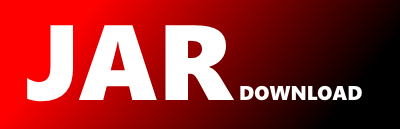
com.pulumi.aws.route53.kotlin.ProfilesResourceAssociationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53.kotlin
import com.pulumi.aws.route53.ProfilesResourceAssociationArgs.builder
import com.pulumi.aws.route53.kotlin.inputs.ProfilesResourceAssociationTimeoutsArgs
import com.pulumi.aws.route53.kotlin.inputs.ProfilesResourceAssociationTimeoutsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource for managing an AWS Route 53 Profiles Resource Association.
* ## Example Usage
* ### Basic Usage
*
* ```yaml
* resources:
* example:
* type: aws:route53:ProfilesProfile
* properties:
* name: example
* exampleVpc:
* type: aws:ec2:Vpc
* name: example
* properties:
* cidr: 10.0.0.0/16
* exampleZone:
* type: aws:route53:Zone
* name: example
* properties:
* name: example.com
* vpcs:
* - vpcId: ${exampleVpc.id}
* exampleProfilesResourceAssociation:
* type: aws:route53:ProfilesResourceAssociation
* name: example
* properties:
* name: example
* profileId: ${example.id}
* resourceArn: ${exampleZone.arn}
* ```
*
* ## Import
* Using `pulumi import`, import Route 53 Profiles Resource Association using the `example_id_arg`. For example:
* ```sh
* $ pulumi import aws:route53/profilesResourceAssociation:ProfilesResourceAssociation example rpa-id-12345678
* ```
* @property name Name of the Profile Resource Association.
* @property profileId ID of the profile associated with the VPC.
* @property resourceArn Resource ID of the resource to be associated with the profile.
* @property resourceProperties Resource properties for the resource to be associated with the profile.
* @property timeouts
*/
public data class ProfilesResourceAssociationArgs(
public val name: Output? = null,
public val profileId: Output? = null,
public val resourceArn: Output? = null,
public val resourceProperties: Output? = null,
public val timeouts: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.route53.ProfilesResourceAssociationArgs =
com.pulumi.aws.route53.ProfilesResourceAssociationArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.profileId(profileId?.applyValue({ args0 -> args0 }))
.resourceArn(resourceArn?.applyValue({ args0 -> args0 }))
.resourceProperties(resourceProperties?.applyValue({ args0 -> args0 }))
.timeouts(timeouts?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ProfilesResourceAssociationArgs].
*/
@PulumiTagMarker
public class ProfilesResourceAssociationArgsBuilder internal constructor() {
private var name: Output? = null
private var profileId: Output? = null
private var resourceArn: Output? = null
private var resourceProperties: Output? = null
private var timeouts: Output? = null
/**
* @param value Name of the Profile Resource Association.
*/
@JvmName("lrcueydvdnhmyhvu")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value ID of the profile associated with the VPC.
*/
@JvmName("qavrusbwqmaxdnme")
public suspend fun profileId(`value`: Output) {
this.profileId = value
}
/**
* @param value Resource ID of the resource to be associated with the profile.
*/
@JvmName("tgovwebasouypdra")
public suspend fun resourceArn(`value`: Output) {
this.resourceArn = value
}
/**
* @param value Resource properties for the resource to be associated with the profile.
*/
@JvmName("uxnndcyhiwhvhrlw")
public suspend fun resourceProperties(`value`: Output) {
this.resourceProperties = value
}
/**
* @param value
*/
@JvmName("prmuxcuduorormjw")
public suspend fun timeouts(`value`: Output) {
this.timeouts = value
}
/**
* @param value Name of the Profile Resource Association.
*/
@JvmName("vtfqiftsebekjaxi")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value ID of the profile associated with the VPC.
*/
@JvmName("pecrtrpumdctphub")
public suspend fun profileId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.profileId = mapped
}
/**
* @param value Resource ID of the resource to be associated with the profile.
*/
@JvmName("gfjnrclatgfyinko")
public suspend fun resourceArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceArn = mapped
}
/**
* @param value Resource properties for the resource to be associated with the profile.
*/
@JvmName("ytbughcivnohigae")
public suspend fun resourceProperties(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceProperties = mapped
}
/**
* @param value
*/
@JvmName("rnjfaencwvjurlrg")
public suspend fun timeouts(`value`: ProfilesResourceAssociationTimeoutsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeouts = mapped
}
/**
* @param argument
*/
@JvmName("ioekgdpojksiwrrp")
public suspend fun timeouts(argument: suspend ProfilesResourceAssociationTimeoutsArgsBuilder.() -> Unit) {
val toBeMapped = ProfilesResourceAssociationTimeoutsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timeouts = mapped
}
internal fun build(): ProfilesResourceAssociationArgs = ProfilesResourceAssociationArgs(
name = name,
profileId = profileId,
resourceArn = resourceArn,
resourceProperties = resourceProperties,
timeouts = timeouts,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy