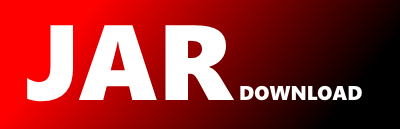
com.pulumi.aws.sagemaker.kotlin.inputs.EndpointDeploymentConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sagemaker.kotlin.inputs
import com.pulumi.aws.sagemaker.inputs.EndpointDeploymentConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property autoRollbackConfiguration Automatic rollback configuration for handling endpoint deployment failures and recovery. See Auto Rollback Configuration.
* @property blueGreenUpdatePolicy Update policy for a blue/green deployment. If this update policy is specified, SageMaker creates a new fleet during the deployment while maintaining the old fleet. SageMaker flips traffic to the new fleet according to the specified traffic routing configuration. Only one update policy should be used in the deployment configuration. If no update policy is specified, SageMaker uses a blue/green deployment strategy with all at once traffic shifting by default. See Blue Green Update Config.
* @property rollingUpdatePolicy Specifies a rolling deployment strategy for updating a SageMaker endpoint. See Rolling Update Policy.
*/
public data class EndpointDeploymentConfigArgs(
public val autoRollbackConfiguration: Output? = null,
public val blueGreenUpdatePolicy: Output? =
null,
public val rollingUpdatePolicy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sagemaker.inputs.EndpointDeploymentConfigArgs =
com.pulumi.aws.sagemaker.inputs.EndpointDeploymentConfigArgs.builder()
.autoRollbackConfiguration(
autoRollbackConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.blueGreenUpdatePolicy(
blueGreenUpdatePolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rollingUpdatePolicy(
rollingUpdatePolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [EndpointDeploymentConfigArgs].
*/
@PulumiTagMarker
public class EndpointDeploymentConfigArgsBuilder internal constructor() {
private var autoRollbackConfiguration:
Output? = null
private var blueGreenUpdatePolicy: Output? =
null
private var rollingUpdatePolicy: Output? = null
/**
* @param value Automatic rollback configuration for handling endpoint deployment failures and recovery. See Auto Rollback Configuration.
*/
@JvmName("vlfjksfajhnwhako")
public suspend fun autoRollbackConfiguration(`value`: Output) {
this.autoRollbackConfiguration = value
}
/**
* @param value Update policy for a blue/green deployment. If this update policy is specified, SageMaker creates a new fleet during the deployment while maintaining the old fleet. SageMaker flips traffic to the new fleet according to the specified traffic routing configuration. Only one update policy should be used in the deployment configuration. If no update policy is specified, SageMaker uses a blue/green deployment strategy with all at once traffic shifting by default. See Blue Green Update Config.
*/
@JvmName("akljdjqutculfqok")
public suspend fun blueGreenUpdatePolicy(`value`: Output) {
this.blueGreenUpdatePolicy = value
}
/**
* @param value Specifies a rolling deployment strategy for updating a SageMaker endpoint. See Rolling Update Policy.
*/
@JvmName("uphxsbelqvyhuibm")
public suspend fun rollingUpdatePolicy(`value`: Output) {
this.rollingUpdatePolicy = value
}
/**
* @param value Automatic rollback configuration for handling endpoint deployment failures and recovery. See Auto Rollback Configuration.
*/
@JvmName("wxxjiirqfjcaotub")
public suspend fun autoRollbackConfiguration(`value`: EndpointDeploymentConfigAutoRollbackConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoRollbackConfiguration = mapped
}
/**
* @param argument Automatic rollback configuration for handling endpoint deployment failures and recovery. See Auto Rollback Configuration.
*/
@JvmName("dfsyfwpylrulibaq")
public suspend fun autoRollbackConfiguration(argument: suspend EndpointDeploymentConfigAutoRollbackConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = EndpointDeploymentConfigAutoRollbackConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.autoRollbackConfiguration = mapped
}
/**
* @param value Update policy for a blue/green deployment. If this update policy is specified, SageMaker creates a new fleet during the deployment while maintaining the old fleet. SageMaker flips traffic to the new fleet according to the specified traffic routing configuration. Only one update policy should be used in the deployment configuration. If no update policy is specified, SageMaker uses a blue/green deployment strategy with all at once traffic shifting by default. See Blue Green Update Config.
*/
@JvmName("itefbwchoxmdeuxt")
public suspend fun blueGreenUpdatePolicy(`value`: EndpointDeploymentConfigBlueGreenUpdatePolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blueGreenUpdatePolicy = mapped
}
/**
* @param argument Update policy for a blue/green deployment. If this update policy is specified, SageMaker creates a new fleet during the deployment while maintaining the old fleet. SageMaker flips traffic to the new fleet according to the specified traffic routing configuration. Only one update policy should be used in the deployment configuration. If no update policy is specified, SageMaker uses a blue/green deployment strategy with all at once traffic shifting by default. See Blue Green Update Config.
*/
@JvmName("dgbydksgafbdttfk")
public suspend fun blueGreenUpdatePolicy(argument: suspend EndpointDeploymentConfigBlueGreenUpdatePolicyArgsBuilder.() -> Unit) {
val toBeMapped = EndpointDeploymentConfigBlueGreenUpdatePolicyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.blueGreenUpdatePolicy = mapped
}
/**
* @param value Specifies a rolling deployment strategy for updating a SageMaker endpoint. See Rolling Update Policy.
*/
@JvmName("enjpblwhxrjfaibd")
public suspend fun rollingUpdatePolicy(`value`: EndpointDeploymentConfigRollingUpdatePolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rollingUpdatePolicy = mapped
}
/**
* @param argument Specifies a rolling deployment strategy for updating a SageMaker endpoint. See Rolling Update Policy.
*/
@JvmName("xywmkqryehrqaqii")
public suspend fun rollingUpdatePolicy(argument: suspend EndpointDeploymentConfigRollingUpdatePolicyArgsBuilder.() -> Unit) {
val toBeMapped = EndpointDeploymentConfigRollingUpdatePolicyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rollingUpdatePolicy = mapped
}
internal fun build(): EndpointDeploymentConfigArgs = EndpointDeploymentConfigArgs(
autoRollbackConfiguration = autoRollbackConfiguration,
blueGreenUpdatePolicy = blueGreenUpdatePolicy,
rollingUpdatePolicy = rollingUpdatePolicy,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy