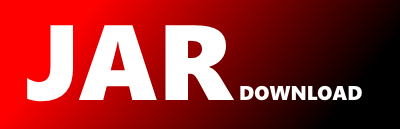
com.pulumi.aws.securityhub.kotlin.inputs.AutomationRuleActionFindingFieldsUpdateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.securityhub.kotlin.inputs
import com.pulumi.aws.securityhub.inputs.AutomationRuleActionFindingFieldsUpdateArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property confidence The rule action updates the `Confidence` field of a finding.
* @property criticality The rule action updates the `Criticality` field of a finding.
* @property note A resource block that updates the note. Documented below.
* @property relatedFindings A resource block that the rule action updates the `RelatedFindings` field of a finding. Documented below.
* @property severity A resource block that updates to the severity information for a finding. Documented below.
* @property types The rule action updates the `Types` field of a finding.
* @property userDefinedFields The rule action updates the `UserDefinedFields` field of a finding.
* @property verificationState The rule action updates the `VerificationState` field of a finding. The allowed values are the following `UNKNOWN`, `TRUE_POSITIVE`, `FALSE_POSITIVE` and `BENIGN_POSITIVE`.
* @property workflow A resource block that is used to update information about the investigation into the finding. Documented below.
*/
public data class AutomationRuleActionFindingFieldsUpdateArgs(
public val confidence: Output? = null,
public val criticality: Output? = null,
public val note: Output? = null,
public val relatedFindings: Output>? = null,
public val severity: Output? = null,
public val types: Output>? = null,
public val userDefinedFields: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy