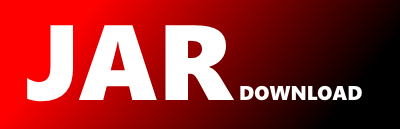
com.pulumi.aws.rds.kotlin.inputs.GetClusterSnapshotPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.rds.kotlin.inputs
import com.pulumi.aws.rds.inputs.GetClusterSnapshotPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getClusterSnapshot.
* @property dbClusterIdentifier Returns the list of snapshots created by the specific db_cluster
* @property dbClusterSnapshotIdentifier Returns information on a specific snapshot_id.
* @property includePublic Set this value to true to include manual DB Cluster Snapshots that are public and can be
* copied or restored by any AWS account, otherwise set this value to false. The default is `false`.
* @property includeShared Set this value to true to include shared manual DB Cluster Snapshots from other
* AWS accounts that this AWS account has been given permission to copy or restore, otherwise set this value to false.
* The default is `false`.
* @property mostRecent If more than one result is returned, use the most recent Snapshot.
* @property snapshotType Type of snapshots to be returned. If you don't specify a SnapshotType
* value, then both automated and manual DB cluster snapshots are returned. Shared and public DB Cluster Snapshots are not
* included in the returned results by default. Possible values are, `automated`, `manual`, `shared`, `public` and `awsbackup`.
* @property tags Mapping of tags, each pair of which must exactly match
* a pair on the desired DB cluster snapshot.
*/
public data class GetClusterSnapshotPlainArgs(
public val dbClusterIdentifier: String? = null,
public val dbClusterSnapshotIdentifier: String? = null,
public val includePublic: Boolean? = null,
public val includeShared: Boolean? = null,
public val mostRecent: Boolean? = null,
public val snapshotType: String? = null,
public val tags: Map? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.rds.inputs.GetClusterSnapshotPlainArgs =
com.pulumi.aws.rds.inputs.GetClusterSnapshotPlainArgs.builder()
.dbClusterIdentifier(dbClusterIdentifier?.let({ args0 -> args0 }))
.dbClusterSnapshotIdentifier(dbClusterSnapshotIdentifier?.let({ args0 -> args0 }))
.includePublic(includePublic?.let({ args0 -> args0 }))
.includeShared(includeShared?.let({ args0 -> args0 }))
.mostRecent(mostRecent?.let({ args0 -> args0 }))
.snapshotType(snapshotType?.let({ args0 -> args0 }))
.tags(tags?.let({ args0 -> args0.map({ args0 -> args0.key.to(args0.value) }).toMap() })).build()
}
/**
* Builder for [GetClusterSnapshotPlainArgs].
*/
@PulumiTagMarker
public class GetClusterSnapshotPlainArgsBuilder internal constructor() {
private var dbClusterIdentifier: String? = null
private var dbClusterSnapshotIdentifier: String? = null
private var includePublic: Boolean? = null
private var includeShared: Boolean? = null
private var mostRecent: Boolean? = null
private var snapshotType: String? = null
private var tags: Map? = null
/**
* @param value Returns the list of snapshots created by the specific db_cluster
*/
@JvmName("wqmyfgvvesnxftba")
public suspend fun dbClusterIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.dbClusterIdentifier = mapped
}
/**
* @param value Returns information on a specific snapshot_id.
*/
@JvmName("tomrpfgftoxcilpg")
public suspend fun dbClusterSnapshotIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.dbClusterSnapshotIdentifier = mapped
}
/**
* @param value Set this value to true to include manual DB Cluster Snapshots that are public and can be
* copied or restored by any AWS account, otherwise set this value to false. The default is `false`.
*/
@JvmName("vwlgpjghnptojryr")
public suspend fun includePublic(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.includePublic = mapped
}
/**
* @param value Set this value to true to include shared manual DB Cluster Snapshots from other
* AWS accounts that this AWS account has been given permission to copy or restore, otherwise set this value to false.
* The default is `false`.
*/
@JvmName("sfgntvfpptwftoso")
public suspend fun includeShared(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.includeShared = mapped
}
/**
* @param value If more than one result is returned, use the most recent Snapshot.
*/
@JvmName("kcscpylluhqmuqmo")
public suspend fun mostRecent(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.mostRecent = mapped
}
/**
* @param value Type of snapshots to be returned. If you don't specify a SnapshotType
* value, then both automated and manual DB cluster snapshots are returned. Shared and public DB Cluster Snapshots are not
* included in the returned results by default. Possible values are, `automated`, `manual`, `shared`, `public` and `awsbackup`.
*/
@JvmName("lrmecsahbqxqvbkd")
public suspend fun snapshotType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.snapshotType = mapped
}
/**
* @param value Mapping of tags, each pair of which must exactly match
* a pair on the desired DB cluster snapshot.
*/
@JvmName("nwdbjwuwxkryfhck")
public suspend fun tags(`value`: Map?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tags = mapped
}
/**
* @param values Mapping of tags, each pair of which must exactly match
* a pair on the desired DB cluster snapshot.
*/
@JvmName("poibcprqokpxjotx")
public fun tags(vararg values: Pair) {
val toBeMapped = values.toMap()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tags = mapped
}
internal fun build(): GetClusterSnapshotPlainArgs = GetClusterSnapshotPlainArgs(
dbClusterIdentifier = dbClusterIdentifier,
dbClusterSnapshotIdentifier = dbClusterSnapshotIdentifier,
includePublic = includePublic,
includeShared = includeShared,
mostRecent = mostRecent,
snapshotType = snapshotType,
tags = tags,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy