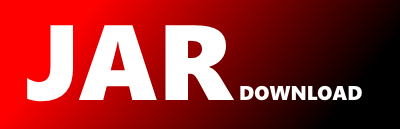
com.pulumi.aws.s3.kotlin.BucketPublicAccessBlockArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin
import com.pulumi.aws.s3.BucketPublicAccessBlockArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages S3 bucket-level Public Access Block configuration. For more information about these settings, see the [AWS S3 Block Public Access documentation](https://docs.aws.amazon.com/AmazonS3/latest/dev/access-control-block-public-access.html).
* > This resource cannot be used with S3 directory buckets.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.s3.BucketV2("example", {bucket: "example"});
* const exampleBucketPublicAccessBlock = new aws.s3.BucketPublicAccessBlock("example", {
* bucket: example.id,
* blockPublicAcls: true,
* blockPublicPolicy: true,
* ignorePublicAcls: true,
* restrictPublicBuckets: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.s3.BucketV2("example", bucket="example")
* example_bucket_public_access_block = aws.s3.BucketPublicAccessBlock("example",
* bucket=example.id,
* block_public_acls=True,
* block_public_policy=True,
* ignore_public_acls=True,
* restrict_public_buckets=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "example",
* });
* var exampleBucketPublicAccessBlock = new Aws.S3.BucketPublicAccessBlock("example", new()
* {
* Bucket = example.Id,
* BlockPublicAcls = true,
* BlockPublicPolicy = true,
* IgnorePublicAcls = true,
* RestrictPublicBuckets = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketPublicAccessBlock(ctx, "example", &s3.BucketPublicAccessBlockArgs{
* Bucket: example.ID(),
* BlockPublicAcls: pulumi.Bool(true),
* BlockPublicPolicy: pulumi.Bool(true),
* IgnorePublicAcls: pulumi.Bool(true),
* RestrictPublicBuckets: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketPublicAccessBlock;
* import com.pulumi.aws.s3.BucketPublicAccessBlockArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("example")
* .build());
* var exampleBucketPublicAccessBlock = new BucketPublicAccessBlock("exampleBucketPublicAccessBlock", BucketPublicAccessBlockArgs.builder()
* .bucket(example.id())
* .blockPublicAcls(true)
* .blockPublicPolicy(true)
* .ignorePublicAcls(true)
* .restrictPublicBuckets(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:s3:BucketV2
* properties:
* bucket: example
* exampleBucketPublicAccessBlock:
* type: aws:s3:BucketPublicAccessBlock
* name: example
* properties:
* bucket: ${example.id}
* blockPublicAcls: true
* blockPublicPolicy: true
* ignorePublicAcls: true
* restrictPublicBuckets: true
* ```
*
* ## Import
* Using `pulumi import`, import `aws_s3_bucket_public_access_block` using the bucket name. For example:
* ```sh
* $ pulumi import aws:s3/bucketPublicAccessBlock:BucketPublicAccessBlock example my-bucket
* ```
* @property blockPublicAcls Whether Amazon S3 should block public ACLs for this bucket. Defaults to `false`. Enabling this setting does not affect existing policies or ACLs. When set to `true` causes the following behavior:
* * PUT Bucket acl and PUT Object acl calls will fail if the specified ACL allows public access.
* * PUT Object calls will fail if the request includes an object ACL.
* @property blockPublicPolicy Whether Amazon S3 should block public bucket policies for this bucket. Defaults to `false`. Enabling this setting does not affect the existing bucket policy. When set to `true` causes Amazon S3 to:
* * Reject calls to PUT Bucket policy if the specified bucket policy allows public access.
* @property bucket S3 Bucket to which this Public Access Block configuration should be applied.
* @property ignorePublicAcls Whether Amazon S3 should ignore public ACLs for this bucket. Defaults to `false`. Enabling this setting does not affect the persistence of any existing ACLs and doesn't prevent new public ACLs from being set. When set to `true` causes Amazon S3 to:
* * Ignore public ACLs on this bucket and any objects that it contains.
* @property restrictPublicBuckets Whether Amazon S3 should restrict public bucket policies for this bucket. Defaults to `false`. Enabling this setting does not affect the previously stored bucket policy, except that public and cross-account access within the public bucket policy, including non-public delegation to specific accounts, is blocked. When set to `true`:
* * Only the bucket owner and AWS Services can access this buckets if it has a public policy.
*/
public data class BucketPublicAccessBlockArgs(
public val blockPublicAcls: Output? = null,
public val blockPublicPolicy: Output? = null,
public val bucket: Output? = null,
public val ignorePublicAcls: Output? = null,
public val restrictPublicBuckets: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3.BucketPublicAccessBlockArgs =
com.pulumi.aws.s3.BucketPublicAccessBlockArgs.builder()
.blockPublicAcls(blockPublicAcls?.applyValue({ args0 -> args0 }))
.blockPublicPolicy(blockPublicPolicy?.applyValue({ args0 -> args0 }))
.bucket(bucket?.applyValue({ args0 -> args0 }))
.ignorePublicAcls(ignorePublicAcls?.applyValue({ args0 -> args0 }))
.restrictPublicBuckets(restrictPublicBuckets?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketPublicAccessBlockArgs].
*/
@PulumiTagMarker
public class BucketPublicAccessBlockArgsBuilder internal constructor() {
private var blockPublicAcls: Output? = null
private var blockPublicPolicy: Output? = null
private var bucket: Output? = null
private var ignorePublicAcls: Output? = null
private var restrictPublicBuckets: Output? = null
/**
* @param value Whether Amazon S3 should block public ACLs for this bucket. Defaults to `false`. Enabling this setting does not affect existing policies or ACLs. When set to `true` causes the following behavior:
* * PUT Bucket acl and PUT Object acl calls will fail if the specified ACL allows public access.
* * PUT Object calls will fail if the request includes an object ACL.
*/
@JvmName("irkleelllwyowmxj")
public suspend fun blockPublicAcls(`value`: Output) {
this.blockPublicAcls = value
}
/**
* @param value Whether Amazon S3 should block public bucket policies for this bucket. Defaults to `false`. Enabling this setting does not affect the existing bucket policy. When set to `true` causes Amazon S3 to:
* * Reject calls to PUT Bucket policy if the specified bucket policy allows public access.
*/
@JvmName("efqvhnehmlaheuwq")
public suspend fun blockPublicPolicy(`value`: Output) {
this.blockPublicPolicy = value
}
/**
* @param value S3 Bucket to which this Public Access Block configuration should be applied.
*/
@JvmName("vqhifkfywraaqiis")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Whether Amazon S3 should ignore public ACLs for this bucket. Defaults to `false`. Enabling this setting does not affect the persistence of any existing ACLs and doesn't prevent new public ACLs from being set. When set to `true` causes Amazon S3 to:
* * Ignore public ACLs on this bucket and any objects that it contains.
*/
@JvmName("xpmtbuwofpkwfpin")
public suspend fun ignorePublicAcls(`value`: Output) {
this.ignorePublicAcls = value
}
/**
* @param value Whether Amazon S3 should restrict public bucket policies for this bucket. Defaults to `false`. Enabling this setting does not affect the previously stored bucket policy, except that public and cross-account access within the public bucket policy, including non-public delegation to specific accounts, is blocked. When set to `true`:
* * Only the bucket owner and AWS Services can access this buckets if it has a public policy.
*/
@JvmName("rhbsqktyrdckwntj")
public suspend fun restrictPublicBuckets(`value`: Output) {
this.restrictPublicBuckets = value
}
/**
* @param value Whether Amazon S3 should block public ACLs for this bucket. Defaults to `false`. Enabling this setting does not affect existing policies or ACLs. When set to `true` causes the following behavior:
* * PUT Bucket acl and PUT Object acl calls will fail if the specified ACL allows public access.
* * PUT Object calls will fail if the request includes an object ACL.
*/
@JvmName("tepfiqfribyqntli")
public suspend fun blockPublicAcls(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blockPublicAcls = mapped
}
/**
* @param value Whether Amazon S3 should block public bucket policies for this bucket. Defaults to `false`. Enabling this setting does not affect the existing bucket policy. When set to `true` causes Amazon S3 to:
* * Reject calls to PUT Bucket policy if the specified bucket policy allows public access.
*/
@JvmName("ccywqrysocsjrqfd")
public suspend fun blockPublicPolicy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blockPublicPolicy = mapped
}
/**
* @param value S3 Bucket to which this Public Access Block configuration should be applied.
*/
@JvmName("shfvuxbijbigcsbu")
public suspend fun bucket(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bucket = mapped
}
/**
* @param value Whether Amazon S3 should ignore public ACLs for this bucket. Defaults to `false`. Enabling this setting does not affect the persistence of any existing ACLs and doesn't prevent new public ACLs from being set. When set to `true` causes Amazon S3 to:
* * Ignore public ACLs on this bucket and any objects that it contains.
*/
@JvmName("eiyygjbbbpuydgmm")
public suspend fun ignorePublicAcls(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ignorePublicAcls = mapped
}
/**
* @param value Whether Amazon S3 should restrict public bucket policies for this bucket. Defaults to `false`. Enabling this setting does not affect the previously stored bucket policy, except that public and cross-account access within the public bucket policy, including non-public delegation to specific accounts, is blocked. When set to `true`:
* * Only the bucket owner and AWS Services can access this buckets if it has a public policy.
*/
@JvmName("hioinvtxuijfpenc")
public suspend fun restrictPublicBuckets(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.restrictPublicBuckets = mapped
}
internal fun build(): BucketPublicAccessBlockArgs = BucketPublicAccessBlockArgs(
blockPublicAcls = blockPublicAcls,
blockPublicPolicy = blockPublicPolicy,
bucket = bucket,
ignorePublicAcls = ignorePublicAcls,
restrictPublicBuckets = restrictPublicBuckets,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy