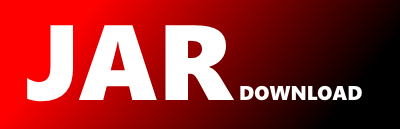
com.pulumi.aws.s3.kotlin.InventoryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin
import com.pulumi.aws.s3.InventoryArgs.builder
import com.pulumi.aws.s3.kotlin.inputs.InventoryDestinationArgs
import com.pulumi.aws.s3.kotlin.inputs.InventoryDestinationArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.InventoryFilterArgs
import com.pulumi.aws.s3.kotlin.inputs.InventoryFilterArgsBuilder
import com.pulumi.aws.s3.kotlin.inputs.InventoryScheduleArgs
import com.pulumi.aws.s3.kotlin.inputs.InventoryScheduleArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a S3 bucket [inventory configuration](https://docs.aws.amazon.com/AmazonS3/latest/dev/storage-inventory.html) resource.
* > This resource cannot be used with S3 directory buckets.
* ## Example Usage
* ### Add inventory configuration
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.s3.BucketV2("test", {bucket: "my-tf-test-bucket"});
* const inventory = new aws.s3.BucketV2("inventory", {bucket: "my-tf-inventory-bucket"});
* const testInventory = new aws.s3.Inventory("test", {
* bucket: test.id,
* name: "EntireBucketDaily",
* includedObjectVersions: "All",
* schedule: {
* frequency: "Daily",
* },
* destination: {
* bucket: {
* format: "ORC",
* bucketArn: inventory.arn,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.s3.BucketV2("test", bucket="my-tf-test-bucket")
* inventory = aws.s3.BucketV2("inventory", bucket="my-tf-inventory-bucket")
* test_inventory = aws.s3.Inventory("test",
* bucket=test.id,
* name="EntireBucketDaily",
* included_object_versions="All",
* schedule={
* "frequency": "Daily",
* },
* destination={
* "bucket": {
* "format": "ORC",
* "bucket_arn": inventory.arn,
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.S3.BucketV2("test", new()
* {
* Bucket = "my-tf-test-bucket",
* });
* var inventory = new Aws.S3.BucketV2("inventory", new()
* {
* Bucket = "my-tf-inventory-bucket",
* });
* var testInventory = new Aws.S3.Inventory("test", new()
* {
* Bucket = test.Id,
* Name = "EntireBucketDaily",
* IncludedObjectVersions = "All",
* Schedule = new Aws.S3.Inputs.InventoryScheduleArgs
* {
* Frequency = "Daily",
* },
* Destination = new Aws.S3.Inputs.InventoryDestinationArgs
* {
* Bucket = new Aws.S3.Inputs.InventoryDestinationBucketArgs
* {
* Format = "ORC",
* BucketArn = inventory.Arn,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := s3.NewBucketV2(ctx, "test", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-test-bucket"),
* })
* if err != nil {
* return err
* }
* inventory, err := s3.NewBucketV2(ctx, "inventory", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-inventory-bucket"),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewInventory(ctx, "test", &s3.InventoryArgs{
* Bucket: test.ID(),
* Name: pulumi.String("EntireBucketDaily"),
* IncludedObjectVersions: pulumi.String("All"),
* Schedule: &s3.InventoryScheduleArgs{
* Frequency: pulumi.String("Daily"),
* },
* Destination: &s3.InventoryDestinationArgs{
* Bucket: &s3.InventoryDestinationBucketArgs{
* Format: pulumi.String("ORC"),
* BucketArn: inventory.Arn,
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.Inventory;
* import com.pulumi.aws.s3.InventoryArgs;
* import com.pulumi.aws.s3.inputs.InventoryScheduleArgs;
* import com.pulumi.aws.s3.inputs.InventoryDestinationArgs;
* import com.pulumi.aws.s3.inputs.InventoryDestinationBucketArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new BucketV2("test", BucketV2Args.builder()
* .bucket("my-tf-test-bucket")
* .build());
* var inventory = new BucketV2("inventory", BucketV2Args.builder()
* .bucket("my-tf-inventory-bucket")
* .build());
* var testInventory = new Inventory("testInventory", InventoryArgs.builder()
* .bucket(test.id())
* .name("EntireBucketDaily")
* .includedObjectVersions("All")
* .schedule(InventoryScheduleArgs.builder()
* .frequency("Daily")
* .build())
* .destination(InventoryDestinationArgs.builder()
* .bucket(InventoryDestinationBucketArgs.builder()
* .format("ORC")
* .bucketArn(inventory.arn())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-test-bucket
* inventory:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-inventory-bucket
* testInventory:
* type: aws:s3:Inventory
* name: test
* properties:
* bucket: ${test.id}
* name: EntireBucketDaily
* includedObjectVersions: All
* schedule:
* frequency: Daily
* destination:
* bucket:
* format: ORC
* bucketArn: ${inventory.arn}
* ```
*
* ### Add inventory configuration with S3 object prefix
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.s3.BucketV2("test", {bucket: "my-tf-test-bucket"});
* const inventory = new aws.s3.BucketV2("inventory", {bucket: "my-tf-inventory-bucket"});
* const test_prefix = new aws.s3.Inventory("test-prefix", {
* bucket: test.id,
* name: "DocumentsWeekly",
* includedObjectVersions: "All",
* schedule: {
* frequency: "Daily",
* },
* filter: {
* prefix: "documents/",
* },
* destination: {
* bucket: {
* format: "ORC",
* bucketArn: inventory.arn,
* prefix: "inventory",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.s3.BucketV2("test", bucket="my-tf-test-bucket")
* inventory = aws.s3.BucketV2("inventory", bucket="my-tf-inventory-bucket")
* test_prefix = aws.s3.Inventory("test-prefix",
* bucket=test.id,
* name="DocumentsWeekly",
* included_object_versions="All",
* schedule={
* "frequency": "Daily",
* },
* filter={
* "prefix": "documents/",
* },
* destination={
* "bucket": {
* "format": "ORC",
* "bucket_arn": inventory.arn,
* "prefix": "inventory",
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.S3.BucketV2("test", new()
* {
* Bucket = "my-tf-test-bucket",
* });
* var inventory = new Aws.S3.BucketV2("inventory", new()
* {
* Bucket = "my-tf-inventory-bucket",
* });
* var test_prefix = new Aws.S3.Inventory("test-prefix", new()
* {
* Bucket = test.Id,
* Name = "DocumentsWeekly",
* IncludedObjectVersions = "All",
* Schedule = new Aws.S3.Inputs.InventoryScheduleArgs
* {
* Frequency = "Daily",
* },
* Filter = new Aws.S3.Inputs.InventoryFilterArgs
* {
* Prefix = "documents/",
* },
* Destination = new Aws.S3.Inputs.InventoryDestinationArgs
* {
* Bucket = new Aws.S3.Inputs.InventoryDestinationBucketArgs
* {
* Format = "ORC",
* BucketArn = inventory.Arn,
* Prefix = "inventory",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := s3.NewBucketV2(ctx, "test", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-test-bucket"),
* })
* if err != nil {
* return err
* }
* inventory, err := s3.NewBucketV2(ctx, "inventory", &s3.BucketV2Args{
* Bucket: pulumi.String("my-tf-inventory-bucket"),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewInventory(ctx, "test-prefix", &s3.InventoryArgs{
* Bucket: test.ID(),
* Name: pulumi.String("DocumentsWeekly"),
* IncludedObjectVersions: pulumi.String("All"),
* Schedule: &s3.InventoryScheduleArgs{
* Frequency: pulumi.String("Daily"),
* },
* Filter: &s3.InventoryFilterArgs{
* Prefix: pulumi.String("documents/"),
* },
* Destination: &s3.InventoryDestinationArgs{
* Bucket: &s3.InventoryDestinationBucketArgs{
* Format: pulumi.String("ORC"),
* BucketArn: inventory.Arn,
* Prefix: pulumi.String("inventory"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.Inventory;
* import com.pulumi.aws.s3.InventoryArgs;
* import com.pulumi.aws.s3.inputs.InventoryScheduleArgs;
* import com.pulumi.aws.s3.inputs.InventoryFilterArgs;
* import com.pulumi.aws.s3.inputs.InventoryDestinationArgs;
* import com.pulumi.aws.s3.inputs.InventoryDestinationBucketArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new BucketV2("test", BucketV2Args.builder()
* .bucket("my-tf-test-bucket")
* .build());
* var inventory = new BucketV2("inventory", BucketV2Args.builder()
* .bucket("my-tf-inventory-bucket")
* .build());
* var test_prefix = new Inventory("test-prefix", InventoryArgs.builder()
* .bucket(test.id())
* .name("DocumentsWeekly")
* .includedObjectVersions("All")
* .schedule(InventoryScheduleArgs.builder()
* .frequency("Daily")
* .build())
* .filter(InventoryFilterArgs.builder()
* .prefix("documents/")
* .build())
* .destination(InventoryDestinationArgs.builder()
* .bucket(InventoryDestinationBucketArgs.builder()
* .format("ORC")
* .bucketArn(inventory.arn())
* .prefix("inventory")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-test-bucket
* inventory:
* type: aws:s3:BucketV2
* properties:
* bucket: my-tf-inventory-bucket
* test-prefix:
* type: aws:s3:Inventory
* properties:
* bucket: ${test.id}
* name: DocumentsWeekly
* includedObjectVersions: All
* schedule:
* frequency: Daily
* filter:
* prefix: documents/
* destination:
* bucket:
* format: ORC
* bucketArn: ${inventory.arn}
* prefix: inventory
* ```
*
* ## Import
* Using `pulumi import`, import S3 bucket inventory configurations using `bucket:inventory`. For example:
* ```sh
* $ pulumi import aws:s3/inventory:Inventory my-bucket-entire-bucket my-bucket:EntireBucket
* ```
* @property bucket Name of the source bucket that inventory lists the objects for.
* @property destination Contains information about where to publish the inventory results (documented below).
* @property enabled Specifies whether the inventory is enabled or disabled.
* @property filter Specifies an inventory filter. The inventory only includes objects that meet the filter's criteria (documented below).
* @property includedObjectVersions Object versions to include in the inventory list. Valid values: `All`, `Current`.
* @property name Unique identifier of the inventory configuration for the bucket.
* @property optionalFields List of optional fields that are included in the inventory results. Please refer to the S3 [documentation](https://docs.aws.amazon.com/AmazonS3/latest/API/API_InventoryConfiguration.html#AmazonS3-Type-InventoryConfiguration-OptionalFields) for more details.
* @property schedule Specifies the schedule for generating inventory results (documented below).
*/
public data class InventoryArgs(
public val bucket: Output? = null,
public val destination: Output? = null,
public val enabled: Output? = null,
public val filter: Output? = null,
public val includedObjectVersions: Output? = null,
public val name: Output? = null,
public val optionalFields: Output>? = null,
public val schedule: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3.InventoryArgs = com.pulumi.aws.s3.InventoryArgs.builder()
.bucket(bucket?.applyValue({ args0 -> args0 }))
.destination(destination?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.filter(filter?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.includedObjectVersions(includedObjectVersions?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.optionalFields(optionalFields?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.schedule(schedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [InventoryArgs].
*/
@PulumiTagMarker
public class InventoryArgsBuilder internal constructor() {
private var bucket: Output? = null
private var destination: Output? = null
private var enabled: Output? = null
private var filter: Output? = null
private var includedObjectVersions: Output? = null
private var name: Output? = null
private var optionalFields: Output>? = null
private var schedule: Output? = null
/**
* @param value Name of the source bucket that inventory lists the objects for.
*/
@JvmName("hwvvwymensjgxehj")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Contains information about where to publish the inventory results (documented below).
*/
@JvmName("vxshkxnucmjgmgus")
public suspend fun destination(`value`: Output) {
this.destination = value
}
/**
* @param value Specifies whether the inventory is enabled or disabled.
*/
@JvmName("cimmrenjykltaqtg")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Specifies an inventory filter. The inventory only includes objects that meet the filter's criteria (documented below).
*/
@JvmName("pbwiycgfkvgfqdyn")
public suspend fun filter(`value`: Output) {
this.filter = value
}
/**
* @param value Object versions to include in the inventory list. Valid values: `All`, `Current`.
*/
@JvmName("tfnsnbmvjentaweh")
public suspend fun includedObjectVersions(`value`: Output) {
this.includedObjectVersions = value
}
/**
* @param value Unique identifier of the inventory configuration for the bucket.
*/
@JvmName("hebghfqaplmyaivd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value List of optional fields that are included in the inventory results. Please refer to the S3 [documentation](https://docs.aws.amazon.com/AmazonS3/latest/API/API_InventoryConfiguration.html#AmazonS3-Type-InventoryConfiguration-OptionalFields) for more details.
*/
@JvmName("tnkimldrgxtowjuw")
public suspend fun optionalFields(`value`: Output>) {
this.optionalFields = value
}
@JvmName("hmfhiechwtojgagc")
public suspend fun optionalFields(vararg values: Output) {
this.optionalFields = Output.all(values.asList())
}
/**
* @param values List of optional fields that are included in the inventory results. Please refer to the S3 [documentation](https://docs.aws.amazon.com/AmazonS3/latest/API/API_InventoryConfiguration.html#AmazonS3-Type-InventoryConfiguration-OptionalFields) for more details.
*/
@JvmName("tsxyocrskbtehkky")
public suspend fun optionalFields(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy