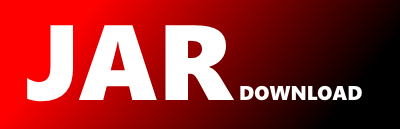
com.pulumi.aws.secretsmanager.kotlin.SecretVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.secretsmanager.kotlin
import com.pulumi.aws.secretsmanager.SecretVersionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a resource to manage AWS Secrets Manager secret version including its secret value. To manage secret metadata, see the `aws.secretsmanager.Secret` resource.
* > **NOTE:** If the `AWSCURRENT` staging label is present on this version during resource deletion, that label cannot be removed and will be skipped to prevent errors when fully deleting the secret. That label will leave this secret version active even after the resource is deleted from this provider unless the secret itself is deleted. Move the `AWSCURRENT` staging label before or after deleting this resource from this provider to fully trigger version deprecation if necessary.
* ## Example Usage
* ### Simple String Value
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.secretsmanager.SecretVersion("example", {
* secretId: exampleAwsSecretsmanagerSecret.id,
* secretString: "example-string-to-protect",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.secretsmanager.SecretVersion("example",
* secret_id=example_aws_secretsmanager_secret["id"],
* secret_string="example-string-to-protect")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.SecretsManager.SecretVersion("example", new()
* {
* SecretId = exampleAwsSecretsmanagerSecret.Id,
* SecretString = "example-string-to-protect",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/secretsmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := secretsmanager.NewSecretVersion(ctx, "example", &secretsmanager.SecretVersionArgs{
* SecretId: pulumi.Any(exampleAwsSecretsmanagerSecret.Id),
* SecretString: pulumi.String("example-string-to-protect"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.secretsmanager.SecretVersion;
* import com.pulumi.aws.secretsmanager.SecretVersionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new SecretVersion("example", SecretVersionArgs.builder()
* .secretId(exampleAwsSecretsmanagerSecret.id())
* .secretString("example-string-to-protect")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:secretsmanager:SecretVersion
* properties:
* secretId: ${exampleAwsSecretsmanagerSecret.id}
* secretString: example-string-to-protect
* ```
*
* ### Key-Value Pairs
* Secrets Manager also accepts key-value pairs in JSON.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const config = new pulumi.Config();
* const example = config.getObject>("example") || {
* key1: "value1",
* key2: "value2",
* };
* const exampleSecretVersion = new aws.secretsmanager.SecretVersion("example", {
* secretId: exampleAwsSecretsmanagerSecret.id,
* secretString: JSON.stringify(example),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* config = pulumi.Config()
* example = config.get_object("example")
* if example is None:
* example = {
* "key1": "value1",
* "key2": "value2",
* }
* example_secret_version = aws.secretsmanager.SecretVersion("example",
* secret_id=example_aws_secretsmanager_secret["id"],
* secret_string=json.dumps(example))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var config = new Config();
* var example = config.GetObject>("example") ??
* {
* { "key1", "value1" },
* { "key2", "value2" },
* };
* var exampleSecretVersion = new Aws.SecretsManager.SecretVersion("example", new()
* {
* SecretId = exampleAwsSecretsmanagerSecret.Id,
* SecretString = JsonSerializer.Serialize(example),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/secretsmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi/config"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* cfg := config.New(ctx, "")
* example := map[string]interface{}{
* "key1": "value1",
* "key2": "value2",
* }
* if param := cfg.GetObject("example"); param != nil {
* example = param
* }
* tmpJSON0, err := json.Marshal(example)
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = secretsmanager.NewSecretVersion(ctx, "example", &secretsmanager.SecretVersionArgs{
* SecretId: pulumi.Any(exampleAwsSecretsmanagerSecret.Id),
* SecretString: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.secretsmanager.SecretVersion;
* import com.pulumi.aws.secretsmanager.SecretVersionArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var example = config.get("example").orElse(%!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference));
* var exampleSecretVersion = new SecretVersion("exampleSecretVersion", SecretVersionArgs.builder()
* .secretId(exampleAwsSecretsmanagerSecret.id())
* .secretString(serializeJson(
* example))
* .build());
* }
* }
* ```
* ```yaml
* configuration:
* # The map here can come from other supported configurations
* # like locals, resource attribute, map() built-in, etc.
* example:
* type: map(string)
* default:
* key1: value1
* key2: value2
* resources:
* exampleSecretVersion:
* type: aws:secretsmanager:SecretVersion
* name: example
* properties:
* secretId: ${exampleAwsSecretsmanagerSecret.id}
* secretString:
* fn::toJSON: ${example}
* ```
*
* Reading key-value pairs from JSON back into a native map
* ## Import
* Using `pulumi import`, import `aws_secretsmanager_secret_version` using the secret ID and version ID. For example:
* ```sh
* $ pulumi import aws:secretsmanager/secretVersion:SecretVersion example 'arn:aws:secretsmanager:us-east-1:123456789012:secret:example-123456|xxxxx-xxxxxxx-xxxxxxx-xxxxx'
* ```
* @property secretBinary Specifies binary data that you want to encrypt and store in this version of the secret. This is required if `secret_string` is not set. Needs to be encoded to base64.
* @property secretId Specifies the secret to which you want to add a new version. You can specify either the Amazon Resource Name (ARN) or the friendly name of the secret. The secret must already exist.
* @property secretString Specifies text data that you want to encrypt and store in this version of the secret. This is required if `secret_binary` is not set.
* @property versionStages Specifies a list of staging labels that are attached to this version of the secret. A staging label must be unique to a single version of the secret. If you specify a staging label that's already associated with a different version of the same secret then that staging label is automatically removed from the other version and attached to this version. If you do not specify a value, then AWS Secrets Manager automatically moves the staging label `AWSCURRENT` to this new version on creation.
* > **NOTE:** If `version_stages` is configured, you must include the `AWSCURRENT` staging label if this secret version is the only version or if the label is currently present on this secret version, otherwise this provider will show a perpetual difference.
*/
public data class SecretVersionArgs(
public val secretBinary: Output? = null,
public val secretId: Output? = null,
public val secretString: Output? = null,
public val versionStages: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.secretsmanager.SecretVersionArgs =
com.pulumi.aws.secretsmanager.SecretVersionArgs.builder()
.secretBinary(secretBinary?.applyValue({ args0 -> args0 }))
.secretId(secretId?.applyValue({ args0 -> args0 }))
.secretString(secretString?.applyValue({ args0 -> args0 }))
.versionStages(versionStages?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [SecretVersionArgs].
*/
@PulumiTagMarker
public class SecretVersionArgsBuilder internal constructor() {
private var secretBinary: Output? = null
private var secretId: Output? = null
private var secretString: Output? = null
private var versionStages: Output>? = null
/**
* @param value Specifies binary data that you want to encrypt and store in this version of the secret. This is required if `secret_string` is not set. Needs to be encoded to base64.
*/
@JvmName("igddbwrsatrimqpg")
public suspend fun secretBinary(`value`: Output) {
this.secretBinary = value
}
/**
* @param value Specifies the secret to which you want to add a new version. You can specify either the Amazon Resource Name (ARN) or the friendly name of the secret. The secret must already exist.
*/
@JvmName("upcxmfngmfgjobuv")
public suspend fun secretId(`value`: Output) {
this.secretId = value
}
/**
* @param value Specifies text data that you want to encrypt and store in this version of the secret. This is required if `secret_binary` is not set.
*/
@JvmName("doiywbfwkcbhksjw")
public suspend fun secretString(`value`: Output) {
this.secretString = value
}
/**
* @param value Specifies a list of staging labels that are attached to this version of the secret. A staging label must be unique to a single version of the secret. If you specify a staging label that's already associated with a different version of the same secret then that staging label is automatically removed from the other version and attached to this version. If you do not specify a value, then AWS Secrets Manager automatically moves the staging label `AWSCURRENT` to this new version on creation.
* > **NOTE:** If `version_stages` is configured, you must include the `AWSCURRENT` staging label if this secret version is the only version or if the label is currently present on this secret version, otherwise this provider will show a perpetual difference.
*/
@JvmName("pgpdvgcxkroqdkco")
public suspend fun versionStages(`value`: Output>) {
this.versionStages = value
}
@JvmName("cfiqgsvtktootkmg")
public suspend fun versionStages(vararg values: Output) {
this.versionStages = Output.all(values.asList())
}
/**
* @param values Specifies a list of staging labels that are attached to this version of the secret. A staging label must be unique to a single version of the secret. If you specify a staging label that's already associated with a different version of the same secret then that staging label is automatically removed from the other version and attached to this version. If you do not specify a value, then AWS Secrets Manager automatically moves the staging label `AWSCURRENT` to this new version on creation.
* > **NOTE:** If `version_stages` is configured, you must include the `AWSCURRENT` staging label if this secret version is the only version or if the label is currently present on this secret version, otherwise this provider will show a perpetual difference.
*/
@JvmName("xqlttbgtecnjnjtp")
public suspend fun versionStages(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy