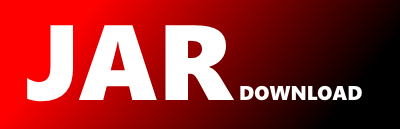
com.pulumi.aws.route53.kotlin.HealthCheck.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [HealthCheck].
*/
@PulumiTagMarker
public class HealthCheckResourceBuilder internal constructor() {
public var name: String? = null
public var args: HealthCheckArgs = HealthCheckArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend HealthCheckArgsBuilder.() -> Unit) {
val builder = HealthCheckArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): HealthCheck {
val builtJavaResource = com.pulumi.aws.route53.HealthCheck(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return HealthCheck(builtJavaResource)
}
}
/**
* Provides a Route53 health check.
* ## Example Usage
* ### Connectivity and HTTP Status Code Check
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.route53.HealthCheck("example", {
* fqdn: "example.com",
* port: 80,
* type: "HTTP",
* resourcePath: "/",
* failureThreshold: 5,
* requestInterval: 30,
* tags: {
* Name: "tf-test-health-check",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.route53.HealthCheck("example",
* fqdn="example.com",
* port=80,
* type="HTTP",
* resource_path="/",
* failure_threshold=5,
* request_interval=30,
* tags={
* "Name": "tf-test-health-check",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Route53.HealthCheck("example", new()
* {
* Fqdn = "example.com",
* Port = 80,
* Type = "HTTP",
* ResourcePath = "/",
* FailureThreshold = 5,
* RequestInterval = 30,
* Tags =
* {
* { "Name", "tf-test-health-check" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := route53.NewHealthCheck(ctx, "example", &route53.HealthCheckArgs{
* Fqdn: pulumi.String("example.com"),
* Port: pulumi.Int(80),
* Type: pulumi.String("HTTP"),
* ResourcePath: pulumi.String("/"),
* FailureThreshold: pulumi.Int(5),
* RequestInterval: pulumi.Int(30),
* Tags: pulumi.StringMap{
* "Name": pulumi.String("tf-test-health-check"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.route53.HealthCheck;
* import com.pulumi.aws.route53.HealthCheckArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new HealthCheck("example", HealthCheckArgs.builder()
* .fqdn("example.com")
* .port(80)
* .type("HTTP")
* .resourcePath("/")
* .failureThreshold("5")
* .requestInterval("30")
* .tags(Map.of("Name", "tf-test-health-check"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:route53:HealthCheck
* properties:
* fqdn: example.com
* port: 80
* type: HTTP
* resourcePath: /
* failureThreshold: '5'
* requestInterval: '30'
* tags:
* Name: tf-test-health-check
* ```
*
* ### Connectivity and String Matching Check
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.route53.HealthCheck("example", {
* failureThreshold: 5,
* fqdn: "example.com",
* port: 443,
* requestInterval: 30,
* resourcePath: "/",
* searchString: "example",
* type: "HTTPS_STR_MATCH",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.route53.HealthCheck("example",
* failure_threshold=5,
* fqdn="example.com",
* port=443,
* request_interval=30,
* resource_path="/",
* search_string="example",
* type="HTTPS_STR_MATCH")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Route53.HealthCheck("example", new()
* {
* FailureThreshold = 5,
* Fqdn = "example.com",
* Port = 443,
* RequestInterval = 30,
* ResourcePath = "/",
* SearchString = "example",
* Type = "HTTPS_STR_MATCH",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := route53.NewHealthCheck(ctx, "example", &route53.HealthCheckArgs{
* FailureThreshold: pulumi.Int(5),
* Fqdn: pulumi.String("example.com"),
* Port: pulumi.Int(443),
* RequestInterval: pulumi.Int(30),
* ResourcePath: pulumi.String("/"),
* SearchString: pulumi.String("example"),
* Type: pulumi.String("HTTPS_STR_MATCH"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.route53.HealthCheck;
* import com.pulumi.aws.route53.HealthCheckArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new HealthCheck("example", HealthCheckArgs.builder()
* .failureThreshold("5")
* .fqdn("example.com")
* .port(443)
* .requestInterval("30")
* .resourcePath("/")
* .searchString("example")
* .type("HTTPS_STR_MATCH")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:route53:HealthCheck
* properties:
* failureThreshold: '5'
* fqdn: example.com
* port: 443
* requestInterval: '30'
* resourcePath: /
* searchString: example
* type: HTTPS_STR_MATCH
* ```
*
* ### Aggregate Check
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const parent = new aws.route53.HealthCheck("parent", {
* type: "CALCULATED",
* childHealthThreshold: 1,
* childHealthchecks: [child.id],
* tags: {
* Name: "tf-test-calculated-health-check",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* parent = aws.route53.HealthCheck("parent",
* type="CALCULATED",
* child_health_threshold=1,
* child_healthchecks=[child["id"]],
* tags={
* "Name": "tf-test-calculated-health-check",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var parent = new Aws.Route53.HealthCheck("parent", new()
* {
* Type = "CALCULATED",
* ChildHealthThreshold = 1,
* ChildHealthchecks = new[]
* {
* child.Id,
* },
* Tags =
* {
* { "Name", "tf-test-calculated-health-check" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := route53.NewHealthCheck(ctx, "parent", &route53.HealthCheckArgs{
* Type: pulumi.String("CALCULATED"),
* ChildHealthThreshold: pulumi.Int(1),
* ChildHealthchecks: pulumi.StringArray{
* child.Id,
* },
* Tags: pulumi.StringMap{
* "Name": pulumi.String("tf-test-calculated-health-check"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.route53.HealthCheck;
* import com.pulumi.aws.route53.HealthCheckArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var parent = new HealthCheck("parent", HealthCheckArgs.builder()
* .type("CALCULATED")
* .childHealthThreshold(1)
* .childHealthchecks(child.id())
* .tags(Map.of("Name", "tf-test-calculated-health-check"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* parent:
* type: aws:route53:HealthCheck
* properties:
* type: CALCULATED
* childHealthThreshold: 1
* childHealthchecks:
* - ${child.id}
* tags:
* Name: tf-test-calculated-health-check
* ```
*
* ### CloudWatch Alarm Check
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foobar = new aws.cloudwatch.MetricAlarm("foobar", {
* name: "test-foobar5",
* comparisonOperator: "GreaterThanOrEqualToThreshold",
* evaluationPeriods: 2,
* metricName: "CPUUtilization",
* namespace: "AWS/EC2",
* period: 120,
* statistic: "Average",
* threshold: 80,
* alarmDescription: "This metric monitors ec2 cpu utilization",
* });
* const foo = new aws.route53.HealthCheck("foo", {
* type: "CLOUDWATCH_METRIC",
* cloudwatchAlarmName: foobar.name,
* cloudwatchAlarmRegion: "us-west-2",
* insufficientDataHealthStatus: "Healthy",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foobar = aws.cloudwatch.MetricAlarm("foobar",
* name="test-foobar5",
* comparison_operator="GreaterThanOrEqualToThreshold",
* evaluation_periods=2,
* metric_name="CPUUtilization",
* namespace="AWS/EC2",
* period=120,
* statistic="Average",
* threshold=80,
* alarm_description="This metric monitors ec2 cpu utilization")
* foo = aws.route53.HealthCheck("foo",
* type="CLOUDWATCH_METRIC",
* cloudwatch_alarm_name=foobar.name,
* cloudwatch_alarm_region="us-west-2",
* insufficient_data_health_status="Healthy")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foobar = new Aws.CloudWatch.MetricAlarm("foobar", new()
* {
* Name = "test-foobar5",
* ComparisonOperator = "GreaterThanOrEqualToThreshold",
* EvaluationPeriods = 2,
* MetricName = "CPUUtilization",
* Namespace = "AWS/EC2",
* Period = 120,
* Statistic = "Average",
* Threshold = 80,
* AlarmDescription = "This metric monitors ec2 cpu utilization",
* });
* var foo = new Aws.Route53.HealthCheck("foo", new()
* {
* Type = "CLOUDWATCH_METRIC",
* CloudwatchAlarmName = foobar.Name,
* CloudwatchAlarmRegion = "us-west-2",
* InsufficientDataHealthStatus = "Healthy",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudwatch"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* foobar, err := cloudwatch.NewMetricAlarm(ctx, "foobar", &cloudwatch.MetricAlarmArgs{
* Name: pulumi.String("test-foobar5"),
* ComparisonOperator: pulumi.String("GreaterThanOrEqualToThreshold"),
* EvaluationPeriods: pulumi.Int(2),
* MetricName: pulumi.String("CPUUtilization"),
* Namespace: pulumi.String("AWS/EC2"),
* Period: pulumi.Int(120),
* Statistic: pulumi.String("Average"),
* Threshold: pulumi.Float64(80),
* AlarmDescription: pulumi.String("This metric monitors ec2 cpu utilization"),
* })
* if err != nil {
* return err
* }
* _, err = route53.NewHealthCheck(ctx, "foo", &route53.HealthCheckArgs{
* Type: pulumi.String("CLOUDWATCH_METRIC"),
* CloudwatchAlarmName: foobar.Name,
* CloudwatchAlarmRegion: pulumi.String("us-west-2"),
* InsufficientDataHealthStatus: pulumi.String("Healthy"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.MetricAlarm;
* import com.pulumi.aws.cloudwatch.MetricAlarmArgs;
* import com.pulumi.aws.route53.HealthCheck;
* import com.pulumi.aws.route53.HealthCheckArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foobar = new MetricAlarm("foobar", MetricAlarmArgs.builder()
* .name("test-foobar5")
* .comparisonOperator("GreaterThanOrEqualToThreshold")
* .evaluationPeriods("2")
* .metricName("CPUUtilization")
* .namespace("AWS/EC2")
* .period("120")
* .statistic("Average")
* .threshold("80")
* .alarmDescription("This metric monitors ec2 cpu utilization")
* .build());
* var foo = new HealthCheck("foo", HealthCheckArgs.builder()
* .type("CLOUDWATCH_METRIC")
* .cloudwatchAlarmName(foobar.name())
* .cloudwatchAlarmRegion("us-west-2")
* .insufficientDataHealthStatus("Healthy")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foobar:
* type: aws:cloudwatch:MetricAlarm
* properties:
* name: test-foobar5
* comparisonOperator: GreaterThanOrEqualToThreshold
* evaluationPeriods: '2'
* metricName: CPUUtilization
* namespace: AWS/EC2
* period: '120'
* statistic: Average
* threshold: '80'
* alarmDescription: This metric monitors ec2 cpu utilization
* foo:
* type: aws:route53:HealthCheck
* properties:
* type: CLOUDWATCH_METRIC
* cloudwatchAlarmName: ${foobar.name}
* cloudwatchAlarmRegion: us-west-2
* insufficientDataHealthStatus: Healthy
* ```
*
* ## Import
* Using `pulumi import`, import Route53 Health Checks using the health check `id`. For example:
* ```sh
* $ pulumi import aws:route53/healthCheck:HealthCheck http_check abcdef11-2222-3333-4444-555555fedcba
* ```
*/
public class HealthCheck internal constructor(
override val javaResource: com.pulumi.aws.route53.HealthCheck,
) : KotlinCustomResource(javaResource, HealthCheckMapper) {
/**
* The Amazon Resource Name (ARN) of the Health Check.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The minimum number of child health checks that must be healthy for Route 53 to consider the parent health check to be healthy. Valid values are integers between 0 and 256, inclusive
*/
public val childHealthThreshold: Output?
get() = javaResource.childHealthThreshold().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* For a specified parent health check, a list of HealthCheckId values for the associated child health checks.
*/
public val childHealthchecks: Output>?
get() = javaResource.childHealthchecks().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The name of the CloudWatch alarm.
*/
public val cloudwatchAlarmName: Output?
get() = javaResource.cloudwatchAlarmName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The CloudWatchRegion that the CloudWatch alarm was created in.
*/
public val cloudwatchAlarmRegion: Output?
get() = javaResource.cloudwatchAlarmRegion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A boolean value that stops Route 53 from performing health checks. When set to true, Route 53 will do the following depending on the type of health check:
* * For health checks that check the health of endpoints, Route5 53 stops submitting requests to your application, server, or other resource.
* * For calculated health checks, Route 53 stops aggregating the status of the referenced health checks.
* * For health checks that monitor CloudWatch alarms, Route 53 stops monitoring the corresponding CloudWatch metrics.
* > **Note:** After you disable a health check, Route 53 considers the status of the health check to always be healthy. If you configured DNS failover, Route 53 continues to route traffic to the corresponding resources. If you want to stop routing traffic to a resource, change the value of `invert_healthcheck`.
*/
public val disabled: Output?
get() = javaResource.disabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A boolean value that indicates whether Route53 should send the `fqdn` to the endpoint when performing the health check. This defaults to AWS' defaults: when the `type` is "HTTPS" `enable_sni` defaults to `true`, when `type` is anything else `enable_sni` defaults to `false`.
*/
public val enableSni: Output
get() = javaResource.enableSni().applyValue({ args0 -> args0 })
/**
* The number of consecutive health checks that an endpoint must pass or fail.
*/
public val failureThreshold: Output
get() = javaResource.failureThreshold().applyValue({ args0 -> args0 })
/**
* The fully qualified domain name of the endpoint to be checked. If a value is set for `ip_address`, the value set for `fqdn` will be passed in the `Host` header.
*/
public val fqdn: Output?
get() = javaResource.fqdn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The status of the health check when CloudWatch has insufficient data about the state of associated alarm. Valid values are `Healthy` , `Unhealthy` and `LastKnownStatus`.
*/
public val insufficientDataHealthStatus: Output?
get() = javaResource.insufficientDataHealthStatus().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A boolean value that indicates whether the status of health check should be inverted. For example, if a health check is healthy but Inverted is True , then Route 53 considers the health check to be unhealthy.
*/
public val invertHealthcheck: Output?
get() = javaResource.invertHealthcheck().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The IP address of the endpoint to be checked.
*/
public val ipAddress: Output?
get() = javaResource.ipAddress().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A Boolean value that indicates whether you want Route 53 to measure the latency between health checkers in multiple AWS regions and your endpoint and to display CloudWatch latency graphs in the Route 53 console.
*/
public val measureLatency: Output?
get() = javaResource.measureLatency().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The port of the endpoint to be checked.
*/
public val port: Output?
get() = javaResource.port().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* This is a reference name used in Caller Reference
* (helpful for identifying single health_check set amongst others)
*/
public val referenceName: Output?
get() = javaResource.referenceName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of AWS regions that you want Amazon Route 53 health checkers to check the specified endpoint from.
*/
public val regions: Output>?
get() = javaResource.regions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The number of seconds between the time that Amazon Route 53 gets a response from your endpoint and the time that it sends the next health-check request.
*/
public val requestInterval: Output?
get() = javaResource.requestInterval().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The path that you want Amazon Route 53 to request when performing health checks.
*/
public val resourcePath: Output?
get() = javaResource.resourcePath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) for the Route 53 Application Recovery Controller routing control. This is used when health check type is `RECOVERY_CONTROL`
*/
public val routingControlArn: Output?
get() = javaResource.routingControlArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* String searched in the first 5120 bytes of the response body for check to be considered healthy. Only valid with `HTTP_STR_MATCH` and `HTTPS_STR_MATCH`.
*/
public val searchString: Output?
get() = javaResource.searchString().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A map of tags to assign to the health check. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy