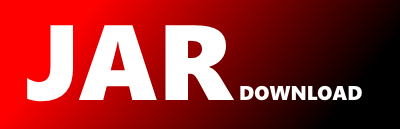
com.pulumi.aws.s3.kotlin.BucketAclV2Args.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin
import com.pulumi.aws.s3.BucketAclV2Args.builder
import com.pulumi.aws.s3.kotlin.inputs.BucketAclV2AccessControlPolicyArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketAclV2AccessControlPolicyArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Provides an S3 bucket ACL resource.
* > **Note:** destroy does not delete the S3 Bucket ACL but does remove the resource from state.
* > This resource cannot be used with S3 directory buckets.
* ## Example Usage
* ### With `private` ACL
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.s3.BucketV2("example", {bucket: "my-tf-example-bucket"});
* const exampleBucketOwnershipControls = new aws.s3.BucketOwnershipControls("example", {
* bucket: example.id,
* rule: {
* objectOwnership: "BucketOwnerPreferred",
* },
* });
* const exampleBucketAclV2 = new aws.s3.BucketAclV2("example", {
* bucket: example.id,
* acl: "private",
* }, {
* dependsOn: [exampleBucketOwnershipControls],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.s3.BucketV2("example", bucket="my-tf-example-bucket")
* example_bucket_ownership_controls = aws.s3.BucketOwnershipControls("example",
* bucket=example.id,
* rule={
* "object_ownership": "BucketOwnerPreferred",
* })
* example_bucket_acl_v2 = aws.s3.BucketAclV2("example",
* bucket=example.id,
* acl="private",
* opts = pulumi.ResourceOptions(depends_on=[example_bucket_ownership_controls]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "my-tf-example-bucket",
* });
* var exampleBucketOwnershipControls = new Aws.S3.BucketOwnershipControls("example", new()
* {
* Bucket = example.Id,
* Rule = new Aws.S3.Inputs.BucketOwnershipControlsRuleArgs
* {
* ObjectOwnership = "BucketOwnerPreferred",
* },
* });
* var exampleBucketAclV2 = new Aws.S3.BucketAclV2("example", new()
* {
* Bucket = example.Id,
* Acl = "private",
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleBucketOwnershipControls,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-example-bucket"),
* })
* if err != nil {
* return err
* }
* exampleBucketOwnershipControls, err := s3.NewBucketOwnershipControls(ctx, "example", &s3.BucketOwnershipControlsArgs{
* Bucket: example.ID(),
* Rule: &s3.BucketOwnershipControlsRuleArgs{
* ObjectOwnership: pulumi.String("BucketOwnerPreferred"),
* },
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketAclV2(ctx, "example", &s3.BucketAclV2Args{
* Bucket: example.ID(),
* Acl: pulumi.String("private"),
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleBucketOwnershipControls,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketOwnershipControls;
* import com.pulumi.aws.s3.BucketOwnershipControlsArgs;
* import com.pulumi.aws.s3.inputs.BucketOwnershipControlsRuleArgs;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("my-tf-example-bucket")
* .build());
* var exampleBucketOwnershipControls = new BucketOwnershipControls("exampleBucketOwnershipControls", BucketOwnershipControlsArgs.builder()
* .bucket(example.id())
* .rule(BucketOwnershipControlsRuleArgs.builder()
* .objectOwnership("BucketOwnerPreferred")
* .build())
* .build());
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(example.id())
* .acl("private")
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleBucketOwnershipControls)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-example-bucket
* exampleBucketOwnershipControls:
* type: aws:s3:BucketOwnershipControls
* name: example
* properties:
* bucket: ${example.id}
* rule:
* objectOwnership: BucketOwnerPreferred
* exampleBucketAclV2:
* type: aws:s3:BucketAclV2
* name: example
* properties:
* bucket: ${example.id}
* acl: private
* options:
* dependson:
* - ${exampleBucketOwnershipControls}
* ```
*
* ### With `public-read` ACL
* > This example explicitly disables the default S3 bucket security settings. This
* should be done with caution, as all bucket objects become publicly exposed.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.s3.BucketV2("example", {bucket: "my-tf-example-bucket"});
* const exampleBucketOwnershipControls = new aws.s3.BucketOwnershipControls("example", {
* bucket: example.id,
* rule: {
* objectOwnership: "BucketOwnerPreferred",
* },
* });
* const exampleBucketPublicAccessBlock = new aws.s3.BucketPublicAccessBlock("example", {
* bucket: example.id,
* blockPublicAcls: false,
* blockPublicPolicy: false,
* ignorePublicAcls: false,
* restrictPublicBuckets: false,
* });
* const exampleBucketAclV2 = new aws.s3.BucketAclV2("example", {
* bucket: example.id,
* acl: "public-read",
* }, {
* dependsOn: [
* exampleBucketOwnershipControls,
* exampleBucketPublicAccessBlock,
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.s3.BucketV2("example", bucket="my-tf-example-bucket")
* example_bucket_ownership_controls = aws.s3.BucketOwnershipControls("example",
* bucket=example.id,
* rule={
* "object_ownership": "BucketOwnerPreferred",
* })
* example_bucket_public_access_block = aws.s3.BucketPublicAccessBlock("example",
* bucket=example.id,
* block_public_acls=False,
* block_public_policy=False,
* ignore_public_acls=False,
* restrict_public_buckets=False)
* example_bucket_acl_v2 = aws.s3.BucketAclV2("example",
* bucket=example.id,
* acl="public-read",
* opts = pulumi.ResourceOptions(depends_on=[
* example_bucket_ownership_controls,
* example_bucket_public_access_block,
* ]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "my-tf-example-bucket",
* });
* var exampleBucketOwnershipControls = new Aws.S3.BucketOwnershipControls("example", new()
* {
* Bucket = example.Id,
* Rule = new Aws.S3.Inputs.BucketOwnershipControlsRuleArgs
* {
* ObjectOwnership = "BucketOwnerPreferred",
* },
* });
* var exampleBucketPublicAccessBlock = new Aws.S3.BucketPublicAccessBlock("example", new()
* {
* Bucket = example.Id,
* BlockPublicAcls = false,
* BlockPublicPolicy = false,
* IgnorePublicAcls = false,
* RestrictPublicBuckets = false,
* });
* var exampleBucketAclV2 = new Aws.S3.BucketAclV2("example", new()
* {
* Bucket = example.Id,
* Acl = "public-read",
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleBucketOwnershipControls,
* exampleBucketPublicAccessBlock,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-example-bucket"),
* })
* if err != nil {
* return err
* }
* exampleBucketOwnershipControls, err := s3.NewBucketOwnershipControls(ctx, "example", &s3.BucketOwnershipControlsArgs{
* Bucket: example.ID(),
* Rule: &s3.BucketOwnershipControlsRuleArgs{
* ObjectOwnership: pulumi.String("BucketOwnerPreferred"),
* },
* })
* if err != nil {
* return err
* }
* exampleBucketPublicAccessBlock, err := s3.NewBucketPublicAccessBlock(ctx, "example", &s3.BucketPublicAccessBlockArgs{
* Bucket: example.ID(),
* BlockPublicAcls: pulumi.Bool(false),
* BlockPublicPolicy: pulumi.Bool(false),
* IgnorePublicAcls: pulumi.Bool(false),
* RestrictPublicBuckets: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketAclV2(ctx, "example", &s3.BucketAclV2Args{
* Bucket: example.ID(),
* Acl: pulumi.String("public-read"),
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleBucketOwnershipControls,
* exampleBucketPublicAccessBlock,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketOwnershipControls;
* import com.pulumi.aws.s3.BucketOwnershipControlsArgs;
* import com.pulumi.aws.s3.inputs.BucketOwnershipControlsRuleArgs;
* import com.pulumi.aws.s3.BucketPublicAccessBlock;
* import com.pulumi.aws.s3.BucketPublicAccessBlockArgs;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("my-tf-example-bucket")
* .build());
* var exampleBucketOwnershipControls = new BucketOwnershipControls("exampleBucketOwnershipControls", BucketOwnershipControlsArgs.builder()
* .bucket(example.id())
* .rule(BucketOwnershipControlsRuleArgs.builder()
* .objectOwnership("BucketOwnerPreferred")
* .build())
* .build());
* var exampleBucketPublicAccessBlock = new BucketPublicAccessBlock("exampleBucketPublicAccessBlock", BucketPublicAccessBlockArgs.builder()
* .bucket(example.id())
* .blockPublicAcls(false)
* .blockPublicPolicy(false)
* .ignorePublicAcls(false)
* .restrictPublicBuckets(false)
* .build());
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(example.id())
* .acl("public-read")
* .build(), CustomResourceOptions.builder()
* .dependsOn(
* exampleBucketOwnershipControls,
* exampleBucketPublicAccessBlock)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-example-bucket
* exampleBucketOwnershipControls:
* type: aws:s3:BucketOwnershipControls
* name: example
* properties:
* bucket: ${example.id}
* rule:
* objectOwnership: BucketOwnerPreferred
* exampleBucketPublicAccessBlock:
* type: aws:s3:BucketPublicAccessBlock
* name: example
* properties:
* bucket: ${example.id}
* blockPublicAcls: false
* blockPublicPolicy: false
* ignorePublicAcls: false
* restrictPublicBuckets: false
* exampleBucketAclV2:
* type: aws:s3:BucketAclV2
* name: example
* properties:
* bucket: ${example.id}
* acl: public-read
* options:
* dependson:
* - ${exampleBucketOwnershipControls}
* - ${exampleBucketPublicAccessBlock}
* ```
*
* ### With Grants
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.s3.getCanonicalUserId({});
* const example = new aws.s3.BucketV2("example", {bucket: "my-tf-example-bucket"});
* const exampleBucketOwnershipControls = new aws.s3.BucketOwnershipControls("example", {
* bucket: example.id,
* rule: {
* objectOwnership: "BucketOwnerPreferred",
* },
* });
* const exampleBucketAclV2 = new aws.s3.BucketAclV2("example", {
* bucket: example.id,
* accessControlPolicy: {
* grants: [
* {
* grantee: {
* id: current.then(current => current.id),
* type: "CanonicalUser",
* },
* permission: "READ",
* },
* {
* grantee: {
* type: "Group",
* uri: "http://acs.amazonaws.com/groups/s3/LogDelivery",
* },
* permission: "READ_ACP",
* },
* ],
* owner: {
* id: current.then(current => current.id),
* },
* },
* }, {
* dependsOn: [exampleBucketOwnershipControls],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.s3.get_canonical_user_id()
* example = aws.s3.BucketV2("example", bucket="my-tf-example-bucket")
* example_bucket_ownership_controls = aws.s3.BucketOwnershipControls("example",
* bucket=example.id,
* rule={
* "object_ownership": "BucketOwnerPreferred",
* })
* example_bucket_acl_v2 = aws.s3.BucketAclV2("example",
* bucket=example.id,
* access_control_policy={
* "grants": [
* {
* "grantee": {
* "id": current.id,
* "type": "CanonicalUser",
* },
* "permission": "READ",
* },
* {
* "grantee": {
* "type": "Group",
* "uri": "http://acs.amazonaws.com/groups/s3/LogDelivery",
* },
* "permission": "READ_ACP",
* },
* ],
* "owner": {
* "id": current.id,
* },
* },
* opts = pulumi.ResourceOptions(depends_on=[example_bucket_ownership_controls]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.S3.GetCanonicalUserId.Invoke();
* var example = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "my-tf-example-bucket",
* });
* var exampleBucketOwnershipControls = new Aws.S3.BucketOwnershipControls("example", new()
* {
* Bucket = example.Id,
* Rule = new Aws.S3.Inputs.BucketOwnershipControlsRuleArgs
* {
* ObjectOwnership = "BucketOwnerPreferred",
* },
* });
* var exampleBucketAclV2 = new Aws.S3.BucketAclV2("example", new()
* {
* Bucket = example.Id,
* AccessControlPolicy = new Aws.S3.Inputs.BucketAclV2AccessControlPolicyArgs
* {
* Grants = new[]
* {
* new Aws.S3.Inputs.BucketAclV2AccessControlPolicyGrantArgs
* {
* Grantee = new Aws.S3.Inputs.BucketAclV2AccessControlPolicyGrantGranteeArgs
* {
* Id = current.Apply(getCanonicalUserIdResult => getCanonicalUserIdResult.Id),
* Type = "CanonicalUser",
* },
* Permission = "READ",
* },
* new Aws.S3.Inputs.BucketAclV2AccessControlPolicyGrantArgs
* {
* Grantee = new Aws.S3.Inputs.BucketAclV2AccessControlPolicyGrantGranteeArgs
* {
* Type = "Group",
* Uri = "http://acs.amazonaws.com/groups/s3/LogDelivery",
* },
* Permission = "READ_ACP",
* },
* },
* Owner = new Aws.S3.Inputs.BucketAclV2AccessControlPolicyOwnerArgs
* {
* Id = current.Apply(getCanonicalUserIdResult => getCanonicalUserIdResult.Id),
* },
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleBucketOwnershipControls,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := s3.GetCanonicalUserId(ctx, map[string]interface{}{}, nil)
* if err != nil {
* return err
* }
* example, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-example-bucket"),
* })
* if err != nil {
* return err
* }
* exampleBucketOwnershipControls, err := s3.NewBucketOwnershipControls(ctx, "example", &s3.BucketOwnershipControlsArgs{
* Bucket: example.ID(),
* Rule: &s3.BucketOwnershipControlsRuleArgs{
* ObjectOwnership: pulumi.String("BucketOwnerPreferred"),
* },
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketAclV2(ctx, "example", &s3.BucketAclV2Args{
* Bucket: example.ID(),
* AccessControlPolicy: &s3.BucketAclV2AccessControlPolicyArgs{
* Grants: s3.BucketAclV2AccessControlPolicyGrantArray{
* &s3.BucketAclV2AccessControlPolicyGrantArgs{
* Grantee: &s3.BucketAclV2AccessControlPolicyGrantGranteeArgs{
* Id: pulumi.String(current.Id),
* Type: pulumi.String("CanonicalUser"),
* },
* Permission: pulumi.String("READ"),
* },
* &s3.BucketAclV2AccessControlPolicyGrantArgs{
* Grantee: &s3.BucketAclV2AccessControlPolicyGrantGranteeArgs{
* Type: pulumi.String("Group"),
* Uri: pulumi.String("http://acs.amazonaws.com/groups/s3/LogDelivery"),
* },
* Permission: pulumi.String("READ_ACP"),
* },
* },
* Owner: &s3.BucketAclV2AccessControlPolicyOwnerArgs{
* Id: pulumi.String(current.Id),
* },
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleBucketOwnershipControls,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.S3Functions;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketOwnershipControls;
* import com.pulumi.aws.s3.BucketOwnershipControlsArgs;
* import com.pulumi.aws.s3.inputs.BucketOwnershipControlsRuleArgs;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.aws.s3.inputs.BucketAclV2AccessControlPolicyArgs;
* import com.pulumi.aws.s3.inputs.BucketAclV2AccessControlPolicyOwnerArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = S3Functions.getCanonicalUserId();
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("my-tf-example-bucket")
* .build());
* var exampleBucketOwnershipControls = new BucketOwnershipControls("exampleBucketOwnershipControls", BucketOwnershipControlsArgs.builder()
* .bucket(example.id())
* .rule(BucketOwnershipControlsRuleArgs.builder()
* .objectOwnership("BucketOwnerPreferred")
* .build())
* .build());
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(example.id())
* .accessControlPolicy(BucketAclV2AccessControlPolicyArgs.builder()
* .grants(
* BucketAclV2AccessControlPolicyGrantArgs.builder()
* .grantee(BucketAclV2AccessControlPolicyGrantGranteeArgs.builder()
* .id(current.applyValue(getCanonicalUserIdResult -> getCanonicalUserIdResult.id()))
* .type("CanonicalUser")
* .build())
* .permission("READ")
* .build(),
* BucketAclV2AccessControlPolicyGrantArgs.builder()
* .grantee(BucketAclV2AccessControlPolicyGrantGranteeArgs.builder()
* .type("Group")
* .uri("http://acs.amazonaws.com/groups/s3/LogDelivery")
* .build())
* .permission("READ_ACP")
* .build())
* .owner(BucketAclV2AccessControlPolicyOwnerArgs.builder()
* .id(current.applyValue(getCanonicalUserIdResult -> getCanonicalUserIdResult.id()))
* .build())
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleBucketOwnershipControls)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-example-bucket
* exampleBucketOwnershipControls:
* type: aws:s3:BucketOwnershipControls
* name: example
* properties:
* bucket: ${example.id}
* rule:
* objectOwnership: BucketOwnerPreferred
* exampleBucketAclV2:
* type: aws:s3:BucketAclV2
* name: example
* properties:
* bucket: ${example.id}
* accessControlPolicy:
* grants:
* - grantee:
* id: ${current.id}
* type: CanonicalUser
* permission: READ
* - grantee:
* type: Group
* uri: http://acs.amazonaws.com/groups/s3/LogDelivery
* permission: READ_ACP
* owner:
* id: ${current.id}
* options:
* dependson:
* - ${exampleBucketOwnershipControls}
* variables:
* current:
* fn::invoke:
* Function: aws:s3:getCanonicalUserId
* Arguments: {}
* ```
*
* ## Import
* If the owner (account ID) of the source bucket is the _same_ account used to configure the AWS Provider, and the source bucket is __configured__ with a
* [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), import using the `bucket` and `acl` separated by a comma (`,`):
* If the owner (account ID) of the source bucket _differs_ from the account used to configure the AWS Provider, and the source bucket is __not configured__ with a [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), imported using the `bucket` and `expected_bucket_owner` separated by a comma (`,`):
* If the owner (account ID) of the source bucket _differs_ from the account used to configure the AWS Provider, and the source bucket is __configured__ with a
* [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), imported using the `bucket`, `expected_bucket_owner`, and `acl` separated by commas (`,`):
* __Using `pulumi import` to import__ using `bucket`, `expected_bucket_owner`, and/or `acl`, depending on your situation. For example:
* If the owner (account ID) of the source bucket is the _same_ account used to configure the AWS Provider, and the source bucket is __not configured__ with a
* [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), import using the `bucket`:
* ```sh
* $ pulumi import aws:s3/bucketAclV2:BucketAclV2 example bucket-name
* ```
* If the owner (account ID) of the source bucket is the _same_ account used to configure the AWS Provider, and the source bucket is __configured__ with a [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), import using the `bucket` and `acl` separated by a comma (`,`):
* ```sh
* $ pulumi import aws:s3/bucketAclV2:BucketAclV2 example bucket-name,private
* ```
* If the owner (account ID) of the source bucket _differs_ from the account used to configure the AWS Provider, and the source bucket is __not configured__ with a [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), imported using the `bucket` and `expected_bucket_owner` separated by a comma (`,`):
* ```sh
* $ pulumi import aws:s3/bucketAclV2:BucketAclV2 example bucket-name,123456789012
* ```
* If the owner (account ID) of the source bucket _differs_ from the account used to configure the AWS Provider, and the source bucket is __configured__ with a [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl) (i.e. predefined grant), imported using the `bucket`, `expected_bucket_owner`, and `acl` separated by commas (`,`):
* ```sh
* $ pulumi import aws:s3/bucketAclV2:BucketAclV2 example bucket-name,123456789012,private
* ```
* @property accessControlPolicy Configuration block that sets the ACL permissions for an object per grantee. See below.
* @property acl Specifies the Canned ACL to apply to the bucket. Valid values: `private`, `public-read`, `public-read-write`, `aws-exec-read`, `authenticated-read`, `bucket-owner-read`, `bucket-owner-full-control`, `log-delivery-write`. Full details are available on the [AWS documentation](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl).
* @property bucket Bucket to which to apply the ACL.
* @property expectedBucketOwner Account ID of the expected bucket owner.
*/
public data class BucketAclV2Args(
public val accessControlPolicy: Output? = null,
public val acl: Output? = null,
public val bucket: Output? = null,
public val expectedBucketOwner: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3.BucketAclV2Args =
com.pulumi.aws.s3.BucketAclV2Args.builder()
.accessControlPolicy(
accessControlPolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.acl(acl?.applyValue({ args0 -> args0 }))
.bucket(bucket?.applyValue({ args0 -> args0 }))
.expectedBucketOwner(expectedBucketOwner?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketAclV2Args].
*/
@PulumiTagMarker
public class BucketAclV2ArgsBuilder internal constructor() {
private var accessControlPolicy: Output? = null
private var acl: Output? = null
private var bucket: Output? = null
private var expectedBucketOwner: Output? = null
/**
* @param value Configuration block that sets the ACL permissions for an object per grantee. See below.
*/
@JvmName("nuonmtidjyrsmoto")
public suspend fun accessControlPolicy(`value`: Output) {
this.accessControlPolicy = value
}
/**
* @param value Specifies the Canned ACL to apply to the bucket. Valid values: `private`, `public-read`, `public-read-write`, `aws-exec-read`, `authenticated-read`, `bucket-owner-read`, `bucket-owner-full-control`, `log-delivery-write`. Full details are available on the [AWS documentation](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl).
*/
@JvmName("fwmiivquprdmnojh")
public suspend fun acl(`value`: Output) {
this.acl = value
}
/**
* @param value Bucket to which to apply the ACL.
*/
@JvmName("wfuuofgchffltxbr")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Account ID of the expected bucket owner.
*/
@JvmName("cynhbfhgwntpclwh")
public suspend fun expectedBucketOwner(`value`: Output) {
this.expectedBucketOwner = value
}
/**
* @param value Configuration block that sets the ACL permissions for an object per grantee. See below.
*/
@JvmName("kuswtvggfnuogklj")
public suspend fun accessControlPolicy(`value`: BucketAclV2AccessControlPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessControlPolicy = mapped
}
/**
* @param argument Configuration block that sets the ACL permissions for an object per grantee. See below.
*/
@JvmName("qructdltnitcsonq")
public suspend fun accessControlPolicy(argument: suspend BucketAclV2AccessControlPolicyArgsBuilder.() -> Unit) {
val toBeMapped = BucketAclV2AccessControlPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.accessControlPolicy = mapped
}
/**
* @param value Specifies the Canned ACL to apply to the bucket. Valid values: `private`, `public-read`, `public-read-write`, `aws-exec-read`, `authenticated-read`, `bucket-owner-read`, `bucket-owner-full-control`, `log-delivery-write`. Full details are available on the [AWS documentation](https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl).
*/
@JvmName("moruwrgmmtkhvrrl")
public suspend fun acl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acl = mapped
}
/**
* @param value Bucket to which to apply the ACL.
*/
@JvmName("ysuftndlkkfiynbg")
public suspend fun bucket(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bucket = mapped
}
/**
* @param value Account ID of the expected bucket owner.
*/
@JvmName("dowvlolpayfjrsfo")
public suspend fun expectedBucketOwner(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expectedBucketOwner = mapped
}
internal fun build(): BucketAclV2Args = BucketAclV2Args(
accessControlPolicy = accessControlPolicy,
acl = acl,
bucket = bucket,
expectedBucketOwner = expectedBucketOwner,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy