result in the association being overwritten.
* ## Example Usage
* ### Aurora MySQL 2.x (MySQL 5.7)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _default = new aws.rds.Cluster("default", {
* clusterIdentifier: "aurora-cluster-demo",
* engine: aws.rds.EngineType.AuroraMysql,
* engineVersion: "5.7.mysql_aurora.2.03.2",
* availabilityZones: [
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* databaseName: "mydb",
* masterUsername: "foo",
* masterPassword: "must_be_eight_characters",
* backupRetentionPeriod: 5,
* preferredBackupWindow: "07:00-09:00",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default = aws.rds.Cluster("default",
* cluster_identifier="aurora-cluster-demo",
* engine=aws.rds.EngineType.AURORA_MYSQL,
* engine_version="5.7.mysql_aurora.2.03.2",
* availability_zones=[
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* database_name="mydb",
* master_username="foo",
* master_password="must_be_eight_characters",
* backup_retention_period=5,
* preferred_backup_window="07:00-09:00")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Aws.Rds.Cluster("default", new()
* {
* ClusterIdentifier = "aurora-cluster-demo",
* Engine = Aws.Rds.EngineType.AuroraMysql,
* EngineVersion = "5.7.mysql_aurora.2.03.2",
* AvailabilityZones = new[]
* {
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* },
* DatabaseName = "mydb",
* MasterUsername = "foo",
* MasterPassword = "must_be_eight_characters",
* BackupRetentionPeriod = 5,
* PreferredBackupWindow = "07:00-09:00",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rds.NewCluster(ctx, "default", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("aurora-cluster-demo"),
* Engine: pulumi.String(rds.EngineTypeAuroraMysql),
* EngineVersion: pulumi.String("5.7.mysql_aurora.2.03.2"),
* AvailabilityZones: pulumi.StringArray{
* pulumi.String("us-west-2a"),
* pulumi.String("us-west-2b"),
* pulumi.String("us-west-2c"),
* },
* DatabaseName: pulumi.String("mydb"),
* MasterUsername: pulumi.String("foo"),
* MasterPassword: pulumi.String("must_be_eight_characters"),
* BackupRetentionPeriod: pulumi.Int(5),
* PreferredBackupWindow: pulumi.String("07:00-09:00"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("aurora-cluster-demo")
* .engine("aurora-mysql")
* .engineVersion("5.7.mysql_aurora.2.03.2")
* .availabilityZones(
* "us-west-2a",
* "us-west-2b",
* "us-west-2c")
* .databaseName("mydb")
* .masterUsername("foo")
* .masterPassword("must_be_eight_characters")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: aurora-cluster-demo
* engine: aurora-mysql
* engineVersion: 5.7.mysql_aurora.2.03.2
* availabilityZones:
* - us-west-2a
* - us-west-2b
* - us-west-2c
* databaseName: mydb
* masterUsername: foo
* masterPassword: must_be_eight_characters
* backupRetentionPeriod: 5
* preferredBackupWindow: 07:00-09:00
* ```
*
* ### Aurora MySQL 1.x (MySQL 5.6)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _default = new aws.rds.Cluster("default", {
* clusterIdentifier: "aurora-cluster-demo",
* availabilityZones: [
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* databaseName: "mydb",
* masterUsername: "foo",
* masterPassword: "must_be_eight_characters",
* backupRetentionPeriod: 5,
* preferredBackupWindow: "07:00-09:00",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default = aws.rds.Cluster("default",
* cluster_identifier="aurora-cluster-demo",
* availability_zones=[
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* database_name="mydb",
* master_username="foo",
* master_password="must_be_eight_characters",
* backup_retention_period=5,
* preferred_backup_window="07:00-09:00")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Aws.Rds.Cluster("default", new()
* {
* ClusterIdentifier = "aurora-cluster-demo",
* AvailabilityZones = new[]
* {
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* },
* DatabaseName = "mydb",
* MasterUsername = "foo",
* MasterPassword = "must_be_eight_characters",
* BackupRetentionPeriod = 5,
* PreferredBackupWindow = "07:00-09:00",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rds.NewCluster(ctx, "default", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("aurora-cluster-demo"),
* AvailabilityZones: pulumi.StringArray{
* pulumi.String("us-west-2a"),
* pulumi.String("us-west-2b"),
* pulumi.String("us-west-2c"),
* },
* DatabaseName: pulumi.String("mydb"),
* MasterUsername: pulumi.String("foo"),
* MasterPassword: pulumi.String("must_be_eight_characters"),
* BackupRetentionPeriod: pulumi.Int(5),
* PreferredBackupWindow: pulumi.String("07:00-09:00"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("aurora-cluster-demo")
* .availabilityZones(
* "us-west-2a",
* "us-west-2b",
* "us-west-2c")
* .databaseName("mydb")
* .masterUsername("foo")
* .masterPassword("must_be_eight_characters")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: aurora-cluster-demo
* availabilityZones:
* - us-west-2a
* - us-west-2b
* - us-west-2c
* databaseName: mydb
* masterUsername: foo
* masterPassword: must_be_eight_characters
* backupRetentionPeriod: 5
* preferredBackupWindow: 07:00-09:00
* ```
*
* ### Aurora with PostgreSQL engine
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const postgresql = new aws.rds.Cluster("postgresql", {
* clusterIdentifier: "aurora-cluster-demo",
* engine: aws.rds.EngineType.AuroraPostgresql,
* availabilityZones: [
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* databaseName: "mydb",
* masterUsername: "foo",
* masterPassword: "must_be_eight_characters",
* backupRetentionPeriod: 5,
* preferredBackupWindow: "07:00-09:00",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* postgresql = aws.rds.Cluster("postgresql",
* cluster_identifier="aurora-cluster-demo",
* engine=aws.rds.EngineType.AURORA_POSTGRESQL,
* availability_zones=[
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* database_name="mydb",
* master_username="foo",
* master_password="must_be_eight_characters",
* backup_retention_period=5,
* preferred_backup_window="07:00-09:00")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var postgresql = new Aws.Rds.Cluster("postgresql", new()
* {
* ClusterIdentifier = "aurora-cluster-demo",
* Engine = Aws.Rds.EngineType.AuroraPostgresql,
* AvailabilityZones = new[]
* {
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* },
* DatabaseName = "mydb",
* MasterUsername = "foo",
* MasterPassword = "must_be_eight_characters",
* BackupRetentionPeriod = 5,
* PreferredBackupWindow = "07:00-09:00",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rds.NewCluster(ctx, "postgresql", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("aurora-cluster-demo"),
* Engine: pulumi.String(rds.EngineTypeAuroraPostgresql),
* AvailabilityZones: pulumi.StringArray{
* pulumi.String("us-west-2a"),
* pulumi.String("us-west-2b"),
* pulumi.String("us-west-2c"),
* },
* DatabaseName: pulumi.String("mydb"),
* MasterUsername: pulumi.String("foo"),
* MasterPassword: pulumi.String("must_be_eight_characters"),
* BackupRetentionPeriod: pulumi.Int(5),
* PreferredBackupWindow: pulumi.String("07:00-09:00"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var postgresql = new Cluster("postgresql", ClusterArgs.builder()
* .clusterIdentifier("aurora-cluster-demo")
* .engine("aurora-postgresql")
* .availabilityZones(
* "us-west-2a",
* "us-west-2b",
* "us-west-2c")
* .databaseName("mydb")
* .masterUsername("foo")
* .masterPassword("must_be_eight_characters")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* postgresql:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: aurora-cluster-demo
* engine: aurora-postgresql
* availabilityZones:
* - us-west-2a
* - us-west-2b
* - us-west-2c
* databaseName: mydb
* masterUsername: foo
* masterPassword: must_be_eight_characters
* backupRetentionPeriod: 5
* preferredBackupWindow: 07:00-09:00
* ```
*
* ### RDS Multi-AZ Cluster
* > More information about RDS Multi-AZ Clusters can be found in the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/multi-az-db-clusters-concepts.html).
* To create a Multi-AZ RDS cluster, you must additionally specify the `engine`, `storage_type`, `allocated_storage`, `iops` and `db_cluster_instance_class` attributes.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.rds.Cluster("example", {
* clusterIdentifier: "example",
* availabilityZones: [
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* engine: "mysql",
* dbClusterInstanceClass: "db.r6gd.xlarge",
* storageType: "io1",
* allocatedStorage: 100,
* iops: 1000,
* masterUsername: "test",
* masterPassword: "mustbeeightcharaters",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.rds.Cluster("example",
* cluster_identifier="example",
* availability_zones=[
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* ],
* engine="mysql",
* db_cluster_instance_class="db.r6gd.xlarge",
* storage_type="io1",
* allocated_storage=100,
* iops=1000,
* master_username="test",
* master_password="mustbeeightcharaters")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Rds.Cluster("example", new()
* {
* ClusterIdentifier = "example",
* AvailabilityZones = new[]
* {
* "us-west-2a",
* "us-west-2b",
* "us-west-2c",
* },
* Engine = "mysql",
* DbClusterInstanceClass = "db.r6gd.xlarge",
* StorageType = "io1",
* AllocatedStorage = 100,
* Iops = 1000,
* MasterUsername = "test",
* MasterPassword = "mustbeeightcharaters",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rds.NewCluster(ctx, "example", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("example"),
* AvailabilityZones: pulumi.StringArray{
* pulumi.String("us-west-2a"),
* pulumi.String("us-west-2b"),
* pulumi.String("us-west-2c"),
* },
* Engine: pulumi.String("mysql"),
* DbClusterInstanceClass: pulumi.String("db.r6gd.xlarge"),
* StorageType: pulumi.String("io1"),
* AllocatedStorage: pulumi.Int(100),
* Iops: pulumi.Int(1000),
* MasterUsername: pulumi.String("test"),
* MasterPassword: pulumi.String("mustbeeightcharaters"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Cluster("example", ClusterArgs.builder()
* .clusterIdentifier("example")
* .availabilityZones(
* "us-west-2a",
* "us-west-2b",
* "us-west-2c")
* .engine("mysql")
* .dbClusterInstanceClass("db.r6gd.xlarge")
* .storageType("io1")
* .allocatedStorage(100)
* .iops(1000)
* .masterUsername("test")
* .masterPassword("mustbeeightcharaters")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: example
* availabilityZones:
* - us-west-2a
* - us-west-2b
* - us-west-2c
* engine: mysql
* dbClusterInstanceClass: db.r6gd.xlarge
* storageType: io1
* allocatedStorage: 100
* iops: 1000
* masterUsername: test
* masterPassword: mustbeeightcharaters
* ```
*
* ### RDS Serverless v2 Cluster
* > More information about RDS Serverless v2 Clusters can be found in the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-serverless-v2.html).
* > **Note:** Unlike Serverless v1, in Serverless v2 the `storage_encrypted` value is set to `false` by default.
* This is because Serverless v1 uses the `serverless` `engine_mode`, but Serverless v2 uses the `provisioned` `engine_mode`.
* To create a Serverless v2 RDS cluster, you must additionally specify the `engine_mode` and `serverlessv2_scaling_configuration` attributes. An `aws.rds.ClusterInstance` resource must also be added to the cluster with the `instance_class` attribute specified.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.rds.Cluster("example", {
* clusterIdentifier: "example",
* engine: aws.rds.EngineType.AuroraPostgresql,
* engineMode: aws.rds.EngineMode.Provisioned,
* engineVersion: "13.6",
* databaseName: "test",
* masterUsername: "test",
* masterPassword: "must_be_eight_characters",
* storageEncrypted: true,
* serverlessv2ScalingConfiguration: {
* maxCapacity: 1,
* minCapacity: 0.5,
* },
* });
* const exampleClusterInstance = new aws.rds.ClusterInstance("example", {
* clusterIdentifier: example.id,
* instanceClass: "db.serverless",
* engine: example.engine,
* engineVersion: example.engineVersion,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.rds.Cluster("example",
* cluster_identifier="example",
* engine=aws.rds.EngineType.AURORA_POSTGRESQL,
* engine_mode=aws.rds.EngineMode.PROVISIONED,
* engine_version="13.6",
* database_name="test",
* master_username="test",
* master_password="must_be_eight_characters",
* storage_encrypted=True,
* serverlessv2_scaling_configuration={
* "max_capacity": 1,
* "min_capacity": 0.5,
* })
* example_cluster_instance = aws.rds.ClusterInstance("example",
* cluster_identifier=example.id,
* instance_class="db.serverless",
* engine=example.engine,
* engine_version=example.engine_version)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Rds.Cluster("example", new()
* {
* ClusterIdentifier = "example",
* Engine = Aws.Rds.EngineType.AuroraPostgresql,
* EngineMode = Aws.Rds.EngineMode.Provisioned,
* EngineVersion = "13.6",
* DatabaseName = "test",
* MasterUsername = "test",
* MasterPassword = "must_be_eight_characters",
* StorageEncrypted = true,
* Serverlessv2ScalingConfiguration = new Aws.Rds.Inputs.ClusterServerlessv2ScalingConfigurationArgs
* {
* MaxCapacity = 1,
* MinCapacity = 0.5,
* },
* });
* var exampleClusterInstance = new Aws.Rds.ClusterInstance("example", new()
* {
* ClusterIdentifier = example.Id,
* InstanceClass = "db.serverless",
* Engine = example.Engine,
* EngineVersion = example.EngineVersion,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := rds.NewCluster(ctx, "example", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("example"),
* Engine: pulumi.String(rds.EngineTypeAuroraPostgresql),
* EngineMode: pulumi.String(rds.EngineModeProvisioned),
* EngineVersion: pulumi.String("13.6"),
* DatabaseName: pulumi.String("test"),
* MasterUsername: pulumi.String("test"),
* MasterPassword: pulumi.String("must_be_eight_characters"),
* StorageEncrypted: pulumi.Bool(true),
* Serverlessv2ScalingConfiguration: &rds.ClusterServerlessv2ScalingConfigurationArgs{
* MaxCapacity: pulumi.Float64(1),
* MinCapacity: pulumi.Float64(0.5),
* },
* })
* if err != nil {
* return err
* }
* _, err = rds.NewClusterInstance(ctx, "example", &rds.ClusterInstanceArgs{
* ClusterIdentifier: example.ID(),
* InstanceClass: pulumi.String("db.serverless"),
* Engine: example.Engine,
* EngineVersion: example.EngineVersion,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import com.pulumi.aws.rds.inputs.ClusterServerlessv2ScalingConfigurationArgs;
* import com.pulumi.aws.rds.ClusterInstance;
* import com.pulumi.aws.rds.ClusterInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Cluster("example", ClusterArgs.builder()
* .clusterIdentifier("example")
* .engine("aurora-postgresql")
* .engineMode("provisioned")
* .engineVersion("13.6")
* .databaseName("test")
* .masterUsername("test")
* .masterPassword("must_be_eight_characters")
* .storageEncrypted(true)
* .serverlessv2ScalingConfiguration(ClusterServerlessv2ScalingConfigurationArgs.builder()
* .maxCapacity(1)
* .minCapacity(0.5)
* .build())
* .build());
* var exampleClusterInstance = new ClusterInstance("exampleClusterInstance", ClusterInstanceArgs.builder()
* .clusterIdentifier(example.id())
* .instanceClass("db.serverless")
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: example
* engine: aurora-postgresql
* engineMode: provisioned
* engineVersion: '13.6'
* databaseName: test
* masterUsername: test
* masterPassword: must_be_eight_characters
* storageEncrypted: true
* serverlessv2ScalingConfiguration:
* maxCapacity: 1
* minCapacity: 0.5
* exampleClusterInstance:
* type: aws:rds:ClusterInstance
* name: example
* properties:
* clusterIdentifier: ${example.id}
* instanceClass: db.serverless
* engine: ${example.engine}
* engineVersion: ${example.engineVersion}
* ```
*
* ### RDS/Aurora Managed Master Passwords via Secrets Manager, default KMS Key
* > More information about RDS/Aurora Aurora integrates with Secrets Manager to manage master user passwords for your DB clusters can be found in the [RDS User Guide](https://aws.amazon.com/about-aws/whats-new/2022/12/amazon-rds-integration-aws-secrets-manager/) and [Aurora User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/rds-secrets-manager.html).
* You can specify the `manage_master_user_password` attribute to enable managing the master password with Secrets Manager. You can also update an existing cluster to use Secrets Manager by specify the `manage_master_user_password` attribute and removing the `master_password` attribute (removal is required).
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.rds.Cluster("test", {
* clusterIdentifier: "example",
* databaseName: "test",
* manageMasterUserPassword: true,
* masterUsername: "test",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.rds.Cluster("test",
* cluster_identifier="example",
* database_name="test",
* manage_master_user_password=True,
* master_username="test")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Rds.Cluster("test", new()
* {
* ClusterIdentifier = "example",
* DatabaseName = "test",
* ManageMasterUserPassword = true,
* MasterUsername = "test",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rds.NewCluster(ctx, "test", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("example"),
* DatabaseName: pulumi.String("test"),
* ManageMasterUserPassword: pulumi.Bool(true),
* MasterUsername: pulumi.String("test"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new Cluster("test", ClusterArgs.builder()
* .clusterIdentifier("example")
* .databaseName("test")
* .manageMasterUserPassword(true)
* .masterUsername("test")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: example
* databaseName: test
* manageMasterUserPassword: true
* masterUsername: test
* ```
*
* ### RDS/Aurora Managed Master Passwords via Secrets Manager, specific KMS Key
* > More information about RDS/Aurora Aurora integrates with Secrets Manager to manage master user passwords for your DB clusters can be found in the [RDS User Guide](https://aws.amazon.com/about-aws/whats-new/2022/12/amazon-rds-integration-aws-secrets-manager/) and [Aurora User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/rds-secrets-manager.html).
* You can specify the `master_user_secret_kms_key_id` attribute to specify a specific KMS Key.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.kms.Key("example", {description: "Example KMS Key"});
* const test = new aws.rds.Cluster("test", {
* clusterIdentifier: "example",
* databaseName: "test",
* manageMasterUserPassword: true,
* masterUsername: "test",
* masterUserSecretKmsKeyId: example.keyId,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.kms.Key("example", description="Example KMS Key")
* test = aws.rds.Cluster("test",
* cluster_identifier="example",
* database_name="test",
* manage_master_user_password=True,
* master_username="test",
* master_user_secret_kms_key_id=example.key_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Kms.Key("example", new()
* {
* Description = "Example KMS Key",
* });
* var test = new Aws.Rds.Cluster("test", new()
* {
* ClusterIdentifier = "example",
* DatabaseName = "test",
* ManageMasterUserPassword = true,
* MasterUsername = "test",
* MasterUserSecretKmsKeyId = example.KeyId,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := kms.NewKey(ctx, "example", &kms.KeyArgs{
* Description: pulumi.String("Example KMS Key"),
* })
* if err != nil {
* return err
* }
* _, err = rds.NewCluster(ctx, "test", &rds.ClusterArgs{
* ClusterIdentifier: pulumi.String("example"),
* DatabaseName: pulumi.String("test"),
* ManageMasterUserPassword: pulumi.Bool(true),
* MasterUsername: pulumi.String("test"),
* MasterUserSecretKmsKeyId: example.KeyId,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Key("example", KeyArgs.builder()
* .description("Example KMS Key")
* .build());
* var test = new Cluster("test", ClusterArgs.builder()
* .clusterIdentifier("example")
* .databaseName("test")
* .manageMasterUserPassword(true)
* .masterUsername("test")
* .masterUserSecretKmsKeyId(example.keyId())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:kms:Key
* properties:
* description: Example KMS Key
* test:
* type: aws:rds:Cluster
* properties:
* clusterIdentifier: example
* databaseName: test
* manageMasterUserPassword: true
* masterUsername: test
* masterUserSecretKmsKeyId: ${example.keyId}
* ```
*
* ### Global Cluster Restored From Snapshot
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.rds.getClusterSnapshot({
* dbClusterIdentifier: "example-original-cluster",
* mostRecent: true,
* });
* const exampleCluster = new aws.rds.Cluster("example", {
* engine: aws.rds.EngineType.Aurora,
* engineVersion: "5.6.mysql_aurora.1.22.4",
* clusterIdentifier: "example",
* snapshotIdentifier: example.then(example => example.id),
* });
* const exampleGlobalCluster = new aws.rds.GlobalCluster("example", {
* globalClusterIdentifier: "example",
* sourceDbClusterIdentifier: exampleCluster.arn,
* forceDestroy: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.rds.get_cluster_snapshot(db_cluster_identifier="example-original-cluster",
* most_recent=True)
* example_cluster = aws.rds.Cluster("example",
* engine=aws.rds.EngineType.AURORA,
* engine_version="5.6.mysql_aurora.1.22.4",
* cluster_identifier="example",
* snapshot_identifier=example.id)
* example_global_cluster = aws.rds.GlobalCluster("example",
* global_cluster_identifier="example",
* source_db_cluster_identifier=example_cluster.arn,
* force_destroy=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Rds.GetClusterSnapshot.Invoke(new()
* {
* DbClusterIdentifier = "example-original-cluster",
* MostRecent = true,
* });
* var exampleCluster = new Aws.Rds.Cluster("example", new()
* {
* Engine = Aws.Rds.EngineType.Aurora,
* EngineVersion = "5.6.mysql_aurora.1.22.4",
* ClusterIdentifier = "example",
* SnapshotIdentifier = example.Apply(getClusterSnapshotResult => getClusterSnapshotResult.Id),
* });
* var exampleGlobalCluster = new Aws.Rds.GlobalCluster("example", new()
* {
* GlobalClusterIdentifier = "example",
* SourceDbClusterIdentifier = exampleCluster.Arn,
* ForceDestroy = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/rds"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := rds.LookupClusterSnapshot(ctx, &rds.LookupClusterSnapshotArgs{
* DbClusterIdentifier: pulumi.StringRef("example-original-cluster"),
* MostRecent: pulumi.BoolRef(true),
* }, nil)
* if err != nil {
* return err
* }
* exampleCluster, err := rds.NewCluster(ctx, "example", &rds.ClusterArgs{
* Engine: pulumi.String(rds.EngineTypeAurora),
* EngineVersion: pulumi.String("5.6.mysql_aurora.1.22.4"),
* ClusterIdentifier: pulumi.String("example"),
* SnapshotIdentifier: pulumi.String(example.Id),
* })
* if err != nil {
* return err
* }
* _, err = rds.NewGlobalCluster(ctx, "example", &rds.GlobalClusterArgs{
* GlobalClusterIdentifier: pulumi.String("example"),
* SourceDbClusterIdentifier: exampleCluster.Arn,
* ForceDestroy: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.RdsFunctions;
* import com.pulumi.aws.rds.inputs.GetClusterSnapshotArgs;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import com.pulumi.aws.rds.GlobalCluster;
* import com.pulumi.aws.rds.GlobalClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = RdsFunctions.getClusterSnapshot(GetClusterSnapshotArgs.builder()
* .dbClusterIdentifier("example-original-cluster")
* .mostRecent(true)
* .build());
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .engine("aurora")
* .engineVersion("5.6.mysql_aurora.1.22.4")
* .clusterIdentifier("example")
* .snapshotIdentifier(example.applyValue(getClusterSnapshotResult -> getClusterSnapshotResult.id()))
* .build());
* var exampleGlobalCluster = new GlobalCluster("exampleGlobalCluster", GlobalClusterArgs.builder()
* .globalClusterIdentifier("example")
* .sourceDbClusterIdentifier(exampleCluster.arn())
* .forceDestroy(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleCluster:
* type: aws:rds:Cluster
* name: example
* properties:
* engine: aurora
* engineVersion: 5.6.mysql_aurora.1.22.4
* clusterIdentifier: example
* snapshotIdentifier: ${example.id}
* exampleGlobalCluster:
* type: aws:rds:GlobalCluster
* name: example
* properties:
* globalClusterIdentifier: example
* sourceDbClusterIdentifier: ${exampleCluster.arn}
* forceDestroy: true
* variables:
* example:
* fn::invoke:
* Function: aws:rds:getClusterSnapshot
* Arguments:
* dbClusterIdentifier: example-original-cluster
* mostRecent: true
* ```
*
* ## Import
* Using `pulumi import`, import RDS Clusters using the `cluster_identifier`. For example:
* ```sh
* $ pulumi import aws:rds/cluster:Cluster aurora_cluster aurora-prod-cluster
* ```
* @property allocatedStorage The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
* @property allowMajorVersionUpgrade Enable to allow major engine version upgrades when changing engine versions. Defaults to `false`.
* @property applyImmediately Specifies whether any cluster modifications are applied immediately, or during the next maintenance window. Default is `false`. See [Amazon RDS Documentation for more information.](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Overview.DBInstance.Modifying.html)
* @property availabilityZones List of EC2 Availability Zones for the DB cluster storage where DB cluster instances can be created.
* RDS automatically assigns 3 AZs if less than 3 AZs are configured, which will show as a difference requiring resource recreation next pulumi up.
* We recommend specifying 3 AZs or using the `lifecycle` configuration block `ignore_changes` argument if necessary.
* A maximum of 3 AZs can be configured.
* @property backtrackWindow Target backtrack window, in seconds. Only available for `aurora` and `aurora-mysql` engines currently. To disable backtracking, set this value to `0`. Defaults to `0`. Must be between `0` and `259200` (72 hours)
* @property backupRetentionPeriod Days to retain backups for. Default `1`
* @property caCertificateIdentifier The CA certificate identifier to use for the DB cluster's server certificate.
* @property clusterIdentifier The cluster identifier. If omitted, this provider will assign a random, unique identifier.
* @property clusterIdentifierPrefix Creates a unique cluster identifier beginning with the specified prefix. Conflicts with `cluster_identifier`.
* @property clusterMembers List of RDS Instances that are a part of this cluster
* @property copyTagsToSnapshot Copy all Cluster `tags` to snapshots. Default is `false`.
* @property databaseName Name for an automatically created database on cluster creation. There are different naming restrictions per database engine: [RDS Naming Constraints](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Limits.html#RDS_Limits.Constraints)
* @property dbClusterInstanceClass The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example `db.m6g.xlarge`. Not all DB instance classes are available in all AWS Regions, or for all database engines. For the full list of DB instance classes and availability for your engine, see [DB instance class](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Concepts.DBInstanceClass.html) in the Amazon RDS User Guide.
* @property dbClusterParameterGroupName A cluster parameter group to associate with the cluster.
* @property dbInstanceParameterGroupName Instance parameter group to associate with all instances of the DB cluster. The `db_instance_parameter_group_name` parameter is only valid in combination with the `allow_major_version_upgrade` parameter.
* @property dbSubnetGroupName DB subnet group to associate with this DB cluster.
* **NOTE:** This must match the `db_subnet_group_name` specified on every `aws.rds.ClusterInstance` in the cluster.
* @property dbSystemId For use with RDS Custom.
* @property deleteAutomatedBackups Specifies whether to remove automated backups immediately after the DB cluster is deleted. Default is `true`.
* @property deletionProtection If the DB cluster should have deletion protection enabled.
* The database can't be deleted when this value is set to `true`.
* The default is `false`.
* @property domain The ID of the Directory Service Active Directory domain to create the cluster in.
* @property domainIamRoleName The name of the IAM role to be used when making API calls to the Directory Service.
* @property enableGlobalWriteForwarding Whether cluster should forward writes to an associated global cluster. Applied to secondary clusters to enable them to forward writes to an `aws.rds.GlobalCluster`'s primary cluster. See the [User Guide for Aurora](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-global-database-write-forwarding.html) for more information.
* @property enableHttpEndpoint Enable HTTP endpoint (data API). Only valid for some combinations of `engine_mode`, `engine` and `engine_version` and only available in some regions. See the [Region and version availability](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/data-api.html#data-api.regions) section of the documentation. This option also does not work with any of these options specified: `snapshot_identifier`, `replication_source_identifier`, `s3_import`.
* @property enableLocalWriteForwarding Whether read replicas can forward write operations to the writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB instances.. See the [User Guide for Aurora](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-mysql-write-forwarding.html) for more information. **NOTE:** Local write forwarding requires Aurora MySQL version 3.04 or higher.
* @property enabledCloudwatchLogsExports Set of log types to export to cloudwatch. If omitted, no logs will be exported. The following log types are supported: `audit`, `error`, `general`, `slowquery`, `postgresql` (PostgreSQL).
* @property engine Name of the database engine to be used for this DB cluster. Valid Values: `aurora-mysql`, `aurora-postgresql`, `mysql`, `postgres`. (Note that `mysql` and `postgres` are Multi-AZ RDS clusters).
* @property engineLifecycleSupport The life cycle type for this DB instance. This setting is valid for cluster types Aurora DB clusters and Multi-AZ DB clusters. Valid values are `open-source-rds-extended-support`, `open-source-rds-extended-support-disabled`. Default value is `open-source-rds-extended-support`. [Using Amazon RDS Extended Support]: https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/extended-support.html
* @property engineMode Database engine mode. Valid values: `global` (only valid for Aurora MySQL 1.21 and earlier), `parallelquery`, `provisioned`, `serverless`. Defaults to: `provisioned`. See the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-serverless.html) for limitations when using `serverless`.
* @property engineVersion Database engine version. Updating this argument results in an outage. See the [Aurora MySQL](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/AuroraMySQL.Updates.html) and [Aurora Postgres](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/AuroraPostgreSQL.Updates.html) documentation for your configured engine to determine this value, or by running `aws rds describe-db-engine-versions`. For example with Aurora MySQL 2, a potential value for this argument is `5.7.mysql_aurora.2.03.2`. The value can contain a partial version where supported by the API. The actual engine version used is returned in the attribute `engine_version_actual`, , see Attribute Reference below.
* @property finalSnapshotIdentifier Name of your final DB snapshot when this DB cluster is deleted. If omitted, no final snapshot will be made.
* @property globalClusterIdentifier Global cluster identifier specified on `aws.rds.GlobalCluster`.
* @property iamDatabaseAuthenticationEnabled Specifies whether or not mappings of AWS Identity and Access Management (IAM) accounts to database accounts is enabled. Please see [AWS Documentation](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/UsingWithRDS.IAMDBAuth.html) for availability and limitations.
* @property iamRoles List of ARNs for the IAM roles to associate to the RDS Cluster.
* @property iops Amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB instance in the Multi-AZ DB cluster. For information about valid Iops values, see [Amazon RDS Provisioned IOPS storage to improve performance](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Storage.html#USER_PIOPS) in the Amazon RDS User Guide. (This setting is required to create a Multi-AZ DB cluster). Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
* @property kmsKeyId ARN for the KMS encryption key. When specifying `kms_key_id`, `storage_encrypted` needs to be set to true.
* @property manageMasterUserPassword Set to true to allow RDS to manage the master user password in Secrets Manager. Cannot be set if `master_password` is provided.
* @property masterPassword Password for the master DB user. Note that this may show up in logs, and it will be stored in the state file. Please refer to the [RDS Naming Constraints](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Limits.html#RDS_Limits.Constraints). Cannot be set if `manage_master_user_password` is set to `true`.
* @property masterUserSecretKmsKeyId Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN. If not specified, the default KMS key for your Amazon Web Services account is used.
* @property masterUsername Username for the master DB user. Please refer to the [RDS Naming Constraints](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Limits.html#RDS_Limits.Constraints). This argument does not support in-place updates and cannot be changed during a restore from snapshot.
* @property networkType Network type of the cluster. Valid values: `IPV4`, `DUAL`.
* @property performanceInsightsEnabled Valid only for Non-Aurora Multi-AZ DB Clusters. Enables Performance Insights for the RDS Cluster
* @property performanceInsightsKmsKeyId Valid only for Non-Aurora Multi-AZ DB Clusters. Specifies the KMS Key ID to encrypt Performance Insights data. If not specified, the default RDS KMS key will be used (`aws/rds`).
* @property performanceInsightsRetentionPeriod Valid only for Non-Aurora Multi-AZ DB Clusters. Specifies the amount of time to retain performance insights data for. Defaults to 7 days if Performance Insights are enabled. Valid values are `7`, `month * 31` (where month is a number of months from 1-23), and `731`. See [here](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_PerfInsights.Overview.cost.html) for more information on retention periods.
* @property port Port on which the DB accepts connections.
* @property preferredBackupWindow Daily time range during which automated backups are created if automated backups are enabled using the BackupRetentionPeriod parameter.Time in UTC. Default: A 30-minute window selected at random from an 8-hour block of time per region, e.g. `04:00-09:00`.
* @property preferredMaintenanceWindow Weekly time range during which system maintenance can occur, in (UTC) e.g., `wed:04:00-wed:04:30`
* @property replicationSourceIdentifier ARN of a source DB cluster or DB instance if this DB cluster is to be created as a Read Replica. **Note:** Removing this attribute after creation will promote the read replica to a standalone cluster. If DB Cluster is part of a Global Cluster, use the `ignoreChanges` resource option to prevent Pulumi from showing differences for this argument instead of configuring this value.
* @property restoreToPointInTime Nested attribute for [point in time restore](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-pitr.html). More details below.
* @property s3Import
* @property scalingConfiguration Nested attribute with scaling properties. Only valid when `engine_mode` is set to `serverless`. More details below.
* @property serverlessv2ScalingConfiguration Nested attribute with scaling properties for ServerlessV2. Only valid when `engine_mode` is set to `provisioned`. More details below.
* @property skipFinalSnapshot Determines whether a final DB snapshot is created before the DB cluster is deleted. If true is specified, no DB snapshot is created. If false is specified, a DB snapshot is created before the DB cluster is deleted, using the value from `final_snapshot_identifier`. Default is `false`.
* @property snapshotIdentifier Specifies whether or not to create this cluster from a snapshot. You can use either the name or ARN when specifying a DB cluster snapshot, or the ARN when specifying a DB snapshot. Conflicts with `global_cluster_identifier`. Clusters cannot be restored from snapshot **and** joined to an existing global cluster in a single operation. See the [AWS documentation](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-global-database-getting-started.html#aurora-global-database.use-snapshot) or the Global Cluster Restored From Snapshot example for instructions on building a global cluster starting with a snapshot.
* @property sourceRegion The source region for an encrypted replica DB cluster.
* @property storageEncrypted Specifies whether the DB cluster is encrypted. The default is `false` for `provisioned` `engine_mode` and `true` for `serverless` `engine_mode`. When restoring an unencrypted `snapshot_identifier`, the `kms_key_id` argument must be provided to encrypt the restored cluster. The provider will only perform drift detection if a configuration value is provided.
* @property storageType (Forces new for Multi-AZ DB clusters) Specifies the storage type to be associated with the DB cluster. For Aurora DB clusters, `storage_type` modifications can be done in-place. For Multi-AZ DB Clusters, the `iops` argument must also be set. Valid values are: `""`, `aurora-iopt1` (Aurora DB Clusters); `io1`, `io2` (Multi-AZ DB Clusters). Default: `""` (Aurora DB Clusters); `io1` (Multi-AZ DB Clusters).
* @property tags A map of tags to assign to the DB cluster. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property vpcSecurityGroupIds List of VPC security groups to associate with the Cluster
*/
public data class ClusterArgs(
public val allocatedStorage: Output? = null,
public val allowMajorVersionUpgrade: Output? = null,
public val applyImmediately: Output? = null,
public val availabilityZones: Output>? = null,
public val backtrackWindow: Output? = null,
public val backupRetentionPeriod: Output? = null,
public val caCertificateIdentifier: Output? = null,
public val clusterIdentifier: Output? = null,
public val clusterIdentifierPrefix: Output? = null,
public val clusterMembers: Output>? = null,
public val copyTagsToSnapshot: Output? = null,
public val databaseName: Output? = null,
public val dbClusterInstanceClass: Output? = null,
public val dbClusterParameterGroupName: Output? = null,
public val dbInstanceParameterGroupName: Output? = null,
public val dbSubnetGroupName: Output? = null,
public val dbSystemId: Output? = null,
public val deleteAutomatedBackups: Output? = null,
public val deletionProtection: Output? = null,
public val domain: Output? = null,
public val domainIamRoleName: Output? = null,
public val enableGlobalWriteForwarding: Output? = null,
public val enableHttpEndpoint: Output? = null,
public val enableLocalWriteForwarding: Output? = null,
public val enabledCloudwatchLogsExports: Output>? = null,
public val engine: Output>? = null,
public val engineLifecycleSupport: Output? = null,
public val engineMode: Output>? = null,
public val engineVersion: Output? = null,
public val finalSnapshotIdentifier: Output? = null,
public val globalClusterIdentifier: Output? = null,
public val iamDatabaseAuthenticationEnabled: Output? = null,
public val iamRoles: Output>? = null,
public val iops: Output? = null,
public val kmsKeyId: Output? = null,
public val manageMasterUserPassword: Output? = null,
public val masterPassword: Output? = null,
public val masterUserSecretKmsKeyId: Output? = null,
public val masterUsername: Output? = null,
public val networkType: Output? = null,
public val performanceInsightsEnabled: Output? = null,
public val performanceInsightsKmsKeyId: Output? = null,
public val performanceInsightsRetentionPeriod: Output? = null,
public val port: Output? = null,
public val preferredBackupWindow: Output? = null,
public val preferredMaintenanceWindow: Output? = null,
public val replicationSourceIdentifier: Output? = null,
public val restoreToPointInTime: Output? = null,
public val s3Import: Output? = null,
public val scalingConfiguration: Output? = null,
public val serverlessv2ScalingConfiguration: Output? =
null,
public val skipFinalSnapshot: Output? = null,
public val snapshotIdentifier: Output? = null,
public val sourceRegion: Output? = null,
public val storageEncrypted: Output? = null,
public val storageType: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy