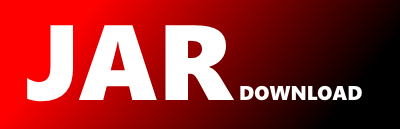
com.pulumi.aws.scheduler.kotlin.inputs.ScheduleTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.scheduler.kotlin.inputs
import com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property arn ARN of the target of this schedule, such as a SQS queue or ECS cluster. For universal targets, this is a [Service ARN specific to the target service](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html#supported-universal-targets).
* @property deadLetterConfig Information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue. Detailed below.
* @property ecsParameters Templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation. Detailed below.
* @property eventbridgeParameters Templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation. Detailed below.
* @property input Text, or well-formed JSON, passed to the target. Read more in [Universal target](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html).
* @property kinesisParameters Templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation. Detailed below.
* @property retryPolicy Information about the retry policy settings. Detailed below.
* @property roleArn ARN of the IAM role that EventBridge Scheduler will use for this target when the schedule is invoked. Read more in [Set up the execution role](https://docs.aws.amazon.com/scheduler/latest/UserGuide/setting-up.html#setting-up-execution-role).
* The following arguments are optional:
* @property sagemakerPipelineParameters Templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation. Detailed below.
* @property sqsParameters The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Detailed below.
*/
public data class ScheduleTargetArgs(
public val arn: Output,
public val deadLetterConfig: Output? = null,
public val ecsParameters: Output? = null,
public val eventbridgeParameters: Output? = null,
public val input: Output? = null,
public val kinesisParameters: Output? = null,
public val retryPolicy: Output? = null,
public val roleArn: Output,
public val sagemakerPipelineParameters: Output? =
null,
public val sqsParameters: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs =
com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs.builder()
.arn(arn.applyValue({ args0 -> args0 }))
.deadLetterConfig(deadLetterConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ecsParameters(ecsParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.eventbridgeParameters(
eventbridgeParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.input(input?.applyValue({ args0 -> args0 }))
.kinesisParameters(kinesisParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retryPolicy(retryPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.sagemakerPipelineParameters(
sagemakerPipelineParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sqsParameters(sqsParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ScheduleTargetArgs].
*/
@PulumiTagMarker
public class ScheduleTargetArgsBuilder internal constructor() {
private var arn: Output? = null
private var deadLetterConfig: Output? = null
private var ecsParameters: Output? = null
private var eventbridgeParameters: Output? = null
private var input: Output? = null
private var kinesisParameters: Output? = null
private var retryPolicy: Output? = null
private var roleArn: Output? = null
private var sagemakerPipelineParameters: Output? =
null
private var sqsParameters: Output? = null
/**
* @param value ARN of the target of this schedule, such as a SQS queue or ECS cluster. For universal targets, this is a [Service ARN specific to the target service](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html#supported-universal-targets).
*/
@JvmName("urnrkkxjrucqkyyk")
public suspend fun arn(`value`: Output) {
this.arn = value
}
/**
* @param value Information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue. Detailed below.
*/
@JvmName("evcjniyturfwylcy")
public suspend fun deadLetterConfig(`value`: Output) {
this.deadLetterConfig = value
}
/**
* @param value Templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation. Detailed below.
*/
@JvmName("nyfmufnebqxlvbsn")
public suspend fun ecsParameters(`value`: Output) {
this.ecsParameters = value
}
/**
* @param value Templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation. Detailed below.
*/
@JvmName("slbrtoguefnmgobc")
public suspend fun eventbridgeParameters(`value`: Output) {
this.eventbridgeParameters = value
}
/**
* @param value Text, or well-formed JSON, passed to the target. Read more in [Universal target](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html).
*/
@JvmName("vdhwnqrqjlmjopxt")
public suspend fun input(`value`: Output) {
this.input = value
}
/**
* @param value Templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation. Detailed below.
*/
@JvmName("xrmptvccnjltsdsg")
public suspend fun kinesisParameters(`value`: Output) {
this.kinesisParameters = value
}
/**
* @param value Information about the retry policy settings. Detailed below.
*/
@JvmName("pkvdaavgfocnrwgh")
public suspend fun retryPolicy(`value`: Output) {
this.retryPolicy = value
}
/**
* @param value ARN of the IAM role that EventBridge Scheduler will use for this target when the schedule is invoked. Read more in [Set up the execution role](https://docs.aws.amazon.com/scheduler/latest/UserGuide/setting-up.html#setting-up-execution-role).
* The following arguments are optional:
*/
@JvmName("ammkwxihvxkvuavu")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value Templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation. Detailed below.
*/
@JvmName("niuftexerhtoouen")
public suspend fun sagemakerPipelineParameters(`value`: Output) {
this.sagemakerPipelineParameters = value
}
/**
* @param value The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Detailed below.
*/
@JvmName("qgiogqhtdmjhvvrn")
public suspend fun sqsParameters(`value`: Output) {
this.sqsParameters = value
}
/**
* @param value ARN of the target of this schedule, such as a SQS queue or ECS cluster. For universal targets, this is a [Service ARN specific to the target service](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html#supported-universal-targets).
*/
@JvmName("nvmxllwhgmoxsvhy")
public suspend fun arn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.arn = mapped
}
/**
* @param value Information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue. Detailed below.
*/
@JvmName("xkpirdwsqdqyrfyr")
public suspend fun deadLetterConfig(`value`: ScheduleTargetDeadLetterConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadLetterConfig = mapped
}
/**
* @param argument Information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue. Detailed below.
*/
@JvmName("mholmqkqdvdmwfpe")
public suspend fun deadLetterConfig(argument: suspend ScheduleTargetDeadLetterConfigArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetDeadLetterConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.deadLetterConfig = mapped
}
/**
* @param value Templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation. Detailed below.
*/
@JvmName("fqqcxavsqlabcrfg")
public suspend fun ecsParameters(`value`: ScheduleTargetEcsParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ecsParameters = mapped
}
/**
* @param argument Templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation. Detailed below.
*/
@JvmName("dmmcxvsoadrdbuma")
public suspend fun ecsParameters(argument: suspend ScheduleTargetEcsParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetEcsParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ecsParameters = mapped
}
/**
* @param value Templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation. Detailed below.
*/
@JvmName("gqkmgweafcgltuvu")
public suspend fun eventbridgeParameters(`value`: ScheduleTargetEventbridgeParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventbridgeParameters = mapped
}
/**
* @param argument Templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation. Detailed below.
*/
@JvmName("fauclppiyqclmgku")
public suspend fun eventbridgeParameters(argument: suspend ScheduleTargetEventbridgeParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetEventbridgeParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.eventbridgeParameters = mapped
}
/**
* @param value Text, or well-formed JSON, passed to the target. Read more in [Universal target](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html).
*/
@JvmName("tmnxbiekdgvxgset")
public suspend fun input(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.input = mapped
}
/**
* @param value Templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation. Detailed below.
*/
@JvmName("caryrpmpqtjjagcf")
public suspend fun kinesisParameters(`value`: ScheduleTargetKinesisParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisParameters = mapped
}
/**
* @param argument Templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation. Detailed below.
*/
@JvmName("pwrcossbrakejfok")
public suspend fun kinesisParameters(argument: suspend ScheduleTargetKinesisParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetKinesisParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.kinesisParameters = mapped
}
/**
* @param value Information about the retry policy settings. Detailed below.
*/
@JvmName("auoxbydkhisjniym")
public suspend fun retryPolicy(`value`: ScheduleTargetRetryPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryPolicy = mapped
}
/**
* @param argument Information about the retry policy settings. Detailed below.
*/
@JvmName("qxiqjjxnvpemublx")
public suspend fun retryPolicy(argument: suspend ScheduleTargetRetryPolicyArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetRetryPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.retryPolicy = mapped
}
/**
* @param value ARN of the IAM role that EventBridge Scheduler will use for this target when the schedule is invoked. Read more in [Set up the execution role](https://docs.aws.amazon.com/scheduler/latest/UserGuide/setting-up.html#setting-up-execution-role).
* The following arguments are optional:
*/
@JvmName("gwodvrgontumlcjf")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value Templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation. Detailed below.
*/
@JvmName("spisalirhyafysoe")
public suspend fun sagemakerPipelineParameters(`value`: ScheduleTargetSagemakerPipelineParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sagemakerPipelineParameters = mapped
}
/**
* @param argument Templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation. Detailed below.
*/
@JvmName("splftjxchnehvymd")
public suspend fun sagemakerPipelineParameters(argument: suspend ScheduleTargetSagemakerPipelineParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetSagemakerPipelineParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sagemakerPipelineParameters = mapped
}
/**
* @param value The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Detailed below.
*/
@JvmName("nkpstikyypibeohh")
public suspend fun sqsParameters(`value`: ScheduleTargetSqsParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqsParameters = mapped
}
/**
* @param argument The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Detailed below.
*/
@JvmName("hxirnbfawujvixsc")
public suspend fun sqsParameters(argument: suspend ScheduleTargetSqsParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleTargetSqsParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sqsParameters = mapped
}
internal fun build(): ScheduleTargetArgs = ScheduleTargetArgs(
arn = arn ?: throw PulumiNullFieldException("arn"),
deadLetterConfig = deadLetterConfig,
ecsParameters = ecsParameters,
eventbridgeParameters = eventbridgeParameters,
input = input,
kinesisParameters = kinesisParameters,
retryPolicy = retryPolicy,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
sagemakerPipelineParameters = sagemakerPipelineParameters,
sqsParameters = sqsParameters,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy