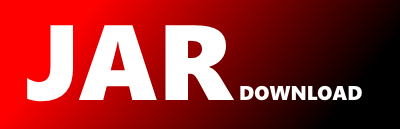
com.pulumi.aws.securityhub.kotlin.inputs.AutomationRuleCriteriaCriticalityArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.securityhub.kotlin.inputs
import com.pulumi.aws.securityhub.inputs.AutomationRuleCriteriaCriticalityArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property eq The equal-to condition to be applied to a single field when querying for findings, provided as a String.
* @property gt
* @property gte The greater-than-equal condition to be applied to a single field when querying for findings, provided as a String.
* @property lt
* @property lte The less-than-equal condition to be applied to a single field when querying for findings, provided as a String.
*/
public data class AutomationRuleCriteriaCriticalityArgs(
public val eq: Output? = null,
public val gt: Output? = null,
public val gte: Output? = null,
public val lt: Output? = null,
public val lte: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.securityhub.inputs.AutomationRuleCriteriaCriticalityArgs =
com.pulumi.aws.securityhub.inputs.AutomationRuleCriteriaCriticalityArgs.builder()
.eq(eq?.applyValue({ args0 -> args0 }))
.gt(gt?.applyValue({ args0 -> args0 }))
.gte(gte?.applyValue({ args0 -> args0 }))
.lt(lt?.applyValue({ args0 -> args0 }))
.lte(lte?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AutomationRuleCriteriaCriticalityArgs].
*/
@PulumiTagMarker
public class AutomationRuleCriteriaCriticalityArgsBuilder internal constructor() {
private var eq: Output? = null
private var gt: Output? = null
private var gte: Output? = null
private var lt: Output? = null
private var lte: Output? = null
/**
* @param value The equal-to condition to be applied to a single field when querying for findings, provided as a String.
*/
@JvmName("ghqnkbtxpneeurxa")
public suspend fun eq(`value`: Output) {
this.eq = value
}
/**
* @param value
*/
@JvmName("adtevwrrecqjoxwh")
public suspend fun gt(`value`: Output) {
this.gt = value
}
/**
* @param value The greater-than-equal condition to be applied to a single field when querying for findings, provided as a String.
*/
@JvmName("daixmtldywesbdgs")
public suspend fun gte(`value`: Output) {
this.gte = value
}
/**
* @param value
*/
@JvmName("tlyhaeembkutlood")
public suspend fun lt(`value`: Output) {
this.lt = value
}
/**
* @param value The less-than-equal condition to be applied to a single field when querying for findings, provided as a String.
*/
@JvmName("btoayvttkulrrejd")
public suspend fun lte(`value`: Output) {
this.lte = value
}
/**
* @param value The equal-to condition to be applied to a single field when querying for findings, provided as a String.
*/
@JvmName("oqycmotdgmegnpts")
public suspend fun eq(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eq = mapped
}
/**
* @param value
*/
@JvmName("dqgxpaftpscrcsnd")
public suspend fun gt(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gt = mapped
}
/**
* @param value The greater-than-equal condition to be applied to a single field when querying for findings, provided as a String.
*/
@JvmName("lsgrghvkdsbuauhp")
public suspend fun gte(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gte = mapped
}
/**
* @param value
*/
@JvmName("xivpabbwyhoykiol")
public suspend fun lt(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lt = mapped
}
/**
* @param value The less-than-equal condition to be applied to a single field when querying for findings, provided as a String.
*/
@JvmName("ktkwtnhnjqawngrd")
public suspend fun lte(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lte = mapped
}
internal fun build(): AutomationRuleCriteriaCriticalityArgs =
AutomationRuleCriteriaCriticalityArgs(
eq = eq,
gt = gt,
gte = gte,
lt = lt,
lte = lte,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy