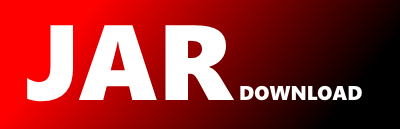
com.pulumi.aws.route53recoverycontrol.kotlin.SafetyRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.route53recoverycontrol.kotlin
import com.pulumi.aws.route53recoverycontrol.SafetyRuleArgs.builder
import com.pulumi.aws.route53recoverycontrol.kotlin.inputs.SafetyRuleRuleConfigArgs
import com.pulumi.aws.route53recoverycontrol.kotlin.inputs.SafetyRuleRuleConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides an AWS Route 53 Recovery Control Config Safety Rule
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.route53recoverycontrol.SafetyRule("example", {
* assertedControls: [exampleAwsRoute53recoverycontrolconfigRoutingControl.arn],
* controlPanelArn: "arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8",
* name: "daisyguttridge",
* waitPeriodMs: 5000,
* ruleConfig: {
* inverted: false,
* threshold: 1,
* type: "ATLEAST",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.route53recoverycontrol.SafetyRule("example",
* asserted_controls=[example_aws_route53recoverycontrolconfig_routing_control["arn"]],
* control_panel_arn="arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8",
* name="daisyguttridge",
* wait_period_ms=5000,
* rule_config={
* "inverted": False,
* "threshold": 1,
* "type": "ATLEAST",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Route53RecoveryControl.SafetyRule("example", new()
* {
* AssertedControls = new[]
* {
* exampleAwsRoute53recoverycontrolconfigRoutingControl.Arn,
* },
* ControlPanelArn = "arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8",
* Name = "daisyguttridge",
* WaitPeriodMs = 5000,
* RuleConfig = new Aws.Route53RecoveryControl.Inputs.SafetyRuleRuleConfigArgs
* {
* Inverted = false,
* Threshold = 1,
* Type = "ATLEAST",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53recoverycontrol"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := route53recoverycontrol.NewSafetyRule(ctx, "example", &route53recoverycontrol.SafetyRuleArgs{
* AssertedControls: pulumi.StringArray{
* exampleAwsRoute53recoverycontrolconfigRoutingControl.Arn,
* },
* ControlPanelArn: pulumi.String("arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8"),
* Name: pulumi.String("daisyguttridge"),
* WaitPeriodMs: pulumi.Int(5000),
* RuleConfig: &route53recoverycontrol.SafetyRuleRuleConfigArgs{
* Inverted: pulumi.Bool(false),
* Threshold: pulumi.Int(1),
* Type: pulumi.String("ATLEAST"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.route53recoverycontrol.SafetyRule;
* import com.pulumi.aws.route53recoverycontrol.SafetyRuleArgs;
* import com.pulumi.aws.route53recoverycontrol.inputs.SafetyRuleRuleConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new SafetyRule("example", SafetyRuleArgs.builder()
* .assertedControls(exampleAwsRoute53recoverycontrolconfigRoutingControl.arn())
* .controlPanelArn("arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8")
* .name("daisyguttridge")
* .waitPeriodMs(5000)
* .ruleConfig(SafetyRuleRuleConfigArgs.builder()
* .inverted(false)
* .threshold(1)
* .type("ATLEAST")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:route53recoverycontrol:SafetyRule
* properties:
* assertedControls:
* - ${exampleAwsRoute53recoverycontrolconfigRoutingControl.arn}
* controlPanelArn: arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8
* name: daisyguttridge
* waitPeriodMs: 5000
* ruleConfig:
* inverted: false
* threshold: 1
* type: ATLEAST
* ```
*
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.route53recoverycontrol.SafetyRule("example", {
* name: "i_o",
* controlPanelArn: "arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8",
* waitPeriodMs: 5000,
* gatingControls: [exampleAwsRoute53recoverycontrolconfigRoutingControl.arn],
* targetControls: [exampleAwsRoute53recoverycontrolconfigRoutingControl.arn],
* ruleConfig: {
* inverted: false,
* threshold: 1,
* type: "ATLEAST",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.route53recoverycontrol.SafetyRule("example",
* name="i_o",
* control_panel_arn="arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8",
* wait_period_ms=5000,
* gating_controls=[example_aws_route53recoverycontrolconfig_routing_control["arn"]],
* target_controls=[example_aws_route53recoverycontrolconfig_routing_control["arn"]],
* rule_config={
* "inverted": False,
* "threshold": 1,
* "type": "ATLEAST",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Route53RecoveryControl.SafetyRule("example", new()
* {
* Name = "i_o",
* ControlPanelArn = "arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8",
* WaitPeriodMs = 5000,
* GatingControls = new[]
* {
* exampleAwsRoute53recoverycontrolconfigRoutingControl.Arn,
* },
* TargetControls = new[]
* {
* exampleAwsRoute53recoverycontrolconfigRoutingControl.Arn,
* },
* RuleConfig = new Aws.Route53RecoveryControl.Inputs.SafetyRuleRuleConfigArgs
* {
* Inverted = false,
* Threshold = 1,
* Type = "ATLEAST",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/route53recoverycontrol"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := route53recoverycontrol.NewSafetyRule(ctx, "example", &route53recoverycontrol.SafetyRuleArgs{
* Name: pulumi.String("i_o"),
* ControlPanelArn: pulumi.String("arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8"),
* WaitPeriodMs: pulumi.Int(5000),
* GatingControls: pulumi.StringArray{
* exampleAwsRoute53recoverycontrolconfigRoutingControl.Arn,
* },
* TargetControls: pulumi.StringArray{
* exampleAwsRoute53recoverycontrolconfigRoutingControl.Arn,
* },
* RuleConfig: &route53recoverycontrol.SafetyRuleRuleConfigArgs{
* Inverted: pulumi.Bool(false),
* Threshold: pulumi.Int(1),
* Type: pulumi.String("ATLEAST"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.route53recoverycontrol.SafetyRule;
* import com.pulumi.aws.route53recoverycontrol.SafetyRuleArgs;
* import com.pulumi.aws.route53recoverycontrol.inputs.SafetyRuleRuleConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new SafetyRule("example", SafetyRuleArgs.builder()
* .name("i_o")
* .controlPanelArn("arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8")
* .waitPeriodMs(5000)
* .gatingControls(exampleAwsRoute53recoverycontrolconfigRoutingControl.arn())
* .targetControls(exampleAwsRoute53recoverycontrolconfigRoutingControl.arn())
* .ruleConfig(SafetyRuleRuleConfigArgs.builder()
* .inverted(false)
* .threshold(1)
* .type("ATLEAST")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:route53recoverycontrol:SafetyRule
* properties:
* name: i_o
* controlPanelArn: arn:aws:route53-recovery-control::313517334327:controlpanel/abd5fbfc052d4844a082dbf400f61da8
* waitPeriodMs: 5000
* gatingControls:
* - ${exampleAwsRoute53recoverycontrolconfigRoutingControl.arn}
* targetControls:
* - ${exampleAwsRoute53recoverycontrolconfigRoutingControl.arn}
* ruleConfig:
* inverted: false
* threshold: 1
* type: ATLEAST
* ```
*
* ## Import
* Using `pulumi import`, import Route53 Recovery Control Config Safety Rule using the safety rule ARN. For example:
* ```sh
* $ pulumi import aws:route53recoverycontrol/safetyRule:SafetyRule myrule arn:aws:route53-recovery-control::313517334327:controlpanel/1bfba17df8684f5dab0467b71424f7e8/safetyrule/3bacc77003364c0f
* ```
* @property assertedControls Routing controls that are part of transactions that are evaluated to determine if a request to change a routing control state is allowed.
* @property controlPanelArn ARN of the control panel in which this safety rule will reside.
* @property gatingControls Gating controls for the new gating rule. That is, routing controls that are evaluated by the rule configuration that you specify.
* @property name Name describing the safety rule.
* @property ruleConfig Configuration block for safety rule criteria. See below.
* @property targetControls Routing controls that can only be set or unset if the specified `rule_config` evaluates to true for the specified `gating_controls`.
* @property waitPeriodMs Evaluation period, in milliseconds (ms), during which any request against the target routing controls will fail.
* The following arguments are optional:
*/
public data class SafetyRuleArgs(
public val assertedControls: Output>? = null,
public val controlPanelArn: Output? = null,
public val gatingControls: Output>? = null,
public val name: Output? = null,
public val ruleConfig: Output? = null,
public val targetControls: Output>? = null,
public val waitPeriodMs: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.route53recoverycontrol.SafetyRuleArgs =
com.pulumi.aws.route53recoverycontrol.SafetyRuleArgs.builder()
.assertedControls(assertedControls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.controlPanelArn(controlPanelArn?.applyValue({ args0 -> args0 }))
.gatingControls(gatingControls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name?.applyValue({ args0 -> args0 }))
.ruleConfig(ruleConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetControls(targetControls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.waitPeriodMs(waitPeriodMs?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SafetyRuleArgs].
*/
@PulumiTagMarker
public class SafetyRuleArgsBuilder internal constructor() {
private var assertedControls: Output>? = null
private var controlPanelArn: Output? = null
private var gatingControls: Output>? = null
private var name: Output? = null
private var ruleConfig: Output? = null
private var targetControls: Output>? = null
private var waitPeriodMs: Output? = null
/**
* @param value Routing controls that are part of transactions that are evaluated to determine if a request to change a routing control state is allowed.
*/
@JvmName("sbjthreocsinyopr")
public suspend fun assertedControls(`value`: Output>) {
this.assertedControls = value
}
@JvmName("fqlfybovwsmbdqsc")
public suspend fun assertedControls(vararg values: Output) {
this.assertedControls = Output.all(values.asList())
}
/**
* @param values Routing controls that are part of transactions that are evaluated to determine if a request to change a routing control state is allowed.
*/
@JvmName("wyhopukkgvtturbx")
public suspend fun assertedControls(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy