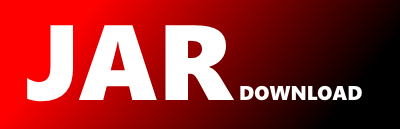
com.pulumi.aws.sagemaker.kotlin.inputs.DomainDefaultSpaceSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sagemaker.kotlin.inputs
import com.pulumi.aws.sagemaker.inputs.DomainDefaultSpaceSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property customFileSystemConfigs The settings for assigning a custom file system to a user profile. Permitted users can access this file system in Amazon SageMaker Studio. See `custom_file_system_config` Block below.
* @property customPosixUserConfig Details about the POSIX identity that is used for file system operations. See `custom_posix_user_config` Block below.
* @property executionRole The execution role for the space.
* @property jupyterLabAppSettings The settings for the JupyterLab application. See `jupyter_lab_app_settings` Block below.
* @property jupyterServerAppSettings The Jupyter server's app settings. See `jupyter_server_app_settings` Block below.
* @property kernelGatewayAppSettings The kernel gateway app settings. See `kernel_gateway_app_settings` Block below.
* @property securityGroups The security groups for the Amazon Virtual Private Cloud that the space uses for communication.
* @property spaceStorageSettings The storage settings for a private space. See `space_storage_settings` Block below.
*/
public data class DomainDefaultSpaceSettingsArgs(
public val customFileSystemConfigs: Output>? = null,
public val customPosixUserConfig: Output? =
null,
public val executionRole: Output,
public val jupyterLabAppSettings: Output? =
null,
public val jupyterServerAppSettings: Output? = null,
public val kernelGatewayAppSettings: Output? = null,
public val securityGroups: Output>? = null,
public val spaceStorageSettings: Output? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sagemaker.inputs.DomainDefaultSpaceSettingsArgs =
com.pulumi.aws.sagemaker.inputs.DomainDefaultSpaceSettingsArgs.builder()
.customFileSystemConfigs(
customFileSystemConfigs?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.customPosixUserConfig(
customPosixUserConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.executionRole(executionRole.applyValue({ args0 -> args0 }))
.jupyterLabAppSettings(
jupyterLabAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.jupyterServerAppSettings(
jupyterServerAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.kernelGatewayAppSettings(
kernelGatewayAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.securityGroups(securityGroups?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.spaceStorageSettings(
spaceStorageSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DomainDefaultSpaceSettingsArgs].
*/
@PulumiTagMarker
public class DomainDefaultSpaceSettingsArgsBuilder internal constructor() {
private var customFileSystemConfigs:
Output>? = null
private var customPosixUserConfig: Output? =
null
private var executionRole: Output? = null
private var jupyterLabAppSettings: Output? =
null
private var jupyterServerAppSettings:
Output? = null
private var kernelGatewayAppSettings:
Output? = null
private var securityGroups: Output>? = null
private var spaceStorageSettings: Output? =
null
/**
* @param value The settings for assigning a custom file system to a user profile. Permitted users can access this file system in Amazon SageMaker Studio. See `custom_file_system_config` Block below.
*/
@JvmName("vqtukbclesqfulxk")
public suspend fun customFileSystemConfigs(`value`: Output>) {
this.customFileSystemConfigs = value
}
@JvmName("pynetrdkcuiopvbt")
public suspend fun customFileSystemConfigs(vararg values: Output) {
this.customFileSystemConfigs = Output.all(values.asList())
}
/**
* @param values The settings for assigning a custom file system to a user profile. Permitted users can access this file system in Amazon SageMaker Studio. See `custom_file_system_config` Block below.
*/
@JvmName("doargpdwnjasjlbn")
public suspend fun customFileSystemConfigs(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy