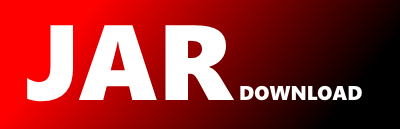
com.pulumi.aws.redshift.kotlin.ScheduledActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.redshift.kotlin
import com.pulumi.aws.redshift.ScheduledActionArgs.builder
import com.pulumi.aws.redshift.kotlin.inputs.ScheduledActionTargetActionArgs
import com.pulumi.aws.redshift.kotlin.inputs.ScheduledActionTargetActionArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ### Pause Cluster Action
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const assumeRole = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["scheduler.redshift.amazonaws.com"],
* }],
* actions: ["sts:AssumeRole"],
* }],
* });
* const exampleRole = new aws.iam.Role("example", {
* name: "redshift_scheduled_action",
* assumeRolePolicy: assumeRole.then(assumeRole => assumeRole.json),
* });
* const example = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* actions: [
* "redshift:PauseCluster",
* "redshift:ResumeCluster",
* "redshift:ResizeCluster",
* ],
* resources: ["*"],
* }],
* });
* const examplePolicy = new aws.iam.Policy("example", {
* name: "redshift_scheduled_action",
* policy: example.then(example => example.json),
* });
* const exampleRolePolicyAttachment = new aws.iam.RolePolicyAttachment("example", {
* policyArn: examplePolicy.arn,
* role: exampleRole.name,
* });
* const exampleScheduledAction = new aws.redshift.ScheduledAction("example", {
* name: "tf-redshift-scheduled-action",
* schedule: "cron(00 23 * * ? *)",
* iamRole: exampleRole.arn,
* targetAction: {
* pauseCluster: {
* clusterIdentifier: "tf-redshift001",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* assume_role = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["scheduler.redshift.amazonaws.com"],
* }],
* "actions": ["sts:AssumeRole"],
* }])
* example_role = aws.iam.Role("example",
* name="redshift_scheduled_action",
* assume_role_policy=assume_role.json)
* example = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "actions": [
* "redshift:PauseCluster",
* "redshift:ResumeCluster",
* "redshift:ResizeCluster",
* ],
* "resources": ["*"],
* }])
* example_policy = aws.iam.Policy("example",
* name="redshift_scheduled_action",
* policy=example.json)
* example_role_policy_attachment = aws.iam.RolePolicyAttachment("example",
* policy_arn=example_policy.arn,
* role=example_role.name)
* example_scheduled_action = aws.redshift.ScheduledAction("example",
* name="tf-redshift-scheduled-action",
* schedule="cron(00 23 * * ? *)",
* iam_role=example_role.arn,
* target_action={
* "pause_cluster": {
* "cluster_identifier": "tf-redshift001",
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var assumeRole = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "scheduler.redshift.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* },
* },
* });
* var exampleRole = new Aws.Iam.Role("example", new()
* {
* Name = "redshift_scheduled_action",
* AssumeRolePolicy = assumeRole.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Actions = new[]
* {
* "redshift:PauseCluster",
* "redshift:ResumeCluster",
* "redshift:ResizeCluster",
* },
* Resources = new[]
* {
* "*",
* },
* },
* },
* });
* var examplePolicy = new Aws.Iam.Policy("example", new()
* {
* Name = "redshift_scheduled_action",
* PolicyDocument = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var exampleRolePolicyAttachment = new Aws.Iam.RolePolicyAttachment("example", new()
* {
* PolicyArn = examplePolicy.Arn,
* Role = exampleRole.Name,
* });
* var exampleScheduledAction = new Aws.RedShift.ScheduledAction("example", new()
* {
* Name = "tf-redshift-scheduled-action",
* Schedule = "cron(00 23 * * ? *)",
* IamRole = exampleRole.Arn,
* TargetAction = new Aws.RedShift.Inputs.ScheduledActionTargetActionArgs
* {
* PauseCluster = new Aws.RedShift.Inputs.ScheduledActionTargetActionPauseClusterArgs
* {
* ClusterIdentifier = "tf-redshift001",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/redshift"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* assumeRole, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "scheduler.redshift.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "sts:AssumeRole",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* exampleRole, err := iam.NewRole(ctx, "example", &iam.RoleArgs{
* Name: pulumi.String("redshift_scheduled_action"),
* AssumeRolePolicy: pulumi.String(assumeRole.Json),
* })
* if err != nil {
* return err
* }
* example, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Actions: []string{
* "redshift:PauseCluster",
* "redshift:ResumeCluster",
* "redshift:ResizeCluster",
* },
* Resources: []string{
* "*",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* examplePolicy, err := iam.NewPolicy(ctx, "example", &iam.PolicyArgs{
* Name: pulumi.String("redshift_scheduled_action"),
* Policy: pulumi.String(example.Json),
* })
* if err != nil {
* return err
* }
* _, err = iam.NewRolePolicyAttachment(ctx, "example", &iam.RolePolicyAttachmentArgs{
* PolicyArn: examplePolicy.Arn,
* Role: exampleRole.Name,
* })
* if err != nil {
* return err
* }
* _, err = redshift.NewScheduledAction(ctx, "example", &redshift.ScheduledActionArgs{
* Name: pulumi.String("tf-redshift-scheduled-action"),
* Schedule: pulumi.String("cron(00 23 * * ? *)"),
* IamRole: exampleRole.Arn,
* TargetAction: &redshift.ScheduledActionTargetActionArgs{
* PauseCluster: &redshift.ScheduledActionTargetActionPauseClusterArgs{
* ClusterIdentifier: pulumi.String("tf-redshift001"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.Policy;
* import com.pulumi.aws.iam.PolicyArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.redshift.ScheduledAction;
* import com.pulumi.aws.redshift.ScheduledActionArgs;
* import com.pulumi.aws.redshift.inputs.ScheduledActionTargetActionArgs;
* import com.pulumi.aws.redshift.inputs.ScheduledActionTargetActionPauseClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("scheduler.redshift.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .name("redshift_scheduled_action")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "redshift:PauseCluster",
* "redshift:ResumeCluster",
* "redshift:ResizeCluster")
* .resources("*")
* .build())
* .build());
* var examplePolicy = new Policy("examplePolicy", PolicyArgs.builder()
* .name("redshift_scheduled_action")
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var exampleRolePolicyAttachment = new RolePolicyAttachment("exampleRolePolicyAttachment", RolePolicyAttachmentArgs.builder()
* .policyArn(examplePolicy.arn())
* .role(exampleRole.name())
* .build());
* var exampleScheduledAction = new ScheduledAction("exampleScheduledAction", ScheduledActionArgs.builder()
* .name("tf-redshift-scheduled-action")
* .schedule("cron(00 23 * * ? *)")
* .iamRole(exampleRole.arn())
* .targetAction(ScheduledActionTargetActionArgs.builder()
* .pauseCluster(ScheduledActionTargetActionPauseClusterArgs.builder()
* .clusterIdentifier("tf-redshift001")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleRole:
* type: aws:iam:Role
* name: example
* properties:
* name: redshift_scheduled_action
* assumeRolePolicy: ${assumeRole.json}
* examplePolicy:
* type: aws:iam:Policy
* name: example
* properties:
* name: redshift_scheduled_action
* policy: ${example.json}
* exampleRolePolicyAttachment:
* type: aws:iam:RolePolicyAttachment
* name: example
* properties:
* policyArn: ${examplePolicy.arn}
* role: ${exampleRole.name}
* exampleScheduledAction:
* type: aws:redshift:ScheduledAction
* name: example
* properties:
* name: tf-redshift-scheduled-action
* schedule: cron(00 23 * * ? *)
* iamRole: ${exampleRole.arn}
* targetAction:
* pauseCluster:
* clusterIdentifier: tf-redshift001
* variables:
* assumeRole:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: Service
* identifiers:
* - scheduler.redshift.amazonaws.com
* actions:
* - sts:AssumeRole
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* actions:
* - redshift:PauseCluster
* - redshift:ResumeCluster
* - redshift:ResizeCluster
* resources:
* - '*'
* ```
*
* ### Resize Cluster Action
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.redshift.ScheduledAction("example", {
* name: "tf-redshift-scheduled-action",
* schedule: "cron(00 23 * * ? *)",
* iamRole: exampleAwsIamRole.arn,
* targetAction: {
* resizeCluster: {
* clusterIdentifier: "tf-redshift001",
* clusterType: "multi-node",
* nodeType: "dc1.large",
* numberOfNodes: 2,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.redshift.ScheduledAction("example",
* name="tf-redshift-scheduled-action",
* schedule="cron(00 23 * * ? *)",
* iam_role=example_aws_iam_role["arn"],
* target_action={
* "resize_cluster": {
* "cluster_identifier": "tf-redshift001",
* "cluster_type": "multi-node",
* "node_type": "dc1.large",
* "number_of_nodes": 2,
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.RedShift.ScheduledAction("example", new()
* {
* Name = "tf-redshift-scheduled-action",
* Schedule = "cron(00 23 * * ? *)",
* IamRole = exampleAwsIamRole.Arn,
* TargetAction = new Aws.RedShift.Inputs.ScheduledActionTargetActionArgs
* {
* ResizeCluster = new Aws.RedShift.Inputs.ScheduledActionTargetActionResizeClusterArgs
* {
* ClusterIdentifier = "tf-redshift001",
* ClusterType = "multi-node",
* NodeType = "dc1.large",
* NumberOfNodes = 2,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/redshift"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := redshift.NewScheduledAction(ctx, "example", &redshift.ScheduledActionArgs{
* Name: pulumi.String("tf-redshift-scheduled-action"),
* Schedule: pulumi.String("cron(00 23 * * ? *)"),
* IamRole: pulumi.Any(exampleAwsIamRole.Arn),
* TargetAction: &redshift.ScheduledActionTargetActionArgs{
* ResizeCluster: &redshift.ScheduledActionTargetActionResizeClusterArgs{
* ClusterIdentifier: pulumi.String("tf-redshift001"),
* ClusterType: pulumi.String("multi-node"),
* NodeType: pulumi.String("dc1.large"),
* NumberOfNodes: pulumi.Int(2),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.redshift.ScheduledAction;
* import com.pulumi.aws.redshift.ScheduledActionArgs;
* import com.pulumi.aws.redshift.inputs.ScheduledActionTargetActionArgs;
* import com.pulumi.aws.redshift.inputs.ScheduledActionTargetActionResizeClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ScheduledAction("example", ScheduledActionArgs.builder()
* .name("tf-redshift-scheduled-action")
* .schedule("cron(00 23 * * ? *)")
* .iamRole(exampleAwsIamRole.arn())
* .targetAction(ScheduledActionTargetActionArgs.builder()
* .resizeCluster(ScheduledActionTargetActionResizeClusterArgs.builder()
* .clusterIdentifier("tf-redshift001")
* .clusterType("multi-node")
* .nodeType("dc1.large")
* .numberOfNodes(2)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:redshift:ScheduledAction
* properties:
* name: tf-redshift-scheduled-action
* schedule: cron(00 23 * * ? *)
* iamRole: ${exampleAwsIamRole.arn}
* targetAction:
* resizeCluster:
* clusterIdentifier: tf-redshift001
* clusterType: multi-node
* nodeType: dc1.large
* numberOfNodes: 2
* ```
*
* ## Import
* Using `pulumi import`, import Redshift Scheduled Action using the `name`. For example:
* ```sh
* $ pulumi import aws:redshift/scheduledAction:ScheduledAction example tf-redshift-scheduled-action
* ```
* @property description The description of the scheduled action.
* @property enable Whether to enable the scheduled action. Default is `true` .
* @property endTime The end time in UTC when the schedule is active, in UTC RFC3339 format(for example, YYYY-MM-DDTHH:MM:SSZ).
* @property iamRole The IAM role to assume to run the scheduled action.
* @property name The scheduled action name.
* @property schedule The schedule of action. The schedule is defined format of "at expression" or "cron expression", for example `at(2016-03-04T17:27:00)` or `cron(0 10 ? * MON *)`. See [Scheduled Action](https://docs.aws.amazon.com/redshift/latest/APIReference/API_ScheduledAction.html) for more information.
* @property startTime The start time in UTC when the schedule is active, in UTC RFC3339 format(for example, YYYY-MM-DDTHH:MM:SSZ).
* @property targetAction Target action. Documented below.
*/
public data class ScheduledActionArgs(
public val description: Output? = null,
public val enable: Output? = null,
public val endTime: Output? = null,
public val iamRole: Output? = null,
public val name: Output? = null,
public val schedule: Output? = null,
public val startTime: Output? = null,
public val targetAction: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.redshift.ScheduledActionArgs =
com.pulumi.aws.redshift.ScheduledActionArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.enable(enable?.applyValue({ args0 -> args0 }))
.endTime(endTime?.applyValue({ args0 -> args0 }))
.iamRole(iamRole?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.schedule(schedule?.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 }))
.targetAction(targetAction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ScheduledActionArgs].
*/
@PulumiTagMarker
public class ScheduledActionArgsBuilder internal constructor() {
private var description: Output? = null
private var enable: Output? = null
private var endTime: Output? = null
private var iamRole: Output? = null
private var name: Output? = null
private var schedule: Output? = null
private var startTime: Output? = null
private var targetAction: Output? = null
/**
* @param value The description of the scheduled action.
*/
@JvmName("mpjgldanqfpaiouk")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Whether to enable the scheduled action. Default is `true` .
*/
@JvmName("kjiombwbcomwgsha")
public suspend fun enable(`value`: Output) {
this.enable = value
}
/**
* @param value The end time in UTC when the schedule is active, in UTC RFC3339 format(for example, YYYY-MM-DDTHH:MM:SSZ).
*/
@JvmName("tnbpgoedukegnctp")
public suspend fun endTime(`value`: Output) {
this.endTime = value
}
/**
* @param value The IAM role to assume to run the scheduled action.
*/
@JvmName("mnllkypjcduxkmnd")
public suspend fun iamRole(`value`: Output) {
this.iamRole = value
}
/**
* @param value The scheduled action name.
*/
@JvmName("xowugkkqlsurmxnh")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The schedule of action. The schedule is defined format of "at expression" or "cron expression", for example `at(2016-03-04T17:27:00)` or `cron(0 10 ? * MON *)`. See [Scheduled Action](https://docs.aws.amazon.com/redshift/latest/APIReference/API_ScheduledAction.html) for more information.
*/
@JvmName("qmhypjwltitslisf")
public suspend fun schedule(`value`: Output) {
this.schedule = value
}
/**
* @param value The start time in UTC when the schedule is active, in UTC RFC3339 format(for example, YYYY-MM-DDTHH:MM:SSZ).
*/
@JvmName("tmrabjnfdbedgdnv")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value Target action. Documented below.
*/
@JvmName("xubqelruqvpshkoo")
public suspend fun targetAction(`value`: Output) {
this.targetAction = value
}
/**
* @param value The description of the scheduled action.
*/
@JvmName("ijumgksckxwcmnsq")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Whether to enable the scheduled action. Default is `true` .
*/
@JvmName("rsmjxfsgwvhspjjf")
public suspend fun enable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enable = mapped
}
/**
* @param value The end time in UTC when the schedule is active, in UTC RFC3339 format(for example, YYYY-MM-DDTHH:MM:SSZ).
*/
@JvmName("rqnbsktlfuehqvyf")
public suspend fun endTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTime = mapped
}
/**
* @param value The IAM role to assume to run the scheduled action.
*/
@JvmName("ygeegdasahsbbmxd")
public suspend fun iamRole(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iamRole = mapped
}
/**
* @param value The scheduled action name.
*/
@JvmName("ixlpidsghbwlrndb")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The schedule of action. The schedule is defined format of "at expression" or "cron expression", for example `at(2016-03-04T17:27:00)` or `cron(0 10 ? * MON *)`. See [Scheduled Action](https://docs.aws.amazon.com/redshift/latest/APIReference/API_ScheduledAction.html) for more information.
*/
@JvmName("tgwcrsxhifxsmyca")
public suspend fun schedule(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schedule = mapped
}
/**
* @param value The start time in UTC when the schedule is active, in UTC RFC3339 format(for example, YYYY-MM-DDTHH:MM:SSZ).
*/
@JvmName("nbxjkepugqmsstdx")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param value Target action. Documented below.
*/
@JvmName("xhpodptooygovubu")
public suspend fun targetAction(`value`: ScheduledActionTargetActionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetAction = mapped
}
/**
* @param argument Target action. Documented below.
*/
@JvmName("uavxdjaoerwdlmig")
public suspend fun targetAction(argument: suspend ScheduledActionTargetActionArgsBuilder.() -> Unit) {
val toBeMapped = ScheduledActionTargetActionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.targetAction = mapped
}
internal fun build(): ScheduledActionArgs = ScheduledActionArgs(
description = description,
enable = enable,
endTime = endTime,
iamRole = iamRole,
name = name,
schedule = schedule,
startTime = startTime,
targetAction = targetAction,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy