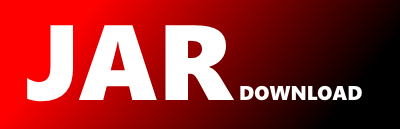
com.pulumi.aws.rds.kotlin.outputs.GetClusterResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.rds.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getCluster.
* @property arn
* @property availabilityZones
* @property backtrackWindow
* @property backupRetentionPeriod
* @property clusterIdentifier
* @property clusterMembers
* @property clusterResourceId
* @property databaseName
* @property dbClusterParameterGroupName
* @property dbSubnetGroupName
* @property dbSystemId
* @property enabledCloudwatchLogsExports
* @property endpoint
* @property engine
* @property engineMode
* @property engineVersion
* @property finalSnapshotIdentifier
* @property hostedZoneId
* @property iamDatabaseAuthenticationEnabled
* @property iamRoles
* @property id The provider-assigned unique ID for this managed resource.
* @property kmsKeyId
* @property masterUserSecrets
* @property masterUsername
* @property networkType
* @property port
* @property preferredBackupWindow
* @property preferredMaintenanceWindow
* @property readerEndpoint
* @property replicationSourceIdentifier
* @property storageEncrypted
* @property tags A map of tags assigned to the resource.
* @property vpcSecurityGroupIds
*/
public data class GetClusterResult(
public val arn: String,
public val availabilityZones: List,
public val backtrackWindow: Int,
public val backupRetentionPeriod: Int,
public val clusterIdentifier: String,
public val clusterMembers: List,
public val clusterResourceId: String,
public val databaseName: String,
public val dbClusterParameterGroupName: String,
public val dbSubnetGroupName: String,
public val dbSystemId: String,
public val enabledCloudwatchLogsExports: List,
public val endpoint: String,
public val engine: String,
public val engineMode: String,
public val engineVersion: String,
public val finalSnapshotIdentifier: String,
public val hostedZoneId: String,
public val iamDatabaseAuthenticationEnabled: Boolean,
public val iamRoles: List,
public val id: String,
public val kmsKeyId: String,
public val masterUserSecrets: List,
public val masterUsername: String,
public val networkType: String,
public val port: Int,
public val preferredBackupWindow: String,
public val preferredMaintenanceWindow: String,
public val readerEndpoint: String,
public val replicationSourceIdentifier: String,
public val storageEncrypted: Boolean,
public val tags: Map,
public val vpcSecurityGroupIds: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.rds.outputs.GetClusterResult): GetClusterResult =
GetClusterResult(
arn = javaType.arn(),
availabilityZones = javaType.availabilityZones().map({ args0 -> args0 }),
backtrackWindow = javaType.backtrackWindow(),
backupRetentionPeriod = javaType.backupRetentionPeriod(),
clusterIdentifier = javaType.clusterIdentifier(),
clusterMembers = javaType.clusterMembers().map({ args0 -> args0 }),
clusterResourceId = javaType.clusterResourceId(),
databaseName = javaType.databaseName(),
dbClusterParameterGroupName = javaType.dbClusterParameterGroupName(),
dbSubnetGroupName = javaType.dbSubnetGroupName(),
dbSystemId = javaType.dbSystemId(),
enabledCloudwatchLogsExports = javaType.enabledCloudwatchLogsExports().map({ args0 -> args0 }),
endpoint = javaType.endpoint(),
engine = javaType.engine(),
engineMode = javaType.engineMode(),
engineVersion = javaType.engineVersion(),
finalSnapshotIdentifier = javaType.finalSnapshotIdentifier(),
hostedZoneId = javaType.hostedZoneId(),
iamDatabaseAuthenticationEnabled = javaType.iamDatabaseAuthenticationEnabled(),
iamRoles = javaType.iamRoles().map({ args0 -> args0 }),
id = javaType.id(),
kmsKeyId = javaType.kmsKeyId(),
masterUserSecrets = javaType.masterUserSecrets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.rds.kotlin.outputs.GetClusterMasterUserSecret.Companion.toKotlin(args0)
})
}),
masterUsername = javaType.masterUsername(),
networkType = javaType.networkType(),
port = javaType.port(),
preferredBackupWindow = javaType.preferredBackupWindow(),
preferredMaintenanceWindow = javaType.preferredMaintenanceWindow(),
readerEndpoint = javaType.readerEndpoint(),
replicationSourceIdentifier = javaType.replicationSourceIdentifier(),
storageEncrypted = javaType.storageEncrypted(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
vpcSecurityGroupIds = javaType.vpcSecurityGroupIds().map({ args0 -> args0 }),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy