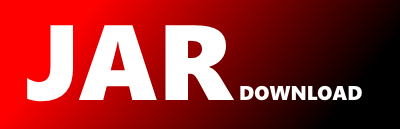
com.pulumi.aws.rds.kotlin.RoleAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.rds.kotlin
import com.pulumi.aws.rds.RoleAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages an RDS DB Instance association with an IAM Role. Example use cases:
* * [Amazon RDS Oracle integration with Amazon S3](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/oracle-s3-integration.html)
* * [Importing Amazon S3 Data into an RDS PostgreSQL DB Instance](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_PostgreSQL.S3Import.html)
* > To manage the RDS DB Instance IAM Role for [Enhanced Monitoring](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_Monitoring.OS.html), see the `aws.rds.Instance` resource `monitoring_role_arn` argument instead.
* ## Import
* Using `pulumi import`, import `aws_db_instance_role_association` using the DB Instance Identifier and IAM Role ARN separated by a comma (`,`). For example:
* ```sh
* $ pulumi import aws:rds/roleAssociation:RoleAssociation example my-db-instance,arn:aws:iam::123456789012:role/my-role
* ```
* @property dbInstanceIdentifier DB Instance Identifier to associate with the IAM Role.
* @property featureName Name of the feature for association. This can be found in the AWS documentation relevant to the integration or a full list is available in the `SupportedFeatureNames` list returned by [AWS CLI rds describe-db-engine-versions](https://docs.aws.amazon.com/cli/latest/reference/rds/describe-db-engine-versions.html).
* @property roleArn Amazon Resource Name (ARN) of the IAM Role to associate with the DB Instance.
*/
public data class RoleAssociationArgs(
public val dbInstanceIdentifier: Output? = null,
public val featureName: Output? = null,
public val roleArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.rds.RoleAssociationArgs =
com.pulumi.aws.rds.RoleAssociationArgs.builder()
.dbInstanceIdentifier(dbInstanceIdentifier?.applyValue({ args0 -> args0 }))
.featureName(featureName?.applyValue({ args0 -> args0 }))
.roleArn(roleArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RoleAssociationArgs].
*/
@PulumiTagMarker
public class RoleAssociationArgsBuilder internal constructor() {
private var dbInstanceIdentifier: Output? = null
private var featureName: Output? = null
private var roleArn: Output? = null
/**
* @param value DB Instance Identifier to associate with the IAM Role.
*/
@JvmName("skjupsttnnemgkcj")
public suspend fun dbInstanceIdentifier(`value`: Output) {
this.dbInstanceIdentifier = value
}
/**
* @param value Name of the feature for association. This can be found in the AWS documentation relevant to the integration or a full list is available in the `SupportedFeatureNames` list returned by [AWS CLI rds describe-db-engine-versions](https://docs.aws.amazon.com/cli/latest/reference/rds/describe-db-engine-versions.html).
*/
@JvmName("gpefbdrexrneqhlo")
public suspend fun featureName(`value`: Output) {
this.featureName = value
}
/**
* @param value Amazon Resource Name (ARN) of the IAM Role to associate with the DB Instance.
*/
@JvmName("lwxfpcodwnqlqgog")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value DB Instance Identifier to associate with the IAM Role.
*/
@JvmName("nrflbwtcpaywhcic")
public suspend fun dbInstanceIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbInstanceIdentifier = mapped
}
/**
* @param value Name of the feature for association. This can be found in the AWS documentation relevant to the integration or a full list is available in the `SupportedFeatureNames` list returned by [AWS CLI rds describe-db-engine-versions](https://docs.aws.amazon.com/cli/latest/reference/rds/describe-db-engine-versions.html).
*/
@JvmName("kygxnlsujvbxuemd")
public suspend fun featureName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.featureName = mapped
}
/**
* @param value Amazon Resource Name (ARN) of the IAM Role to associate with the DB Instance.
*/
@JvmName("lpesahkrjklcgupg")
public suspend fun roleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.roleArn = mapped
}
internal fun build(): RoleAssociationArgs = RoleAssociationArgs(
dbInstanceIdentifier = dbInstanceIdentifier,
featureName = featureName,
roleArn = roleArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy