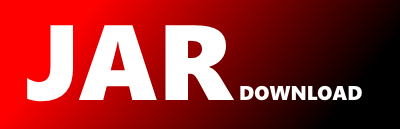
com.pulumi.aws.sagemaker.kotlin.inputs.EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sagemaker.kotlin.inputs
import com.pulumi.aws.sagemaker.inputs.EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property canarySize Batch size for the first step to turn on traffic on the new endpoint fleet. Value must be less than or equal to 50% of the variant's total instance count. See Canary Size.
* @property linearStepSize Batch size for each step to turn on traffic on the new endpoint fleet. Value must be 10-50% of the variant's total instance count. See Linear Step Size.
* @property type Traffic routing strategy type. Valid values are: `ALL_AT_ONCE`, `CANARY`, and `LINEAR`.
* @property waitIntervalInSeconds The waiting time (in seconds) between incremental steps to turn on traffic on the new endpoint fleet. Valid values are between `0` and `3600`.
*/
public data class EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs(
public val canarySize: Output? =
null,
public val linearStepSize: Output? =
null,
public val type: Output,
public val waitIntervalInSeconds: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sagemaker.inputs.EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs =
com.pulumi.aws.sagemaker.inputs.EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs.builder()
.canarySize(canarySize?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.linearStepSize(linearStepSize?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.type(type.applyValue({ args0 -> args0 }))
.waitIntervalInSeconds(waitIntervalInSeconds.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs].
*/
@PulumiTagMarker
public class EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgsBuilder
internal constructor() {
private var canarySize:
Output? =
null
private var linearStepSize:
Output? =
null
private var type: Output? = null
private var waitIntervalInSeconds: Output? = null
/**
* @param value Batch size for the first step to turn on traffic on the new endpoint fleet. Value must be less than or equal to 50% of the variant's total instance count. See Canary Size.
*/
@JvmName("mhwrarmskkxgvahp")
public suspend fun canarySize(`value`: Output) {
this.canarySize = value
}
/**
* @param value Batch size for each step to turn on traffic on the new endpoint fleet. Value must be 10-50% of the variant's total instance count. See Linear Step Size.
*/
@JvmName("jpgfyakbygmujsln")
public suspend fun linearStepSize(`value`: Output) {
this.linearStepSize = value
}
/**
* @param value Traffic routing strategy type. Valid values are: `ALL_AT_ONCE`, `CANARY`, and `LINEAR`.
*/
@JvmName("jgvpcbkvdambkysa")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The waiting time (in seconds) between incremental steps to turn on traffic on the new endpoint fleet. Valid values are between `0` and `3600`.
*/
@JvmName("lpnqcgkgvgmldfqo")
public suspend fun waitIntervalInSeconds(`value`: Output) {
this.waitIntervalInSeconds = value
}
/**
* @param value Batch size for the first step to turn on traffic on the new endpoint fleet. Value must be less than or equal to 50% of the variant's total instance count. See Canary Size.
*/
@JvmName("uvpetukxjwbntqxb")
public suspend fun canarySize(`value`: EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationCanarySizeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.canarySize = mapped
}
/**
* @param argument Batch size for the first step to turn on traffic on the new endpoint fleet. Value must be less than or equal to 50% of the variant's total instance count. See Canary Size.
*/
@JvmName("hahknmrmnmlugsxo")
public suspend fun canarySize(argument: suspend EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationCanarySizeArgsBuilder.() -> Unit) {
val toBeMapped =
EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationCanarySizeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.canarySize = mapped
}
/**
* @param value Batch size for each step to turn on traffic on the new endpoint fleet. Value must be 10-50% of the variant's total instance count. See Linear Step Size.
*/
@JvmName("kwjhmgegtxhximnx")
public suspend fun linearStepSize(`value`: EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationLinearStepSizeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linearStepSize = mapped
}
/**
* @param argument Batch size for each step to turn on traffic on the new endpoint fleet. Value must be 10-50% of the variant's total instance count. See Linear Step Size.
*/
@JvmName("bdubmcxfpukpfgfj")
public suspend fun linearStepSize(argument: suspend EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationLinearStepSizeArgsBuilder.() -> Unit) {
val toBeMapped =
EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationLinearStepSizeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.linearStepSize = mapped
}
/**
* @param value Traffic routing strategy type. Valid values are: `ALL_AT_ONCE`, `CANARY`, and `LINEAR`.
*/
@JvmName("owrfhwskjmdjwdsf")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value The waiting time (in seconds) between incremental steps to turn on traffic on the new endpoint fleet. Valid values are between `0` and `3600`.
*/
@JvmName("ngnmtrojamcisjdc")
public suspend fun waitIntervalInSeconds(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.waitIntervalInSeconds = mapped
}
internal fun build(): EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs = EndpointDeploymentConfigBlueGreenUpdatePolicyTrafficRoutingConfigurationArgs(
canarySize = canarySize,
linearStepSize = linearStepSize,
type = type ?: throw PulumiNullFieldException("type"),
waitIntervalInSeconds = waitIntervalInSeconds ?: throw
PulumiNullFieldException("waitIntervalInSeconds"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy