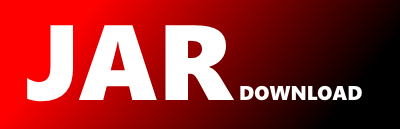
com.pulumi.aws.s3.kotlin.inputs.BucketV2LifecycleRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3.kotlin.inputs
import com.pulumi.aws.s3.inputs.BucketV2LifecycleRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property abortIncompleteMultipartUploadDays Specifies the number of days after initiating a multipart upload when the multipart upload must be completed.
* @property enabled Specifies lifecycle rule status.
* @property expirations Specifies a period in the object's expire. See Expiration below for details.
* @property id Unique identifier for the rule. Must be less than or equal to 255 characters in length.
* @property noncurrentVersionExpirations Specifies when noncurrent object versions expire. See Noncurrent Version Expiration below for details.
* @property noncurrentVersionTransitions Specifies when noncurrent object versions transitions. See Noncurrent Version Transition below for details.
* @property prefix Object key prefix identifying one or more objects to which the rule applies.
* @property tags Specifies object tags key and value.
* @property transitions Specifies a period in the object's transitions. See Transition below for details.
*/
public data class BucketV2LifecycleRuleArgs(
public val abortIncompleteMultipartUploadDays: Output? = null,
public val enabled: Output,
public val expirations: Output>? = null,
public val id: Output? = null,
public val noncurrentVersionExpirations: Output>? = null,
public val noncurrentVersionTransitions: Output>? = null,
public val prefix: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy