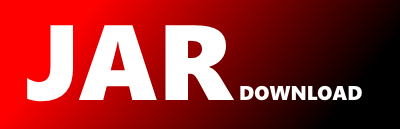
com.pulumi.aws.route53.kotlin.inputs.GetTrafficPolicyDocumentRuleLocation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53.kotlin.inputs
import com.pulumi.aws.route53.inputs.GetTrafficPolicyDocumentRuleLocation.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property continent Value of a continent.
* @property country Value of a country.
* @property endpointReference References to an endpoint.
* @property evaluateTargetHealth Indicates whether you want Amazon Route 53 to evaluate the health of the endpoint and route traffic only to healthy endpoints.
* @property healthCheck If you want to associate a health check with the endpoint or rule.
* @property isDefault Indicates whether this set of values represents the default location.
* @property ruleReference References to a rule.
* @property subdivision Value of a subdivision.
*/
public data class GetTrafficPolicyDocumentRuleLocation(
public val continent: String? = null,
public val country: String? = null,
public val endpointReference: String? = null,
public val evaluateTargetHealth: Boolean? = null,
public val healthCheck: String? = null,
public val isDefault: Boolean? = null,
public val ruleReference: String? = null,
public val subdivision: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.route53.inputs.GetTrafficPolicyDocumentRuleLocation =
com.pulumi.aws.route53.inputs.GetTrafficPolicyDocumentRuleLocation.builder()
.continent(continent?.let({ args0 -> args0 }))
.country(country?.let({ args0 -> args0 }))
.endpointReference(endpointReference?.let({ args0 -> args0 }))
.evaluateTargetHealth(evaluateTargetHealth?.let({ args0 -> args0 }))
.healthCheck(healthCheck?.let({ args0 -> args0 }))
.isDefault(isDefault?.let({ args0 -> args0 }))
.ruleReference(ruleReference?.let({ args0 -> args0 }))
.subdivision(subdivision?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetTrafficPolicyDocumentRuleLocation].
*/
@PulumiTagMarker
public class GetTrafficPolicyDocumentRuleLocationBuilder internal constructor() {
private var continent: String? = null
private var country: String? = null
private var endpointReference: String? = null
private var evaluateTargetHealth: Boolean? = null
private var healthCheck: String? = null
private var isDefault: Boolean? = null
private var ruleReference: String? = null
private var subdivision: String? = null
/**
* @param value Value of a continent.
*/
@JvmName("uxxqloxrsnpevwau")
public suspend fun continent(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.continent = mapped
}
/**
* @param value Value of a country.
*/
@JvmName("wkfabukylumapnxl")
public suspend fun country(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.country = mapped
}
/**
* @param value References to an endpoint.
*/
@JvmName("xtqejlnembsvwtow")
public suspend fun endpointReference(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.endpointReference = mapped
}
/**
* @param value Indicates whether you want Amazon Route 53 to evaluate the health of the endpoint and route traffic only to healthy endpoints.
*/
@JvmName("jcltlfvnyfacnfvr")
public suspend fun evaluateTargetHealth(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.evaluateTargetHealth = mapped
}
/**
* @param value If you want to associate a health check with the endpoint or rule.
*/
@JvmName("febgiwowthrqgoad")
public suspend fun healthCheck(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.healthCheck = mapped
}
/**
* @param value Indicates whether this set of values represents the default location.
*/
@JvmName("fekfsltabglyxieu")
public suspend fun isDefault(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.isDefault = mapped
}
/**
* @param value References to a rule.
*/
@JvmName("xhtqkqiorpwcivjj")
public suspend fun ruleReference(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ruleReference = mapped
}
/**
* @param value Value of a subdivision.
*/
@JvmName("rmbiivjjljahdepm")
public suspend fun subdivision(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.subdivision = mapped
}
internal fun build(): GetTrafficPolicyDocumentRuleLocation = GetTrafficPolicyDocumentRuleLocation(
continent = continent,
country = country,
endpointReference = endpointReference,
evaluateTargetHealth = evaluateTargetHealth,
healthCheck = healthCheck,
isDefault = isDefault,
ruleReference = ruleReference,
subdivision = subdivision,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy