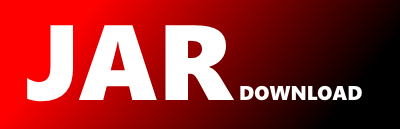
com.pulumi.aws.route53.kotlin.ResolverConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53.kotlin
import com.pulumi.aws.route53.ResolverConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a Route 53 Resolver config resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ec2.Vpc("example", {
* cidrBlock: "10.0.0.0/16",
* enableDnsSupport: true,
* enableDnsHostnames: true,
* });
* const exampleResolverConfig = new aws.route53.ResolverConfig("example", {
* resourceId: example.id,
* autodefinedReverseFlag: "DISABLE",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ec2.Vpc("example",
* cidr_block="10.0.0.0/16",
* enable_dns_support=True,
* enable_dns_hostnames=True)
* example_resolver_config = aws.route53.ResolverConfig("example",
* resource_id=example.id,
* autodefined_reverse_flag="DISABLE")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ec2.Vpc("example", new()
* {
* CidrBlock = "10.0.0.0/16",
* EnableDnsSupport = true,
* EnableDnsHostnames = true,
* });
* var exampleResolverConfig = new Aws.Route53.ResolverConfig("example", new()
* {
* ResourceId = example.Id,
* AutodefinedReverseFlag = "DISABLE",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := ec2.NewVpc(ctx, "example", &ec2.VpcArgs{
* CidrBlock: pulumi.String("10.0.0.0/16"),
* EnableDnsSupport: pulumi.Bool(true),
* EnableDnsHostnames: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = route53.NewResolverConfig(ctx, "example", &route53.ResolverConfigArgs{
* ResourceId: example.ID(),
* AutodefinedReverseFlag: pulumi.String("DISABLE"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.route53.ResolverConfig;
* import com.pulumi.aws.route53.ResolverConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Vpc("example", VpcArgs.builder()
* .cidrBlock("10.0.0.0/16")
* .enableDnsSupport(true)
* .enableDnsHostnames(true)
* .build());
* var exampleResolverConfig = new ResolverConfig("exampleResolverConfig", ResolverConfigArgs.builder()
* .resourceId(example.id())
* .autodefinedReverseFlag("DISABLE")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ec2:Vpc
* properties:
* cidrBlock: 10.0.0.0/16
* enableDnsSupport: true
* enableDnsHostnames: true
* exampleResolverConfig:
* type: aws:route53:ResolverConfig
* name: example
* properties:
* resourceId: ${example.id}
* autodefinedReverseFlag: DISABLE
* ```
*
* ## Import
* Using `pulumi import`, import Route 53 Resolver configs using the Route 53 Resolver config ID. For example:
* ```sh
* $ pulumi import aws:route53/resolverConfig:ResolverConfig example rslvr-rc-715aa20c73a23da7
* ```
* @property autodefinedReverseFlag Indicates whether or not the Resolver will create autodefined rules for reverse DNS lookups. Valid values: `ENABLE`, `DISABLE`.
* @property resourceId The ID of the VPC that the configuration is for.
*/
public data class ResolverConfigArgs(
public val autodefinedReverseFlag: Output? = null,
public val resourceId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.route53.ResolverConfigArgs =
com.pulumi.aws.route53.ResolverConfigArgs.builder()
.autodefinedReverseFlag(autodefinedReverseFlag?.applyValue({ args0 -> args0 }))
.resourceId(resourceId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ResolverConfigArgs].
*/
@PulumiTagMarker
public class ResolverConfigArgsBuilder internal constructor() {
private var autodefinedReverseFlag: Output? = null
private var resourceId: Output? = null
/**
* @param value Indicates whether or not the Resolver will create autodefined rules for reverse DNS lookups. Valid values: `ENABLE`, `DISABLE`.
*/
@JvmName("ngrqpplqnawweekr")
public suspend fun autodefinedReverseFlag(`value`: Output) {
this.autodefinedReverseFlag = value
}
/**
* @param value The ID of the VPC that the configuration is for.
*/
@JvmName("vxxbinbjhaxhlmkd")
public suspend fun resourceId(`value`: Output) {
this.resourceId = value
}
/**
* @param value Indicates whether or not the Resolver will create autodefined rules for reverse DNS lookups. Valid values: `ENABLE`, `DISABLE`.
*/
@JvmName("ivrtliehdejvkyip")
public suspend fun autodefinedReverseFlag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autodefinedReverseFlag = mapped
}
/**
* @param value The ID of the VPC that the configuration is for.
*/
@JvmName("bqdfhhvuovjmolfy")
public suspend fun resourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceId = mapped
}
internal fun build(): ResolverConfigArgs = ResolverConfigArgs(
autodefinedReverseFlag = autodefinedReverseFlag,
resourceId = resourceId,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy