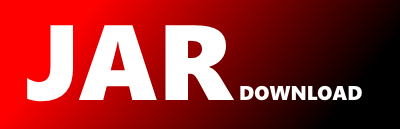
com.pulumi.aws.s3.kotlin.BucketObjectLockConfigurationV2Args.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin
import com.pulumi.aws.s3.BucketObjectLockConfigurationV2Args.builder
import com.pulumi.aws.s3.kotlin.inputs.BucketObjectLockConfigurationV2RuleArgs
import com.pulumi.aws.s3.kotlin.inputs.BucketObjectLockConfigurationV2RuleArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Provides an S3 bucket Object Lock configuration resource. For more information about Object Locking, go to [Using S3 Object Lock](https://docs.aws.amazon.com/AmazonS3/latest/userguide/object-lock.html) in the Amazon S3 User Guide.
* > This resource can be used enable Object Lock for **new** and **existing** buckets.
* > This resource cannot be used with S3 directory buckets.
* ## Example Usage
* ### Object Lock configuration for new or existing buckets
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.s3.BucketV2("example", {bucket: "mybucket"});
* const exampleBucketVersioningV2 = new aws.s3.BucketVersioningV2("example", {
* bucket: example.id,
* versioningConfiguration: {
* status: "Enabled",
* },
* });
* const exampleBucketObjectLockConfigurationV2 = new aws.s3.BucketObjectLockConfigurationV2("example", {
* bucket: example.id,
* rule: {
* defaultRetention: {
* mode: "COMPLIANCE",
* days: 5,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.s3.BucketV2("example", bucket="mybucket")
* example_bucket_versioning_v2 = aws.s3.BucketVersioningV2("example",
* bucket=example.id,
* versioning_configuration={
* "status": "Enabled",
* })
* example_bucket_object_lock_configuration_v2 = aws.s3.BucketObjectLockConfigurationV2("example",
* bucket=example.id,
* rule={
* "default_retention": {
* "mode": "COMPLIANCE",
* "days": 5,
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "mybucket",
* });
* var exampleBucketVersioningV2 = new Aws.S3.BucketVersioningV2("example", new()
* {
* Bucket = example.Id,
* VersioningConfiguration = new Aws.S3.Inputs.BucketVersioningV2VersioningConfigurationArgs
* {
* Status = "Enabled",
* },
* });
* var exampleBucketObjectLockConfigurationV2 = new Aws.S3.BucketObjectLockConfigurationV2("example", new()
* {
* Bucket = example.Id,
* Rule = new Aws.S3.Inputs.BucketObjectLockConfigurationV2RuleArgs
* {
* DefaultRetention = new Aws.S3.Inputs.BucketObjectLockConfigurationV2RuleDefaultRetentionArgs
* {
* Mode = "COMPLIANCE",
* Days = 5,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("mybucket"),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketVersioningV2(ctx, "example", &s3.BucketVersioningV2Args{
* Bucket: example.ID(),
* VersioningConfiguration: &s3.BucketVersioningV2VersioningConfigurationArgs{
* Status: pulumi.String("Enabled"),
* },
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketObjectLockConfigurationV2(ctx, "example", &s3.BucketObjectLockConfigurationV2Args{
* Bucket: example.ID(),
* Rule: &s3.BucketObjectLockConfigurationV2RuleArgs{
* DefaultRetention: &s3.BucketObjectLockConfigurationV2RuleDefaultRetentionArgs{
* Mode: pulumi.String("COMPLIANCE"),
* Days: pulumi.Int(5),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketVersioningV2;
* import com.pulumi.aws.s3.BucketVersioningV2Args;
* import com.pulumi.aws.s3.inputs.BucketVersioningV2VersioningConfigurationArgs;
* import com.pulumi.aws.s3.BucketObjectLockConfigurationV2;
* import com.pulumi.aws.s3.BucketObjectLockConfigurationV2Args;
* import com.pulumi.aws.s3.inputs.BucketObjectLockConfigurationV2RuleArgs;
* import com.pulumi.aws.s3.inputs.BucketObjectLockConfigurationV2RuleDefaultRetentionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("mybucket")
* .build());
* var exampleBucketVersioningV2 = new BucketVersioningV2("exampleBucketVersioningV2", BucketVersioningV2Args.builder()
* .bucket(example.id())
* .versioningConfiguration(BucketVersioningV2VersioningConfigurationArgs.builder()
* .status("Enabled")
* .build())
* .build());
* var exampleBucketObjectLockConfigurationV2 = new BucketObjectLockConfigurationV2("exampleBucketObjectLockConfigurationV2", BucketObjectLockConfigurationV2Args.builder()
* .bucket(example.id())
* .rule(BucketObjectLockConfigurationV2RuleArgs.builder()
* .defaultRetention(BucketObjectLockConfigurationV2RuleDefaultRetentionArgs.builder()
* .mode("COMPLIANCE")
* .days(5)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:s3:BucketV2
* properties:
* bucket: mybucket
* exampleBucketVersioningV2:
* type: aws:s3:BucketVersioningV2
* name: example
* properties:
* bucket: ${example.id}
* versioningConfiguration:
* status: Enabled
* exampleBucketObjectLockConfigurationV2:
* type: aws:s3:BucketObjectLockConfigurationV2
* name: example
* properties:
* bucket: ${example.id}
* rule:
* defaultRetention:
* mode: COMPLIANCE
* days: 5
* ```
*
* ## Import
* If the owner (account ID) of the source bucket differs from the account used to configure the AWS Provider, import using the `bucket` and `expected_bucket_owner`, separated by a comma (`,`). For example:
* __Using `pulumi import`__, import an S3 bucket Object Lock Configuration using one of two forms. If the owner (account ID) of the source bucket is the same account used to configure the AWS Provider, import using the `bucket`. For example:
* ```sh
* $ pulumi import aws:s3/bucketObjectLockConfigurationV2:BucketObjectLockConfigurationV2 example bucket-name
* ```
* If the owner (account ID) of the source bucket differs from the account used to configure the AWS Provider, import using the `bucket` and `expected_bucket_owner`, separated by a comma (`,`). For example:
* ```sh
* $ pulumi import aws:s3/bucketObjectLockConfigurationV2:BucketObjectLockConfigurationV2 example bucket-name,123456789012
* ```
* @property bucket Name of the bucket.
* @property expectedBucketOwner Account ID of the expected bucket owner.
* @property objectLockEnabled Indicates whether this bucket has an Object Lock configuration enabled. Defaults to `Enabled`. Valid values: `Enabled`.
* @property rule Configuration block for specifying the Object Lock rule for the specified object. See below.
* @property token This argument is deprecated and no longer needed to enable Object Lock.
* To enable Object Lock for an existing bucket, you must first enable versioning on the bucket and then enable Object Lock. For more details on versioning, see the `aws.s3.BucketVersioningV2` resource.
*/
public data class BucketObjectLockConfigurationV2Args(
public val bucket: Output? = null,
public val expectedBucketOwner: Output? = null,
public val objectLockEnabled: Output? = null,
public val rule: Output? = null,
public val token: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3.BucketObjectLockConfigurationV2Args =
com.pulumi.aws.s3.BucketObjectLockConfigurationV2Args.builder()
.bucket(bucket?.applyValue({ args0 -> args0 }))
.expectedBucketOwner(expectedBucketOwner?.applyValue({ args0 -> args0 }))
.objectLockEnabled(objectLockEnabled?.applyValue({ args0 -> args0 }))
.rule(rule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.token(token?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketObjectLockConfigurationV2Args].
*/
@PulumiTagMarker
public class BucketObjectLockConfigurationV2ArgsBuilder internal constructor() {
private var bucket: Output? = null
private var expectedBucketOwner: Output? = null
private var objectLockEnabled: Output? = null
private var rule: Output? = null
private var token: Output? = null
/**
* @param value Name of the bucket.
*/
@JvmName("sffavjxmbkyduvqw")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Account ID of the expected bucket owner.
*/
@JvmName("txnrfndjytithcbh")
public suspend fun expectedBucketOwner(`value`: Output) {
this.expectedBucketOwner = value
}
/**
* @param value Indicates whether this bucket has an Object Lock configuration enabled. Defaults to `Enabled`. Valid values: `Enabled`.
*/
@JvmName("jstcsdlkrqlaabwq")
public suspend fun objectLockEnabled(`value`: Output) {
this.objectLockEnabled = value
}
/**
* @param value Configuration block for specifying the Object Lock rule for the specified object. See below.
*/
@JvmName("nmuehfbfklsqkpqn")
public suspend fun rule(`value`: Output) {
this.rule = value
}
/**
* @param value This argument is deprecated and no longer needed to enable Object Lock.
* To enable Object Lock for an existing bucket, you must first enable versioning on the bucket and then enable Object Lock. For more details on versioning, see the `aws.s3.BucketVersioningV2` resource.
*/
@JvmName("jhmrohqhecwijmmm")
public suspend fun token(`value`: Output) {
this.token = value
}
/**
* @param value Name of the bucket.
*/
@JvmName("ykexfaslmfsweevh")
public suspend fun bucket(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bucket = mapped
}
/**
* @param value Account ID of the expected bucket owner.
*/
@JvmName("thyhvvjkccuwtlul")
public suspend fun expectedBucketOwner(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expectedBucketOwner = mapped
}
/**
* @param value Indicates whether this bucket has an Object Lock configuration enabled. Defaults to `Enabled`. Valid values: `Enabled`.
*/
@JvmName("appswnqljreixidq")
public suspend fun objectLockEnabled(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.objectLockEnabled = mapped
}
/**
* @param value Configuration block for specifying the Object Lock rule for the specified object. See below.
*/
@JvmName("tvvjstimwaqueipc")
public suspend fun rule(`value`: BucketObjectLockConfigurationV2RuleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rule = mapped
}
/**
* @param argument Configuration block for specifying the Object Lock rule for the specified object. See below.
*/
@JvmName("crrosialrcrisexx")
public suspend fun rule(argument: suspend BucketObjectLockConfigurationV2RuleArgsBuilder.() -> Unit) {
val toBeMapped = BucketObjectLockConfigurationV2RuleArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rule = mapped
}
/**
* @param value This argument is deprecated and no longer needed to enable Object Lock.
* To enable Object Lock for an existing bucket, you must first enable versioning on the bucket and then enable Object Lock. For more details on versioning, see the `aws.s3.BucketVersioningV2` resource.
*/
@JvmName("tbkxilpdhqykjwsm")
public suspend fun token(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.token = mapped
}
internal fun build(): BucketObjectLockConfigurationV2Args = BucketObjectLockConfigurationV2Args(
bucket = bucket,
expectedBucketOwner = expectedBucketOwner,
objectLockEnabled = objectLockEnabled,
rule = rule,
token = token,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy