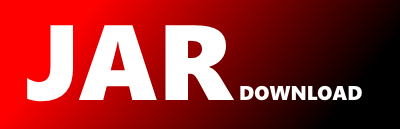
com.pulumi.aws.scheduler.kotlin.ScheduleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.scheduler.kotlin
import com.pulumi.aws.scheduler.ScheduleArgs.builder
import com.pulumi.aws.scheduler.kotlin.inputs.ScheduleFlexibleTimeWindowArgs
import com.pulumi.aws.scheduler.kotlin.inputs.ScheduleFlexibleTimeWindowArgsBuilder
import com.pulumi.aws.scheduler.kotlin.inputs.ScheduleTargetArgs
import com.pulumi.aws.scheduler.kotlin.inputs.ScheduleTargetArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Provides an EventBridge Scheduler Schedule resource.
* You can find out more about EventBridge Scheduler in the [User Guide](https://docs.aws.amazon.com/scheduler/latest/UserGuide/what-is-scheduler.html).
* > **Note:** EventBridge was formerly known as CloudWatch Events. The functionality is identical.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.scheduler.Schedule("example", {
* name: "my-schedule",
* groupName: "default",
* flexibleTimeWindow: {
* mode: "OFF",
* },
* scheduleExpression: "rate(1 hours)",
* target: {
* arn: exampleAwsSqsQueue.arn,
* roleArn: exampleAwsIamRole.arn,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.scheduler.Schedule("example",
* name="my-schedule",
* group_name="default",
* flexible_time_window={
* "mode": "OFF",
* },
* schedule_expression="rate(1 hours)",
* target={
* "arn": example_aws_sqs_queue["arn"],
* "role_arn": example_aws_iam_role["arn"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Scheduler.Schedule("example", new()
* {
* Name = "my-schedule",
* GroupName = "default",
* FlexibleTimeWindow = new Aws.Scheduler.Inputs.ScheduleFlexibleTimeWindowArgs
* {
* Mode = "OFF",
* },
* ScheduleExpression = "rate(1 hours)",
* Target = new Aws.Scheduler.Inputs.ScheduleTargetArgs
* {
* Arn = exampleAwsSqsQueue.Arn,
* RoleArn = exampleAwsIamRole.Arn,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/scheduler"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := scheduler.NewSchedule(ctx, "example", &scheduler.ScheduleArgs{
* Name: pulumi.String("my-schedule"),
* GroupName: pulumi.String("default"),
* FlexibleTimeWindow: &scheduler.ScheduleFlexibleTimeWindowArgs{
* Mode: pulumi.String("OFF"),
* },
* ScheduleExpression: pulumi.String("rate(1 hours)"),
* Target: &scheduler.ScheduleTargetArgs{
* Arn: pulumi.Any(exampleAwsSqsQueue.Arn),
* RoleArn: pulumi.Any(exampleAwsIamRole.Arn),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.scheduler.Schedule;
* import com.pulumi.aws.scheduler.ScheduleArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleFlexibleTimeWindowArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Schedule("example", ScheduleArgs.builder()
* .name("my-schedule")
* .groupName("default")
* .flexibleTimeWindow(ScheduleFlexibleTimeWindowArgs.builder()
* .mode("OFF")
* .build())
* .scheduleExpression("rate(1 hours)")
* .target(ScheduleTargetArgs.builder()
* .arn(exampleAwsSqsQueue.arn())
* .roleArn(exampleAwsIamRole.arn())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:scheduler:Schedule
* properties:
* name: my-schedule
* groupName: default
* flexibleTimeWindow:
* mode: OFF
* scheduleExpression: rate(1 hours)
* target:
* arn: ${exampleAwsSqsQueue.arn}
* roleArn: ${exampleAwsIamRole.arn}
* ```
*
* ### Universal Target
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.sqs.Queue("example", {});
* const exampleSchedule = new aws.scheduler.Schedule("example", {
* name: "my-schedule",
* flexibleTimeWindow: {
* mode: "OFF",
* },
* scheduleExpression: "rate(1 hours)",
* target: {
* arn: "arn:aws:scheduler:::aws-sdk:sqs:sendMessage",
* roleArn: exampleAwsIamRole.arn,
* input: pulumi.jsonStringify({
* MessageBody: "Greetings, programs!",
* QueueUrl: example.url,
* }),
* },
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* example = aws.sqs.Queue("example")
* example_schedule = aws.scheduler.Schedule("example",
* name="my-schedule",
* flexible_time_window={
* "mode": "OFF",
* },
* schedule_expression="rate(1 hours)",
* target={
* "arn": "arn:aws:scheduler:::aws-sdk:sqs:sendMessage",
* "role_arn": example_aws_iam_role["arn"],
* "input": pulumi.Output.json_dumps({
* "MessageBody": "Greetings, programs!",
* "QueueUrl": example.url,
* }),
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Sqs.Queue("example");
* var exampleSchedule = new Aws.Scheduler.Schedule("example", new()
* {
* Name = "my-schedule",
* FlexibleTimeWindow = new Aws.Scheduler.Inputs.ScheduleFlexibleTimeWindowArgs
* {
* Mode = "OFF",
* },
* ScheduleExpression = "rate(1 hours)",
* Target = new Aws.Scheduler.Inputs.ScheduleTargetArgs
* {
* Arn = "arn:aws:scheduler:::aws-sdk:sqs:sendMessage",
* RoleArn = exampleAwsIamRole.Arn,
* Input = Output.JsonSerialize(Output.Create(new Dictionary
* {
* ["MessageBody"] = "Greetings, programs!",
* ["QueueUrl"] = example.Url,
* })),
* },
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/scheduler"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sqs"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := sqs.NewQueue(ctx, "example", nil)
* if err != nil {
* return err
* }
* _, err = scheduler.NewSchedule(ctx, "example", &scheduler.ScheduleArgs{
* Name: pulumi.String("my-schedule"),
* FlexibleTimeWindow: &scheduler.ScheduleFlexibleTimeWindowArgs{
* Mode: pulumi.String("OFF"),
* },
* ScheduleExpression: pulumi.String("rate(1 hours)"),
* Target: &scheduler.ScheduleTargetArgs{
* Arn: pulumi.String("arn:aws:scheduler:::aws-sdk:sqs:sendMessage"),
* RoleArn: pulumi.Any(exampleAwsIamRole.Arn),
* Input: example.Url.ApplyT(func(url string) (pulumi.String, error) {
* var _zero pulumi.String
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "MessageBody": "Greetings, programs!",
* "QueueUrl": url,
* })
* if err != nil {
* return _zero, err
* }
* json0 := string(tmpJSON0)
* return pulumi.String(json0), nil
* }).(pulumi.StringOutput),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sqs.Queue;
* import com.pulumi.aws.scheduler.Schedule;
* import com.pulumi.aws.scheduler.ScheduleArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleFlexibleTimeWindowArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Queue("example");
* var exampleSchedule = new Schedule("exampleSchedule", ScheduleArgs.builder()
* .name("my-schedule")
* .flexibleTimeWindow(ScheduleFlexibleTimeWindowArgs.builder()
* .mode("OFF")
* .build())
* .scheduleExpression("rate(1 hours)")
* .target(ScheduleTargetArgs.builder()
* .arn("arn:aws:scheduler:::aws-sdk:sqs:sendMessage")
* .roleArn(exampleAwsIamRole.arn())
* .input(example.url().applyValue(url -> serializeJson(
* jsonObject(
* jsonProperty("MessageBody", "Greetings, programs!"),
* jsonProperty("QueueUrl", url)
* ))))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:sqs:Queue
* exampleSchedule:
* type: aws:scheduler:Schedule
* name: example
* properties:
* name: my-schedule
* flexibleTimeWindow:
* mode: OFF
* scheduleExpression: rate(1 hours)
* target:
* arn: arn:aws:scheduler:::aws-sdk:sqs:sendMessage
* roleArn: ${exampleAwsIamRole.arn}
* input:
* fn::toJSON:
* MessageBody: Greetings, programs!
* QueueUrl: ${example.url}
* ```
*
* ## Import
* Using `pulumi import`, import schedules using the combination `group_name/name`. For example:
* ```sh
* $ pulumi import aws:scheduler/schedule:Schedule example my-schedule-group/my-schedule
* ```
* @property description Brief description of the schedule.
* @property endDate The date, in UTC, before which the schedule can invoke its target. Depending on the schedule's recurrence expression, invocations might stop on, or before, the end date you specify. EventBridge Scheduler ignores the end date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
* @property flexibleTimeWindow Configures a time window during which EventBridge Scheduler invokes the schedule. Detailed below.
* @property groupName Name of the schedule group to associate with this schedule. When omitted, the `default` schedule group is used.
* @property kmsKeyArn ARN for the customer managed KMS key that EventBridge Scheduler will use to encrypt and decrypt your data.
* @property name Name of the schedule. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
* @property namePrefix Creates a unique name beginning with the specified prefix. Conflicts with `name`.
* @property scheduleExpression Defines when the schedule runs. Read more in [Schedule types on EventBridge Scheduler](https://docs.aws.amazon.com/scheduler/latest/UserGuide/schedule-types.html).
* @property scheduleExpressionTimezone Timezone in which the scheduling expression is evaluated. Defaults to `UTC`. Example: `Australia/Sydney`.
* @property startDate The date, in UTC, after which the schedule can begin invoking its target. Depending on the schedule's recurrence expression, invocations might occur on, or after, the start date you specify. EventBridge Scheduler ignores the start date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
* @property state Specifies whether the schedule is enabled or disabled. One of: `ENABLED` (default), `DISABLED`.
* @property target Configures the target of the schedule. Detailed below.
* The following arguments are optional:
*/
public data class ScheduleArgs(
public val description: Output? = null,
public val endDate: Output? = null,
public val flexibleTimeWindow: Output? = null,
public val groupName: Output? = null,
public val kmsKeyArn: Output? = null,
public val name: Output? = null,
public val namePrefix: Output? = null,
public val scheduleExpression: Output? = null,
public val scheduleExpressionTimezone: Output? = null,
public val startDate: Output? = null,
public val state: Output? = null,
public val target: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.scheduler.ScheduleArgs =
com.pulumi.aws.scheduler.ScheduleArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.endDate(endDate?.applyValue({ args0 -> args0 }))
.flexibleTimeWindow(
flexibleTimeWindow?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.groupName(groupName?.applyValue({ args0 -> args0 }))
.kmsKeyArn(kmsKeyArn?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namePrefix(namePrefix?.applyValue({ args0 -> args0 }))
.scheduleExpression(scheduleExpression?.applyValue({ args0 -> args0 }))
.scheduleExpressionTimezone(scheduleExpressionTimezone?.applyValue({ args0 -> args0 }))
.startDate(startDate?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0 }))
.target(target?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ScheduleArgs].
*/
@PulumiTagMarker
public class ScheduleArgsBuilder internal constructor() {
private var description: Output? = null
private var endDate: Output? = null
private var flexibleTimeWindow: Output? = null
private var groupName: Output? = null
private var kmsKeyArn: Output? = null
private var name: Output? = null
private var namePrefix: Output? = null
private var scheduleExpression: Output? = null
private var scheduleExpressionTimezone: Output? = null
private var startDate: Output? = null
private var state: Output? = null
private var target: Output? = null
/**
* @param value Brief description of the schedule.
*/
@JvmName("ocwlgvtbbqlfbkuy")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The date, in UTC, before which the schedule can invoke its target. Depending on the schedule's recurrence expression, invocations might stop on, or before, the end date you specify. EventBridge Scheduler ignores the end date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*/
@JvmName("jrnshieppsexeeip")
public suspend fun endDate(`value`: Output) {
this.endDate = value
}
/**
* @param value Configures a time window during which EventBridge Scheduler invokes the schedule. Detailed below.
*/
@JvmName("rkuduorfjwdiawqm")
public suspend fun flexibleTimeWindow(`value`: Output) {
this.flexibleTimeWindow = value
}
/**
* @param value Name of the schedule group to associate with this schedule. When omitted, the `default` schedule group is used.
*/
@JvmName("gxvdogubopxcpsdi")
public suspend fun groupName(`value`: Output) {
this.groupName = value
}
/**
* @param value ARN for the customer managed KMS key that EventBridge Scheduler will use to encrypt and decrypt your data.
*/
@JvmName("cstffxswyehgibca")
public suspend fun kmsKeyArn(`value`: Output) {
this.kmsKeyArn = value
}
/**
* @param value Name of the schedule. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*/
@JvmName("ljuletknueplnsdj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*/
@JvmName("irvhxgbsogkqoyeo")
public suspend fun namePrefix(`value`: Output) {
this.namePrefix = value
}
/**
* @param value Defines when the schedule runs. Read more in [Schedule types on EventBridge Scheduler](https://docs.aws.amazon.com/scheduler/latest/UserGuide/schedule-types.html).
*/
@JvmName("vupnwewemwmwyrsx")
public suspend fun scheduleExpression(`value`: Output) {
this.scheduleExpression = value
}
/**
* @param value Timezone in which the scheduling expression is evaluated. Defaults to `UTC`. Example: `Australia/Sydney`.
*/
@JvmName("tkddrttpcwnkajur")
public suspend fun scheduleExpressionTimezone(`value`: Output) {
this.scheduleExpressionTimezone = value
}
/**
* @param value The date, in UTC, after which the schedule can begin invoking its target. Depending on the schedule's recurrence expression, invocations might occur on, or after, the start date you specify. EventBridge Scheduler ignores the start date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*/
@JvmName("fpsvwiltgvyoekvw")
public suspend fun startDate(`value`: Output) {
this.startDate = value
}
/**
* @param value Specifies whether the schedule is enabled or disabled. One of: `ENABLED` (default), `DISABLED`.
*/
@JvmName("vojmfsdnkdombhaf")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value Configures the target of the schedule. Detailed below.
* The following arguments are optional:
*/
@JvmName("tnbeforfgeflgeii")
public suspend fun target(`value`: Output) {
this.target = value
}
/**
* @param value Brief description of the schedule.
*/
@JvmName("nrdfeehjvhjshyfe")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The date, in UTC, before which the schedule can invoke its target. Depending on the schedule's recurrence expression, invocations might stop on, or before, the end date you specify. EventBridge Scheduler ignores the end date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*/
@JvmName("amxfaiuhvwyeaihn")
public suspend fun endDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endDate = mapped
}
/**
* @param value Configures a time window during which EventBridge Scheduler invokes the schedule. Detailed below.
*/
@JvmName("oenroclirgmbylyj")
public suspend fun flexibleTimeWindow(`value`: ScheduleFlexibleTimeWindowArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.flexibleTimeWindow = mapped
}
/**
* @param argument Configures a time window during which EventBridge Scheduler invokes the schedule. Detailed below.
*/
@JvmName("cdgbrjhbfnkgcgyx")
public suspend fun flexibleTimeWindow(argument: suspend ScheduleFlexibleTimeWindowArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleFlexibleTimeWindowArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.flexibleTimeWindow = mapped
}
/**
* @param value Name of the schedule group to associate with this schedule. When omitted, the `default` schedule group is used.
*/
@JvmName("gfgxvuwksnnuxmfs")
public suspend fun groupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupName = mapped
}
/**
* @param value ARN for the customer managed KMS key that EventBridge Scheduler will use to encrypt and decrypt your data.
*/
@JvmName("oapdwqgyaliiitfd")
public suspend fun kmsKeyArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyArn = mapped
}
/**
* @param value Name of the schedule. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*/
@JvmName("pqeenljruijlmrcy")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*/
@JvmName("akiforlcnhwqromi")
public suspend fun namePrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namePrefix = mapped
}
/**
* @param value Defines when the schedule runs. Read more in [Schedule types on EventBridge Scheduler](https://docs.aws.amazon.com/scheduler/latest/UserGuide/schedule-types.html).
*/
@JvmName("kknbtjjnvscjrjmf")
public suspend fun scheduleExpression(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduleExpression = mapped
}
/**
* @param value Timezone in which the scheduling expression is evaluated. Defaults to `UTC`. Example: `Australia/Sydney`.
*/
@JvmName("nodcauxlprxfuhux")
public suspend fun scheduleExpressionTimezone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduleExpressionTimezone = mapped
}
/**
* @param value The date, in UTC, after which the schedule can begin invoking its target. Depending on the schedule's recurrence expression, invocations might occur on, or after, the start date you specify. EventBridge Scheduler ignores the start date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*/
@JvmName("msnpspydcantiwog")
public suspend fun startDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startDate = mapped
}
/**
* @param value Specifies whether the schedule is enabled or disabled. One of: `ENABLED` (default), `DISABLED`.
*/
@JvmName("vrmxyimbefivkpep")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value Configures the target of the schedule. Detailed below.
* The following arguments are optional:
*/
@JvmName("uvcxjkojvhgnsclp")
public suspend fun target(`value`: ScheduleTargetArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.target = mapped
}
/**
* @param argument Configures the target of the schedule. Detailed below.
* The following arguments are optional:
*/
@JvmName("orxhtacyyjqadrfq")
public suspend fun target(argument: suspend ScheduleTargetArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.target = mapped
}
internal fun build(): ScheduleArgs = ScheduleArgs(
description = description,
endDate = endDate,
flexibleTimeWindow = flexibleTimeWindow,
groupName = groupName,
kmsKeyArn = kmsKeyArn,
name = name,
namePrefix = namePrefix,
scheduleExpression = scheduleExpression,
scheduleExpressionTimezone = scheduleExpressionTimezone,
startDate = startDate,
state = state,
target = target,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy