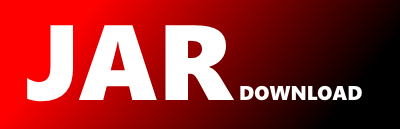
com.pulumi.aws.s3tables.kotlin.TablePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.s3tables.kotlin
import com.pulumi.aws.s3tables.TablePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource for managing an Amazon S3 Tables Table Policy.
* ## Example Usage
* ### Basic Usage
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.s3tables.TablePolicy;
* import com.pulumi.aws.s3tables.TablePolicyArgs;
* import com.pulumi.aws.s3tables.TableBucket;
* import com.pulumi.aws.s3tables.TableBucketArgs;
* import com.pulumi.aws.s3tables.Namespace;
* import com.pulumi.aws.s3tables.NamespaceArgs;
* import com.pulumi.aws.s3tables.Table;
* import com.pulumi.aws.s3tables.TableArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements()
* .build());
* var exampleTablePolicy = new TablePolicy("exampleTablePolicy", TablePolicyArgs.builder()
* .resourcePolicy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .name(test.name())
* .namespace(test.namespace())
* .tableBucketArn(test.tableBucketArn())
* .build());
* var exampleTableBucket = new TableBucket("exampleTableBucket", TableBucketArgs.builder()
* .name("example-bucket")
* .build());
* var exampleNamespace = new Namespace("exampleNamespace", NamespaceArgs.builder()
* .namespace("example-namespace")
* .tableBucketArn(exampleTableBucket.arn())
* .build());
* var exampleTable = new Table("exampleTable", TableArgs.builder()
* .name("example-table")
* .namespace(exampleNamespace)
* .tableBucketArn(exampleNamespace.tableBucketArn())
* .format("ICEBERG")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleTablePolicy:
* type: aws:s3tables:TablePolicy
* name: example
* properties:
* resourcePolicy: ${example.json}
* name: ${test.name}
* namespace: ${test.namespace}
* tableBucketArn: ${test.tableBucketArn}
* exampleTable:
* type: aws:s3tables:Table
* name: example
* properties:
* name: example-table
* namespace: ${exampleNamespace}
* tableBucketArn: ${exampleNamespace.tableBucketArn}
* format: ICEBERG
* exampleNamespace:
* type: aws:s3tables:Namespace
* name: example
* properties:
* namespace:
* - example-namespace
* tableBucketArn: ${exampleTableBucket.arn}
* exampleTableBucket:
* type: aws:s3tables:TableBucket
* name: example
* properties:
* name: example-bucket
* variables:
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - {}
* ```
*
* ## Import
* Using `pulumi import`, import S3 Tables Table Policy using the `table_bucket_arn`, the value of `namespace`, and the value of `name`, separated by a semicolon (`;`). For example:
* ```sh
* $ pulumi import aws:s3tables/tablePolicy:TablePolicy example 'arn:aws:s3tables:us-west-2:123456789012:bucket/example-bucket;example-namespace;example-table'
* ```
* @property name Name of the table.
* Must be between 1 and 255 characters in length.
* Can consist of lowercase letters, numbers, and underscores, and must begin and end with a lowercase letter or number.
* @property namespace Name of the namespace for this table.
* Must be between 1 and 255 characters in length.
* Can consist of lowercase letters, numbers, and underscores, and must begin and end with a lowercase letter or number.
* @property resourcePolicy Amazon Web Services resource-based policy document in JSON format.
* @property tableBucketArn ARN referencing the Table Bucket that contains this Namespace.
*/
public data class TablePolicyArgs(
public val name: Output? = null,
public val namespace: Output? = null,
public val resourcePolicy: Output? = null,
public val tableBucketArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.s3tables.TablePolicyArgs =
com.pulumi.aws.s3tables.TablePolicyArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.resourcePolicy(resourcePolicy?.applyValue({ args0 -> args0 }))
.tableBucketArn(tableBucketArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TablePolicyArgs].
*/
@PulumiTagMarker
public class TablePolicyArgsBuilder internal constructor() {
private var name: Output? = null
private var namespace: Output? = null
private var resourcePolicy: Output? = null
private var tableBucketArn: Output? = null
/**
* @param value Name of the table.
* Must be between 1 and 255 characters in length.
* Can consist of lowercase letters, numbers, and underscores, and must begin and end with a lowercase letter or number.
*/
@JvmName("senaioqelpqoqxjr")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Name of the namespace for this table.
* Must be between 1 and 255 characters in length.
* Can consist of lowercase letters, numbers, and underscores, and must begin and end with a lowercase letter or number.
*/
@JvmName("plrcxecygsecplxl")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Amazon Web Services resource-based policy document in JSON format.
*/
@JvmName("psixcomgtyhsvxfd")
public suspend fun resourcePolicy(`value`: Output) {
this.resourcePolicy = value
}
/**
* @param value ARN referencing the Table Bucket that contains this Namespace.
*/
@JvmName("gsqmcujahdbrgxrg")
public suspend fun tableBucketArn(`value`: Output) {
this.tableBucketArn = value
}
/**
* @param value Name of the table.
* Must be between 1 and 255 characters in length.
* Can consist of lowercase letters, numbers, and underscores, and must begin and end with a lowercase letter or number.
*/
@JvmName("kxiuflmaxmjulgyh")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Name of the namespace for this table.
* Must be between 1 and 255 characters in length.
* Can consist of lowercase letters, numbers, and underscores, and must begin and end with a lowercase letter or number.
*/
@JvmName("bpmpksslfwfctoop")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Amazon Web Services resource-based policy document in JSON format.
*/
@JvmName("ctvfrvaidsfjwhqo")
public suspend fun resourcePolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePolicy = mapped
}
/**
* @param value ARN referencing the Table Bucket that contains this Namespace.
*/
@JvmName("bvbljjbyaeqawkwx")
public suspend fun tableBucketArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tableBucketArn = mapped
}
internal fun build(): TablePolicyArgs = TablePolicyArgs(
name = name,
namespace = namespace,
resourcePolicy = resourcePolicy,
tableBucketArn = tableBucketArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy