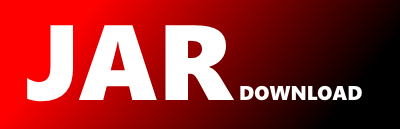
com.pulumi.aws.redshift.kotlin.DataShareConsumerAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.redshift.kotlin
import com.pulumi.aws.redshift.DataShareConsumerAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource for managing an AWS Redshift Data Share Consumer Association.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.redshift.DataShareConsumerAssociation("example", {
* dataShareArn: "arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example",
* associateEntireAccount: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.redshift.DataShareConsumerAssociation("example",
* data_share_arn="arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example",
* associate_entire_account=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.RedShift.DataShareConsumerAssociation("example", new()
* {
* DataShareArn = "arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example",
* AssociateEntireAccount = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/redshift"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := redshift.NewDataShareConsumerAssociation(ctx, "example", &redshift.DataShareConsumerAssociationArgs{
* DataShareArn: pulumi.String("arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example"),
* AssociateEntireAccount: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.redshift.DataShareConsumerAssociation;
* import com.pulumi.aws.redshift.DataShareConsumerAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new DataShareConsumerAssociation("example", DataShareConsumerAssociationArgs.builder()
* .dataShareArn("arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example")
* .associateEntireAccount(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:redshift:DataShareConsumerAssociation
* properties:
* dataShareArn: arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example
* associateEntireAccount: true
* ```
*
* ### Consumer Region
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.redshift.DataShareConsumerAssociation("example", {
* dataShareArn: "arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example",
* consumerRegion: "us-west-2",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.redshift.DataShareConsumerAssociation("example",
* data_share_arn="arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example",
* consumer_region="us-west-2")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.RedShift.DataShareConsumerAssociation("example", new()
* {
* DataShareArn = "arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example",
* ConsumerRegion = "us-west-2",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/redshift"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := redshift.NewDataShareConsumerAssociation(ctx, "example", &redshift.DataShareConsumerAssociationArgs{
* DataShareArn: pulumi.String("arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example"),
* ConsumerRegion: pulumi.String("us-west-2"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.redshift.DataShareConsumerAssociation;
* import com.pulumi.aws.redshift.DataShareConsumerAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new DataShareConsumerAssociation("example", DataShareConsumerAssociationArgs.builder()
* .dataShareArn("arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example")
* .consumerRegion("us-west-2")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:redshift:DataShareConsumerAssociation
* properties:
* dataShareArn: arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example
* consumerRegion: us-west-2
* ```
*
* ## Import
* Using `pulumi import`, import Redshift Data Share Consumer Association using the `id`. For example:
* ```sh
* $ pulumi import aws:redshift/dataShareConsumerAssociation:DataShareConsumerAssociation example arn:aws:redshift:us-west-2:123456789012:datashare:b3bfde75-73fd-408b-9086-d6fccfd6d588/example,,,us-west-2
* ```
* @property allowWrites Whether to allow write operations for a datashare.
* @property associateEntireAccount Whether the datashare is associated with the entire account. Conflicts with `consumer_arn` and `consumer_region`.
* @property consumerArn Amazon Resource Name (ARN) of the consumer that is associated with the datashare. Conflicts with `associate_entire_account` and `consumer_region`.
* @property consumerRegion From a datashare consumer account, associates a datashare with all existing and future namespaces in the specified AWS Region. Conflicts with `associate_entire_account` and `consumer_arn`.
* @property dataShareArn Amazon Resource Name (ARN) of the datashare that the consumer is to use with the account or the namespace.
* The following arguments are optional:
*/
public data class DataShareConsumerAssociationArgs(
public val allowWrites: Output? = null,
public val associateEntireAccount: Output? = null,
public val consumerArn: Output? = null,
public val consumerRegion: Output? = null,
public val dataShareArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.redshift.DataShareConsumerAssociationArgs =
com.pulumi.aws.redshift.DataShareConsumerAssociationArgs.builder()
.allowWrites(allowWrites?.applyValue({ args0 -> args0 }))
.associateEntireAccount(associateEntireAccount?.applyValue({ args0 -> args0 }))
.consumerArn(consumerArn?.applyValue({ args0 -> args0 }))
.consumerRegion(consumerRegion?.applyValue({ args0 -> args0 }))
.dataShareArn(dataShareArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DataShareConsumerAssociationArgs].
*/
@PulumiTagMarker
public class DataShareConsumerAssociationArgsBuilder internal constructor() {
private var allowWrites: Output? = null
private var associateEntireAccount: Output? = null
private var consumerArn: Output? = null
private var consumerRegion: Output? = null
private var dataShareArn: Output? = null
/**
* @param value Whether to allow write operations for a datashare.
*/
@JvmName("ntqsxoisjiydltln")
public suspend fun allowWrites(`value`: Output) {
this.allowWrites = value
}
/**
* @param value Whether the datashare is associated with the entire account. Conflicts with `consumer_arn` and `consumer_region`.
*/
@JvmName("rcflbepcculcuqlr")
public suspend fun associateEntireAccount(`value`: Output) {
this.associateEntireAccount = value
}
/**
* @param value Amazon Resource Name (ARN) of the consumer that is associated with the datashare. Conflicts with `associate_entire_account` and `consumer_region`.
*/
@JvmName("vpwtjnpqaqtpeldv")
public suspend fun consumerArn(`value`: Output) {
this.consumerArn = value
}
/**
* @param value From a datashare consumer account, associates a datashare with all existing and future namespaces in the specified AWS Region. Conflicts with `associate_entire_account` and `consumer_arn`.
*/
@JvmName("hllvwabkuyoeamqs")
public suspend fun consumerRegion(`value`: Output) {
this.consumerRegion = value
}
/**
* @param value Amazon Resource Name (ARN) of the datashare that the consumer is to use with the account or the namespace.
* The following arguments are optional:
*/
@JvmName("lfnwchoopbadxyhc")
public suspend fun dataShareArn(`value`: Output) {
this.dataShareArn = value
}
/**
* @param value Whether to allow write operations for a datashare.
*/
@JvmName("mlwlximeoerreynu")
public suspend fun allowWrites(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowWrites = mapped
}
/**
* @param value Whether the datashare is associated with the entire account. Conflicts with `consumer_arn` and `consumer_region`.
*/
@JvmName("vbwesojxplhncfuo")
public suspend fun associateEntireAccount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.associateEntireAccount = mapped
}
/**
* @param value Amazon Resource Name (ARN) of the consumer that is associated with the datashare. Conflicts with `associate_entire_account` and `consumer_region`.
*/
@JvmName("niwuaqucjuojycsg")
public suspend fun consumerArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consumerArn = mapped
}
/**
* @param value From a datashare consumer account, associates a datashare with all existing and future namespaces in the specified AWS Region. Conflicts with `associate_entire_account` and `consumer_arn`.
*/
@JvmName("lljalinpgtepfrfm")
public suspend fun consumerRegion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consumerRegion = mapped
}
/**
* @param value Amazon Resource Name (ARN) of the datashare that the consumer is to use with the account or the namespace.
* The following arguments are optional:
*/
@JvmName("jfgnfwivubqseuof")
public suspend fun dataShareArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataShareArn = mapped
}
internal fun build(): DataShareConsumerAssociationArgs = DataShareConsumerAssociationArgs(
allowWrites = allowWrites,
associateEntireAccount = associateEntireAccount,
consumerArn = consumerArn,
consumerRegion = consumerRegion,
dataShareArn = dataShareArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy