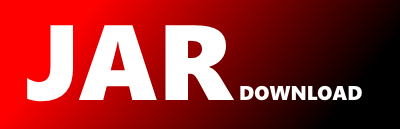
com.pulumi.aws.rds.kotlin.outputs.GetOrderableDbInstanceResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.rds.kotlin.outputs
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getOrderableDbInstance.
* @property availabilityZoneGroup
* @property availabilityZones Availability zones where the instance is available.
* @property engine
* @property engineLatestVersion
* @property engineVersion
* @property id The provider-assigned unique ID for this managed resource.
* @property instanceClass
* @property licenseModel
* @property maxIopsPerDbInstance Maximum total provisioned IOPS for a DB instance.
* @property maxIopsPerGib Maximum provisioned IOPS per GiB for a DB instance.
* @property maxStorageSize Maximum storage size for a DB instance.
* @property minIopsPerDbInstance Minimum total provisioned IOPS for a DB instance.
* @property minIopsPerGib Minimum provisioned IOPS per GiB for a DB instance.
* @property minStorageSize Minimum storage size for a DB instance.
* @property multiAzCapable Whether a DB instance is Multi-AZ capable.
* @property outpostCapable Whether a DB instance supports RDS on Outposts.
* @property preferredEngineVersions
* @property preferredInstanceClasses
* @property readReplicaCapable
* @property storageType
* @property supportedEngineModes
* @property supportedNetworkTypes
* @property supportsClusters
* @property supportsEnhancedMonitoring
* @property supportsGlobalDatabases
* @property supportsIamDatabaseAuthentication
* @property supportsIops
* @property supportsKerberosAuthentication
* @property supportsMultiAz
* @property supportsPerformanceInsights
* @property supportsStorageAutoscaling
* @property supportsStorageEncryption
* @property vpc
*/
public data class GetOrderableDbInstanceResult(
public val availabilityZoneGroup: String,
public val availabilityZones: List,
public val engine: String,
public val engineLatestVersion: Boolean? = null,
public val engineVersion: String,
public val id: String,
public val instanceClass: String,
public val licenseModel: String,
public val maxIopsPerDbInstance: Int,
public val maxIopsPerGib: Double,
public val maxStorageSize: Int,
public val minIopsPerDbInstance: Int,
public val minIopsPerGib: Double,
public val minStorageSize: Int,
public val multiAzCapable: Boolean,
public val outpostCapable: Boolean,
public val preferredEngineVersions: List? = null,
public val preferredInstanceClasses: List? = null,
public val readReplicaCapable: Boolean,
public val storageType: String,
public val supportedEngineModes: List,
public val supportedNetworkTypes: List,
public val supportsClusters: Boolean,
public val supportsEnhancedMonitoring: Boolean,
public val supportsGlobalDatabases: Boolean,
public val supportsIamDatabaseAuthentication: Boolean,
public val supportsIops: Boolean,
public val supportsKerberosAuthentication: Boolean,
public val supportsMultiAz: Boolean,
public val supportsPerformanceInsights: Boolean,
public val supportsStorageAutoscaling: Boolean,
public val supportsStorageEncryption: Boolean,
public val vpc: Boolean,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.rds.outputs.GetOrderableDbInstanceResult): GetOrderableDbInstanceResult = GetOrderableDbInstanceResult(
availabilityZoneGroup = javaType.availabilityZoneGroup(),
availabilityZones = javaType.availabilityZones().map({ args0 -> args0 }),
engine = javaType.engine(),
engineLatestVersion = javaType.engineLatestVersion().map({ args0 -> args0 }).orElse(null),
engineVersion = javaType.engineVersion(),
id = javaType.id(),
instanceClass = javaType.instanceClass(),
licenseModel = javaType.licenseModel(),
maxIopsPerDbInstance = javaType.maxIopsPerDbInstance(),
maxIopsPerGib = javaType.maxIopsPerGib(),
maxStorageSize = javaType.maxStorageSize(),
minIopsPerDbInstance = javaType.minIopsPerDbInstance(),
minIopsPerGib = javaType.minIopsPerGib(),
minStorageSize = javaType.minStorageSize(),
multiAzCapable = javaType.multiAzCapable(),
outpostCapable = javaType.outpostCapable(),
preferredEngineVersions = javaType.preferredEngineVersions().map({ args0 -> args0 }),
preferredInstanceClasses = javaType.preferredInstanceClasses().map({ args0 -> args0 }),
readReplicaCapable = javaType.readReplicaCapable(),
storageType = javaType.storageType(),
supportedEngineModes = javaType.supportedEngineModes().map({ args0 -> args0 }),
supportedNetworkTypes = javaType.supportedNetworkTypes().map({ args0 -> args0 }),
supportsClusters = javaType.supportsClusters(),
supportsEnhancedMonitoring = javaType.supportsEnhancedMonitoring(),
supportsGlobalDatabases = javaType.supportsGlobalDatabases(),
supportsIamDatabaseAuthentication = javaType.supportsIamDatabaseAuthentication(),
supportsIops = javaType.supportsIops(),
supportsKerberosAuthentication = javaType.supportsKerberosAuthentication(),
supportsMultiAz = javaType.supportsMultiAz(),
supportsPerformanceInsights = javaType.supportsPerformanceInsights(),
supportsStorageAutoscaling = javaType.supportsStorageAutoscaling(),
supportsStorageEncryption = javaType.supportsStorageEncryption(),
vpc = javaType.vpc(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy