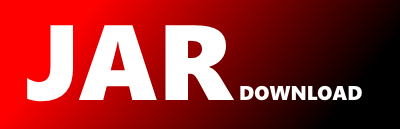
com.pulumi.aws.s3.kotlin.inputs.BucketReplicationConfigurationRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin.inputs
import com.pulumi.aws.s3.inputs.BucketReplicationConfigurationRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property deleteMarkerReplicationStatus Whether delete markers are replicated. The only valid value is `Enabled`. To disable, omit this argument. This argument is only valid with V2 replication configurations (i.e., when `filter` is used).
* @property destination Specifies the destination for the rule (documented below).
* @property filter Filter that identifies subset of objects to which the replication rule applies (documented below).
* @property id Unique identifier for the rule. Must be less than or equal to 255 characters in length.
* @property prefix Object keyname prefix identifying one or more objects to which the rule applies. Must be less than or equal to 1024 characters in length.
* @property priority The priority associated with the rule. Priority should only be set if `filter` is configured. If not provided, defaults to `0`. Priority must be unique between multiple rules.
* @property sourceSelectionCriteria Specifies special object selection criteria (documented below).
* @property status The status of the rule. Either `Enabled` or `Disabled`. The rule is ignored if status is not Enabled.
* > **NOTE:** Replication to multiple destination buckets requires that `priority` is specified in the `rules` object. If the corresponding rule requires no filter, an empty configuration block `filter {}` must be specified.
*/
public data class BucketReplicationConfigurationRuleArgs(
public val deleteMarkerReplicationStatus: Output? = null,
public val destination: Output,
public val filter: Output? = null,
public val id: Output? = null,
public val prefix: Output? = null,
public val priority: Output? = null,
public val sourceSelectionCriteria: Output? = null,
public val status: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3.inputs.BucketReplicationConfigurationRuleArgs =
com.pulumi.aws.s3.inputs.BucketReplicationConfigurationRuleArgs.builder()
.deleteMarkerReplicationStatus(deleteMarkerReplicationStatus?.applyValue({ args0 -> args0 }))
.destination(destination.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.filter(filter?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.id(id?.applyValue({ args0 -> args0 }))
.prefix(prefix?.applyValue({ args0 -> args0 }))
.priority(priority?.applyValue({ args0 -> args0 }))
.sourceSelectionCriteria(
sourceSelectionCriteria?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.status(status.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketReplicationConfigurationRuleArgs].
*/
@PulumiTagMarker
public class BucketReplicationConfigurationRuleArgsBuilder internal constructor() {
private var deleteMarkerReplicationStatus: Output? = null
private var destination: Output? = null
private var filter: Output? = null
private var id: Output? = null
private var prefix: Output? = null
private var priority: Output? = null
private var sourceSelectionCriteria:
Output? = null
private var status: Output? = null
/**
* @param value Whether delete markers are replicated. The only valid value is `Enabled`. To disable, omit this argument. This argument is only valid with V2 replication configurations (i.e., when `filter` is used).
*/
@JvmName("xhfcnwfsbmutugho")
public suspend fun deleteMarkerReplicationStatus(`value`: Output) {
this.deleteMarkerReplicationStatus = value
}
/**
* @param value Specifies the destination for the rule (documented below).
*/
@JvmName("ompskcrgvbmfhnge")
public suspend fun destination(`value`: Output) {
this.destination = value
}
/**
* @param value Filter that identifies subset of objects to which the replication rule applies (documented below).
*/
@JvmName("erusabamiyrfnapu")
public suspend fun filter(`value`: Output) {
this.filter = value
}
/**
* @param value Unique identifier for the rule. Must be less than or equal to 255 characters in length.
*/
@JvmName("fouqdyjmxlluxaii")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Object keyname prefix identifying one or more objects to which the rule applies. Must be less than or equal to 1024 characters in length.
*/
@JvmName("jkigsglqpeivvpqw")
public suspend fun prefix(`value`: Output) {
this.prefix = value
}
/**
* @param value The priority associated with the rule. Priority should only be set if `filter` is configured. If not provided, defaults to `0`. Priority must be unique between multiple rules.
*/
@JvmName("kvfjtbrmtryhifuj")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value Specifies special object selection criteria (documented below).
*/
@JvmName("ludymhytjfjelqic")
public suspend fun sourceSelectionCriteria(`value`: Output) {
this.sourceSelectionCriteria = value
}
/**
* @param value The status of the rule. Either `Enabled` or `Disabled`. The rule is ignored if status is not Enabled.
* > **NOTE:** Replication to multiple destination buckets requires that `priority` is specified in the `rules` object. If the corresponding rule requires no filter, an empty configuration block `filter {}` must be specified.
*/
@JvmName("bharutnodyiibpab")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value Whether delete markers are replicated. The only valid value is `Enabled`. To disable, omit this argument. This argument is only valid with V2 replication configurations (i.e., when `filter` is used).
*/
@JvmName("getprxalghrwebmv")
public suspend fun deleteMarkerReplicationStatus(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteMarkerReplicationStatus = mapped
}
/**
* @param value Specifies the destination for the rule (documented below).
*/
@JvmName("njejmeotlicesnak")
public suspend fun destination(`value`: BucketReplicationConfigurationRuleDestinationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.destination = mapped
}
/**
* @param argument Specifies the destination for the rule (documented below).
*/
@JvmName("vwtiuixkpdinwwbc")
public suspend fun destination(argument: suspend BucketReplicationConfigurationRuleDestinationArgsBuilder.() -> Unit) {
val toBeMapped = BucketReplicationConfigurationRuleDestinationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.destination = mapped
}
/**
* @param value Filter that identifies subset of objects to which the replication rule applies (documented below).
*/
@JvmName("fnmmcggtsrpphxvk")
public suspend fun filter(`value`: BucketReplicationConfigurationRuleFilterArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filter = mapped
}
/**
* @param argument Filter that identifies subset of objects to which the replication rule applies (documented below).
*/
@JvmName("lukwkduhatdlmaxv")
public suspend fun filter(argument: suspend BucketReplicationConfigurationRuleFilterArgsBuilder.() -> Unit) {
val toBeMapped = BucketReplicationConfigurationRuleFilterArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.filter = mapped
}
/**
* @param value Unique identifier for the rule. Must be less than or equal to 255 characters in length.
*/
@JvmName("qlcktlxpppwvhskf")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Object keyname prefix identifying one or more objects to which the rule applies. Must be less than or equal to 1024 characters in length.
*/
@JvmName("btjitsuocflhlqdt")
public suspend fun prefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.prefix = mapped
}
/**
* @param value The priority associated with the rule. Priority should only be set if `filter` is configured. If not provided, defaults to `0`. Priority must be unique between multiple rules.
*/
@JvmName("blvtqtqjfgflidjx")
public suspend fun priority(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.priority = mapped
}
/**
* @param value Specifies special object selection criteria (documented below).
*/
@JvmName("xnwbjolqcqsybypt")
public suspend fun sourceSelectionCriteria(`value`: BucketReplicationConfigurationRuleSourceSelectionCriteriaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceSelectionCriteria = mapped
}
/**
* @param argument Specifies special object selection criteria (documented below).
*/
@JvmName("uwfpembvikjvquuy")
public suspend fun sourceSelectionCriteria(argument: suspend BucketReplicationConfigurationRuleSourceSelectionCriteriaArgsBuilder.() -> Unit) {
val toBeMapped =
BucketReplicationConfigurationRuleSourceSelectionCriteriaArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sourceSelectionCriteria = mapped
}
/**
* @param value The status of the rule. Either `Enabled` or `Disabled`. The rule is ignored if status is not Enabled.
* > **NOTE:** Replication to multiple destination buckets requires that `priority` is specified in the `rules` object. If the corresponding rule requires no filter, an empty configuration block `filter {}` must be specified.
*/
@JvmName("qmxrkunlserxxnia")
public suspend fun status(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.status = mapped
}
internal fun build(): BucketReplicationConfigurationRuleArgs =
BucketReplicationConfigurationRuleArgs(
deleteMarkerReplicationStatus = deleteMarkerReplicationStatus,
destination = destination ?: throw PulumiNullFieldException("destination"),
filter = filter,
id = id,
prefix = prefix,
priority = priority,
sourceSelectionCriteria = sourceSelectionCriteria,
status = status ?: throw PulumiNullFieldException("status"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy