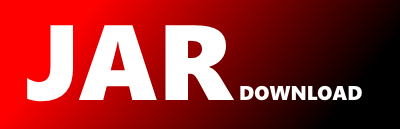
com.pulumi.aws.route53.kotlin.ProfilesAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53.kotlin
import com.pulumi.aws.route53.ProfilesAssociationArgs.builder
import com.pulumi.aws.route53.kotlin.inputs.ProfilesAssociationTimeoutsArgs
import com.pulumi.aws.route53.kotlin.inputs.ProfilesAssociationTimeoutsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource for managing an AWS Route 53 Profiles Association.
* ## Example Usage
* ### Basic Usage
*
* ```yaml
* resources:
* example:
* type: aws:route53:ProfilesProfile
* properties:
* name: example
* exampleVpc:
* type: aws:ec2:Vpc
* name: example
* properties:
* cidr: 10.0.0.0/16
* exampleProfilesAssociation:
* type: aws:route53:ProfilesAssociation
* name: example
* properties:
* name: example
* profileId: ${example.id}
* resourceId: ${exampleVpc.id}
* ```
*
* ## Import
* Using `pulumi import`, import Route 53 Profiles Association using the `example_id_arg`. For example:
* ```sh
* $ pulumi import aws:route53/profilesAssociation:ProfilesAssociation example rpa-id-12345678
* ```
* @property name Name of the Profile Association. Must match a regex of `(?!^[0-9]+$)([a-zA-Z0-9\\-_' ']+)`.
* @property profileId ID of the profile associated with the VPC.
* @property resourceId Resource ID of the VPC the profile to be associated with.
* @property tags
* @property timeouts
*/
public data class ProfilesAssociationArgs(
public val name: Output? = null,
public val profileId: Output? = null,
public val resourceId: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy