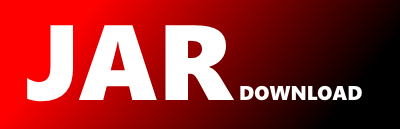
com.pulumi.aws.resourceexplorer.kotlin.ResourceexplorerFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.resourceexplorer.kotlin
import com.pulumi.aws.resourceexplorer.ResourceexplorerFunctions.searchPlain
import com.pulumi.aws.resourceexplorer.kotlin.inputs.SearchPlainArgs
import com.pulumi.aws.resourceexplorer.kotlin.inputs.SearchPlainArgsBuilder
import com.pulumi.aws.resourceexplorer.kotlin.outputs.SearchResult
import com.pulumi.aws.resourceexplorer.kotlin.outputs.SearchResult.Companion.toKotlin
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
public object ResourceexplorerFunctions {
/**
* Data source for managing an AWS Resource Explorer Search.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.resourceexplorer.Search({
* queryString: "region:us-west-2",
* viewArn: test.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.resourceexplorer.search(query_string="region:us-west-2",
* view_arn=test["arn"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.ResourceExplorer.Search.Invoke(new()
* {
* QueryString = "region:us-west-2",
* ViewArn = test.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/resourceexplorer"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := resourceexplorer.Search(ctx, &resourceexplorer.SearchArgs{
* QueryString: "region:us-west-2",
* ViewArn: pulumi.StringRef(test.Arn),
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.resourceexplorer.ResourceexplorerFunctions;
* import com.pulumi.aws.resourceexplorer.inputs.SearchArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ResourceexplorerFunctions.Search(SearchArgs.builder()
* .queryString("region:us-west-2")
* .viewArn(test.arn())
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:resourceexplorer:Search
* Arguments:
* queryString: region:us-west-2
* viewArn: ${test.arn}
* ```
*
* @param argument A collection of arguments for invoking Search.
* @return A collection of values returned by Search.
*/
public suspend fun Search(argument: SearchPlainArgs): SearchResult =
toKotlin(searchPlain(argument.toJava()).await())
/**
* @see [Search].
* @param queryString String that includes keywords and filters that specify the resources that you want to include in the results. For the complete syntax supported by the QueryString parameter, see Search query syntax reference for [Resource Explorer](https://docs.aws.amazon.com/resource-explorer/latest/userguide/using-search-query-syntax.html). The search is completely case insensitive. You can specify an empty string to return all results up to the limit of 1,000 total results. The operation can return only the first 1,000 results. If the resource you want is not included, then use a different value for QueryString to refine the results.
* The following arguments are optional:
* @param viewArn Specifies the Amazon resource name (ARN) of the view to use for the query. If you don't specify a value for this parameter, then the operation automatically uses the default view for the AWS Region in which you called this operation. If the Region either doesn't have a default view or if you don't have permission to use the default view, then the operation fails with a `401 Unauthorized` exception.
* @return A collection of values returned by Search.
*/
public suspend fun Search(queryString: String, viewArn: String? = null): SearchResult {
val argument = SearchPlainArgs(
queryString = queryString,
viewArn = viewArn,
)
return toKotlin(searchPlain(argument.toJava()).await())
}
/**
* @see [Search].
* @param argument Builder for [com.pulumi.aws.resourceexplorer.kotlin.inputs.SearchPlainArgs].
* @return A collection of values returned by Search.
*/
public suspend fun Search(argument: suspend SearchPlainArgsBuilder.() -> Unit): SearchResult {
val builder = SearchPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return toKotlin(searchPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy