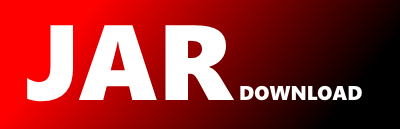
com.pulumi.aws.s3.kotlin.BucketArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin
import com.pulumi.aws.s3.BucketArgs.builder
import com.pulumi.aws.s3.kotlin.enums.CannedAcl
import com.pulumi.aws.s3.kotlin.inputs.BucketCorsRuleArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketCorsRuleArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketGrantArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketGrantArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketLifecycleRuleArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketLifecycleRuleArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketLoggingArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketLoggingArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketObjectLockConfigurationArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketObjectLockConfigurationArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketReplicationConfigurationArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketReplicationConfigurationArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketServerSideEncryptionConfigurationArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketServerSideEncryptionConfigurationArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketVersioningArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketVersioningArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.BucketWebsiteArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketWebsiteArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a S3 bucket resource.
* > **NOTE:** Please use [aws.s3.BucketV2](https://www.pulumi.com/registry/packages/aws/api-docs/s3/bucketv2) instead.
* This resource is maintained for backwards compatibility only. Please see [BucketV2 Migration
* Guide](https://www.pulumi.com/registry/packages/aws/how-to-guides/bucketv2-migration/) for instructions on migrating
* existing Bucket resources to BucketV2.
* ## Example Usage
* ### Private Bucket w/ Tags
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const b = new aws.s3.Bucket("b", {
* bucket: "my-tf-test-bucket",
* acl: aws.s3.CannedAcl.Private,
* tags: {
* Name: "My bucket",
* Environment: "Dev",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* b = aws.s3.Bucket("b",
* bucket="my-tf-test-bucket",
* acl=aws.s3.CannedAcl.PRIVATE,
* tags={
* "Name": "My bucket",
* "Environment": "Dev",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var b = new Aws.S3.Bucket("b", new()
* {
* BucketName = "my-tf-test-bucket",
* Acl = Aws.S3.CannedAcl.Private,
* Tags =
* {
* { "Name", "My bucket" },
* { "Environment", "Dev" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := s3.NewBucket(ctx, "b", &s3.BucketArgs{
* Bucket: pulumi.String("my-tf-test-bucket"),
* Acl: pulumi.String(s3.CannedAclPrivate),
* Tags: pulumi.StringMap{
* "Name": pulumi.String("My bucket"),
* "Environment": pulumi.String("Dev"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var b = new Bucket("b", BucketArgs.builder()
* .bucket("my-tf-test-bucket")
* .acl("private")
* .tags(Map.ofEntries(
* Map.entry("Name", "My bucket"),
* Map.entry("Environment", "Dev")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* b:
* type: aws:s3:Bucket
* properties:
* bucket: my-tf-test-bucket
* acl: private
* tags:
* Name: My bucket
* Environment: Dev
* ```
*
* ### Static Website Hosting
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* import * as std from "@pulumi/std";
* const b = new aws.s3.Bucket("b", {
* bucket: "s3-website-test.mydomain.com",
* acl: aws.s3.CannedAcl.PublicRead,
* policy: std.file({
* input: "policy.json",
* }).then(invoke => invoke.result),
* website: {
* indexDocument: "index.html",
* errorDocument: "error.html",
* routingRules: `[{
* "Condition": {
* "KeyPrefixEquals": "docs/"
* },
* "Redirect": {
* "ReplaceKeyPrefixWith": "documents/"
* }
* }]
* `,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* import pulumi_std as std
* b = aws.s3.Bucket("b",
* bucket="s3-website-test.mydomain.com",
* acl=aws.s3.CannedAcl.PUBLIC_READ,
* policy=std.file(input="policy.json").result,
* website={
* "index_document": "index.html",
* "error_document": "error.html",
* "routing_rules": """[{
* "Condition": {
* "KeyPrefixEquals": "docs/"
* },
* "Redirect": {
* "ReplaceKeyPrefixWith": "documents/"
* }
* }]
* """,
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var b = new Aws.S3.Bucket("b", new()
* {
* BucketName = "s3-website-test.mydomain.com",
* Acl = Aws.S3.CannedAcl.PublicRead,
* Policy = Std.File.Invoke(new()
* {
* Input = "policy.json",
* }).Apply(invoke => invoke.Result),
* Website = new Aws.S3.Inputs.BucketWebsiteArgs
* {
* IndexDocument = "index.html",
* ErrorDocument = "error.html",
* RoutingRules = @"[{
* ""Condition"": {
* ""KeyPrefixEquals"": ""docs/""
* },
* ""Redirect"": {
* ""ReplaceKeyPrefixWith"": ""documents/""
* }
* }]
* ",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* invokeFile, err := std.File(ctx, &std.FileArgs{
* Input: "policy.json",
* }, nil)
* if err != nil {
* return err
* }
* _, err = s3.NewBucket(ctx, "b", &s3.BucketArgs{
* Bucket: pulumi.String("s3-website-test.mydomain.com"),
* Acl: pulumi.String(s3.CannedAclPublicRead),
* Policy: pulumi.String(invokeFile.Result),
* Website: &s3.BucketWebsiteArgs{
* IndexDocument: pulumi.String("index.html"),
* ErrorDocument: pulumi.String("error.html"),
* RoutingRules: pulumi.Any(`[{
* "Condition": {
* "KeyPrefixEquals": "docs/"
* },
* "Redirect": {
* "ReplaceKeyPrefixWith": "documents/"
* }
* }]
* `),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketWebsiteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var b = new Bucket("b", BucketArgs.builder()
* .bucket("s3-website-test.mydomain.com")
* .acl("public-read")
* .policy(StdFunctions.file(FileArgs.builder()
* .input("policy.json")
* .build()).result())
* .website(BucketWebsiteArgs.builder()
* .indexDocument("index.html")
* .errorDocument("error.html")
* .routingRules("""
* [{
* "Condition": {
* "KeyPrefixEquals": "docs/"
* },
* "Redirect": {
* "ReplaceKeyPrefixWith": "documents/"
* }
* }]
* """)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* b:
* type: aws:s3:Bucket
* properties:
* bucket: s3-website-test.mydomain.com
* acl: public-read
* policy:
* fn::invoke:
* Function: std:file
* Arguments:
* input: policy.json
* Return: result
* website:
* indexDocument: index.html
* errorDocument: error.html
* routingRules: |
* [{
* "Condition": {
* "KeyPrefixEquals": "docs/"
* },
* "Redirect": {
* "ReplaceKeyPrefixWith": "documents/"
* }
* }]
* ```
*
* ### Using CORS
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const b = new aws.s3.Bucket("b", {
* bucket: "s3-website-test.mydomain.com",
* acl: aws.s3.CannedAcl.PublicRead,
* corsRules: [{
* allowedHeaders: ["*"],
* allowedMethods: [
* "PUT",
* "POST",
* ],
* allowedOrigins: ["https://s3-website-test.mydomain.com"],
* exposeHeaders: ["ETag"],
* maxAgeSeconds: 3000,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* b = aws.s3.Bucket("b",
* bucket="s3-website-test.mydomain.com",
* acl=aws.s3.CannedAcl.PUBLIC_READ,
* cors_rules=[{
* "allowed_headers": ["*"],
* "allowed_methods": [
* "PUT",
* "POST",
* ],
* "allowed_origins": ["https://s3-website-test.mydomain.com"],
* "expose_headers": ["ETag"],
* "max_age_seconds": 3000,
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var b = new Aws.S3.Bucket("b", new()
* {
* BucketName = "s3-website-test.mydomain.com",
* Acl = Aws.S3.CannedAcl.PublicRead,
* CorsRules = new[]
* {
* new Aws.S3.Inputs.BucketCorsRuleArgs
* {
* AllowedHeaders = new[]
* {
* "*",
* },
* AllowedMethods = new[]
* {
* "PUT",
* "POST",
* },
* AllowedOrigins = new[]
* {
* "https://s3-website-test.mydomain.com",
* },
* ExposeHeaders = new[]
* {
* "ETag",
* },
* MaxAgeSeconds = 3000,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := s3.NewBucket(ctx, "b", &s3.BucketArgs{
* Bucket: pulumi.String("s3-website-test.mydomain.com"),
* Acl: pulumi.String(s3.CannedAclPublicRead),
* CorsRules: s3.BucketCorsRuleArray{
* &s3.BucketCorsRuleArgs{
* AllowedHeaders: pulumi.StringArray{
* pulumi.String("*"),
* },
* AllowedMethods: pulumi.StringArray{
* pulumi.String("PUT"),
* pulumi.String("POST"),
* },
* AllowedOrigins: pulumi.StringArray{
* pulumi.String("https://s3-website-test.mydomain.com"),
* },
* ExposeHeaders: pulumi.StringArray{
* pulumi.String("ETag"),
* },
* MaxAgeSeconds: pulumi.Int(3000),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketCorsRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var b = new Bucket("b", BucketArgs.builder()
* .bucket("s3-website-test.mydomain.com")
* .acl("public-read")
* .corsRules(BucketCorsRuleArgs.builder()
* .allowedHeaders("*")
* .allowedMethods(
* "PUT",
* "POST")
* .allowedOrigins("https://s3-website-test.mydomain.com")
* .exposeHeaders("ETag")
* .maxAgeSeconds(3000)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* b:
* type: aws:s3:Bucket
* properties:
* bucket: s3-website-test.mydomain.com
* acl: public-read
* corsRules:
* - allowedHeaders:
* - '*'
* allowedMethods:
* - PUT
* - POST
* allowedOrigins:
* - https://s3-website-test.mydomain.com
* exposeHeaders:
* - ETag
* maxAgeSeconds: 3000
* ```
*
* ### Using versioning
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const b = new aws.s3.Bucket("b", {
* bucket: "my-tf-test-bucket",
* acl: aws.s3.CannedAcl.Private,
* versioning: {
* enabled: true,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* b = aws.s3.Bucket("b",
* bucket="my-tf-test-bucket",
* acl=aws.s3.CannedAcl.PRIVATE,
* versioning={
* "enabled": True,
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var b = new Aws.S3.Bucket("b", new()
* {
* BucketName = "my-tf-test-bucket",
* Acl = Aws.S3.CannedAcl.Private,
* Versioning = new Aws.S3.Inputs.BucketVersioningArgs
* {
* Enabled = true,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := s3.NewBucket(ctx, "b", &s3.BucketArgs{
* Bucket: pulumi.String("my-tf-test-bucket"),
* Acl: pulumi.String(s3.CannedAclPrivate),
* Versioning: &s3.BucketVersioningArgs{
* Enabled: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketVersioningArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var b = new Bucket("b", BucketArgs.builder()
* .bucket("my-tf-test-bucket")
* .acl("private")
* .versioning(BucketVersioningArgs.builder()
* .enabled(true)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* b:
* type: aws:s3:Bucket
* properties:
* bucket: my-tf-test-bucket
* acl: private
* versioning:
* enabled: true
* ```
*
* ### Enable Logging
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const logBucket = new aws.s3.Bucket("log_bucket", {
* bucket: "my-tf-log-bucket",
* acl: aws.s3.CannedAcl.LogDeliveryWrite,
* });
* const b = new aws.s3.Bucket("b", {
* bucket: "my-tf-test-bucket",
* acl: aws.s3.CannedAcl.Private,
* loggings: [{
* targetBucket: logBucket.id,
* targetPrefix: "log/",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* log_bucket = aws.s3.Bucket("log_bucket",
* bucket="my-tf-log-bucket",
* acl=aws.s3.CannedAcl.LOG_DELIVERY_WRITE)
* b = aws.s3.Bucket("b",
* bucket="my-tf-test-bucket",
* acl=aws.s3.CannedAcl.PRIVATE,
* loggings=[{
* "target_bucket": log_bucket.id,
* "target_prefix": "log/",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var logBucket = new Aws.S3.Bucket("log_bucket", new()
* {
* BucketName = "my-tf-log-bucket",
* Acl = Aws.S3.CannedAcl.LogDeliveryWrite,
* });
* var b = new Aws.S3.Bucket("b", new()
* {
* BucketName = "my-tf-test-bucket",
* Acl = Aws.S3.CannedAcl.Private,
* Loggings = new[]
* {
* new Aws.S3.Inputs.BucketLoggingArgs
* {
* TargetBucket = logBucket.Id,
* TargetPrefix = "log/",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* logBucket, err := s3.NewBucket(ctx, "log_bucket", &s3.BucketArgs{
* Bucket: pulumi.String("my-tf-log-bucket"),
* Acl: pulumi.String(s3.CannedAclLogDeliveryWrite),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucket(ctx, "b", &s3.BucketArgs{
* Bucket: pulumi.String("my-tf-test-bucket"),
* Acl: pulumi.String(s3.CannedAclPrivate),
* Loggings: s3.BucketLoggingArray{
* &s3.BucketLoggingArgs{
* TargetBucket: logBucket.ID(),
* TargetPrefix: pulumi.String("log/"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketLoggingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var logBucket = new Bucket("logBucket", BucketArgs.builder()
* .bucket("my-tf-log-bucket")
* .acl("log-delivery-write")
* .build());
* var b = new Bucket("b", BucketArgs.builder()
* .bucket("my-tf-test-bucket")
* .acl("private")
* .loggings(BucketLoggingArgs.builder()
* .targetBucket(logBucket.id())
* .targetPrefix("log/")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* logBucket:
* type: aws:s3:Bucket
* name: log_bucket
* properties:
* bucket: my-tf-log-bucket
* acl: log-delivery-write
* b:
* type: aws:s3:Bucket
* properties:
* bucket: my-tf-test-bucket
* acl: private
* loggings:
* - targetBucket: ${logBucket.id}
* targetPrefix: log/
* ```
*
* ### Using object lifecycle
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const bucket = new aws.s3.Bucket("bucket", {
* bucket: "my-bucket",
* acl: aws.s3.CannedAcl.Private,
* lifecycleRules: [
* {
* id: "log",
* enabled: true,
* prefix: "log/",
* tags: {
* rule: "log",
* autoclean: "true",
* },
* transitions: [
* {
* days: 30,
* storageClass: "STANDARD_IA",
* },
* {
* days: 60,
* storageClass: "GLACIER",
* },
* ],
* expiration: {
* days: 90,
* },
* },
* {
* id: "tmp",
* prefix: "tmp/",
* enabled: true,
* expiration: {
* date: "2016-01-12",
* },
* },
* ],
* });
* const versioningBucket = new aws.s3.Bucket("versioning_bucket", {
* bucket: "my-versioning-bucket",
* acl: aws.s3.CannedAcl.Private,
* versioning: {
* enabled: true,
* },
* lifecycleRules: [{
* prefix: "config/",
* enabled: true,
* noncurrentVersionTransitions: [
* {
* days: 30,
* storageClass: "STANDARD_IA",
* },
* {
* days: 60,
* storageClass: "GLACIER",
* },
* ],
* noncurrentVersionExpiration: {
* days: 90,
* },
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* bucket = aws.s3.Bucket("bucket",
* bucket="my-bucket",
* acl=aws.s3.CannedAcl.PRIVATE,
* lifecycle_rules=[
* {
* "id": "log",
* "enabled": True,
* "prefix": "log/",
* "tags": {
* "rule": "log",
* "autoclean": "true",
* },
* "transitions": [
* {
* "days": 30,
* "storage_class": "STANDARD_IA",
* },
* {
* "days": 60,
* "storage_class": "GLACIER",
* },
* ],
* "expiration": {
* "days": 90,
* },
* },
* {
* "id": "tmp",
* "prefix": "tmp/",
* "enabled": True,
* "expiration": {
* "date": "2016-01-12",
* },
* },
* ])
* versioning_bucket = aws.s3.Bucket("versioning_bucket",
* bucket="my-versioning-bucket",
* acl=aws.s3.CannedAcl.PRIVATE,
* versioning={
* "enabled": True,
* },
* lifecycle_rules=[{
* "prefix": "config/",
* "enabled": True,
* "noncurrent_version_transitions": [
* {
* "days": 30,
* "storage_class": "STANDARD_IA",
* },
* {
* "days": 60,
* "storage_class": "GLACIER",
* },
* ],
* "noncurrent_version_expiration": {
* "days": 90,
* },
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var bucket = new Aws.S3.Bucket("bucket", new()
* {
* BucketName = "my-bucket",
* Acl = Aws.S3.CannedAcl.Private,
* LifecycleRules = new[]
* {
* new Aws.S3.Inputs.BucketLifecycleRuleArgs
* {
* Id = "log",
* Enabled = true,
* Prefix = "log/",
* Tags =
* {
* { "rule", "log" },
* { "autoclean", "true" },
* },
* Transitions = new[]
* {
* new Aws.S3.Inputs.BucketLifecycleRuleTransitionArgs
* {
* Days = 30,
* StorageClass = "STANDARD_IA",
* },
* new Aws.S3.Inputs.BucketLifecycleRuleTransitionArgs
* {
* Days = 60,
* StorageClass = "GLACIER",
* },
* },
* Expiration = new Aws.S3.Inputs.BucketLifecycleRuleExpirationArgs
* {
* Days = 90,
* },
* },
* new Aws.S3.Inputs.BucketLifecycleRuleArgs
* {
* Id = "tmp",
* Prefix = "tmp/",
* Enabled = true,
* Expiration = new Aws.S3.Inputs.BucketLifecycleRuleExpirationArgs
* {
* Date = "2016-01-12",
* },
* },
* },
* });
* var versioningBucket = new Aws.S3.Bucket("versioning_bucket", new()
* {
* BucketName = "my-versioning-bucket",
* Acl = Aws.S3.CannedAcl.Private,
* Versioning = new Aws.S3.Inputs.BucketVersioningArgs
* {
* Enabled = true,
* },
* LifecycleRules = new[]
* {
* new Aws.S3.Inputs.BucketLifecycleRuleArgs
* {
* Prefix = "config/",
* Enabled = true,
* NoncurrentVersionTransitions = new[]
* {
* new Aws.S3.Inputs.BucketLifecycleRuleNoncurrentVersionTransitionArgs
* {
* Days = 30,
* StorageClass = "STANDARD_IA",
* },
* new Aws.S3.Inputs.BucketLifecycleRuleNoncurrentVersionTransitionArgs
* {
* Days = 60,
* StorageClass = "GLACIER",
* },
* },
* NoncurrentVersionExpiration = new Aws.S3.Inputs.BucketLifecycleRuleNoncurrentVersionExpirationArgs
* {
* Days = 90,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := s3.NewBucket(ctx, "bucket", &s3.BucketArgs{
* Bucket: pulumi.String("my-bucket"),
* Acl: pulumi.String(s3.CannedAclPrivate),
* LifecycleRules: s3.BucketLifecycleRuleArray{
* &s3.BucketLifecycleRuleArgs{
* Id: pulumi.String("log"),
* Enabled: pulumi.Bool(true),
* Prefix: pulumi.String("log/"),
* Tags: pulumi.StringMap{
* "rule": pulumi.String("log"),
* "autoclean": pulumi.String("true"),
* },
* Transitions: s3.BucketLifecycleRuleTransitionArray{
* &s3.BucketLifecycleRuleTransitionArgs{
* Days: pulumi.Int(30),
* StorageClass: pulumi.String("STANDARD_IA"),
* },
* &s3.BucketLifecycleRuleTransitionArgs{
* Days: pulumi.Int(60),
* StorageClass: pulumi.String("GLACIER"),
* },
* },
* Expiration: &s3.BucketLifecycleRuleExpirationArgs{
* Days: pulumi.Int(90),
* },
* },
* &s3.BucketLifecycleRuleArgs{
* Id: pulumi.String("tmp"),
* Prefix: pulumi.String("tmp/"),
* Enabled: pulumi.Bool(true),
* Expiration: &s3.BucketLifecycleRuleExpirationArgs{
* Date: pulumi.String("2016-01-12"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucket(ctx, "versioning_bucket", &s3.BucketArgs{
* Bucket: pulumi.String("my-versioning-bucket"),
* Acl: pulumi.String(s3.CannedAclPrivate),
* Versioning: &s3.BucketVersioningArgs{
* Enabled: pulumi.Bool(true),
* },
* LifecycleRules: s3.BucketLifecycleRuleArray{
* &s3.BucketLifecycleRuleArgs{
* Prefix: pulumi.String("config/"),
* Enabled: pulumi.Bool(true),
* NoncurrentVersionTransitions: s3.BucketLifecycleRuleNoncurrentVersionTransitionArray{
* &s3.BucketLifecycleRuleNoncurrentVersionTransitionArgs{
* Days: pulumi.Int(30),
* StorageClass: pulumi.String("STANDARD_IA"),
* },
* &s3.BucketLifecycleRuleNoncurrentVersionTransitionArgs{
* Days: pulumi.Int(60),
* StorageClass: pulumi.String("GLACIER"),
* },
* },
* NoncurrentVersionExpiration: &s3.BucketLifecycleRuleNoncurrentVersionExpirationArgs{
* Days: pulumi.Int(90),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketLifecycleRuleArgs;
* import com.pulumi.aws.s3.inputs.BucketLifecycleRuleExpirationArgs;
* import com.pulumi.aws.s3.inputs.BucketVersioningArgs;
* import com.pulumi.aws.s3.inputs.BucketLifecycleRuleNoncurrentVersionExpirationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .bucket("my-bucket")
* .acl("private")
* .lifecycleRules(
* BucketLifecycleRuleArgs.builder()
* .id("log")
* .enabled(true)
* .prefix("log/")
* .tags(Map.ofEntries(
* Map.entry("rule", "log"),
* Map.entry("autoclean", "true")
* ))
* .transitions(
* BucketLifecycleRuleTransitionArgs.builder()
* .days(30)
* .storageClass("STANDARD_IA")
* .build(),
* BucketLifecycleRuleTransitionArgs.builder()
* .days(60)
* .storageClass("GLACIER")
* .build())
* .expiration(BucketLifecycleRuleExpirationArgs.builder()
* .days(90)
* .build())
* .build(),
* BucketLifecycleRuleArgs.builder()
* .id("tmp")
* .prefix("tmp/")
* .enabled(true)
* .expiration(BucketLifecycleRuleExpirationArgs.builder()
* .date("2016-01-12")
* .build())
* .build())
* .build());
* var versioningBucket = new Bucket("versioningBucket", BucketArgs.builder()
* .bucket("my-versioning-bucket")
* .acl("private")
* .versioning(BucketVersioningArgs.builder()
* .enabled(true)
* .build())
* .lifecycleRules(BucketLifecycleRuleArgs.builder()
* .prefix("config/")
* .enabled(true)
* .noncurrentVersionTransitions(
* BucketLifecycleRuleNoncurrentVersionTransitionArgs.builder()
* .days(30)
* .storageClass("STANDARD_IA")
* .build(),
* BucketLifecycleRuleNoncurrentVersionTransitionArgs.builder()
* .days(60)
* .storageClass("GLACIER")
* .build())
* .noncurrentVersionExpiration(BucketLifecycleRuleNoncurrentVersionExpirationArgs.builder()
* .days(90)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* bucket:
* type: aws:s3:Bucket
* properties:
* bucket: my-bucket
* acl: private
* lifecycleRules:
* - id: log
* enabled: true
* prefix: log/
* tags:
* rule: log
* autoclean: 'true'
* transitions:
* - days: 30
* storageClass: STANDARD_IA
* - days: 60
* storageClass: GLACIER
* expiration:
* days: 90
* - id: tmp
* prefix: tmp/
* enabled: true
* expiration:
* date: 2016-01-12
* versioningBucket:
* type: aws:s3:Bucket
* name: versioning_bucket
* properties:
* bucket: my-versioning-bucket
* acl: private
* versioning:
* enabled: true
* lifecycleRules:
* - prefix: config/
* enabled: true
* noncurrentVersionTransitions:
* - days: 30
* storageClass: STANDARD_IA
* - days: 60
* storageClass: GLACIER
* noncurrentVersionExpiration:
* days: 90
* ```
*
* ### Using replication configuration
* > **NOTE:** See the `aws.s3.BucketReplicationConfig` resource to support bi-directional replication configuration and additional features.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const replication = new aws.iam.Role("replication", {
* name: "tf-iam-role-replication-12345",
* assumeRolePolicy: `{
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "sts:AssumeRole",
* "Principal": {
* "Service": "s3.amazonaws.com"
* },
* "Effect": "Allow",
* "Sid": ""
* }
* ]
* }
* `,
* });
* const destination = new aws.s3.Bucket("destination", {
* bucket: "tf-test-bucket-destination-12345",
* versioning: {
* enabled: true,
* },
* });
* const source = new aws.s3.Bucket("source", {
* bucket: "tf-test-bucket-source-12345",
* acl: aws.s3.CannedAcl.Private,
* versioning: {
* enabled: true,
* },
* replicationConfiguration: {
* role: replication.arn,
* rules: [{
* id: "foobar",
* status: "Enabled",
* filter: {
* tags: {},
* },
* destination: {
* bucket: destination.arn,
* storageClass: "STANDARD",
* replicationTime: {
* status: "Enabled",
* minutes: 15,
* },
* metrics: {
* status: "Enabled",
* minutes: 15,
* },
* },
* }],
* },
* });
* const replicationPolicy = new aws.iam.Policy("replication", {
* name: "tf-iam-role-policy-replication-12345",
* policy: pulumi.interpolate`{
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": [
* "s3:GetReplicationConfiguration",
* "s3:ListBucket"
* ],
* "Effect": "Allow",
* "Resource": [
* "${source.arn}"
* ]
* },
* {
* "Action": [
* "s3:GetObjectVersionForReplication",
* "s3:GetObjectVersionAcl",
* "s3:GetObjectVersionTagging"
* ],
* "Effect": "Allow",
* "Resource": [
* "${source.arn}/*"
* ]
* },
* {
* "Action": [
* "s3:ReplicateObject",
* "s3:ReplicateDelete",
* "s3:ReplicateTags"
* ],
* "Effect": "Allow",
* "Resource": "${destination.arn}/*"
* }
* ]
* }
* `,
* });
* const replicationRolePolicyAttachment = new aws.iam.RolePolicyAttachment("replication", {
* role: replication.name,
* policyArn: replicationPolicy.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* replication = aws.iam.Role("replication",
* name="tf-iam-role-replication-12345",
* assume_role_policy="""{
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "sts:AssumeRole",
* "Principal": {
* "Service": "s3.amazonaws.com"
* },
* "Effect": "Allow",
* "Sid": ""
* }
* ]
* }
* """)
* destination = aws.s3.Bucket("destination",
* bucket="tf-test-bucket-destination-12345",
* versioning={
* "enabled": True,
* })
* source = aws.s3.Bucket("source",
* bucket="tf-test-bucket-source-12345",
* acl=aws.s3.CannedAcl.PRIVATE,
* versioning={
* "enabled": True,
* },
* replication_configuration={
* "role": replication.arn,
* "rules": [{
* "id": "foobar",
* "status": "Enabled",
* "filter": {
* "tags": {},
* },
* "destination": {
* "bucket": destination.arn,
* "storage_class": "STANDARD",
* "replication_time": {
* "status": "Enabled",
* "minutes": 15,
* },
* "metrics": {
* "status": "Enabled",
* "minutes": 15,
* },
* },
* }],
* })
* replication_policy = aws.iam.Policy("replication",
* name="tf-iam-role-policy-replication-12345",
* policy=pulumi.Output.all(
* sourceArn=source.arn,
* sourceArn1=source.arn,
* destinationArn=destination.arn
* ).apply(lambda resolved_outputs: f"""{{
* "Version": "2012-10-17",
* "Statement": [
* {{
* "Action": [
* "s3:GetReplicationConfiguration",
* "s3:ListBucket"
* ],
* "Effect": "Allow",
* "Resource": [
* "{resolved_outputs['sourceArn']}"
* ]
* }},
* {{
* "Action": [
* "s3:GetObjectVersionForReplication",
* "s3:GetObjectVersionAcl",
* "s3:GetObjectVersionTagging"
* ],
* "Effect": "Allow",
* "Resource": [
* "{resolved_outputs['sourceArn1']}/*"
* ]
* }},
* {{
* "Action": [
* "s3:ReplicateObject",
* "s3:ReplicateDelete",
* "s3:ReplicateTags"
* ],
* "Effect": "Allow",
* "Resource": "{resolved_outputs['destinationArn']}/*"
* }}
* ]
* }}
* """)
* )
* replication_role_policy_attachment = aws.iam.RolePolicyAttachment("replication",
* role=replication.name,
* policy_arn=replication_policy.arn)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var replication = new Aws.Iam.Role("replication", new()
* {
* Name = "tf-iam-role-replication-12345",
* AssumeRolePolicy = @"{
* ""Version"": ""2012-10-17"",
* ""Statement"": [
* {
* ""Action"": ""sts:AssumeRole"",
* ""Principal"": {
* ""Service"": ""s3.amazonaws.com""
* },
* ""Effect"": ""Allow"",
* ""Sid"": """"
* }
* ]
* }
* ",
* });
* var destination = new Aws.S3.Bucket("destination", new()
* {
* BucketName = "tf-test-bucket-destination-12345",
* Versioning = new Aws.S3.Inputs.BucketVersioningArgs
* {
* Enabled = true,
* },
* });
* var source = new Aws.S3.Bucket("source", new()
* {
* BucketName = "tf-test-bucket-source-12345",
* Acl = Aws.S3.CannedAcl.Private,
* Versioning = new Aws.S3.Inputs.BucketVersioningArgs
* {
* Enabled = true,
* },
* ReplicationConfiguration = new Aws.S3.Inputs.BucketReplicationConfigurationArgs
* {
* Role = replication.Arn,
* Rules = new[]
* {
* new Aws.S3.Inputs.BucketReplicationConfigurationRuleArgs
* {
* Id = "foobar",
* Status = "Enabled",
* Filter = new Aws.S3.Inputs.BucketReplicationConfigurationRuleFilterArgs
* {
* Tags = null,
* },
* Destination = new Aws.S3.Inputs.BucketReplicationConfigurationRuleDestinationArgs
* {
* Bucket = destination.Arn,
* StorageClass = "STANDARD",
* ReplicationTime = new Aws.S3.Inputs.BucketReplicationConfigurationRuleDestinationReplicationTimeArgs
* {
* Status = "Enabled",
* Minutes = 15,
* },
* Metrics = new Aws.S3.Inputs.BucketReplicationConfigurationRuleDestinationMetricsArgs
* {
* Status = "Enabled",
* Minutes = 15,
* },
* },
* },
* },
* },
* });
* var replicationPolicy = new Aws.Iam.Policy("replication", new()
* {
* Name = "tf-iam-role-policy-replication-12345",
* PolicyDocument = Output.Tuple(source.Arn, source.Arn, destination.Arn).Apply(values =>
* {
* var sourceArn = values.Item1;
* var sourceArn1 = values.Item2;
* var destinationArn = values.Item3;
* return @$"{{
* ""Version"": ""2012-10-17"",
* ""Statement"": [
* {{
* ""Action"": [
* ""s3:GetReplicationConfiguration"",
* ""s3:ListBucket""
* ],
* ""Effect"": ""Allow"",
* ""Resource"": [
* ""{sourceArn}""
* ]
* }},
* {{
* ""Action"": [
* ""s3:GetObjectVersionForReplication"",
* ""s3:GetObjectVersionAcl"",
* ""s3:GetObjectVersionTagging""
* ],
* ""Effect"": ""Allow"",
* ""Resource"": [
* ""{sourceArn1}/*""
* ]
* }},
* {{
* ""Action"": [
* ""s3:ReplicateObject"",
* ""s3:ReplicateDelete"",
* ""s3:ReplicateTags""
* ],
* ""Effect"": ""Allow"",
* ""Resource"": ""{destinationArn}/*""
* }}
* ]
* }}
* ";
* }),
* });
* var replicationRolePolicyAttachment = new Aws.Iam.RolePolicyAttachment("replication", new()
* {
* Role = replication.Name,
* PolicyArn = replicationPolicy.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* replication, err := iam.NewRole(ctx, "replication", &iam.RoleArgs{
* Name: pulumi.String("tf-iam-role-replication-12345"),
* AssumeRolePolicy: pulumi.Any(`{
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "sts:AssumeRole",
* "Principal": {
* "Service": "s3.amazonaws.com"
* },
* "Effect": "Allow",
* "Sid": ""
* }
* ]
* }
* `),
* })
* if err != nil {
* return err
* }
* destination, err := s3.NewBucket(ctx, "destination", &s3.BucketArgs{
* Bucket: pulumi.String("tf-test-bucket-destination-12345"),
* Versioning: &s3.BucketVersioningArgs{
* Enabled: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* source, err := s3.NewBucket(ctx, "source", &s3.BucketArgs{
* Bucket: pulumi.String("tf-test-bucket-source-12345"),
* Acl: pulumi.String(s3.CannedAclPrivate),
* Versioning: &s3.BucketVersioningArgs{
* Enabled: pulumi.Bool(true),
* },
* ReplicationConfiguration: &s3.BucketReplicationConfigurationArgs{
* Role: replication.Arn,
* Rules: s3.BucketReplicationConfigurationRuleArray{
* &s3.BucketReplicationConfigurationRuleArgs{
* Id: pulumi.String("foobar"),
* Status: pulumi.String("Enabled"),
* Filter: &s3.BucketReplicationConfigurationRuleFilterArgs{
* Tags: pulumi.StringMap{},
* },
* Destination: &s3.BucketReplicationConfigurationRuleDestinationArgs{
* Bucket: destination.Arn,
* StorageClass: pulumi.String("STANDARD"),
* ReplicationTime: &s3.BucketReplicationConfigurationRuleDestinationReplicationTimeArgs{
* Status: pulumi.String("Enabled"),
* Minutes: pulumi.Int(15),
* },
* Metrics: &s3.BucketReplicationConfigurationRuleDestinationMetricsArgs{
* Status: pulumi.String("Enabled"),
* Minutes: pulumi.Int(15),
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* replicationPolicy, err := iam.NewPolicy(ctx, "replication", &iam.PolicyArgs{
* Name: pulumi.String("tf-iam-role-policy-replication-12345"),
* Policy: pulumi.All(source.Arn, source.Arn, destination.Arn).ApplyT(func(_args []interface{}) (string, error) {
* sourceArn := _args[0].(string)
* sourceArn1 := _args[1].(string)
* destinationArn := _args[2].(string)
* return fmt.Sprintf(`{
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": [
* "s3:GetReplicationConfiguration",
* "s3:ListBucket"
* ],
* "Effect": "Allow",
* "Resource": [
* "%v"
* ]
* },
* {
* "Action": [
* "s3:GetObjectVersionForReplication",
* "s3:GetObjectVersionAcl",
* "s3:GetObjectVersionTagging"
* ],
* "Effect": "Allow",
* "Resource": [
* "%v/*"
* ]
* },
* {
* "Action": [
* "s3:ReplicateObject",
* "s3:ReplicateDelete",
* "s3:ReplicateTags"
* ],
* "Effect": "Allow",
* "Resource": "%v/*"
* }
* ]
* }
* `, sourceArn, sourceArn1, destinationArn), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* _, err = iam.NewRolePolicyAttachment(ctx, "replication", &iam.RolePolicyAttachmentArgs{
* Role: replication.Name,
* PolicyArn: replicationPolicy.Arn,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketVersioningArgs;
* import com.pulumi.aws.s3.inputs.BucketReplicationConfigurationArgs;
* import com.pulumi.aws.iam.Policy;
* import com.pulumi.aws.iam.PolicyArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var replication = new Role("replication", RoleArgs.builder()
* .name("tf-iam-role-replication-12345")
* .assumeRolePolicy("""
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "sts:AssumeRole",
* "Principal": {
* "Service": "s3.amazonaws.com"
* },
* "Effect": "Allow",
* "Sid": ""
* }
* ]
* }
* """)
* .build());
* var destination = new Bucket("destination", BucketArgs.builder()
* .bucket("tf-test-bucket-destination-12345")
* .versioning(BucketVersioningArgs.builder()
* .enabled(true)
* .build())
* .build());
* var source = new Bucket("source", BucketArgs.builder()
* .bucket("tf-test-bucket-source-12345")
* .acl("private")
* .versioning(BucketVersioningArgs.builder()
* .enabled(true)
* .build())
* .replicationConfiguration(BucketReplicationConfigurationArgs.builder()
* .role(replication.arn())
* .rules(BucketReplicationConfigurationRuleArgs.builder()
* .id("foobar")
* .status("Enabled")
* .filter(BucketReplicationConfigurationRuleFilterArgs.builder()
* .tags()
* .build())
* .destination(BucketReplicationConfigurationRuleDestinationArgs.builder()
* .bucket(destination.arn())
* .storageClass("STANDARD")
* .replicationTime(BucketReplicationConfigurationRuleDestinationReplicationTimeArgs.builder()
* .status("Enabled")
* .minutes(15)
* .build())
* .metrics(BucketReplicationConfigurationRuleDestinationMetricsArgs.builder()
* .status("Enabled")
* .minutes(15)
* .build())
* .build())
* .build())
* .build())
* .build());
* var replicationPolicy = new Policy("replicationPolicy", PolicyArgs.builder()
* .name("tf-iam-role-policy-replication-12345")
* .policy(Output.tuple(source.arn(), source.arn(), destination.arn()).applyValue(values -> {
* var sourceArn = values.t1;
* var sourceArn1 = values.t2;
* var destinationArn = values.t3;
* return """
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": [
* "s3:GetReplicationConfiguration",
* "s3:ListBucket"
* ],
* "Effect": "Allow",
* "Resource": [
* "%s"
* ]
* },
* {
* "Action": [
* "s3:GetObjectVersionForReplication",
* "s3:GetObjectVersionAcl",
* "s3:GetObjectVersionTagging"
* ],
* "Effect": "Allow",
* "Resource": [
* "%s/*"
* ]
* },
* {
* "Action": [
* "s3:ReplicateObject",
* "s3:ReplicateDelete",
* "s3:ReplicateTags"
* ],
* "Effect": "Allow",
* "Resource": "%s/*"
* }
* ]
* }
* ", sourceArn,sourceArn1,destinationArn);
* }))
* .build());
* var replicationRolePolicyAttachment = new RolePolicyAttachment("replicationRolePolicyAttachment", RolePolicyAttachmentArgs.builder()
* .role(replication.name())
* .policyArn(replicationPolicy.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* replication:
* type: aws:iam:Role
* properties:
* name: tf-iam-role-replication-12345
* assumeRolePolicy: |
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "sts:AssumeRole",
* "Principal": {
* "Service": "s3.amazonaws.com"
* },
* "Effect": "Allow",
* "Sid": ""
* }
* ]
* }
* replicationPolicy:
* type: aws:iam:Policy
* name: replication
* properties:
* name: tf-iam-role-policy-replication-12345
* policy: |
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": [
* "s3:GetReplicationConfiguration",
* "s3:ListBucket"
* ],
* "Effect": "Allow",
* "Resource": [
* "${source.arn}"
* ]
* },
* {
* "Action": [
* "s3:GetObjectVersionForReplication",
* "s3:GetObjectVersionAcl",
* "s3:GetObjectVersionTagging"
* ],
* "Effect": "Allow",
* "Resource": [
* "${source.arn}/*"
* ]
* },
* {
* "Action": [
* "s3:ReplicateObject",
* "s3:ReplicateDelete",
* "s3:ReplicateTags"
* ],
* "Effect": "Allow",
* "Resource": "${destination.arn}/*"
* }
* ]
* }
* replicationRolePolicyAttachment:
* type: aws:iam:RolePolicyAttachment
* name: replication
* properties:
* role: ${replication.name}
* policyArn: ${replicationPolicy.arn}
* destination:
* type: aws:s3:Bucket
* properties:
* bucket: tf-test-bucket-destination-12345
* versioning:
* enabled: true
* source:
* type: aws:s3:Bucket
* properties:
* bucket: tf-test-bucket-source-12345
* acl: private
* versioning:
* enabled: true
* replicationConfiguration:
* role: ${replication.arn}
* rules:
* - id: foobar
* status: Enabled
* filter:
* tags: {}
* destination:
* bucket: ${destination.arn}
* storageClass: STANDARD
* replicationTime:
* status: Enabled
* minutes: 15
* metrics:
* status: Enabled
* minutes: 15
* ```
*
* ### Enable Default Server Side Encryption
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const mykey = new aws.kms.Key("mykey", {
* description: "This key is used to encrypt bucket objects",
* deletionWindowInDays: 10,
* });
* const mybucket = new aws.s3.Bucket("mybucket", {
* bucket: "mybucket",
* serverSideEncryptionConfiguration: {
* rule: {
* applyServerSideEncryptionByDefault: {
* kmsMasterKeyId: mykey.arn,
* sseAlgorithm: "aws:kms",
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* mykey = aws.kms.Key("mykey",
* description="This key is used to encrypt bucket objects",
* deletion_window_in_days=10)
* mybucket = aws.s3.Bucket("mybucket",
* bucket="mybucket",
* server_side_encryption_configuration={
* "rule": {
* "apply_server_side_encryption_by_default": {
* "kms_master_key_id": mykey.arn,
* "sse_algorithm": "aws:kms",
* },
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var mykey = new Aws.Kms.Key("mykey", new()
* {
* Description = "This key is used to encrypt bucket objects",
* DeletionWindowInDays = 10,
* });
* var mybucket = new Aws.S3.Bucket("mybucket", new()
* {
* BucketName = "mybucket",
* ServerSideEncryptionConfiguration = new Aws.S3.Inputs.BucketServerSideEncryptionConfigurationArgs
* {
* Rule = new Aws.S3.Inputs.BucketServerSideEncryptionConfigurationRuleArgs
* {
* ApplyServerSideEncryptionByDefault = new Aws.S3.Inputs.BucketServerSideEncryptionConfigurationRuleApplyServerSideEncryptionByDefaultArgs
* {
* KmsMasterKeyId = mykey.Arn,
* SseAlgorithm = "aws:kms",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* mykey, err := kms.NewKey(ctx, "mykey", &kms.KeyArgs{
* Description: pulumi.String("This key is used to encrypt bucket objects"),
* DeletionWindowInDays: pulumi.Int(10),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucket(ctx, "mybucket", &s3.BucketArgs{
* Bucket: pulumi.String("mybucket"),
* ServerSideEncryptionConfiguration: &s3.BucketServerSideEncryptionConfigurationArgs{
* Rule: &s3.BucketServerSideEncryptionConfigurationRuleArgs{
* ApplyServerSideEncryptionByDefault: &s3.BucketServerSideEncryptionConfigurationRuleApplyServerSideEncryptionByDefaultArgs{
* KmsMasterKeyId: mykey.Arn,
* SseAlgorithm: pulumi.String("aws:kms"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketServerSideEncryptionConfigurationArgs;
* import com.pulumi.aws.s3.inputs.BucketServerSideEncryptionConfigurationRuleArgs;
* import com.pulumi.aws.s3.inputs.BucketServerSideEncryptionConfigurationRuleApplyServerSideEncryptionByDefaultArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var mykey = new Key("mykey", KeyArgs.builder()
* .description("This key is used to encrypt bucket objects")
* .deletionWindowInDays(10)
* .build());
* var mybucket = new Bucket("mybucket", BucketArgs.builder()
* .bucket("mybucket")
* .serverSideEncryptionConfiguration(BucketServerSideEncryptionConfigurationArgs.builder()
* .rule(BucketServerSideEncryptionConfigurationRuleArgs.builder()
* .applyServerSideEncryptionByDefault(BucketServerSideEncryptionConfigurationRuleApplyServerSideEncryptionByDefaultArgs.builder()
* .kmsMasterKeyId(mykey.arn())
* .sseAlgorithm("aws:kms")
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* mykey:
* type: aws:kms:Key
* properties:
* description: This key is used to encrypt bucket objects
* deletionWindowInDays: 10
* mybucket:
* type: aws:s3:Bucket
* properties:
* bucket: mybucket
* serverSideEncryptionConfiguration:
* rule:
* applyServerSideEncryptionByDefault:
* kmsMasterKeyId: ${mykey.arn}
* sseAlgorithm: aws:kms
* ```
*
* ### Using ACL policy grants
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const currentUser = aws.s3.getCanonicalUserId({});
* const bucket = new aws.s3.Bucket("bucket", {
* bucket: "mybucket",
* grants: [
* {
* id: currentUser.then(currentUser => currentUser.id),
* type: "CanonicalUser",
* permissions: ["FULL_CONTROL"],
* },
* {
* type: "Group",
* permissions: [
* "READ_ACP",
* "WRITE",
* ],
* uri: "http://acs.amazonaws.com/groups/s3/LogDelivery",
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current_user = aws.s3.get_canonical_user_id()
* bucket = aws.s3.Bucket("bucket",
* bucket="mybucket",
* grants=[
* {
* "id": current_user.id,
* "type": "CanonicalUser",
* "permissions": ["FULL_CONTROL"],
* },
* {
* "type": "Group",
* "permissions": [
* "READ_ACP",
* "WRITE",
* ],
* "uri": "http://acs.amazonaws.com/groups/s3/LogDelivery",
* },
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var currentUser = Aws.S3.GetCanonicalUserId.Invoke();
* var bucket = new Aws.S3.Bucket("bucket", new()
* {
* BucketName = "mybucket",
* Grants = new[]
* {
* new Aws.S3.Inputs.BucketGrantArgs
* {
* Id = currentUser.Apply(getCanonicalUserIdResult => getCanonicalUserIdResult.Id),
* Type = "CanonicalUser",
* Permissions = new[]
* {
* "FULL_CONTROL",
* },
* },
* new Aws.S3.Inputs.BucketGrantArgs
* {
* Type = "Group",
* Permissions = new[]
* {
* "READ_ACP",
* "WRITE",
* },
* Uri = "http://acs.amazonaws.com/groups/s3/LogDelivery",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* currentUser, err := s3.GetCanonicalUserId(ctx, map[string]interface{}{}, nil)
* if err != nil {
* return err
* }
* _, err = s3.NewBucket(ctx, "bucket", &s3.BucketArgs{
* Bucket: pulumi.String("mybucket"),
* Grants: s3.BucketGrantArray{
* &s3.BucketGrantArgs{
* Id: pulumi.String(currentUser.Id),
* Type: pulumi.String("CanonicalUser"),
* Permissions: pulumi.StringArray{
* pulumi.String("FULL_CONTROL"),
* },
* },
* &s3.BucketGrantArgs{
* Type: pulumi.String("Group"),
* Permissions: pulumi.StringArray{
* pulumi.String("READ_ACP"),
* pulumi.String("WRITE"),
* },
* Uri: pulumi.String("http://acs.amazonaws.com/groups/s3/LogDelivery"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.S3Functions;
* import com.pulumi.aws.s3.Bucket;
* import com.pulumi.aws.s3.BucketArgs;
* import com.pulumi.aws.s3.inputs.BucketGrantArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var currentUser = S3Functions.getCanonicalUserId();
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .bucket("mybucket")
* .grants(
* BucketGrantArgs.builder()
* .id(currentUser.applyValue(getCanonicalUserIdResult -> getCanonicalUserIdResult.id()))
* .type("CanonicalUser")
* .permissions("FULL_CONTROL")
* .build(),
* BucketGrantArgs.builder()
* .type("Group")
* .permissions(
* "READ_ACP",
* "WRITE")
* .uri("http://acs.amazonaws.com/groups/s3/LogDelivery")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* bucket:
* type: aws:s3:Bucket
* properties:
* bucket: mybucket
* grants:
* - id: ${currentUser.id}
* type: CanonicalUser
* permissions:
* - FULL_CONTROL
* - type: Group
* permissions:
* - READ_ACP
* - WRITE
* uri: http://acs.amazonaws.com/groups/s3/LogDelivery
* variables:
* currentUser:
* fn::invoke:
* Function: aws:s3:getCanonicalUserId
* Arguments: {}
* ```
*
* ## Import
* S3 bucket can be imported using the `bucket`, e.g.,
* ```sh
* $ pulumi import aws:s3/bucket:Bucket bucket bucket-name
* ```
* The `policy` argument is not imported and will be deprecated in a future version of the provider. Use the `aws_s3_bucket_policy` resource to manage the S3 Bucket Policy instead.
* @property accelerationStatus Sets the accelerate configuration of an existing bucket. Can be `Enabled` or `Suspended`.
* @property acl The [canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/dev/acl-overview.html#canned-acl) to apply. Valid values are `private`, `public-read`, `public-read-write`, `aws-exec-read`, `authenticated-read`, and `log-delivery-write`. Defaults to `private`. Conflicts with `grant`.
* @property arn The ARN of the bucket. Will be of format `arn:aws:s3:::bucketname`.
* @property bucket The name of the bucket. If omitted, this provider will assign a random, unique name. Must be lowercase and less than or equal to 63 characters in length. A full list of bucket naming rules [may be found here](https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html).
* @property bucketPrefix Creates a unique bucket name beginning with the specified prefix. Conflicts with `bucket`. Must be lowercase and less than or equal to 37 characters in length. A full list of bucket naming rules [may be found here](https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html).
* @property corsRules A rule of [Cross-Origin Resource Sharing](https://docs.aws.amazon.com/AmazonS3/latest/dev/cors.html) (documented below).
* @property forceDestroy A boolean that indicates all objects (including any [locked objects](https://docs.aws.amazon.com/AmazonS3/latest/dev/object-lock-overview.html)) should be deleted from the bucket so that the bucket can be destroyed without error. These objects are *not* recoverable.
* @property grants An [ACL policy grant](https://docs.aws.amazon.com/AmazonS3/latest/dev/acl-overview.html#sample-acl) (documented below). Conflicts with `acl`.
* @property hostedZoneId The [Route 53 Hosted Zone ID](https://docs.aws.amazon.com/general/latest/gr/rande.html#s3_website_region_endpoints) for this bucket's region.
* @property lifecycleRules A configuration of [object lifecycle management](http://docs.aws.amazon.com/AmazonS3/latest/dev/object-lifecycle-mgmt.html) (documented below).
* @property loggings A settings of [bucket logging](https://docs.aws.amazon.com/AmazonS3/latest/UG/ManagingBucketLogging.html) (documented below).
* @property objectLockConfiguration A configuration of [S3 object locking](https://docs.aws.amazon.com/AmazonS3/latest/dev/object-lock.html) (documented below)
* > **NOTE:** You cannot use `acceleration_status` in `cn-north-1` or `us-gov-west-1`
* @property policy A valid [bucket policy](https://docs.aws.amazon.com/AmazonS3/latest/dev/example-bucket-policies.html) JSON document. Note that if the policy document is not specific enough (but still valid), this provider may view the policy as constantly changing in a `pulumi preview`. In this case, please make sure you use the verbose/specific version of the policy.
* @property replicationConfiguration A configuration of [replication configuration](http://docs.aws.amazon.com/AmazonS3/latest/dev/crr.html) (documented below).
* @property requestPayer Specifies who should bear the cost of Amazon S3 data transfer.
* Can be either `BucketOwner` or `Requester`. By default, the owner of the S3 bucket would incur
* the costs of any data transfer. See [Requester Pays Buckets](http://docs.aws.amazon.com/AmazonS3/latest/dev/RequesterPaysBuckets.html)
* developer guide for more information.
* @property serverSideEncryptionConfiguration A configuration of [server-side encryption configuration](http://docs.aws.amazon.com/AmazonS3/latest/dev/bucket-encryption.html) (documented below)
* @property tags A map of tags to assign to the bucket. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property versioning A state of [versioning](https://docs.aws.amazon.com/AmazonS3/latest/dev/Versioning.html) (documented below)
* @property website A website object (documented below).
* @property websiteDomain The domain of the website endpoint, if the bucket is configured with a website. If not, this will be an empty string. This is used to create Route 53 alias records.
* @property websiteEndpoint The website endpoint, if the bucket is configured with a website. If not, this will be an empty string.
* */*/*/*/*/*/*/*/*/*/*/*/
*/
public data class BucketArgs(
public val accelerationStatus: Output? = null,
public val acl: Output>? = null,
public val arn: Output? = null,
public val bucket: Output? = null,
public val bucketPrefix: Output? = null,
public val corsRules: Output>? = null,
public val forceDestroy: Output? = null,
public val grants: Output>? = null,
public val hostedZoneId: Output? = null,
public val lifecycleRules: Output>? = null,
public val loggings: Output>? = null,
public val objectLockConfiguration: Output? = null,
public val policy: Output? = null,
public val replicationConfiguration: Output? = null,
public val requestPayer: Output? = null,
public val serverSideEncryptionConfiguration: Output? =
null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy