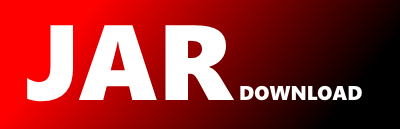
com.pulumi.aws.sagemaker.kotlin.inputs.UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.sagemaker.kotlin.inputs
import com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property assumableRoleArns An array of Amazon Resource Names (ARNs) of the IAM roles that the execution role of SageMaker can assume for performing operations or tasks related to Amazon EMR clusters or Amazon EMR Serverless applications. These roles define the permissions and access policies required when performing Amazon EMR-related operations, such as listing, connecting to, or terminating Amazon EMR clusters or Amazon EMR Serverless applications. They are typically used in cross-account access scenarios, where the Amazon EMR resources (clusters or serverless applications) are located in a different AWS account than the SageMaker domain.
* @property executionRoleArns An array of Amazon Resource Names (ARNs) of the IAM roles used by the Amazon EMR cluster instances or job execution environments to access other AWS services and resources needed during the runtime of your Amazon EMR or Amazon EMR Serverless workloads, such as Amazon S3 for data access, Amazon CloudWatch for logging, or other AWS services based on the particular workload requirements.
*/
public data class UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgs(
public val assumableRoleArns: Output>? = null,
public val executionRoleArns: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgs =
com.pulumi.aws.sagemaker.inputs.UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgs.builder()
.assumableRoleArns(assumableRoleArns?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.executionRoleArns(executionRoleArns?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgs].
*/
@PulumiTagMarker
public class UserProfileUserSettingsJupyterLabAppSettingsEmrSettingsArgsBuilder internal constructor() {
private var assumableRoleArns: Output>? = null
private var executionRoleArns: Output>? = null
/**
* @param value An array of Amazon Resource Names (ARNs) of the IAM roles that the execution role of SageMaker can assume for performing operations or tasks related to Amazon EMR clusters or Amazon EMR Serverless applications. These roles define the permissions and access policies required when performing Amazon EMR-related operations, such as listing, connecting to, or terminating Amazon EMR clusters or Amazon EMR Serverless applications. They are typically used in cross-account access scenarios, where the Amazon EMR resources (clusters or serverless applications) are located in a different AWS account than the SageMaker domain.
*/
@JvmName("qknjumhgbymmmcte")
public suspend fun assumableRoleArns(`value`: Output>) {
this.assumableRoleArns = value
}
@JvmName("ilwvletqiypkvsmm")
public suspend fun assumableRoleArns(vararg values: Output) {
this.assumableRoleArns = Output.all(values.asList())
}
/**
* @param values An array of Amazon Resource Names (ARNs) of the IAM roles that the execution role of SageMaker can assume for performing operations or tasks related to Amazon EMR clusters or Amazon EMR Serverless applications. These roles define the permissions and access policies required when performing Amazon EMR-related operations, such as listing, connecting to, or terminating Amazon EMR clusters or Amazon EMR Serverless applications. They are typically used in cross-account access scenarios, where the Amazon EMR resources (clusters or serverless applications) are located in a different AWS account than the SageMaker domain.
*/
@JvmName("ttjcxyondlliaejr")
public suspend fun assumableRoleArns(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy