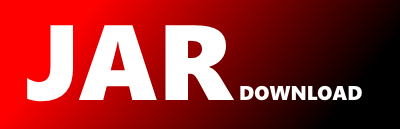
com.pulumi.aws.redshift.kotlin.SnapshotScheduleAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.redshift.kotlin
import com.pulumi.aws.redshift.SnapshotScheduleAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _default = new aws.redshift.Cluster("default", {
* clusterIdentifier: "tf-redshift-cluster",
* databaseName: "mydb",
* masterUsername: "foo",
* masterPassword: "Mustbe8characters",
* nodeType: "dc1.large",
* clusterType: "single-node",
* });
* const defaultSnapshotSchedule = new aws.redshift.SnapshotSchedule("default", {
* identifier: "tf-redshift-snapshot-schedule",
* definitions: ["rate(12 hours)"],
* });
* const defaultSnapshotScheduleAssociation = new aws.redshift.SnapshotScheduleAssociation("default", {
* clusterIdentifier: _default.id,
* scheduleIdentifier: defaultSnapshotSchedule.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default = aws.redshift.Cluster("default",
* cluster_identifier="tf-redshift-cluster",
* database_name="mydb",
* master_username="foo",
* master_password="Mustbe8characters",
* node_type="dc1.large",
* cluster_type="single-node")
* default_snapshot_schedule = aws.redshift.SnapshotSchedule("default",
* identifier="tf-redshift-snapshot-schedule",
* definitions=["rate(12 hours)"])
* default_snapshot_schedule_association = aws.redshift.SnapshotScheduleAssociation("default",
* cluster_identifier=default.id,
* schedule_identifier=default_snapshot_schedule.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Aws.RedShift.Cluster("default", new()
* {
* ClusterIdentifier = "tf-redshift-cluster",
* DatabaseName = "mydb",
* MasterUsername = "foo",
* MasterPassword = "Mustbe8characters",
* NodeType = "dc1.large",
* ClusterType = "single-node",
* });
* var defaultSnapshotSchedule = new Aws.RedShift.SnapshotSchedule("default", new()
* {
* Identifier = "tf-redshift-snapshot-schedule",
* Definitions = new[]
* {
* "rate(12 hours)",
* },
* });
* var defaultSnapshotScheduleAssociation = new Aws.RedShift.SnapshotScheduleAssociation("default", new()
* {
* ClusterIdentifier = @default.Id,
* ScheduleIdentifier = defaultSnapshotSchedule.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/redshift"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := redshift.NewCluster(ctx, "default", &redshift.ClusterArgs{
* ClusterIdentifier: pulumi.String("tf-redshift-cluster"),
* DatabaseName: pulumi.String("mydb"),
* MasterUsername: pulumi.String("foo"),
* MasterPassword: pulumi.String("Mustbe8characters"),
* NodeType: pulumi.String("dc1.large"),
* ClusterType: pulumi.String("single-node"),
* })
* if err != nil {
* return err
* }
* defaultSnapshotSchedule, err := redshift.NewSnapshotSchedule(ctx, "default", &redshift.SnapshotScheduleArgs{
* Identifier: pulumi.String("tf-redshift-snapshot-schedule"),
* Definitions: pulumi.StringArray{
* pulumi.String("rate(12 hours)"),
* },
* })
* if err != nil {
* return err
* }
* _, err = redshift.NewSnapshotScheduleAssociation(ctx, "default", &redshift.SnapshotScheduleAssociationArgs{
* ClusterIdentifier: _default.ID(),
* ScheduleIdentifier: defaultSnapshotSchedule.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.redshift.Cluster;
* import com.pulumi.aws.redshift.ClusterArgs;
* import com.pulumi.aws.redshift.SnapshotSchedule;
* import com.pulumi.aws.redshift.SnapshotScheduleArgs;
* import com.pulumi.aws.redshift.SnapshotScheduleAssociation;
* import com.pulumi.aws.redshift.SnapshotScheduleAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("tf-redshift-cluster")
* .databaseName("mydb")
* .masterUsername("foo")
* .masterPassword("Mustbe8characters")
* .nodeType("dc1.large")
* .clusterType("single-node")
* .build());
* var defaultSnapshotSchedule = new SnapshotSchedule("defaultSnapshotSchedule", SnapshotScheduleArgs.builder()
* .identifier("tf-redshift-snapshot-schedule")
* .definitions("rate(12 hours)")
* .build());
* var defaultSnapshotScheduleAssociation = new SnapshotScheduleAssociation("defaultSnapshotScheduleAssociation", SnapshotScheduleAssociationArgs.builder()
* .clusterIdentifier(default_.id())
* .scheduleIdentifier(defaultSnapshotSchedule.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: aws:redshift:Cluster
* properties:
* clusterIdentifier: tf-redshift-cluster
* databaseName: mydb
* masterUsername: foo
* masterPassword: Mustbe8characters
* nodeType: dc1.large
* clusterType: single-node
* defaultSnapshotSchedule:
* type: aws:redshift:SnapshotSchedule
* name: default
* properties:
* identifier: tf-redshift-snapshot-schedule
* definitions:
* - rate(12 hours)
* defaultSnapshotScheduleAssociation:
* type: aws:redshift:SnapshotScheduleAssociation
* name: default
* properties:
* clusterIdentifier: ${default.id}
* scheduleIdentifier: ${defaultSnapshotSchedule.id}
* ```
*
* ## Import
* Using `pulumi import`, import Redshift Snapshot Schedule Association using the `/`. For example:
* ```sh
* $ pulumi import aws:redshift/snapshotScheduleAssociation:SnapshotScheduleAssociation default tf-redshift-cluster/tf-redshift-snapshot-schedule
* ```
* @property clusterIdentifier The cluster identifier.
* @property scheduleIdentifier The snapshot schedule identifier.
*/
public data class SnapshotScheduleAssociationArgs(
public val clusterIdentifier: Output? = null,
public val scheduleIdentifier: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.redshift.SnapshotScheduleAssociationArgs =
com.pulumi.aws.redshift.SnapshotScheduleAssociationArgs.builder()
.clusterIdentifier(clusterIdentifier?.applyValue({ args0 -> args0 }))
.scheduleIdentifier(scheduleIdentifier?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SnapshotScheduleAssociationArgs].
*/
@PulumiTagMarker
public class SnapshotScheduleAssociationArgsBuilder internal constructor() {
private var clusterIdentifier: Output? = null
private var scheduleIdentifier: Output? = null
/**
* @param value The cluster identifier.
*/
@JvmName("brchorrikwxwtugm")
public suspend fun clusterIdentifier(`value`: Output) {
this.clusterIdentifier = value
}
/**
* @param value The snapshot schedule identifier.
*/
@JvmName("bdnbesxsnrihirti")
public suspend fun scheduleIdentifier(`value`: Output) {
this.scheduleIdentifier = value
}
/**
* @param value The cluster identifier.
*/
@JvmName("yeprhlcadhqdqxxi")
public suspend fun clusterIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterIdentifier = mapped
}
/**
* @param value The snapshot schedule identifier.
*/
@JvmName("whhtcjalggufyxjy")
public suspend fun scheduleIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduleIdentifier = mapped
}
internal fun build(): SnapshotScheduleAssociationArgs = SnapshotScheduleAssociationArgs(
clusterIdentifier = clusterIdentifier,
scheduleIdentifier = scheduleIdentifier,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy