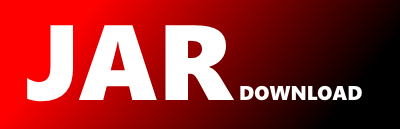
com.pulumi.aws.sagemaker.kotlin.inputs.DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sagemaker.kotlin.inputs
import com.pulumi.aws.sagemaker.inputs.DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property endpointName An endpoint in customer's account which has `data_capture_config` enabled.
* @property localPath Path to the filesystem where the endpoint data is available to the container. Defaults to `/opt/ml/processing/input`.
* @property s3DataDistributionType Whether input data distributed in Amazon S3 is fully replicated or sharded by an S3 key. Defaults to `FullyReplicated`. Valid values are `FullyReplicated` or `ShardedByS3Key`
* @property s3InputMode Whether the `Pipe` or `File` is used as the input mode for transferring data for the monitoring job. `Pipe` mode is recommended for large datasets. `File` mode is useful for small files that fit in memory. Defaults to `File`. Valid values are `Pipe` or `File`
*/
public data class DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs(
public val endpointName: Output,
public val localPath: Output? = null,
public val s3DataDistributionType: Output? = null,
public val s3InputMode: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sagemaker.inputs.DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs =
com.pulumi.aws.sagemaker.inputs.DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs.builder()
.endpointName(endpointName.applyValue({ args0 -> args0 }))
.localPath(localPath?.applyValue({ args0 -> args0 }))
.s3DataDistributionType(s3DataDistributionType?.applyValue({ args0 -> args0 }))
.s3InputMode(s3InputMode?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs].
*/
@PulumiTagMarker
public class DataQualityJobDefinitionDataQualityJobInputEndpointInputArgsBuilder internal constructor() {
private var endpointName: Output? = null
private var localPath: Output? = null
private var s3DataDistributionType: Output? = null
private var s3InputMode: Output? = null
/**
* @param value An endpoint in customer's account which has `data_capture_config` enabled.
*/
@JvmName("xiapskkwhhiyrttx")
public suspend fun endpointName(`value`: Output) {
this.endpointName = value
}
/**
* @param value Path to the filesystem where the endpoint data is available to the container. Defaults to `/opt/ml/processing/input`.
*/
@JvmName("edwvsnxixomiewmo")
public suspend fun localPath(`value`: Output) {
this.localPath = value
}
/**
* @param value Whether input data distributed in Amazon S3 is fully replicated or sharded by an S3 key. Defaults to `FullyReplicated`. Valid values are `FullyReplicated` or `ShardedByS3Key`
*/
@JvmName("wcvprusqkmtnfvxa")
public suspend fun s3DataDistributionType(`value`: Output) {
this.s3DataDistributionType = value
}
/**
* @param value Whether the `Pipe` or `File` is used as the input mode for transferring data for the monitoring job. `Pipe` mode is recommended for large datasets. `File` mode is useful for small files that fit in memory. Defaults to `File`. Valid values are `Pipe` or `File`
*/
@JvmName("yrowwtikueqcpqpv")
public suspend fun s3InputMode(`value`: Output) {
this.s3InputMode = value
}
/**
* @param value An endpoint in customer's account which has `data_capture_config` enabled.
*/
@JvmName("okcoaabwvsdrsuju")
public suspend fun endpointName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpointName = mapped
}
/**
* @param value Path to the filesystem where the endpoint data is available to the container. Defaults to `/opt/ml/processing/input`.
*/
@JvmName("xbyxjcxbluwanmcu")
public suspend fun localPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.localPath = mapped
}
/**
* @param value Whether input data distributed in Amazon S3 is fully replicated or sharded by an S3 key. Defaults to `FullyReplicated`. Valid values are `FullyReplicated` or `ShardedByS3Key`
*/
@JvmName("guixkqegxlpwnigc")
public suspend fun s3DataDistributionType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3DataDistributionType = mapped
}
/**
* @param value Whether the `Pipe` or `File` is used as the input mode for transferring data for the monitoring job. `Pipe` mode is recommended for large datasets. `File` mode is useful for small files that fit in memory. Defaults to `File`. Valid values are `Pipe` or `File`
*/
@JvmName("jwbaawcvkjekckot")
public suspend fun s3InputMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3InputMode = mapped
}
internal fun build(): DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs =
DataQualityJobDefinitionDataQualityJobInputEndpointInputArgs(
endpointName = endpointName ?: throw PulumiNullFieldException("endpointName"),
localPath = localPath,
s3DataDistributionType = s3DataDistributionType,
s3InputMode = s3InputMode,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy