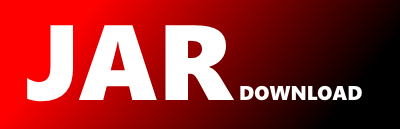
com.pulumi.aws.s3.kotlin.inputs.BucketReplicationConfigRuleDestinationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin.inputs
import com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleDestinationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property accessControlTranslation Configuration block that specifies the overrides to use for object owners on replication. See below. Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS account that owns the source object. Must be used in conjunction with `account` owner override configuration.
* @property account Account ID to specify the replica ownership. Must be used in conjunction with `access_control_translation` override configuration.
* @property bucket ARN of the bucket where you want Amazon S3 to store the results.
* @property encryptionConfiguration Configuration block that provides information about encryption. See below. If `source_selection_criteria` is specified, you must specify this element.
* @property metrics Configuration block that specifies replication metrics-related settings enabling replication metrics and events. See below.
* @property replicationTime Configuration block that specifies S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. See below. Replication Time Control must be used in conjunction with `metrics`.
* @property storageClass The [storage class](https://docs.aws.amazon.com/AmazonS3/latest/API/API_Destination.html#AmazonS3-Type-Destination-StorageClass) used to store the object. By default, Amazon S3 uses the storage class of the source object to create the object replica.
*/
public data class BucketReplicationConfigRuleDestinationArgs(
public val accessControlTranslation: Output? = null,
public val account: Output? = null,
public val bucket: Output,
public val encryptionConfiguration: Output? = null,
public val metrics: Output? = null,
public val replicationTime: Output? =
null,
public val storageClass: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleDestinationArgs =
com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleDestinationArgs.builder()
.accessControlTranslation(
accessControlTranslation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.account(account?.applyValue({ args0 -> args0 }))
.bucket(bucket.applyValue({ args0 -> args0 }))
.encryptionConfiguration(
encryptionConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.metrics(metrics?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.replicationTime(replicationTime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.storageClass(storageClass?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketReplicationConfigRuleDestinationArgs].
*/
@PulumiTagMarker
public class BucketReplicationConfigRuleDestinationArgsBuilder internal constructor() {
private var accessControlTranslation:
Output? = null
private var account: Output? = null
private var bucket: Output? = null
private var encryptionConfiguration:
Output? = null
private var metrics: Output? = null
private var replicationTime: Output? =
null
private var storageClass: Output? = null
/**
* @param value Configuration block that specifies the overrides to use for object owners on replication. See below. Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS account that owns the source object. Must be used in conjunction with `account` owner override configuration.
*/
@JvmName("qseampgxvmmvbxdv")
public suspend fun accessControlTranslation(`value`: Output) {
this.accessControlTranslation = value
}
/**
* @param value Account ID to specify the replica ownership. Must be used in conjunction with `access_control_translation` override configuration.
*/
@JvmName("ifditpneflgutidi")
public suspend fun account(`value`: Output) {
this.account = value
}
/**
* @param value ARN of the bucket where you want Amazon S3 to store the results.
*/
@JvmName("gwqwwjinddfagock")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Configuration block that provides information about encryption. See below. If `source_selection_criteria` is specified, you must specify this element.
*/
@JvmName("tfondvnfnbftgdou")
public suspend fun encryptionConfiguration(`value`: Output) {
this.encryptionConfiguration = value
}
/**
* @param value Configuration block that specifies replication metrics-related settings enabling replication metrics and events. See below.
*/
@JvmName("mnigppsvrfaagolu")
public suspend fun metrics(`value`: Output) {
this.metrics = value
}
/**
* @param value Configuration block that specifies S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. See below. Replication Time Control must be used in conjunction with `metrics`.
*/
@JvmName("gegbpkolqxcppjfk")
public suspend fun replicationTime(`value`: Output) {
this.replicationTime = value
}
/**
* @param value The [storage class](https://docs.aws.amazon.com/AmazonS3/latest/API/API_Destination.html#AmazonS3-Type-Destination-StorageClass) used to store the object. By default, Amazon S3 uses the storage class of the source object to create the object replica.
*/
@JvmName("tydibmyeccpoeywr")
public suspend fun storageClass(`value`: Output) {
this.storageClass = value
}
/**
* @param value Configuration block that specifies the overrides to use for object owners on replication. See below. Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS account that owns the source object. Must be used in conjunction with `account` owner override configuration.
*/
@JvmName("uenuqnhocmgfsfey")
public suspend fun accessControlTranslation(`value`: BucketReplicationConfigRuleDestinationAccessControlTranslationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessControlTranslation = mapped
}
/**
* @param argument Configuration block that specifies the overrides to use for object owners on replication. See below. Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS account that owns the source object. Must be used in conjunction with `account` owner override configuration.
*/
@JvmName("mfiwdnjwjyftifaj")
public suspend fun accessControlTranslation(argument: suspend BucketReplicationConfigRuleDestinationAccessControlTranslationArgsBuilder.() -> Unit) {
val toBeMapped =
BucketReplicationConfigRuleDestinationAccessControlTranslationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.accessControlTranslation = mapped
}
/**
* @param value Account ID to specify the replica ownership. Must be used in conjunction with `access_control_translation` override configuration.
*/
@JvmName("cgplsvpgbthvwlwl")
public suspend fun account(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.account = mapped
}
/**
* @param value ARN of the bucket where you want Amazon S3 to store the results.
*/
@JvmName("rttfwbuienniawnr")
public suspend fun bucket(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucket = mapped
}
/**
* @param value Configuration block that provides information about encryption. See below. If `source_selection_criteria` is specified, you must specify this element.
*/
@JvmName("dsgtuaplqewppxxp")
public suspend fun encryptionConfiguration(`value`: BucketReplicationConfigRuleDestinationEncryptionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfiguration = mapped
}
/**
* @param argument Configuration block that provides information about encryption. See below. If `source_selection_criteria` is specified, you must specify this element.
*/
@JvmName("vqwvebnfcmtmgxyx")
public suspend fun encryptionConfiguration(argument: suspend BucketReplicationConfigRuleDestinationEncryptionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
BucketReplicationConfigRuleDestinationEncryptionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.encryptionConfiguration = mapped
}
/**
* @param value Configuration block that specifies replication metrics-related settings enabling replication metrics and events. See below.
*/
@JvmName("wvjgmggeibepneji")
public suspend fun metrics(`value`: BucketReplicationConfigRuleDestinationMetricsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metrics = mapped
}
/**
* @param argument Configuration block that specifies replication metrics-related settings enabling replication metrics and events. See below.
*/
@JvmName("rcjdfxthwnuyvydh")
public suspend fun metrics(argument: suspend BucketReplicationConfigRuleDestinationMetricsArgsBuilder.() -> Unit) {
val toBeMapped = BucketReplicationConfigRuleDestinationMetricsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.metrics = mapped
}
/**
* @param value Configuration block that specifies S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. See below. Replication Time Control must be used in conjunction with `metrics`.
*/
@JvmName("dxiymrgdhonihhms")
public suspend fun replicationTime(`value`: BucketReplicationConfigRuleDestinationReplicationTimeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replicationTime = mapped
}
/**
* @param argument Configuration block that specifies S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. See below. Replication Time Control must be used in conjunction with `metrics`.
*/
@JvmName("ydpukvqpgcoicsnf")
public suspend fun replicationTime(argument: suspend BucketReplicationConfigRuleDestinationReplicationTimeArgsBuilder.() -> Unit) {
val toBeMapped =
BucketReplicationConfigRuleDestinationReplicationTimeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.replicationTime = mapped
}
/**
* @param value The [storage class](https://docs.aws.amazon.com/AmazonS3/latest/API/API_Destination.html#AmazonS3-Type-Destination-StorageClass) used to store the object. By default, Amazon S3 uses the storage class of the source object to create the object replica.
*/
@JvmName("kkfmycjteyflssoj")
public suspend fun storageClass(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageClass = mapped
}
internal fun build(): BucketReplicationConfigRuleDestinationArgs =
BucketReplicationConfigRuleDestinationArgs(
accessControlTranslation = accessControlTranslation,
account = account,
bucket = bucket ?: throw PulumiNullFieldException("bucket"),
encryptionConfiguration = encryptionConfiguration,
metrics = metrics,
replicationTime = replicationTime,
storageClass = storageClass,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy