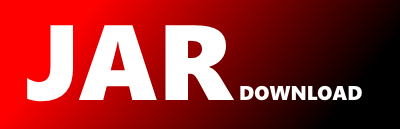
com.pulumi.aws.route53.kotlin.inputs.GetResolverRulesPlainArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53.kotlin.inputs
import com.pulumi.aws.route53.inputs.GetResolverRulesPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getResolverRules.
* @property nameRegex Regex string to filter resolver rule names.
* The filtering is done locally, so could have a performance impact if the result is large.
* This argument should be used along with other arguments to limit the number of results returned.
* @property ownerId When the desired resolver rules are shared with another AWS account, the account ID of the account that the rules are shared with.
* @property resolverEndpointId ID of the outbound resolver endpoint for the desired resolver rules.
* @property ruleType Rule type of the desired resolver rules. Valid values are `FORWARD`, `SYSTEM` and `RECURSIVE`.
* @property shareStatus Whether the desired resolver rules are shared and, if so, whether the current account is sharing the rules with another account, or another account is sharing the rules with the current account. Valid values are `NOT_SHARED`, `SHARED_BY_ME` or `SHARED_WITH_ME`
*/
public data class GetResolverRulesPlainArgs(
public val nameRegex: String? = null,
public val ownerId: String? = null,
public val resolverEndpointId: String? = null,
public val ruleType: String? = null,
public val shareStatus: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.route53.inputs.GetResolverRulesPlainArgs =
com.pulumi.aws.route53.inputs.GetResolverRulesPlainArgs.builder()
.nameRegex(nameRegex?.let({ args0 -> args0 }))
.ownerId(ownerId?.let({ args0 -> args0 }))
.resolverEndpointId(resolverEndpointId?.let({ args0 -> args0 }))
.ruleType(ruleType?.let({ args0 -> args0 }))
.shareStatus(shareStatus?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetResolverRulesPlainArgs].
*/
@PulumiTagMarker
public class GetResolverRulesPlainArgsBuilder internal constructor() {
private var nameRegex: String? = null
private var ownerId: String? = null
private var resolverEndpointId: String? = null
private var ruleType: String? = null
private var shareStatus: String? = null
/**
* @param value Regex string to filter resolver rule names.
* The filtering is done locally, so could have a performance impact if the result is large.
* This argument should be used along with other arguments to limit the number of results returned.
*/
@JvmName("xxcgtqqqlqfmqmhy")
public suspend fun nameRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.nameRegex = mapped
}
/**
* @param value When the desired resolver rules are shared with another AWS account, the account ID of the account that the rules are shared with.
*/
@JvmName("hkrardcgoixuuiat")
public suspend fun ownerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ownerId = mapped
}
/**
* @param value ID of the outbound resolver endpoint for the desired resolver rules.
*/
@JvmName("dystlbpbubbjsirf")
public suspend fun resolverEndpointId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.resolverEndpointId = mapped
}
/**
* @param value Rule type of the desired resolver rules. Valid values are `FORWARD`, `SYSTEM` and `RECURSIVE`.
*/
@JvmName("orlpresjllvwnfey")
public suspend fun ruleType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ruleType = mapped
}
/**
* @param value Whether the desired resolver rules are shared and, if so, whether the current account is sharing the rules with another account, or another account is sharing the rules with the current account. Valid values are `NOT_SHARED`, `SHARED_BY_ME` or `SHARED_WITH_ME`
*/
@JvmName("ytaurwtstushwkbh")
public suspend fun shareStatus(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.shareStatus = mapped
}
internal fun build(): GetResolverRulesPlainArgs = GetResolverRulesPlainArgs(
nameRegex = nameRegex,
ownerId = ownerId,
resolverEndpointId = resolverEndpointId,
ruleType = ruleType,
shareStatus = shareStatus,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy