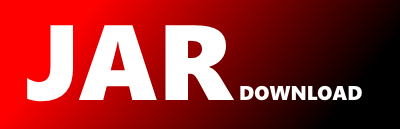
com.pulumi.aws.secretsmanager.kotlin.inputs.SecretReplicaArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.secretsmanager.kotlin.inputs
import com.pulumi.aws.secretsmanager.inputs.SecretReplicaArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property kmsKeyId ARN, Key ID, or Alias of the AWS KMS key within the region secret is replicated to. If one is not specified, then Secrets Manager defaults to using the AWS account's default KMS key (`aws/secretsmanager`) in the region or creates one for use if non-existent.
* @property lastAccessedDate Date that you last accessed the secret in the Region.
* @property region Region for replicating the secret.
* @property status Status can be `InProgress`, `Failed`, or `InSync`.
* @property statusMessage Message such as `Replication succeeded` or `Secret with this name already exists in this region`.
*/
public data class SecretReplicaArgs(
public val kmsKeyId: Output? = null,
public val lastAccessedDate: Output? = null,
public val region: Output,
public val status: Output? = null,
public val statusMessage: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.secretsmanager.inputs.SecretReplicaArgs =
com.pulumi.aws.secretsmanager.inputs.SecretReplicaArgs.builder()
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.lastAccessedDate(lastAccessedDate?.applyValue({ args0 -> args0 }))
.region(region.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 }))
.statusMessage(statusMessage?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretReplicaArgs].
*/
@PulumiTagMarker
public class SecretReplicaArgsBuilder internal constructor() {
private var kmsKeyId: Output? = null
private var lastAccessedDate: Output? = null
private var region: Output? = null
private var status: Output? = null
private var statusMessage: Output? = null
/**
* @param value ARN, Key ID, or Alias of the AWS KMS key within the region secret is replicated to. If one is not specified, then Secrets Manager defaults to using the AWS account's default KMS key (`aws/secretsmanager`) in the region or creates one for use if non-existent.
*/
@JvmName("oivvukwmqouidesm")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value Date that you last accessed the secret in the Region.
*/
@JvmName("guuogtxwilhgloif")
public suspend fun lastAccessedDate(`value`: Output) {
this.lastAccessedDate = value
}
/**
* @param value Region for replicating the secret.
*/
@JvmName("oqubhowwddtnrllx")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value Status can be `InProgress`, `Failed`, or `InSync`.
*/
@JvmName("pcvfekvkedllqrey")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value Message such as `Replication succeeded` or `Secret with this name already exists in this region`.
*/
@JvmName("axclkxapcgdrqbex")
public suspend fun statusMessage(`value`: Output) {
this.statusMessage = value
}
/**
* @param value ARN, Key ID, or Alias of the AWS KMS key within the region secret is replicated to. If one is not specified, then Secrets Manager defaults to using the AWS account's default KMS key (`aws/secretsmanager`) in the region or creates one for use if non-existent.
*/
@JvmName("jqefssqeokycpdqu")
public suspend fun kmsKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyId = mapped
}
/**
* @param value Date that you last accessed the secret in the Region.
*/
@JvmName("rahqyqohlcqyalni")
public suspend fun lastAccessedDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastAccessedDate = mapped
}
/**
* @param value Region for replicating the secret.
*/
@JvmName("oocggrbqxemyigut")
public suspend fun region(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value Status can be `InProgress`, `Failed`, or `InSync`.
*/
@JvmName("crejxwtrvmcisbiv")
public suspend fun status(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value Message such as `Replication succeeded` or `Secret with this name already exists in this region`.
*/
@JvmName("cpwlriieumxavugx")
public suspend fun statusMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.statusMessage = mapped
}
internal fun build(): SecretReplicaArgs = SecretReplicaArgs(
kmsKeyId = kmsKeyId,
lastAccessedDate = lastAccessedDate,
region = region ?: throw PulumiNullFieldException("region"),
status = status,
statusMessage = statusMessage,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy