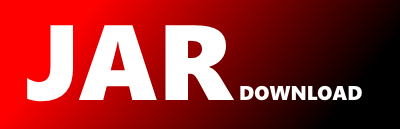
com.pulumi.aws.resiliencehub.kotlin.inputs.ResiliencyPolicyPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.resiliencehub.kotlin.inputs
import com.pulumi.aws.resiliencehub.inputs.ResiliencyPolicyPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property az Specifies Availability Zone failure policy. See `policy.az`
* @property hardware Specifies Infrastructure failure policy. See `policy.hardware`
* @property region Specifies Region failure policy. `policy.region`
* @property software Specifies Application failure policy. See `policy.software`
* The following arguments are optional:
*/
public data class ResiliencyPolicyPolicyArgs(
public val az: Output? = null,
public val hardware: Output? = null,
public val region: Output? = null,
public val software: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.resiliencehub.inputs.ResiliencyPolicyPolicyArgs =
com.pulumi.aws.resiliencehub.inputs.ResiliencyPolicyPolicyArgs.builder()
.az(az?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.hardware(hardware?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.region(region?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.software(software?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ResiliencyPolicyPolicyArgs].
*/
@PulumiTagMarker
public class ResiliencyPolicyPolicyArgsBuilder internal constructor() {
private var az: Output? = null
private var hardware: Output? = null
private var region: Output? = null
private var software: Output? = null
/**
* @param value Specifies Availability Zone failure policy. See `policy.az`
*/
@JvmName("wjvbfdjdkuyfiijl")
public suspend fun az(`value`: Output) {
this.az = value
}
/**
* @param value Specifies Infrastructure failure policy. See `policy.hardware`
*/
@JvmName("snxndbxunruwvirq")
public suspend fun hardware(`value`: Output) {
this.hardware = value
}
/**
* @param value Specifies Region failure policy. `policy.region`
*/
@JvmName("khosbghononshwyk")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value Specifies Application failure policy. See `policy.software`
* The following arguments are optional:
*/
@JvmName("nhfybrjaknnqvdmk")
public suspend fun software(`value`: Output) {
this.software = value
}
/**
* @param value Specifies Availability Zone failure policy. See `policy.az`
*/
@JvmName("moycvnchgjgycwlg")
public suspend fun az(`value`: ResiliencyPolicyPolicyAzArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.az = mapped
}
/**
* @param argument Specifies Availability Zone failure policy. See `policy.az`
*/
@JvmName("qsecsmixuaycsylj")
public suspend fun az(argument: suspend ResiliencyPolicyPolicyAzArgsBuilder.() -> Unit) {
val toBeMapped = ResiliencyPolicyPolicyAzArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.az = mapped
}
/**
* @param value Specifies Infrastructure failure policy. See `policy.hardware`
*/
@JvmName("yqssubcpidgktfux")
public suspend fun hardware(`value`: ResiliencyPolicyPolicyHardwareArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hardware = mapped
}
/**
* @param argument Specifies Infrastructure failure policy. See `policy.hardware`
*/
@JvmName("extfcmpxwaqdohbc")
public suspend fun hardware(argument: suspend ResiliencyPolicyPolicyHardwareArgsBuilder.() -> Unit) {
val toBeMapped = ResiliencyPolicyPolicyHardwareArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.hardware = mapped
}
/**
* @param value Specifies Region failure policy. `policy.region`
*/
@JvmName("avpvnfgyvfrqvelv")
public suspend fun region(`value`: ResiliencyPolicyPolicyRegionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param argument Specifies Region failure policy. `policy.region`
*/
@JvmName("vqktjpdgdsglawct")
public suspend fun region(argument: suspend ResiliencyPolicyPolicyRegionArgsBuilder.() -> Unit) {
val toBeMapped = ResiliencyPolicyPolicyRegionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.region = mapped
}
/**
* @param value Specifies Application failure policy. See `policy.software`
* The following arguments are optional:
*/
@JvmName("ufswpripuwlympkd")
public suspend fun software(`value`: ResiliencyPolicyPolicySoftwareArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.software = mapped
}
/**
* @param argument Specifies Application failure policy. See `policy.software`
* The following arguments are optional:
*/
@JvmName("xvsvqsvnpcbnvkuq")
public suspend fun software(argument: suspend ResiliencyPolicyPolicySoftwareArgsBuilder.() -> Unit) {
val toBeMapped = ResiliencyPolicyPolicySoftwareArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.software = mapped
}
internal fun build(): ResiliencyPolicyPolicyArgs = ResiliencyPolicyPolicyArgs(
az = az,
hardware = hardware,
region = region,
software = software,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy